har库导入:
{
"license": "",
"devDependencies": {},
"author": "",
"name": "entry",
"description": "Please describe the basic information.",
"main": "",
"version": "1.0.0",
"dependencies": {
"@ohos/DonseeDevice": "file:./src/libs/DonseeDeviceLib.har"
}
}
导入身份证阅读器相关类:
import CommonContants from '../common/CommonContants';
import DonseeDevice from '@ohos/DonseeDevice/src/main/ets/model/DonseeDevice';
import { IDCardInfor } from '@ohos/DonseeDevice/src/main/ets/model/IDCardInfor';
导入社保卡读卡器相关类:
import CommonContants from '../common/CommonContants';
import DonseeDevice from '@ohos/DonseeDevice/src/main/ets/model/DonseeDevice';
import { IDCardInfor } from '@ohos/DonseeDevice/src/main/ets/model/IDCardInfor';
import { SSCardInfor } from '@ohos/DonseeDevice/src/main/ets/model/SSCardInfor';
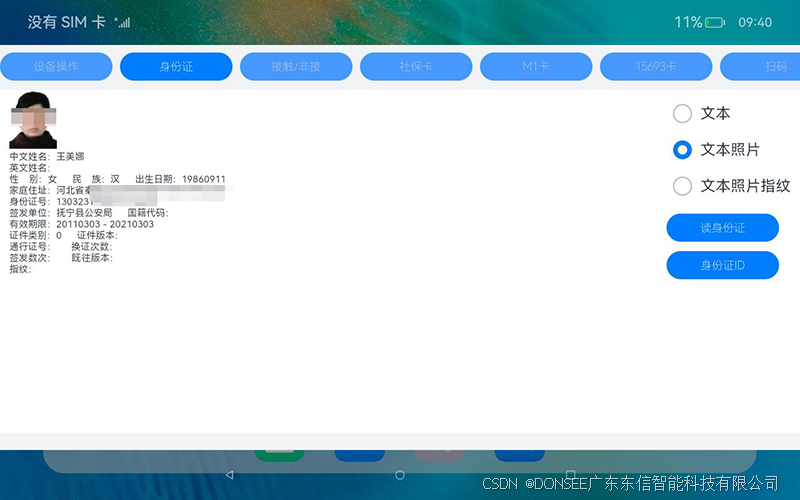
身份证阅读器调用相关代码:
import CommonContants from '../common/CommonContants';
import DonseeDevice from '@ohos/DonseeDevice/src/main/ets/model/DonseeDevice';
import { IDCardInfor } from '@ohos/DonseeDevice/src/main/ets/model/IDCardInfor';
/**
* Card content contains the information list.
*/
@Component
export struct IDCardComponent {
@State tvResult: string = '';
@State imgBase64: string = '';
@State imageVisible: Visibility = Visibility.None;
@State nType : number = 1;//0,文本信息;1,文本+照片;2,文本+照片+指纹
@Provide showSelector: boolean = false // 是否展开下拉菜单
// @Provide modesData: [number,string] = [{id: 1,name: '文本信息'},{id: 2,name: '文本照片'},{id: 3,name: '文本照片指纹'}]
@Provide modeId: number = 0 // 当前选中项id
build() {
Column() {
Row() {
Column() {
Image(this.imgBase64)
.visibility(this.imageVisible)
.width(51)
.height(63)
.objectFit(ImageFit.Contain)
Text(this.tvResult)
.fontSize(10)
.margin({ top: 2 })
}
.layoutWeight(1)
.margin({left:10})
.alignItems(HorizontalAlign.Start)
Column() {
Column() {
Row() {
Radio({ value: "文本", group: "1234" })
.checked(this.nType === 0 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.nType = 0;
})
Text('文本')
}.margin({ left: 10 })
Row() {
Radio({ value: "文本照片", group: "1234" })
.checked(this.nType === 1 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.nType = 1;
console.info("Radio onClick")
})
Text('文本照片')
}.margin({ left: 10 })
Row() {
Radio({ value: "文本照片指纹", group: "1234" })
.checked(this.nType === 2 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.nType = 2;
console.info("Radio onClick")
})
Text('文本照片指纹')
}.margin({ left: 10 })
}.justifyContent(FlexAlign.Start)
.alignItems(HorizontalAlign.Start)
Button("读身份证")
