🎮 项目实战 | 实现一套精确、可视化的游戏时间同步机制,让你的多人在线游戏摆脱"时间不一致"噩梦!
效果如图:
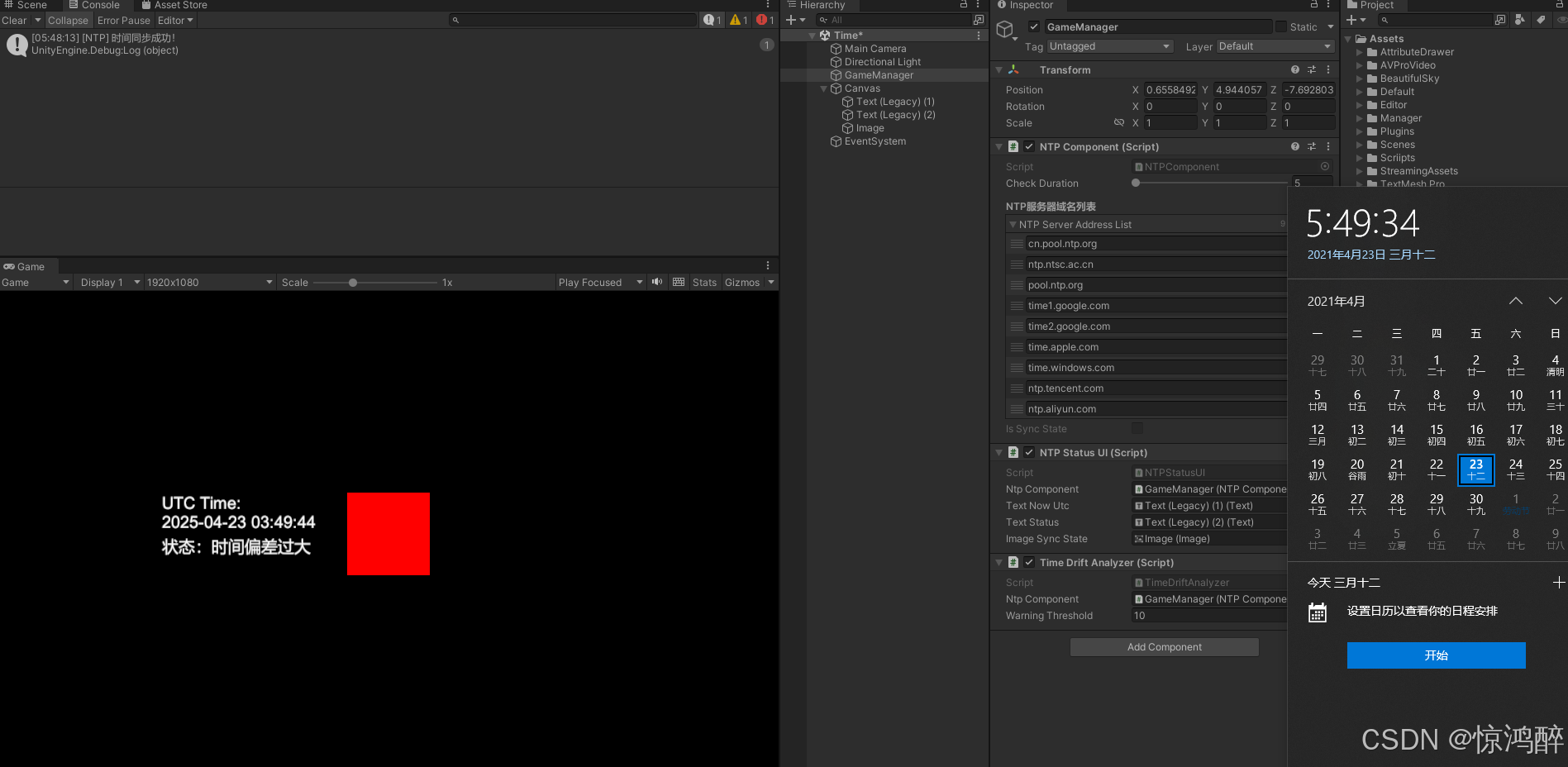
📌 一、前言:为什么不能只信本地时间?
