本章主要学习知识点
- 掌握如何拉伸二维平面为三维物体
- 掌握如何通过扫描生成物体
- 了解多边形轮廓概念
- 掌握如何实现多边形圆弧
- 掌握如何实现多边形内孔
拉伸
「拉伸」
功能可以理解为将二维图形「变厚」成三维模型,就像把一张纸变成一块砖头。
垂直拉伸(ExtrudeGeometry)------像「挤牙膏」
将一个2D图形(如圆形、五角星)沿着垂直方向拉高,形成3D物体。
js
const shape = new THREE.Shape([
new THREE.Vector2(-2,-2),
new THREE.Vector2(-2,2),
new THREE.Vector2(2,2),
new THREE.Vector2(2,-2)
])
const geometry = new THREE.ExtrudeGeometry(
shape,
{
depth: 12, // 拉伸长度
bevelThickness: 2, // 倒角尺寸 拉伸方向
bevelSize: 2, // 倒角尺寸 垂直拉伸方向
bevelSegments: 50, // 倒圆角
bevelEnabled: true, // 禁止倒角 默认true
// bevelSegments: 1 // 倒直角
}
)
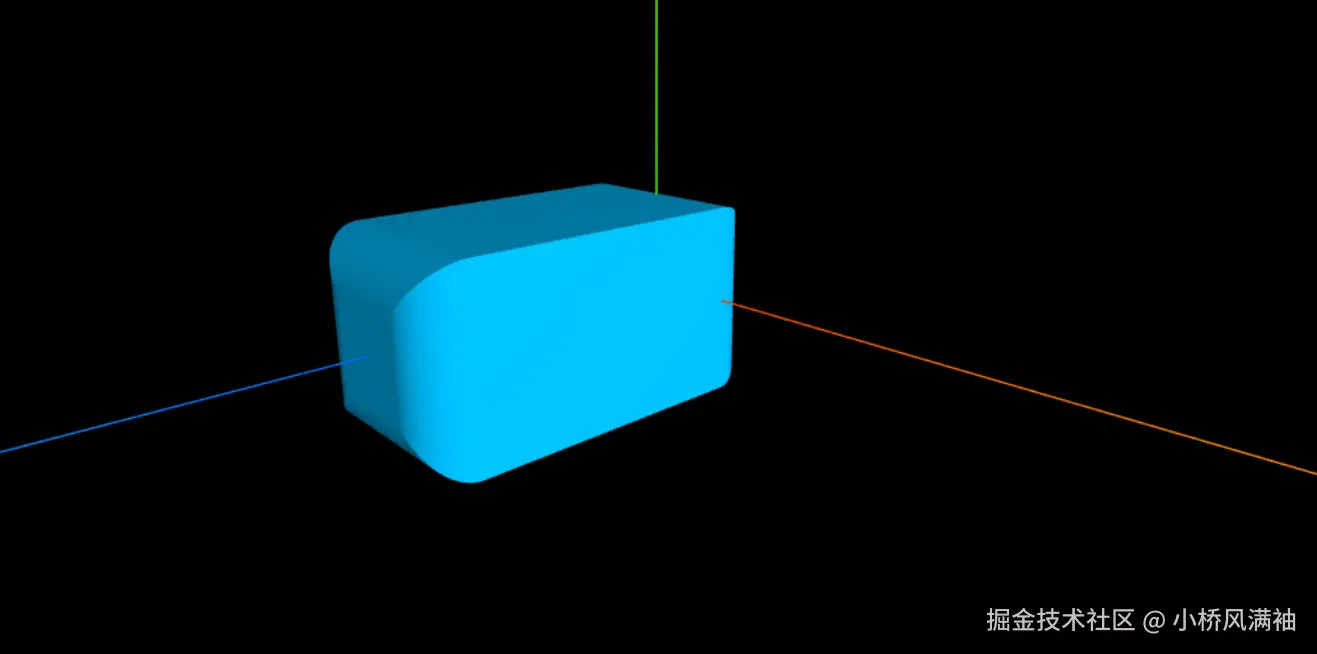
沿路径拉伸(TubeGeometry)------像「捏橡皮泥」
让2D图形沿着一条弯曲的路径移动,形成管道或弹簧。
扫描
扫描有两种常见的形式:一种是几何体生成方式 (如将2D图形拉伸为3D模型),另一种是动态视觉特效(如雷达波扩散)。
