目录
引言
Qt6 Quick框架提供了一套丰富的日历相关组件,包括 MonthGrid
、DayOfWeekRow
和 WeekNumberColumn
,使开发者能够轻松实现各种日历功能。本文将通过一个简单的日历应用示例,展示如何组合这些组件创建一个完整的日历界面。
相关阅读
在开始实现日历应用前,我们需要了解以下几个Qt Quick Controls中的核心日历组件:
1. DayOfWeekRow
DayOfWeekRow是一个用于显示星期几标题的组件,通常作为日历的标题行。它可以根据不同的地区设置(locale)自动调整显示格式,支持从周日或周一开始的不同显示方式。
主要属性包括:
locale
: 设置地区,影响星期名称的显示month
: 设置月份(0-11)year
: 设置年份delegate
: 自定义每个星期标题的显示样式
2. MonthGrid
MonthGrid是日历的核心组件,用于显示一个月的日期网格。它提供了显示日期、处理日期选择和导航等基本功能。
主要属性包括:
month
: 设置显示的月份(0-11)year
: 设置显示的年份locale
: 设置地区,影响日期的显示格式delegate
: 自定义每个日期单元格的显示样式title
: 月份标题
月份定义:
3. WeekNumberColumn
WeekNumberColumn用于在日历旁边显示周数(第几周),通常与MonthGrid结合使用。周数的计算方式取决于locale设置。
主要属性包括:
month
: 设置月份,与MonthGrid对应year
: 设置年份,与MonthGrid对应locale
: 设置地区,影响周数的计算方式delegate
: 自定义周数显示的样式
项目结构及实现
工程结构图
qml_calendar项目 main.cpp Main.qml components MonthGridComponent.qml DayOfWeekRowComponent.qml WeekNumberColumnComponent.qml CMakeLists.txt
代码实现及解析
1. 组件封装
首先,我们将三个基础日历组件封装成单独的QML文件,便于复用和维护。
MonthGridComponent.qml
qml
import QtQuick
import QtQuick.Controls
MonthGrid {
id: monthGrid
locale: Qt.locale("zh_CN") // 本地化设置
delegate: Text { // 自定义日期显示
text: model.day
horizontalAlignment: Text.AlignHCenter
verticalAlignment: Text.AlignVCenter
}
}
这个组件封装了月份网格,设置了中文本地化,自定义了日期显示样式。
DayOfWeekRowComponent.qml
qml
import QtQuick
import QtQuick.Controls
DayOfWeekRow {
locale: Qt.locale("zh_CN")
delegate: Text {
text: model.shortName
font.bold: true
horizontalAlignment: Text.AlignHCenter
}
}
这个组件封装了星期标题行,同样使用中文本地化,加粗显示星期名称。
WeekNumberColumnComponent.qml
qml
import QtQuick
import QtQuick.Controls
WeekNumberColumn {
locale: Qt.locale("zh_CN")
delegate: Text {
text: model.weekNumber
horizontalAlignment: Text.AlignHCenter
verticalAlignment: Text.AlignVCenter
}
}
这个组件封装了周数列,显示每周的周数。
2. 主界面实现
Main.qml
qml
import QtQuick
import QtQuick.Controls
import "components"
ApplicationWindow {
visible: true
width: 600
height: 500
property date currentDate: new Date()
property int currentMonth: currentDate.getMonth()
property int currentYear: currentDate.getFullYear()
Column {
anchors.fill: parent
anchors.margins: 20
spacing: 10
// 月份导航
Row {
spacing: 10