在 Unity 游戏开发中,时间几乎参与了每一个核心系统:
- 日常签到系统;
- 限时活动触发;
- 多人 PVP 同步帧逻辑;
- 防作弊逻辑(例如加速器检测);
但系统时间不是你想信就能信的。比如:
- 用户手动修改手机时间就能无限领奖励;
- 不同设备系统时间不一致会造成数据写入乱序;
- 时区、平台、网络延迟都可能导致时间错乱。
因此:从可信网络获取统一时间源,是游戏后端逻辑稳定性的关键。
🌐 二、NTP 协议科普:啥是 NTP?
NTP,全称是 Network Time Protocol,用于同步设备与"世界标准时间 UTC"。
- 📡 使用 UDP 协议(123 端口)通信;
- 🕰️ 时间精度可达毫秒级;
- 🌍 支持全球公开服务器(例如 Google、阿里云、微软等);
- ✅ 可作为防篡改时间源;
🧱 三、核心功能一:网络时间同步组件 NTPComponent.cs
这个模块做了三件大事:
- 向多个 NTP 服务并发请求时间;
- 谁先返回就用谁的,更新
NowUtc
; - 每隔 N 秒自动刷新一次。
📦 完整源码如下:
cs
using System.Collections.Generic;
using UnityEngine;
using System;
using System.Net.Sockets;
using System.Net;
using System.Threading.Tasks;
using System.Linq;
namespace GameContent
{
[DisallowMultipleComponent]
public class NTPComponent : MonoBehaviour
{
[Range(5f, 60f)]
public float CheckDuration = 5f;
[Header("NTP服务器域名列表")]
public List<string> NTPServerAddressList = new List<string>
{
"cn.pool.ntp.org",
"ntp.ntsc.ac.cn",
"pool.ntp.org",
"time1.google.com",
"time2.google.com",
"time.apple.com",
"time.windows.com",
"ntp.tencent.com",
"ntp.aliyun.com"
};
public bool IsValid { get; private set; }
public DateTime NowUtc { get; private set; }
[ReadOnly] public bool IsSyncState = false;
private float mResidualCheckTime = 0f;
private void Start()
{
mResidualCheckTime = CheckDuration;
IsValid = false;
NowUtc = DateTime.UtcNow;
SearchNTPAddresses();
}
private void Update()
{
if (IsValid)
NowUtc = NowUtc.AddSeconds(Time.unscaledDeltaTime);
mResidualCheckTime -= Time.unscaledDeltaTime;
if (mResidualCheckTime <= 0)
{
mResidualCheckTime = CheckDuration;
SearchNTPAddresses();
}
}
public async void SearchNTPAddresses()
{
var tasks = NTPServerAddressList.Select(serverAddress =>
Task.Run(async () => await GetNetworkUtcTimeAsync(serverAddress, 2000))).ToArray();
while (tasks.Length > 0)
{
var completedTask = await Task.WhenAny(tasks);
DateTime networkDateTime = completedTask.Result;
if (networkDateTime != DateTime.MinValue)
{
bool oldState = IsValid;
IsValid = true;
NowUtc = networkDateTime;
TimeSpan diff = NowUtc - DateTime.UtcNow;
IsSyncState = Mathf.Abs((float)diff.TotalSeconds) <= 10;
if (!oldState)
{
Debug.Log("[NTP] 时间同步成功!");
}
return;
}
tasks = tasks.Where(task => task != completedTask).ToArray();
}
IsValid = false;
Debug.LogWarning("[NTP] 所有服务器请求失败!");
}
private async Task<DateTime> GetNetworkUtcTimeAsync(string ntpServer, int timeoutMilliseconds = 5000)
{
try
{
const int udpPort = 123;
var ntpData = new byte[48];
ntpData[0] = 0x1B;
var addresses = await Dns.GetHostAddressesAsync(ntpServer);
var ipEndPoint = new IPEndPoint(addresses[0], udpPort);
var socket = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp)
{
ReceiveTimeout = timeoutMilliseconds
};
await socket.ConnectAsync(ipEndPoint);