几何体扫描(类似3D打印)
这就就像是用打印机喷头沿着路径移动堆积材料,Three.js 可以将一个平面图形(如圆形、五角星)沿着特定轨迹"扫"成3D形状。
创建一个平面形状
js
const shape = new THREE.Shape([
new THREE.Vector2(0,0),
new THREE.Vector2(0,2),
new THREE.Vector2(2,2),
new THREE.Vector2(2,0)
])
通过CatmullRomCurve3
创建路径
js
const curve = new THREE.CatmullRomCurve3([
// 定义曲线的四个控制点
new THREE.Vector3(-1,-5,-5),
new THREE.Vector3(1,0,0),
new THREE.Vector3(0.8,5,5),
new THREE.Vector3(-5,3,12)
])
进行扫描
js
const geometry = new THREE.ExtrudeGeometry(
shape,{
extrudePath: curve, // 扫描轨迹
steps: 100, // 扫描步数
bevelEnabled: false, // 是否开启倒角
}
)
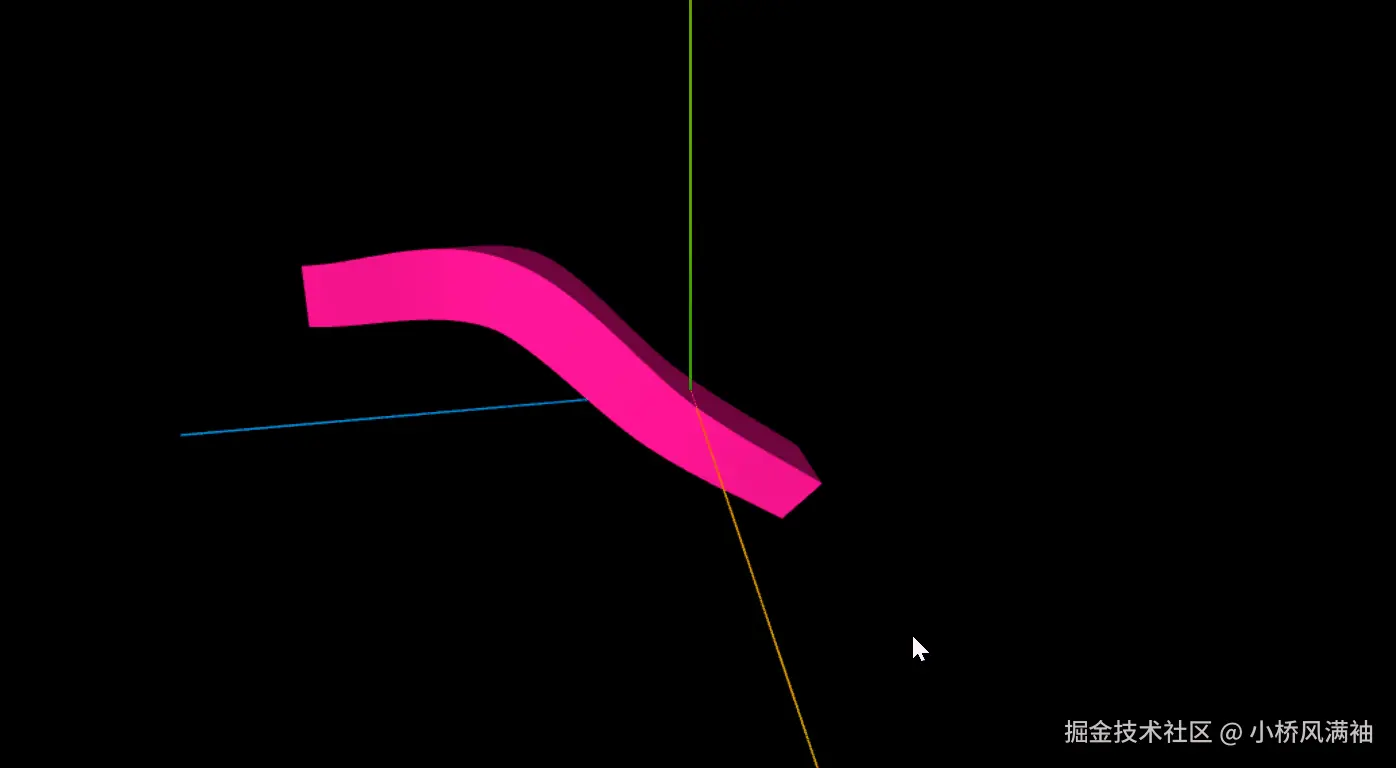
多边形轮廓简介
「多边形轮廓」
可以理解为通过一组二维顶点坐标生成平面或立体形状的边缘线条,类似用绳子绕着一圈钉子勾勒出形状。
下面是一个简单的例子
js
