在当今数据驱动的世界中,MongoDB 作为最流行的 NoSQL 数据库之一,因其灵活的数据模型和强大的扩展能力而广受开发者喜爱。然而,与任何复杂系统一样,在使用 MongoDB 过程中难免会遇到各种错误和性能问题。本文将全面介绍 MongoDB 的错误处理与调试技巧,帮助您构建更健壮的应用程序。
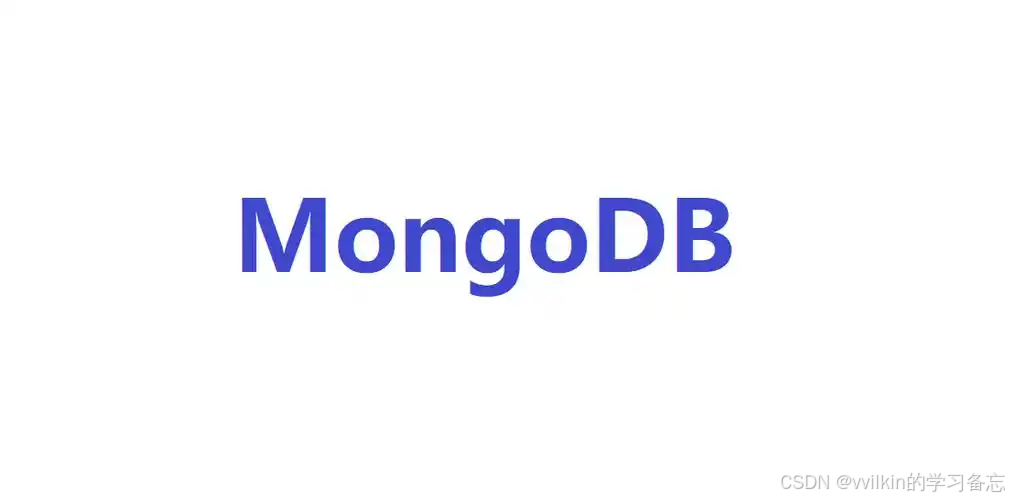
一、MongoDB 错误处理基础
1.1 MongoDB 错误类型解析
MongoDB 错误通常可以分为以下几大类:
-
连接错误:发生在与数据库建立连接阶段
-
认证失败 (Error 18)
-
连接超时
-
网络不可达
-
-
查询错误:执行读取操作时出现
-
无效查询语法
-
权限不足
-
集合不存在
-
-
写入错误:执行写入操作时出现
-
唯一键冲突 (Error 11000)
-
文档验证失败
-
写入超时
-
-
配置错误:服务器或客户端配置不当导致
-
副本集配置错误
-
分片配置问题
-
索引配置不当
-
1.2 错误处理的基本模式
在 Node.js 驱动中,处理 MongoDB 错误的标准模式是 try-catch 块:
const { MongoClient } = require('mongodb');
async function connectToMongoDB() {
const client = new MongoClient('mongodb://localhost:27017');
try {
await client.connect();
console.log('Connected successfully to server');
const db = client.db('myDatabase');
const collection = db.collection('myCollection');
// 执行数据库操作...
} catch (err) {
console.error('Database operation failed:', {
message: err.message,
code: err.code,
stack: err.stack
});
// 根据错误类型采取特定措施
if (err.code === 11000) {
console.error('Duplicate key error detected');
// 处理重复键逻辑
} else if (err.code === 18) {
console.error('Authentication failed');
// 处理认证失败逻辑
}
} finally {
await client.close();
}
}
二、深入 MongoDB 错误调试
2.1 启用详细日志记录
日志是调试的第一道防线。MongoDB 提供了多层次的日志记录功能:
服务器端日志配置:
// 在mongod.conf中配置
systemLog:
destination: file
path: "/var/log/mongodb/mongod.log"
logAppend: true
verbosity: 2 # 0=安静, 1=默认, 2=详细, 3-5=更详细
客户端日志配置(Node.js驱动):
const { MongoClient } = require('mongodb');
const { Logger } = require('mongodb');
// 设置全局日志级别
Logger.setLevel('debug');
const client = new MongoClient(uri, {
loggerLevel: 'debug',
monitorCommands: true // 启用命令监控
});
2.2 使用 explain() 分析查询性能
explain()
方法是理解 MongoDB 查询行为的强大工具,它提供了查询执行的详细信息:
const explain = await collection.find({
age: { $gt: 25 },
status: 'active'
})
.sort({ name: 1 })
.limit(100)
.explain('executionStats'); // 也可以使用'allPlansExecution'
console.log(JSON.stringify(explain, null, 2));
关键指标解读:
-
executionStats.executionTimeMillis:查询总耗时
-
executionStats.totalKeysExamined:检查的索引键数量
-
executionStats.totalDocsExamined:检查的文档数量
-
executionStats.nReturned:返回的文档数量
-
executionStats.executionStages:详细的执行阶段信息
理想情况下,totalDocsExamined
应该接近 nReturned
,如果前者远大于后者,说明查询效率低下,可能需要优化索引。
2.3 索引分析与优化
索引是 MongoDB 性能的关键。以下是检查和优化索引的方法:
查看现有索引:
const indexes = await collection.indexes();
console.table(indexes.map(idx => ({
name: idx.name,
key: JSON.stringify(idx.key),
unique: idx.unique || false,
sparse: idx.sparse || false
})));
创建适当索引:
// 单字段索引
await collection.createIndex({ age: 1 });
// 复合索引
await collection.createIndex({ age: 1, status: 1 });
// 多键索引(数组字段)
await collection.createIndex({ tags: 1 });
// 文本索引(全文搜索)
await collection.createIndex({ description: 'text' });
// 唯一索引
await collection.createIndex({ email: 1 }, { unique: true });
// TTL索引(自动过期)
