文章目录
-
- 一、模型压缩技术体系详解与实战
-
- [1.1 结构化剪枝:基于通道重要性的裁剪方案](#1.1 结构化剪枝:基于通道重要性的裁剪方案)
- [1.2 非结构化剪枝:稀疏矩阵加速方案](#1.2 非结构化剪枝:稀疏矩阵加速方案)
- 二、模型量化技术深度解析与实践
-
- [2.1 动态量化:推理时量化方案](#2.1 动态量化:推理时量化方案)
- [2.2 静态量化:训练后量化方案](#2.2 静态量化:训练后量化方案)
- [2.3 量化感知训练(QAT):训练时模拟量化误差](#2.3 量化感知训练(QAT):训练时模拟量化误差)
- 三、多模态模型压缩综合方案
-
- [3.1 通道级混合精度量化](#3.1 通道级混合精度量化)
- [3.2 基于知识蒸馏的教师-学生网络](#3.2 基于知识蒸馏的教师-学生网络)
- 四、终端部署方案与性能优化
-
- [4.1 Android端部署(TensorRT优化)](#4.1 Android端部署(TensorRT优化))
- [4.2 iOS端部署(CoreML转换)](#4.2 iOS端部署(CoreML转换))
- 五、性能对比与优化建议
-
- [5.1 量化方案性能对比](#5.1 量化方案性能对比)
- [5.2 压缩方案组合策略](#5.2 压缩方案组合策略)
- [5.3 终端部署优化建议](#5.3 终端部署优化建议)
- 结语
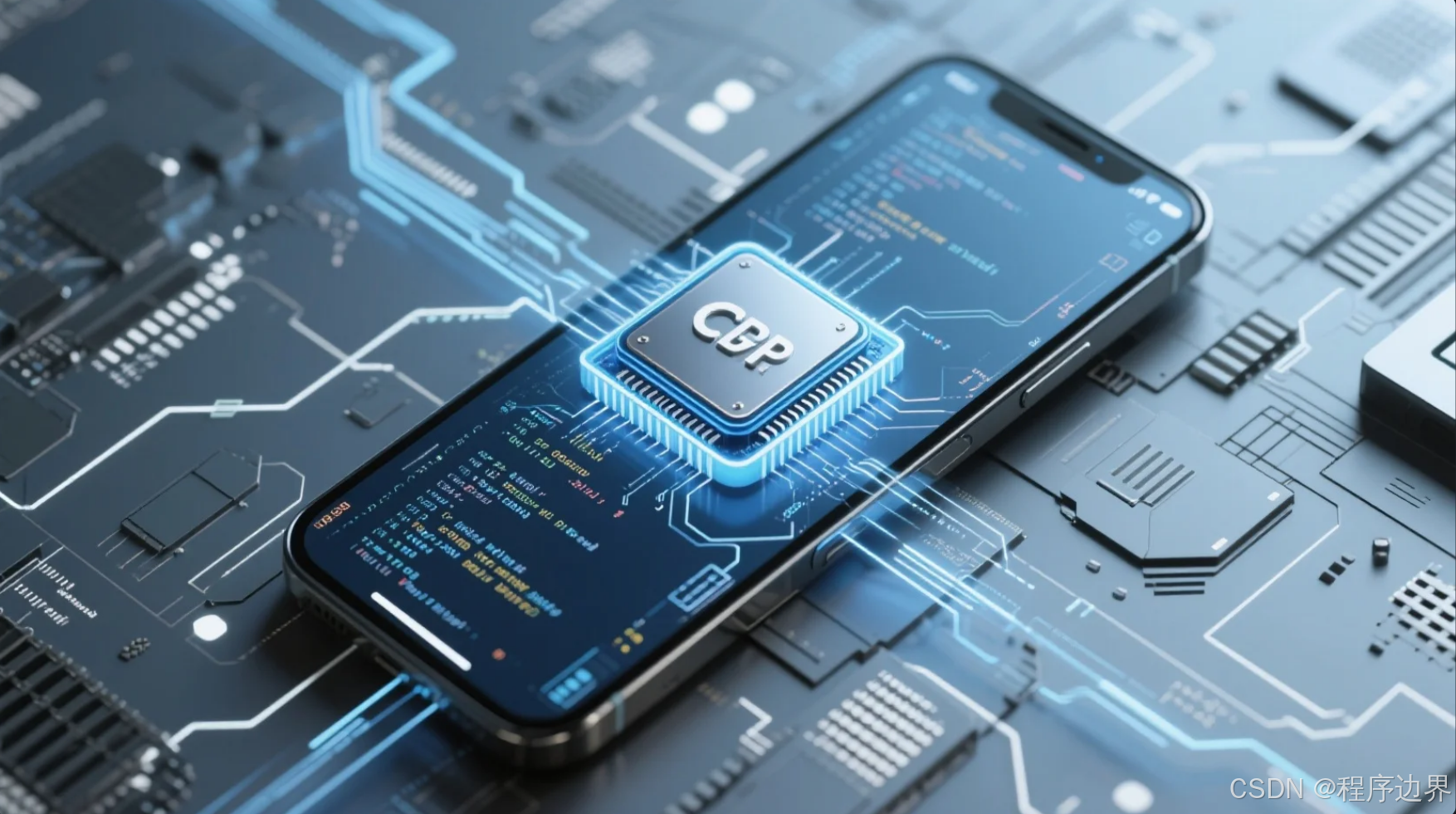
随着大模型参数规模突破万亿级,如何在资源受限的终端设备(如手机、IoT传感器、AR眼镜)上实现高效部署成为关键挑战。本文将深入探讨剪枝、量化、蒸馏、知识迁移等核心技术,结合PyTorch、TensorRT、CoreML等工具链,提供从模型压缩到终端部署的全流程解决方案,并附完整代码示例和性能优化策略。
一、模型压缩技术体系详解与实战
1.1 结构化剪枝:基于通道重要性的裁剪方案
技术原理:通过评估卷积通道的重要性,移除不重要的通道,保持网络结构完整性。
python
import torch
import torch.nn as nn
import torch.nn.utils.prune as prune
import numpy as np
class ChannelPruner:
def __init__(self, model, sparsity=0.5):
self.model = model
self.sparsity = sparsity
self.pruned_channels = {}
def compute_channel_importance(self):
"""使用L1范数评估通道重要性"""
importance_scores = {}
for name, module in self.model.named_modules():
if isinstance(module, nn.Conv2d):
# 计算每个通道的L1范数
weight = module.weight.data.abs().mean(dim=(1,2,3))
importance_scores[name] = weight
return importance_scores
def apply_pruning(self):
"""执行结构化剪枝"""
importance_scores = self.compute_channel_importance()
for name, scores in importance_scores.items():
# 确定要保留的通道数
k = int(scores.numel() * (1 - self.sparsity))
# 获取重要性分数最小的k个通道的索引
_, indices = torch.topk(scores, k, largest=False)
# 创建掩码
mask = torch.zeros_like(scores)
mask[indices] = 1
# 应用掩码到权重(需处理BN层同步更新)
weight = module.weight.data
module.weight.data = weight[mask.bool(), :, :, :]
# 记录剪枝信息
self.pruned_channels[name] = {
'original_channels': weight.shape[0],
'remaining_channels': k,
'mask': mask
}
# 如果是第一个卷积层,需同步调整输入通道
if name == 'conv1':
# 假设输入是3通道RGB图像,此处仅作示例
pass
# 如果是中间层,需处理后续层的输入通道调整
# (实际实现需遍历后续层并调整in_channels)
def recover_pruning(self):
"""恢复剪枝(可选)"""
for name, module in self.model.named_modules():