anchors.horizontalCenter: parent.horizontalCenter
Button {
text: "<"
onClicked: {
if (currentMonth === 0) {
currentMonth = 11
currentYear--
} else {
currentMonth--
}
}
}
Label {
text: Qt.locale().standaloneMonthName(currentMonth) + " " + currentYear
font.pixelSize: 18
font.bold: true
}
Button {
text: ">"
onClicked: {
if (currentMonth === 11) {
currentMonth = 0
currentYear++
} else {
currentMonth++
}
}
}
}
// 日历主体
Row {
spacing: 10
// 周数列
WeekNumberColumnComponent {
width: 40
height: monthGrid.height
month: currentMonth
year: currentYear
delegate: Rectangle {
implicitWidth: 40
implicitHeight: 40
color: "transparent"
Text {
text: model.weekNumber
anchors.centerIn: parent
color: "gray"
}
}
}
Column {
spacing: 5
// 星期标题行
DayOfWeekRowComponent {
width: monthGrid.width
height: 40
delegate: Rectangle {
implicitWidth: 40
implicitHeight: 40
color: "#f0f0f0"
Text {
text: model.shortName
anchors.centerIn: parent
font.bold: true
}
}
}
// 月历网格
MonthGridComponent {
id: monthGrid
month: currentMonth
year: currentYear
delegate: Rectangle {
implicitWidth: 40
implicitHeight: 40
color: {
if (!model.day)
return "transparent"
if (model.today)
return "#e6f3ff"
if (model.month === monthGrid.month)
return "white"
return "#f9f9f9"
}
border.color: "#e0e0e0"
Text {
text: model.day
anchors.centerIn: parent
color: {
if (!model.day)
return "transparent"
if (model.month !== monthGrid.month)
return "gray"
return "black"
}
font.bold: model.today
}
MouseArea {
anchors.fill: parent
enabled: model.day && model.month === monthGrid.month
onClicked: console.log("选择的日期:", model.date.toLocaleDateString())
}
}
}
}
}
}
}
主界面实现了以下功能:
日期属性定义:
- 使用属性定义当前日期、月份和年份,作为整个日历的数据源
月份导航:
- 通过两个按钮实现上个月和下个月的切换功能
日历主体布局:
- 左侧是周数列(WeekNumberColumnComponent)
- 右侧顶部是星期标题行(DayOfWeekRowComponent)
- 右侧主体是月份网格(MonthGridComponent)
日期单元格样式:
- 当前日期高亮显示(浅蓝色背景)
- 非当前月份的日期显示为灰色
- 每个日期单元格都有鼠标点击事件
运行效果
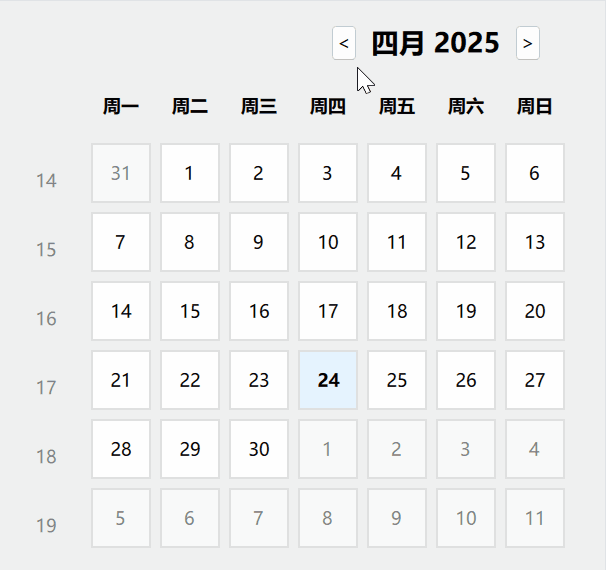
总结
通过本文,学习了如何使用Qt Quick Controls提供的日历组件(DayOfWeekRow、MonthGrid和WeekNumberColumn)来构建一个功能完整的日历应用。我们将这些组件封装成可复用的QML文件,然后在主界面中组合使用,实现了一个具有月份导航、日期显示和选择功能的日历。
下载链接
完整的工程代码可以从Gitcode下载:GitCode -> QML日历示例
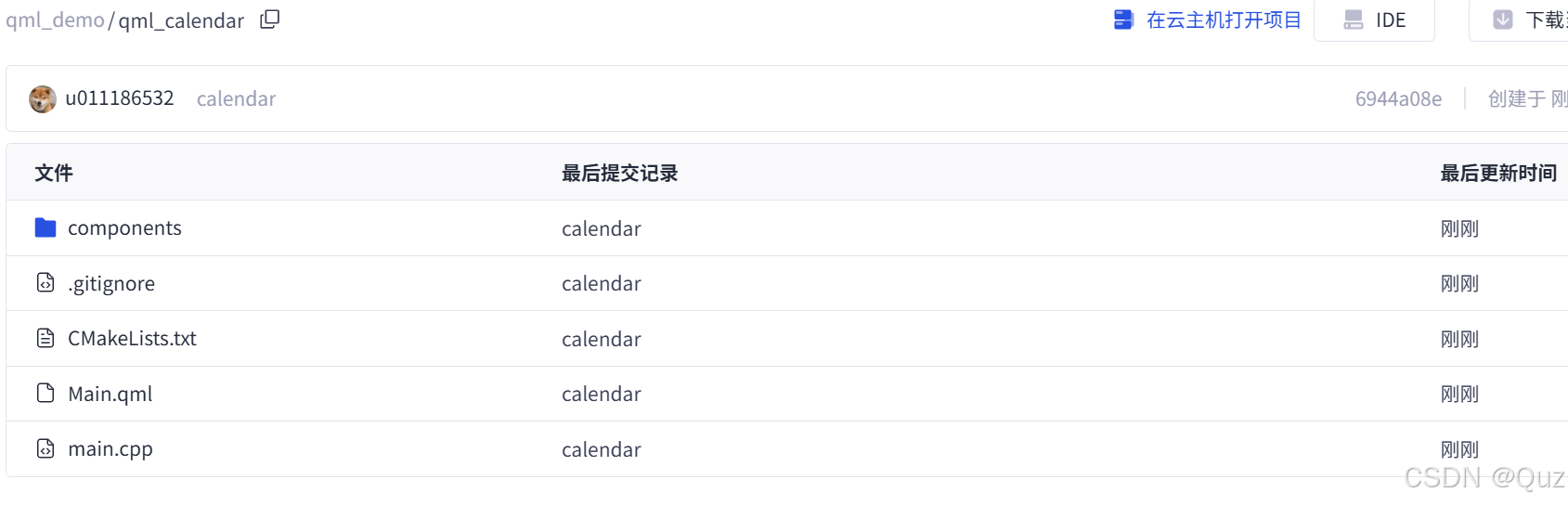