await socket.SendAsync(new ArraySegment<byte>(ntpData), SocketFlags.None);
var receiveBuffer = new byte[48];
await socket.ReceiveAsync(new ArraySegment<byte>(receiveBuffer), SocketFlags.None);
socket.Dispose();
const byte serverReplyTime = 40;
ulong intPart = BitConverter.ToUInt32(receiveBuffer, serverReplyTime);
ulong fractPart = BitConverter.ToUInt32(receiveBuffer, serverReplyTime + 4);
intPart = SwapEndianness(intPart);
fractPart = SwapEndianness(fractPart);
var milliseconds = (intPart * 1000) + ((fractPart * 1000) / 0x100000000L);
var networkUtcDateTime = new DateTime(1900, 1, 1).AddMilliseconds((long)milliseconds);
return networkUtcDateTime;
}
catch
{
return DateTime.MinValue;
}
}
private uint SwapEndianness(ulong x)
{
return (uint)(
((x & 0x000000ff) << 24) +
((x & 0x0000ff00) << 8) +
((x & 0x00ff0000) >> 8) +
((x & 0xff000000) >> 24));
}
}
}
🎨 四、核心功能二:实时 UI 显示状态 NTPStatusUI.cs
✅ 展示功能:
- 当前 UTC 时间;
- 是否同步成功;
- 时间误差是否超出阈值;
- 可视化状态颜色指示(红绿黄三色灯);
📋 完整源码如下:
cs
using UnityEngine;
using UnityEngine.UI;
using System;
namespace GameContent
{
public class NTPStatusUI : MonoBehaviour
{
public NTPComponent ntpComponent;
public Text textNowUtc;
public Text textStatus;
public Image imageSyncState;
private void Update()
{
if (ntpComponent == null) return;
textNowUtc.text = $"UTC Time: {ntpComponent.NowUtc:yyyy-MM-dd HH:mm:ss}";
if (!ntpComponent.IsValid)
{
textStatus.text = "状态:正在同步...";
imageSyncState.color = Color.yellow;
}
else if (ntpComponent.IsSyncState)
{
textStatus.text = "状态:同步成功 ";
imageSyncState.color = Color.green;
}
else
{
textStatus.text = "状态:时间偏差过大 ";
imageSyncState.color = Color.red;
}
}
}
}
📊 五、核心功能三:时间偏差统计 TimeDriftAnalyzer.cs
这个组件用于记录并分析:每次系统时间 vs NTP 时间之间的偏差情况。
✅ 功能概览:
- 实时记录时间误差;
- 计算平均偏差、最大偏差;
- 日志输出偏差超标的记录。
📋 完整源码如下:
cs
using UnityEngine;
using System;
using System.Collections.Generic;
using System.Linq;
namespace GameContent
{
public class TimeDriftAnalyzer : MonoBehaviour
{
public NTPComponent ntpComponent;
public float warningThreshold = 10f;
private List<float> driftRecords = new List<float>();
private void Update()
{
if (ntpComponent == null || !ntpComponent.IsValid)
return;
float drift = Mathf.Abs((float)(ntpComponent.NowUtc - DateTime.UtcNow).TotalSeconds);
driftRecords.Add(drift);
if (drift > warningThreshold)
{
Debug.LogWarning($"[DriftAnalyzer] 时间偏差过大:{drift:F2} 秒");
}
}
public float GetAverageDrift() =>
driftRecords.Count == 0 ? 0f : Mathf.Round(driftRecords.Average() * 100f) / 100f;
public float GetMaxDrift() =>
driftRecords.Count == 0 ? 0f : Mathf.Round(driftRecords.Max() * 100f) / 100f;
public int GetDriftCount() => driftRecords.Count;
}
}
💡 最后的话
这个方案已经被用于我的多人项目中,强烈建议你把它接入到你的时间相关模块。毕竟,精准的时间,是一切游戏逻辑的根基。