await collection.createIndex({ createdAt: 1 }, { expireAfterSeconds: 3600 });
删除不需要的索引:
await collection.dropIndex('age_1_status_1');
2.4 使用数据库分析器
MongoDB 内置的分析器可以记录操作性能数据:
启用分析器:
// 设置分析级别:0=关闭,1=记录慢查询,2=记录所有操作
await db.command({ profile: 2, slowms: 100 });
查询分析结果:
const profileData = await db.collection('system.profile')
.find()
.sort({ ts: -1 })
.limit(10)
.toArray();
profileData.forEach(op => {
console.log(`Operation: ${op.op}`,
`Duration: ${op.millis}ms`,
`Query: ${JSON.stringify(op.query)}`);
});
三、高级错误处理模式
3.1 实现重试逻辑
对于网络不稳定或暂时性错误,实现重试机制可以提高系统韧性:
async function withRetry(operation, maxRetries = 3, delay = 1000) {
let attempts = 0;
let lastError;
while (attempts < maxRetries) {
try {
return await operation();
} catch (err) {
lastError = err;
attempts++;
// 可重试的错误代码列表
const retryableCodes = new Set([
6, // HostUnreachable
7, // HostNotFound
89, // NetworkTimeout
91, // ShutdownInProgress
189, // PrimarySteppedDown
262, // ExceededTimeLimit
317, // SocketException
344, // NotMaster
11600 // InterruptedAtShutdown
]);
if (!retryableCodes.has(err.code)) {
break;
}
// 指数退避
const waitTime = delay * Math.pow(2, attempts - 1);
console.warn(`Retry attempt ${attempts}, waiting ${waitTime}ms...`);
await new Promise(resolve => setTimeout(resolve, waitTime));
}
}
throw lastError;
}
// 使用示例
await withRetry(async () => {
return await collection.insertOne(document);
});
3.2 事务中的错误处理
MongoDB 4.0+ 支持多文档事务,需要特别注意错误处理:
const session = client.startSession();
try {
await session.withTransaction(async () => {
// 事务操作1
await collection1.updateOne(
{ _id: id1 },
{ $inc: { balance: -amount } },
{ session }
);
// 事务操作2
await collection2.updateOne(
{ _id: id2 },
{ $inc: { balance: amount } },
{ session }
);
});
console.log('Transaction committed');
} catch (err) {
console.error('Transaction aborted:', err);
// 检查是否可重试
if (err.errorLabels && err.errorLabels.includes('TransientTransactionError')) {
console.log('Transient error, may retry transaction');
}
} finally {
await session.endSession();
}
四、生产环境最佳实践
4.1 监控与告警
在生产环境中,完善的监控系统至关重要:
-
关键指标监控:
-
连接数
-
操作延迟
-
内存使用
-
CPU利用率
-
复制延迟(副本集)
-
分片平衡状态(分片集群)
-
-
推荐工具:
-
MongoDB Atlas 内置监控
-
Prometheus + Grafana
-
Datadog
-
Percona Monitoring and Management (PMM)
-
4.2 性能优化技巧
-
查询优化:
-
使用投影只返回必要字段
-
避免全集合扫描
-
合理使用排序和分页
-
批量操作优于单文档操作
-
-
写入优化:
-
批量插入使用
insertMany
而非多次insertOne
-
适当使用有序/无序批量写入
-
考虑写入关注级别(write concern)
-
-
架构优化:
-
合理设计分片键
-
预分配文档空间减少移动
-
冷热数据分离
-
4.3 灾难恢复策略
-
备份策略:
-
定期全量备份 + 增量备份
-
使用
mongodump
或 Ops Manager -
测试备份恢复流程
-
-
故障转移:
-
配置合适的副本集成员
-
设置正确的优先级和隐藏节点
-
监控复制延迟
-
-
安全措施:
-
启用认证和加密
-
定期审计
-
遵循最小权限原则
-
总结
MongoDB 的错误处理与调试是一项需要综合知识的技能,涉及从基本的错误捕获到高级的性能分析。通过本文介绍的技术,您应该能够:
-
识别和处理常见的 MongoDB 错误
-
使用各种工具和技术诊断问题
-
实现健壮的错误处理模式
-
优化查询和写入性能
-
建立生产环境的最佳实践
记住,有效的调试不仅在于解决问题,更在于预防问题。建立完善的监控系统,遵循最佳实践,并持续学习 MongoDB 的新特性,将帮助您构建更可靠、高性能的应用程序。
最后,当遇到棘手问题时,不要忘记 MongoDB 的官方文档和社区是非常宝贵的资源。