😏*★,°* :.☆( ̄▽ ̄)/$:.°★ 😏
这篇文章主要介绍创建BMI计算器应用并添加依赖和打包。
学其所用,用其所学。------梁启超欢迎来到我的博客,一起学习,共同进步。
喜欢的朋友可以关注一下,下次更新不迷路🥞
文章目录
-
- [:smirk:1. BMI计算器示例](#:smirk:1. BMI计算器示例)
- [:blush:2. 添加依赖](#:blush:2. 添加依赖)
- [:satisfied:3. 应用打包](#:satisfied:3. 应用打包)
😏1. BMI计算器示例
继续前面第一篇的,在显示时间的基础上增加BMI计算器,为了方便,各个功能分成单独dart文件实现,共3个文件。
main.dart
dart
import 'package:flutter/material.dart';
import 'time_display.dart';
import 'bmi_calculator.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'frank_test',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatelessWidget {
const HomeScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('frank_test'),
),
body: SingleChildScrollView(
child: Column(
children: const [
TimeDisplayScreen(),
BMICalculator(),
],
),
),
);
}
}
time_display.dart
dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import 'dart:async';
class TimeDisplayScreen extends StatefulWidget {
const TimeDisplayScreen({super.key});
@override
State<TimeDisplayScreen> createState() => _TimeDisplayScreenState();
}
class _TimeDisplayScreenState extends State<TimeDisplayScreen> {
String _currentTime = '';
late Timer _timer;
@override
void initState() {
super.initState();
_updateTime();
_timer = Timer.periodic(const Duration(seconds: 1), (timer) {
_updateTime();
});
}
@override
void dispose() {
_timer.cancel();
super.dispose();
}
void _updateTime() {
setState(() {
_currentTime = DateFormat('yyyy-MM-dd HH:mm:ss').format(DateTime.now());
});
}
@override
Widget build(BuildContext context) {
return Card(
elevation: 4,
margin: const EdgeInsets.all(16),
child: Padding(
padding: const EdgeInsets.all(16),
child: Column(
children: [
const Text(
'当前时间',
style: TextStyle(fontSize: 20, fontWeight: FontWeight.bold),
),
const SizedBox(height: 16),
Text(
_currentTime,
style: const TextStyle(fontSize: 24),
),
],
),
),
);
}
}
bmi_caculator.dart
dart
import 'package:flutter/material.dart';
class BMICalculator extends StatefulWidget {
const BMICalculator({super.key});
@override
State<BMICalculator> createState() => _BMICalculatorState();
}
class _BMICalculatorState extends State<BMICalculator> {
final TextEditingController _heightController = TextEditingController();
final TextEditingController _weightController = TextEditingController();
double _bmiResult = 0;
String _bmiCategory = '';
void _calculateBMI() {
final double height = double.tryParse(_heightController.text) ?? 0;
final double weight = double.tryParse(_weightController.text) ?? 0;
if (height > 0 && weight > 0) {
setState(() {
_bmiResult = weight / ((height / 100) * (height / 100));
_updateBMICategory();
});
}
}
void _updateBMICategory() {
if (_bmiResult < 18.5) {
_bmiCategory = '偏瘦';
} else if (_bmiResult < 24) {
_bmiCategory = '正常';
} else if (_bmiResult < 28) {
_bmiCategory = '过重';
} else {
_bmiCategory = '肥胖';
}
}
@override
void dispose() {
_heightController.dispose();
_weightController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Card(
elevation: 4,
margin: const EdgeInsets.all(16),
child: Padding(
padding: const EdgeInsets.all(16),
child: Column(
children: [
const Text(
'BMI 计算器',
style: TextStyle(fontSize: 20, fontWeight: FontWeight.bold),
),
const SizedBox(height: 16),
TextField(
controller: _heightController,
keyboardType: TextInputType.number,
decoration: const InputDecoration(
labelText: '身高 (cm)',
border: OutlineInputBorder(),
),
),
const SizedBox(height: 16),
TextField(
controller: _weightController,
keyboardType: TextInputType.number,
decoration: const InputDecoration(
labelText: '体重 (kg)',
border: OutlineInputBorder(),
),
),
const SizedBox(height: 16),
ElevatedButton(
onPressed: _calculateBMI,
child: const Text('计算 BMI'),
),
const SizedBox(height: 16),
if (_bmiResult > 0)
Column(
children: [
Text(
'BMI: ${_bmiResult.toStringAsFixed(1)}',
style: const TextStyle(fontSize: 18),
),
Text(
'状态: $_bmiCategory',
style: TextStyle(
fontSize: 18,
color: _getCategoryColor(),
),
),
],
),
],
),
),
);
}
Color _getCategoryColor() {
switch (_bmiCategory) {
case '偏瘦':
return Colors.blue;
case '正常':
return Colors.green;
case '过重':
return Colors.orange;
case '肥胖':
return Colors.red;
default:
return Colors.black;
}
}
}
😊2. 添加依赖
如果程序依赖一些库,比如这里依赖intl,需要在pubspec.yaml文件中添加:
bash
dependencies:
flutter:
sdk: flutter
intl: ^0.18.1
😆3. 应用打包
打包web:
bash
flutter build web --release
# 运行
cd build/web
python3 -m http.server 8000
打包linux:
bash
flutter build linux --release
./build/linux/x64/release/bundle/frank_test
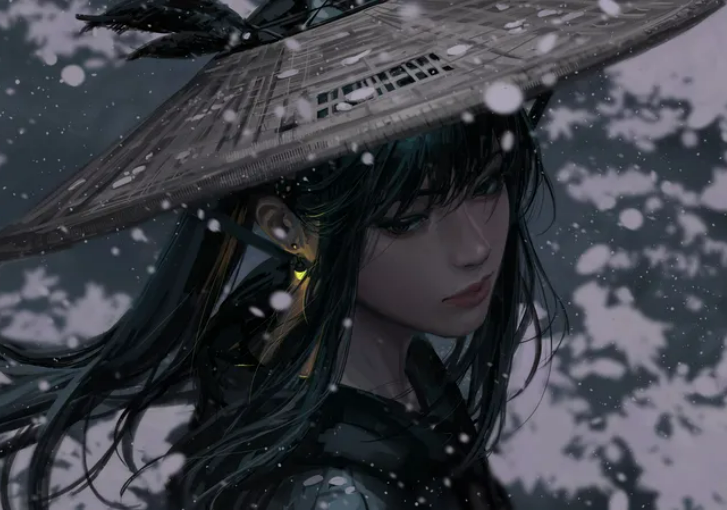
以上。