1.准备数据
使用之前创建的springbootdata的数据库,该数据库有两个表t_article和t_comment,这两个表预先插入几条测试数据。
2.编写数据库表对应的实体类
java
@Entity(name = "t_comment")
public class Comment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
private String content;
private String author;
@Column(name = "a_id")
private Integer aId;
//补充get set toString
}
3.创建CommentRepository
java
public interface CommentRepository extends JpaRepository<Comment,Integer>{
@Transactional
@Modifying
@Query("UPDATE t_comment c SET c.author= ?1 WHERE c.id = ?2")
public int updateComment(String author,Integer id);
}
4.创建CommentService
在该类中编写数据的查询、修改和删除操作
java
@Service
public class CommentService {
@Autowired
private CommentRepository commentRepository;
public Comment findById(int comment_id){
Optional<Comment> optional = commentRepository.findById(comment_id);
if(optional.isPresent()){
return optional.get();
}
return null;
}
public Comment updateComment(Comment comment){
commentRepository.updateComment(comment.getAuthor(), comment.getId());
return comment;
}
public void deleteComment(int comment_id){
commentRepository.deleteById(comment_id);
}
}
5.创建 CommentController
使用注入的CommentService实例对象编写对Comment评论数据的查询、修改和删除方法。
java
@RestController
public class CommentController {
@Autowired
private CommentService commentService;
@GetMapping("/get/{id}")
public Comment findById(@PathVariable("id") int comment_id) {
Comment comment = commentService.findById(comment_id);
return comment;
}
@GetMapping("/update/{id}/{author}")
public Comment updateComment(@PathVariable("id") int comment_id, @PathVariable("author") String author) {
Comment comment = commentService.findById(comment_id);
comment.setAuthor(author);
Comment updateComment = commentService.updateComment(comment);
return updateComment;
}
@GetMapping("/delete/{id}")
public void deleteComment(@PathVariable("id") int comment_id) {
commentService.deleteComment(comment_id);
}
}
6.设置配置信息
spring.datasource.url=jdbc:mysql://localhost:3306/springbootdata?serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=root
spring.jpa.show-sql=true
7.项目测试
项目启动成功后,在浏览器上访问http://localhost:8080/get/1,浏览器每刷新一次,控制台会新输出一条SQL语句
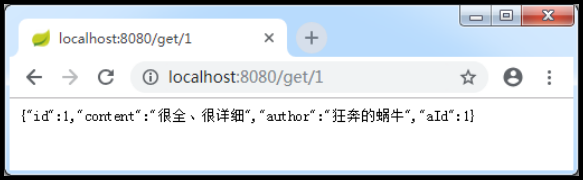
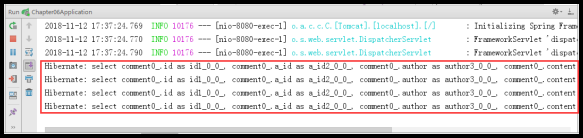
每执行一次查询操作,都会访问一次数据库并执行一次SQL语句。
8、 启动类上添加@EnableCaching注解
开启基于注解的缓存支持
java
//启动类
@EnableCaching
@SpringBootApplication
public class Chapter06Application {
public static void main(String[] args) {
SpringApplication.run(Chapter06Application.class, args);
}
}
9、使用@Cacheable注解对数据操作方法进行缓存管理
//业务层
@Cacheable(cacheNames = "comment")
public Comment findById(int comment_id){
Optional<Comment> optional = commentRepository.findById(comment_id);
if(optional.isPresent()){
return optional.get();
}
return null;
}
10、Spring Boot默认缓存测试
项目启动成功后,在浏览器上访问http://localhost:8080/get/1,不论浏览器刷新多少次,页面的查询结果都会显示同一条数据
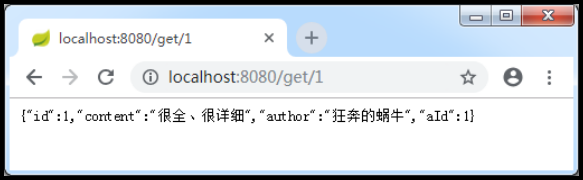
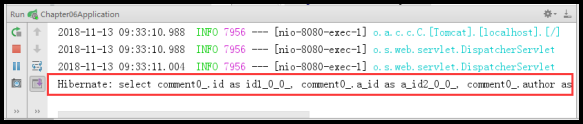
重复进行同样的查询操作,数据库只执行了一次SQL查询语句,说明项目开启的默认缓存支持已经生效。