.fontSize($r('app.float.submit_button_font_size'))
.fontWeight(CommonContants.FONT_WEIGHT)
.height(30)
.width(120)
.onClick(() => {
let idInfo:IDCardInfor = DonseeDevice.Donsee_ReadIDCard(this.nType)
if(idInfo.ENfullnameOther.length>0){
idInfo.ENfullname += idInfo.ENfullnameOther
}
this.tvResult =
"中文姓名:"+ idInfo.name+" "
+"英文姓名:"+ idInfo.ENfullname+"\n"
+"性 别:"+ idInfo.sex+" "
+"民 族:"+ idInfo.people+" "
+"出生日期:"+ idInfo.birthday+"\n"
+"家庭住址:"+ idInfo.address+"\n"
+"身份证号:"+ idInfo.number+"\n"
+"签发单位:"+ idInfo.organs+" "
+"国籍代码:"+ idInfo.nationality+"\n"
+"有效期限:"+ idInfo.signdate+" - "+ idInfo.validterm+"\n"
+"证件类别:"+ idInfo.certType+" "
+"证件版本:"+ idInfo.certVersion+"\n"
+"通行证号:"+ idInfo.passNu+" "
+"换证次数:"+ idInfo.changCount+"\n"
+"签发数次:"+ idInfo.signCount+" "
+"既往版本:"+ idInfo.oldNumber+"\n"
+"指纹:"+ idInfo.figData+"\n"
if(idInfo.imgBase64.length>0){
this.imgBase64 = 'data:image/png;base64,'+idInfo.imgBase64
this.imageVisible = Visibility.Visible
}else{
this.imageVisible = Visibility.None
}
}else{
this.imgBase64 = ''
this.tvResult = "读取失败:"+ idInfo.result
}
}).margin({top:10})
Button("身份证ID")
.fontSize($r('app.float.submit_button_font_size'))
.fontWeight(CommonContants.FONT_WEIGHT)
.height(30)
.width(120)
.onClick(() => {
let datas:[number,string] = DonseeDevice.Donsee_ReadIDCardUid()
if(datas[0] == 0){
this.tvResult = "Uid:"+ datas[1]
}else{
this.tvResult = "Uid读取失败:"+ datas[0]
}
}).margin({top:10})
}.justifyContent(FlexAlign.End)
.margin({right:10})
}.justifyContent(FlexAlign.Start)
.width(CommonContants.FULL_PARENT)
}.justifyContent(FlexAlign.Start)
.backgroundColor($r('app.color.card_background'))
.width(CommonContants.FULL_PARENT)
.height(CommonContants.FULL_PARENT)
}
// 获取选中项的内容
// getSelectedText() {
// const selectedItem = this.modesData.find(item => {
// console.info('this.modeId==='+this.modeId)
// console.info('item.id==='+item.id)
// return item.id == this.modeId
// })
// if (selectedItem) {
// console.info('selectedItem.name==='+selectedItem.name)
// return selectedItem.name
// }
// return ''
// }
}
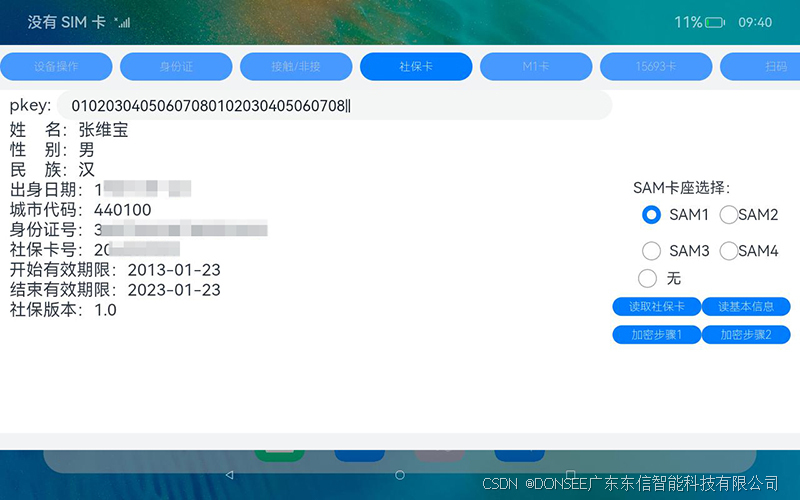
社保卡读卡器相关调用代码:
import CommonContants from '../common/CommonContants';
import DonseeDevice from '@ohos/DonseeDevice/src/main/ets/model/DonseeDevice';
import { IDCardInfor } from '@ohos/DonseeDevice/src/main/ets/model/IDCardInfor';
import { SSCardInfor } from '@ohos/DonseeDevice/src/main/ets/model/SSCardInfor';
import util from '@ohos.util';
/**
* Card content contains the information list.