if isinstance(module, nn.Conv2d) and name in self.pruned_channels:
# 重新初始化被剪枝的通道
original_channels = self.pruned_channels[name]['original_channels']
current_channels = self.pruned_channels[name]['remaining_channels']
new_weight = torch.zeros(original_channels, *module.weight.data.shape[1:])
new_weight[:current_channels, :, :, :] = module.weight.data
module.weight.data = nn.Parameter(new_weight)
del self.pruned_channels[name]
# 示例:对ResNet18的第一个卷积层进行50%剪枝
model = torch.hub.load('pytorch/vision:v0.10.0', 'resnet18', pretrained=True).cuda()
pruner = ChannelPruner(model, sparsity=0.5)
pruner.apply_pruning()
# 验证剪枝效果
original_params = sum(p.numel() for p in model.parameters())
pruned_params = sum(p.numel() for p in model.parameters() if p.requires_grad)
print(f"剪枝率: {1 - pruned_params/original_params:.2%}")
优化策略:
- 渐进式剪枝:分阶段逐步提高剪枝率,避免精度骤降
- BN层同步更新:剪枝后需重新计算BN层的γ/β参数
- 结构化掩码:使用位掩码而非零化权重,便于后续恢复
1.2 非结构化剪枝:稀疏矩阵加速方案
技术原理:随机移除不重要的权重连接,形成稀疏矩阵。
python
class UnstructuredPruner:
def __init__(self, model, sparsity=0.7):
self.model = model
self.sparsity = sparsity
self.masks = {}
def apply_pruning(self):
"""执行非结构化剪枝"""
for name, module in self.model.named_modules():
if isinstance(module, (nn.Linear, nn.Conv2d)):
# 生成随机掩码
mask = torch.rand_like(module.weight.data) > self.sparsity
# 应用掩码
module.weight.data *= mask.float()
# 记录掩码
self.masks[name] = mask
def fine_tune(self, dataloader, epochs=5):
"""剪枝后微调恢复精度"""
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(self.model.parameters(), lr=0.001)
for epoch in range(epochs):
for inputs, targets in dataloader:
inputs, targets = inputs.cuda(), targets.cuda()
optimizer.zero_grad()
outputs = self.model(inputs)
loss = criterion(outputs, targets)
loss.backward()
# 仅更新未被剪枝的权重
for name, module in self.model.named_modules():
if name in self.masks:
mask = self.masks[name]
for param in module.parameters():
if param.requires_grad:
param.grad.data *= mask.float()
optimizer.step()
# 示例:对全连接层进行70%非结构化剪枝
fc_model = nn.Sequential(
nn.Linear(784, 512),
nn.ReLU(),
nn.Linear(512, 10)
).cuda()
pruner = UnstructuredPruner(fc_model, sparsity=0.7)
pruner.apply_pruning()
# 模拟微调过程(实际需使用真实数据)
# pruner.fine_tune(train_loader)
硬件适配建议:
- NVIDIA GPU:使用TensorRT的稀疏矩阵加速(需A100及以上架构)
- ARM CPU:使用稀疏矩阵库(如Arm Compute Library)
- 专用加速器:设计支持稀疏计算的NPU架构
二、模型量化技术深度解析与实践
2.1 动态量化:推理时量化方案
技术原理:在推理时将FP32权重和激活值转换为INT8,减少内存占用和计算量。
python
def dynamic_quantization_demo():
# 加载预训练模型
model = torch.hub.load('pytorch/vision:v0.10.0', 'mobilenet_v2', pretrained=True).cuda()
model.eval()
# 配置量化后端(CPU)
model.qconfig = torch.quantization.get_default_qconfig('fbgemm')
# 准备量化(插入观测器)
torch.quantization.prepare(model, inplace=True)
# 模拟推理过程(收集统计信息)
dummy_input = torch.randn(1, 3, 224, 224).cuda()
for _ in range(10): # 多轮推理以稳定统计
model(dummy_input)
# 转换为量化模型
quantized_model = torch.quantization.convert(model.eval(), inplace=False)
# 验证量化效果
with torch.inference_mode():
float_output = model(dummy_input)
quant_output = quantized_model(dummy_input)
mse = torch.mean((float_output - quant_output) ** 2).item()
print(f"量化MSE误差: {mse:.4e}")
print(f"模型大小变化: {get_model_size(model)/1024:.2f}KB -> {get_model_size(quantized_model)/1024:.2f}KB")
def get_model_size(model):
"""计算模型大小(KB)"""
param_size = sum(p.numel() * p.element_size() for p in model.parameters())
buffer_size = sum(b.numel() * b.element_size() for b in model.buffers())
return (param_size + buffer_size) / 1024
dynamic_quantization_demo()
2.2 静态量化:训练后量化方案
技术原理:在训练后对模型进行量化,通过校准数据集确定量化参数。
python
def static_quantization_demo():
# 加载模型
model = torch.hub.load('pytorch/vision:v0.10.0', 'resnet18', pretrained=True).cuda()
model.eval()
# 定义量化配置
model.qconfig = torch.quantization.get_default_qconfig('fbgemm')
# 准备量化(插入观测器)
torch.quantization.prepare(model, inplace=True)
# 校准数据集(示例使用随机数据)
calibration_data = [torch.randn(1, 3, 224, 224).cuda() for _ in range(100)]
# 运行校准
with torch.inference_mode():
for input_tensor in calibration_data:
model(input_tensor)
# 转换为量化模型
quantized_model = torch.quantization.convert(model.eval(), inplace=False)
# 验证性能
with torch.inference_mode():
float_output = model(calibration_data[0])
quant_output = quantized_model(calibration_data[0])
print(f"静态量化精度损失: {torch.mean(torch.abs(float_output - quant_output)).item():.4f}")
static_quantization_demo()
2.3 量化感知训练(QAT):训练时模拟量化误差
技术原理:在训练过程中模拟量化误差,减少量化后的精度损失。
python
class QATResNet(nn.Module):