const shape = new THREE.Shape();
shape.moveTo(5,0)
shape.lineTo(5,5)
shape.lineTo(0,5)
shape.lineTo(3,0)
const geometry = new THREE.ExtrudeGeometry(shape, {
depth: 12 // 拉伸长度
})
const material = new THREE.MeshBasicMaterial({color: 'deeppink'})
const mesh = new THREE.Mesh(geometry,material)
scene.add(mesh)
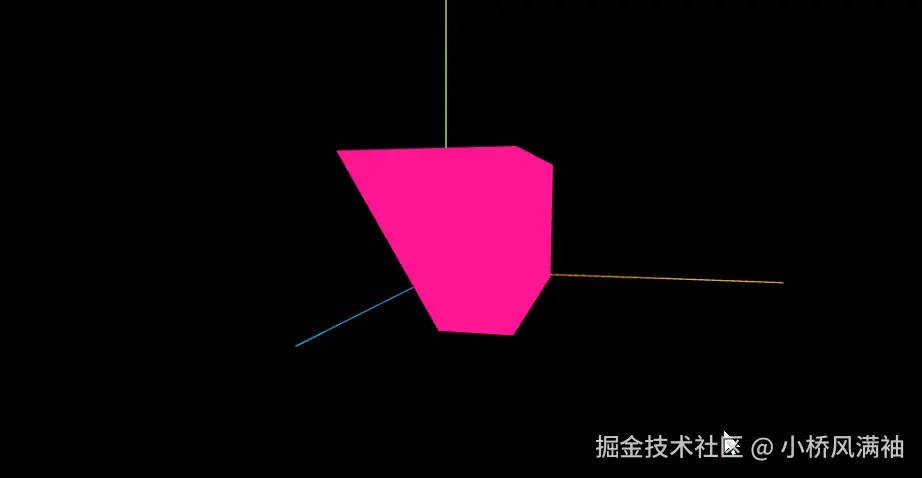
多边形轮廓圆弧
轮廓圆弧可以理解为将多边形的尖角变成圆角,或者让多边形沿着圆弧路径延展,就像把折纸的棱角用圆规修圆一样。
使用arc
绘制圆弧
js
const shape = new THREE.Shape();
shape.lineTo(15,0);
// 绘制一个圆弧,圆心坐标为(-5,0),半径为5,起始角度为0,结束角度为Math.PI/2,逆时针绘制
shape.arc(-5,0,5,0,Math.PI/2,false);
shape.lineTo(0,5);
scene.add(shape)
添加另一个shape进行对比
js
const shape2 = new THREE.Shape();
shape2.lineTo(10,0);
shape2.absarc(12,0,5,0,Math.PI/2,false);