*/
@Component
export struct SSCardComponent {
@State tvResult: string = '';
@State pKkey: string = '01020304050607080102030405060708|01020304050607080102030405060708|';
@State slot : number = 0;//卡座
private textInputController: TextInputController = new TextInputController();
build() {
Column() {
Row() {
Column() {
Row() {
Text("pkey:")
.fontSize(18)
TextInput({ controller: this.textInputController,text:'01020304050607080102030405060708|01020304050607080102030405060708|' })
.type(InputType.Normal)
.height(32)
.margin({ left: $r('app.float.text_input_margin_left') })
.layoutWeight(CommonContants.TEXTINPUT_LAYOUT_WEIGHT)
.onChange(value => {
this.pKkey = value
})
}
Text(this.tvResult)
.fontSize(18)
Text("").layoutWeight(1)
}
.layoutWeight(1)
.margin({left:10})
.alignItems(HorizontalAlign.Start)
Column() {
Column() {
Row() {
Text('SAM卡座选择:')
}.margin({ left: 10 })
Row() {
Radio({ value: "SCard", group: "3" })
.checked(this.slot === 1 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.slot = 1;
console.info("Radio onClick")
})
Text('SAM1')
Radio({ value: "SCard", group: "3" })
.checked(this.slot === 2 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.slot = 2;
console.info("Radio onClick")
}).margin({ left: 10 })
Text('SAM2')
}.margin({ left: 10 })
Row() {
Radio({ value: "SCard", group: "3" })
.checked(this.slot === 3 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.slot = 3;
console.info("Radio onClick")
})
Text('SAM3')
Radio({ value: "SCard", group: "3" })
.checked(this.slot === 4 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.slot = 4;
console.info("Radio onClick")
}).margin({ left: 10 })
Text('SAM4')
}.margin({ left: 10 })
Row() {
Radio({ value: "SCard", group: "3" })
.checked(this.slot === 0 ? true : false)
.height(20)
.width(20)
.onClick(() => {
this.slot = 0;
console.info("Radio onClick")
}).margin({ left: 15 })
Text('无').margin({ left: 10 })
}
}.justifyContent(FlexAlign.Start)
.alignItems(HorizontalAlign.Start)
Row() {
Button("读取社保卡")
.fontSize($r('app.float.submit_button_font_size'))
.fontWeight(CommonContants.FONT_WEIGHT)
.height(20)
.width(95)
.onClick(() => {
//nType 1, 有SAM卡返回全部信息 2, 无SAM卡返回卡号
let nType:number = 1
if (this.slot == 0) {
nType = 2
}
console.info("nType = " + nType)
console.info("this.slot = " + this.slot)
let ssCardInfor: SSCardInfor = DonseeDevice.Donsee_ReadSSCardPre(this.slot, nType)
console.info("ssCardInfor.result = " + ssCardInfor.result)
if (ssCardInfor.result == 0) {
// const str = new util.TextDecoder('gbk').decode(ssCardInfor.name)
// let gbkDecoder = new util.TextDecoder("gbk",{ignoreBOM: true});
console.info("ssCardInfor.name = " + ssCardInfor.nation)
console.info("ssCardInfor.name = " + ssCardInfor.name)
// this.tvResult = "姓 名:"+ ssCardInfor.name
this.tvResult = "姓 名:" + ssCardInfor.name + "\n"
-
"性 别:" + ssCardInfor.sex + "\n"
-
"民 族:" + ssCardInfor.nation + "\n"
-
"出身日期:" + ssCardInfor.birthday + "\n"
-
"城市代码:" + ssCardInfor.city + "\n"
-
"身份证号:" + ssCardInfor.idnumber + "\n"
-
"社保卡号:" + ssCardInfor.cardnumber + "\n"
-
"开始有效期限:" + ssCardInfor.signdate + "\n"
-
"结束有效期限:" + ssCardInfor.validterm + "\n"
-
"社保版本:" + ssCardInfor.cardveVrsion + "\n"
} else {
this.tvResult = "读卡失败:" + ssCardInfor.result+"\n errMsg = "+ssCardInfor.errMsg.trim()
}
})
Button("读基本信息")
.fontSize($r('app.float.submit_button_font_size'))
.fontWeight(CommonContants.FONT_WEIGHT)
.height(20)
.width(95)
.onClick(() => {
let datas:[number, string] = DonseeDevice.Donsee_iReadCardBas(3)
if (datas[0] == 0) {
this.tvResult = "读基本信息成功:" + datas[1]
} else {
this.tvResult = "读基本信息失败:" + datas[0]
}
})
}.margin({ top: 10 })
Row() {
Button("加密步骤1")
.fontSize($r('app.float.submit_button_font_size'))
.fontWeight(CommonContants.FONT_WEIGHT)
.height(20)
.width(95)
.onClick(() => {
let datas:[number, string] = DonseeDevice.Donsee_iReadCardBas_HSM_Step1(3)
if (datas[0] == 0) {
this.tvResult = "读卡成功:" + datas[1]
} else {
this.tvResult = "读卡失败:" + datas[0]
}
})
Button("加密步骤2")
.fontSize($r('app.float.submit_button_font_size'))
.fontWeight(CommonContants.FONT_WEIGHT)
.height(20)
.width(95)
.onClick(() => {
let datas:[number, string] = DonseeDevice.Donsee_iReadCardBas_HSM_Step2(this.pKkey)
if (datas[0] == 0) {
this.tvResult = "读卡成功:" + datas[1]
} else {
this.tvResult = "读卡失败:" + datas[0]
}
})
} .margin({ top: 10 })
}.justifyContent(FlexAlign.End)
.margin({right:10})
}.justifyContent(FlexAlign.Start)
.width(CommonContants.FULL_PARENT)
}.justifyContent(FlexAlign.Start)
.backgroundColor($r('app.color.card_background'))
.width(CommonContants.FULL_PARENT)
.height(CommonContants.FULL_PARENT)
}
}