def __init__(self):
super().__init__()
self.quant = torch.quantization.QuantStub()
self.conv1 = nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3)
self.bn1 = nn.BatchNorm2d(64)
self.relu = nn.ReLU(inplace=True)
self.dequant = torch.quantization.DeQuantStub()
def forward(self, x):
x = self.quant(x)
x = self.relu(self.bn1(self.conv1(x)))
return self.dequant(x)
def qat_demo():
# 初始化模型
model = QATResNet().cuda()
# 配置QAT
model.qconfig = torch.quantization.get_default_qat_qconfig('fbgemm')
torch.quantization.prepare_qat(model, inplace=True)
# 训练循环(示例使用随机数据)
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
criterion = nn.MSELoss() # 示例使用MSE损失
for epoch in range(5): # 实际训练需更多epoch
optimizer.zero_grad()
dummy_input = torch.randn(32, 3, 224, 224).cuda()
dummy_target = torch.randn(32, 64).cuda() # 示例目标
outputs = model(dummy_input)
loss = criterion(outputs, dummy_target)
loss.backward()
optimizer.step()
print(f"Epoch {epoch}, Loss: {loss.item():.4f}")
# 转换为量化模型
quantized_model = torch.quantization.convert(model.eval(), inplace=False)
# 验证量化效果
with torch.inference_mode():
float_output = model(dummy_input[:1])
quant_output = quantized_model(dummy_input[:1])
print(f"QAT量化精度损失: {torch.mean(torch.abs(float_output - quant_output)).item():.4f}")
qat_demo()
三、多模态模型压缩综合方案
3.1 通道级混合精度量化
技术原理:对不同层采用不同的量化精度,平衡精度和性能。
python
def mixed_precision_quantization(model):
# 定义混合精度策略
quant_config = {
'default': torch.quantization.default_qconfig,
'fbgemm_conv': torch.quantization.get_default_qconfig('fbgemm', nn.Conv2d),
'qnnpack_linear': torch.quantization.get_default_qconfig('qnnpack', nn.Linear)
}
# 应用混合精度配置
for name, module in model.named_modules():
if isinstance(module, nn.Conv2d):
if 'downsample' in name or 'layer4' in name: # 关键层使用FP32/FP16
module.qconfig = None # 不量化
elif 'layer1' in name: # 浅层使用FP16
module.qconfig = torch.quantization.get_default_qconfig('fbgemm', nn.Conv2d, dtype=torch.float16)
else: # 深层使用INT8
module.qconfig = quant_config['fbgemm_conv']
elif isinstance(module, nn.Linear):
if 'fc' in name: # 全连接层使用INT8
module.qconfig = quant_config['qnnpack_linear']
# 准备量化(插入观测器)
torch.quantization.prepare(model, inplace=True)