shape2.lineTo(0,5);
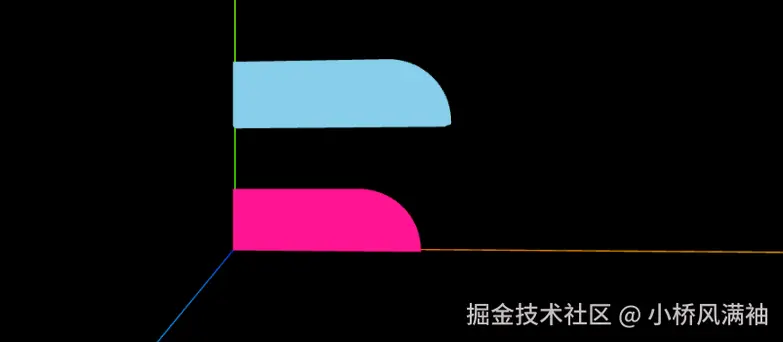
多边形内孔
「多边形内孔」
可以理解为在平面或立体模型上挖出镂空区域,就像在一张纸上剪出一个洞,或者把3D模型内部掏空。
创建一个形状
js
const shape = new THREE.Shape();
shape.lineTo(10,0)
shape.lineTo(10,10)
shape.lineTo(0,10)
通过Path
创建内孔路径,这里创建一个大圆和一个小圆,以及一个矩形
js
// 内孔轮廓
const path1 = new THREE.Path();
path1.absarc(2,2,2);
const path2 = new THREE.Path();
// 绘制一个半径为1,圆心坐标为(8,2)的圆弧
path2.absarc(8,2,1);
// 方形孔
const path3 = new THREE.Path();
path3.moveTo(8,4)
path3.lineTo(8,8)
path3.lineTo(4,8)
path3.lineTo(4,4)
最后将path1
、path2
、path3
添加到shape.holes
数组中
js
shape.holes.push(path1, path2, path3)
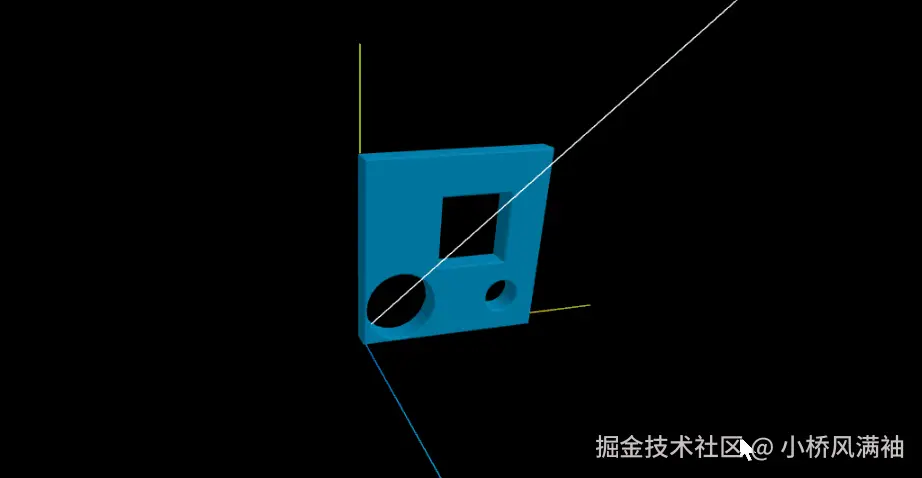
以上案例均可在案例中心查看体验
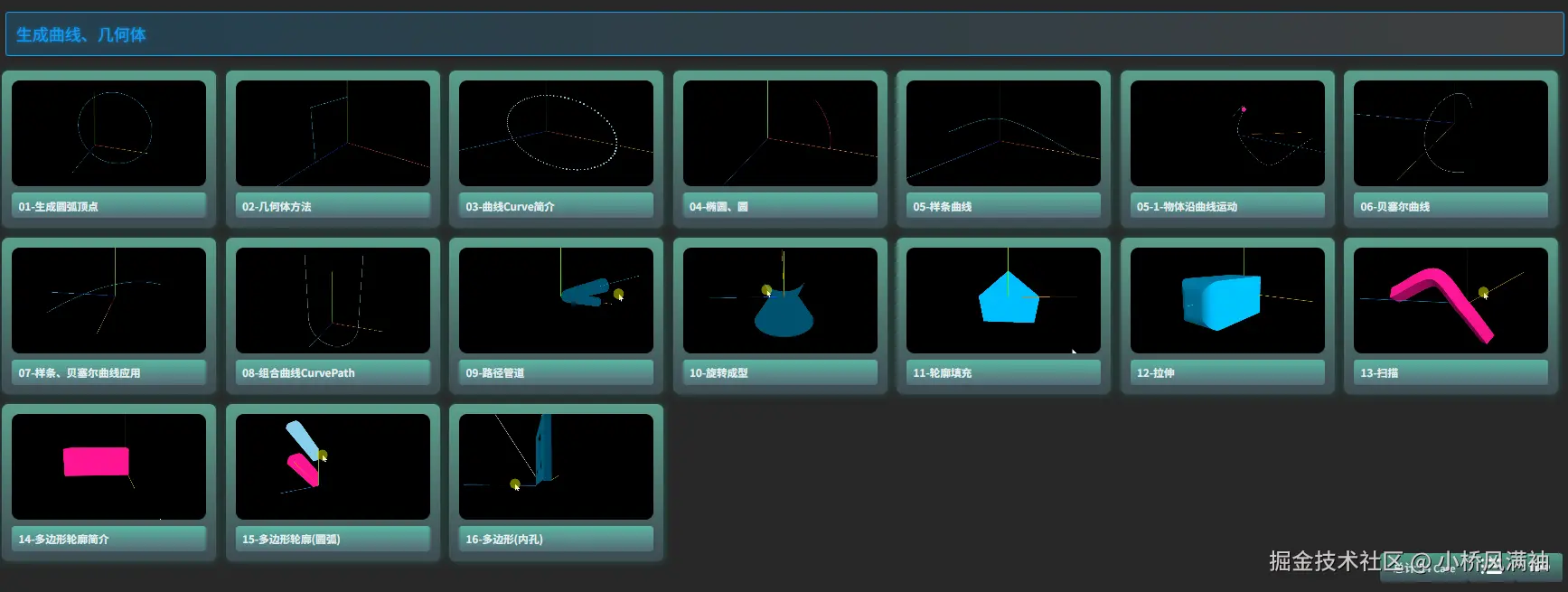