子组件:
html
<template>
<div>
<!-- 这里以 el-time-select 为例,实际可以是任何表单控件 -->
<el-time-select
v-model="innerValue.time"
:picker-options="timeOptions"
@change="handleTimeChange"
placeholder="选择时间"
/>
</div>
</template>
<script>
export default {
props: {
value: { // 必须使用 value 作为 prop 名
type: Object,
default: () => ({
time: '',
// 其他可能的属性
}),
required: true
}
},
data() {
return {
timeOptions: {
start: '08:30',
step: '00:15',
end: '18:30'
},
// 创建内部副本以避免直接修改 prop
innerValue: JSON.parse(JSON.stringify(this.value))
}
},
watch: {
value: {
handler(newVal) {
this.innerValue = JSON.parse(JSON.stringify(newVal))
},
deep: true
}
},
methods: {
handleTimeChange(newTime) {
const newValue = { ...this.internalValue, time: newTime };
this.internalValue = newValue;
// 触发 input 事件以更新 v-model
this.$emit('input', newValue)
}
}
}
</script>
父组件:
html
<template>
<div>
<my-component v-model="formData" />
<p>当前时间: {{ formData.time }}</p>
<pre>{{ formData }}</pre>
</div>
</template>
<script>
import MyComponent from './time.vue'
export default {
components: {
MyComponent
},
data() {
return {
formData: {
time: '09:00',
// 可以包含其他属性
meta: 'additional data'
}
}
}
}
</script>
注意:
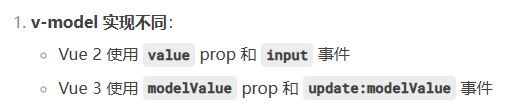