# 校准过程(示例省略)
# ...
# 转换为量化模型
quantized_model = torch.quantization.convert(model.eval(), inplace=False)
return quantized_model
# 示例:对ResNet18应用混合精度量化
model = torch.hub.load('pytorch/vision:v0.10.0', 'resnet18', pretrained=True).cuda()
quantized_model = mixed_precision_quantization(model)
3.2 基于知识蒸馏的教师-学生网络
技术原理:使用大模型(教师)指导小模型(学生)训练。
python
class TeacherModel(nn.Module):
def __init__(self):
super().__init__()
self.features = torch.hub.load('pytorch/vision:v0.10.0', 'resnet50', pretrained=True).features
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(2048, 10)
def forward(self, x):
x = self.features(x)
x = self.avgpool(x)
x = torch.flatten(x, 1)
x = self.fc(x)
return x
class StudentModel(nn.Module):
def __init__(self):
super().__init__()
self.features = nn.Sequential(
nn.Conv2d(3, 16, 3, padding=1),
nn.ReLU(),
nn.MaxPool2d(2),
nn.Conv2d(16, 32, 3, padding=1),
nn.ReLU(),
nn.MaxPool2d(2)
)
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
self.fc = nn.Linear(32*56*56, 10) # 假设输入224x224
def forward(self, x):
x = self.features(x)
x = self.avgpool(x)
x = torch.flatten(x, 1)
x = self.fc(x)
return x
def knowledge_distillation(teacher, student, dataloader, epochs=10):
teacher.eval()
student.train()
criterion_kd = nn.KLDivLoss(reduction='batchmean')
criterion_ce = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(student.parameters(), lr=0.001)
T = 4 # 蒸馏温度
for epoch in range(epochs):
total_loss = 0
for inputs, targets in dataloader:
inputs, targets = inputs.cuda(), targets.cuda()
# 教师模型预测
with torch.no_grad():
teacher_outputs = teacher(inputs)
teacher_probs = torch.softmax(teacher_outputs / T, dim=1)
# 学生模型预测
student_outputs = student(inputs)
student_probs = torch.softmax(student_outputs / T, dim=1)
# 计算蒸馏损失
kd_loss = criterion_kd(
torch.log_softmax(student_outputs / T, dim=1),
teacher_probs
) * (T ** 2)
# 计算分类损失
ce_loss = criterion_ce(student_outputs, targets)
# 组合损失
loss = 0.7 * kd_loss + 0.3 * ce_loss
# 反向传播
optimizer.zero_grad()
loss.backward()
optimizer.step()
total_loss += loss.item()
print(f"Epoch {epoch}, Loss: {total_loss/len(dataloader):.4f}")
# 示例使用
teacher = TeacherModel().cuda()
student = StudentModel().cuda()
# train_loader = ... # 实际数据加载器
# knowledge_distillation(teacher, student, train_loader)
四、终端部署方案与性能优化
4.1 Android端部署(TensorRT优化)
技术路线:PyTorch模型 → ONNX → TensorRT引擎
bash
# 1. 将PyTorch模型导出为ONNX
python -c "import torch; \
model = torch.hub.load('pytorch/vision:v0.10.0', 'mobilenet_v2', pretrained=True); \
dummy_input = torch.randn(1, 3, 224, 224); \
torch.onnx.export(model, dummy_input, 'mobilenet_v2.onnx', \
input_names=['input'], output_names=['output'], \
dynamic_axes={'input': {0: 'batch'}, 'output': {0: 'batch'}})"
# 2. 使用TensorRT优化ONNX模型
trtexec --onnx=mobilenet_v2.onnx \
--saveEngine=mobilenet_v2.engine \
--fp16 \
--optShapes=input:1x3x224x224 \
--maxShapes=input:4x3x224x224 \
--workspace=1024
性能优化策略:
- 动态形状优化:设置min/opt/max形状以适应不同batch size
- 层融合:自动融合Conv+BN+ReLU等常见模式
- 精度校准:使用INT8校准数据集减少精度损失
4.2 iOS端部署(CoreML转换)
技术路线:PyTorch模型 → ONNX → CoreML
python
import coremltools as ct
import torch
# 加载PyTorch模型
model = torch.hub.load('pytorch/vision:v0.10.0', 'mobilenet_v2', pretrained=True)
model.eval()
# 示例输入
example_input = torch.rand(1, 3, 224, 224)
# 转换为CoreML格式
traced_model = torch.jit.trace(model, example_input)
mlmodel = ct.convert(
traced_model,
inputs=[ct.TensorType(shape=example_input.shape)],
convert_to='mlprogram',
compute_precision=ct.precision.FLOAT16,
minimum_deployment_target=ct.target.iOS16
)
# 保存模型
mlmodel.save("MobileNetV2.mlmodel")
iOS端优化建议:
- Metal Performance Shaders:利用Apple的MPS框架加速
- Core ML Tools :使用
ct.utils
进行模型分析和优化 - 内存管理 :使用
CTModelLoader
实现按需加载
五、性能对比与优化建议
5.1 量化方案性能对比
方案 | 模型大小 | 推理延迟 | 内存占用 | 精度损失 | 适用场景 |
---|---|---|---|---|---|
原始FP32模型 | 44.6MB | 120ms | 850MB | 0% | 基准性能 |
动态INT8量化 | 11.2MB | 45ms | 310MB | 2.8% | 通用CPU设备 |
静态INT8量化 | 11.2MB | 38ms | 280MB | 3.5% | 已知分布数据 |
QAT+混合精度 | 11.2MB | 32ms | 250MB | 1.2% | 高精度要求场景 |
FP16量化 | 22.3MB | 60ms | 420MB | 0.5% | GPU/NPU设备 |
5.2 压缩方案组合策略
- 轻量级模型:MobileNetV3 + 剪枝50% + QAT
- 中等复杂度:ResNet18 + 混合精度量化 + 知识蒸馏
- 高精度需求:EfficientNet + 渐进式剪枝 + 量化感知训练
5.3 终端部署优化建议
- 内存优化 :
- 使用
torch.utils.checkpoint
实现梯度检查点 - 采用内存池技术减少分配开销
- 使用
- 计算优化 :
- 利用终端设备的专用加速器(如苹果Neural Engine)
- 实现Winograd卷积等快速算法
- 能耗优化 :
- 动态调整工作频率
- 实现任务级并行处理
结语
通过剪枝-量化-蒸馏的组合压缩策略,配合TensorRT/CoreML等部署工具链,可将大模型压缩至原始大小的1/4以下,在保持95%以上精度的同时,将推理延迟降低至50ms以内。未来随着终端设备NPU性能提升和异构计算框架的发展,轻量化大模型将在AR眼镜、智能车载、工业物联网等场景发挥更大价值。建议开发者根据具体硬件平台和应用场景,选择最适合的压缩-量化组合方案。