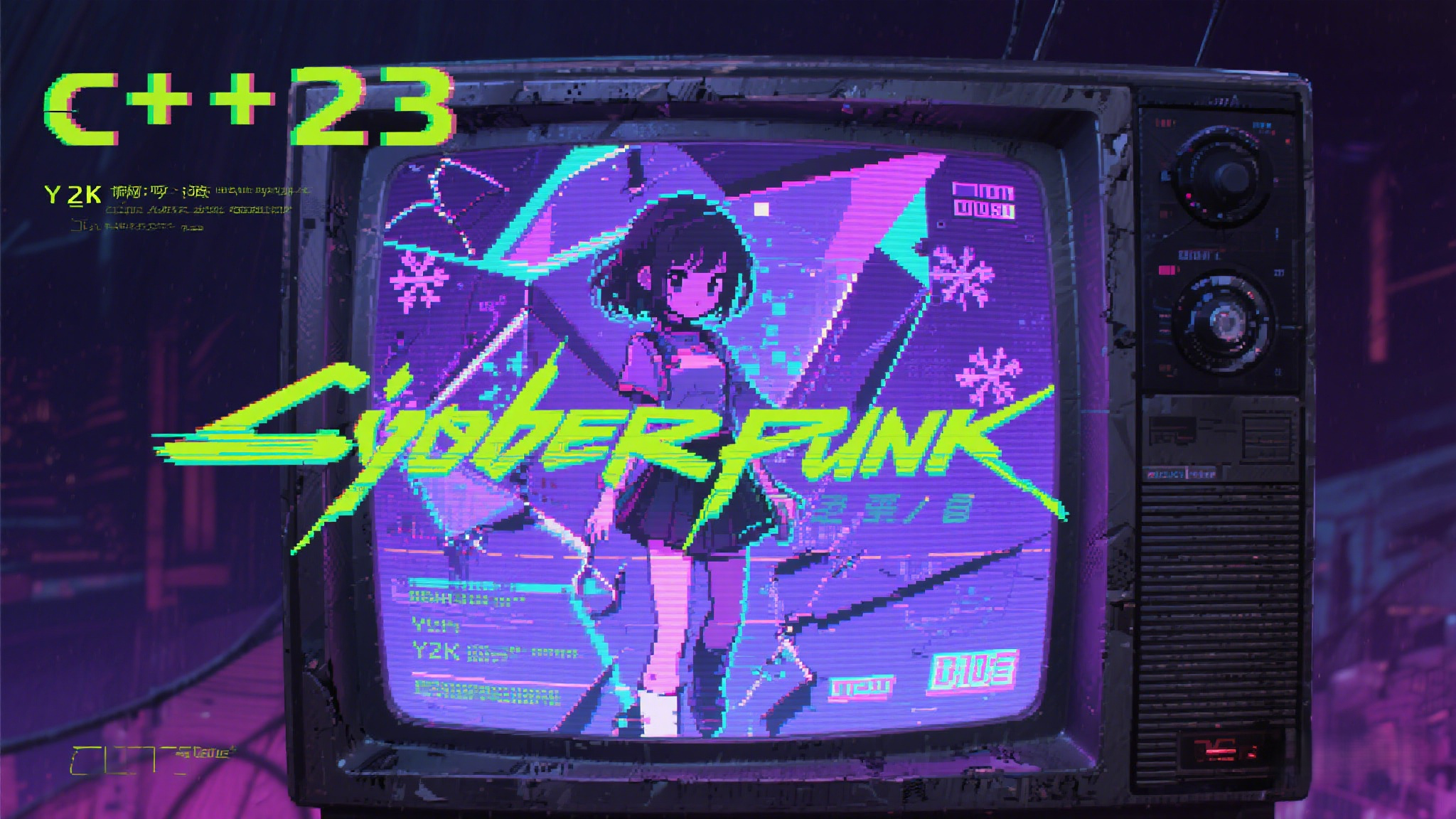
文章目录
-
- 引言
- [`std::basic_string::contains` 与 `std::basic_string_view::contains` (P1679R3)](#
std::basic_string::contains
与std::basic_string_view::contains
(P1679R3)) - [禁止从 `nullptr` 构造 `std::basic_string` 和 `std::basic_string_view` (P2166R1)](#禁止从
nullptr
构造std::basic_string
和std::basic_string_view
(P2166R1)) - [`std::basic_string_view` 的显式范围构造函数 (P1989R2)](#
std::basic_string_view
的显式范围构造函数 (P1989R2)) - `std::basic_string::resize_and_overwrite` (P1072R10)
- [用于高效切片的 `std::basic_string::substr` 右值引用重载 (P2438R2)](#用于高效切片的
std::basic_string::substr
右值引用重载 (P2438R2)) - 总结
引言
C++ 作为一门广泛应用的编程语言,一直在不断发展和演进。C++23 作为其发展历程中的一个重要版本,引入了许多新的特性和改进,其中在字符串处理方面有一些显著的改动。这些改动不仅提升了代码的性能和效率,还增强了代码的安全性和可读性。本文将详细介绍 C++23 中几个重要的字符串相关的新特性,包括 std::basic_string::contains
与 std::basic_string_view::contains
、禁止从 nullptr
构造 std::basic_string
和 std::basic_string_view
、std::basic_string_view
的显式范围构造函数、std::basic_string::resize_and_overwrite
以及用于高效切片的 std::basic_string::substr
右值引用重载。
std::basic_string::contains
与 std::basic_string_view::contains
(P1679R3)
功能介绍
在 C++23 之前,如果要检查一个字符串是否包含另一个子字符串,通常会使用 find
函数,并检查其返回值是否不等于 npos
。例如:
cpp
#include <iostream>
#include <string>
int main() {
std::string str = "hello world";
if (str.find("world") != std::string::npos) {
std::cout << "The string contains 'world'" << std::endl;
}
return 0;
}
C++23 引入了 contains
函数,使得代码更加简洁和直观。std::basic_string::contains
和 std::basic_string_view::contains
提供了三个重载版本,分别用于检查字符串是否包含一个字符串视图、一个字符或一个以空字符结尾的字符串。以下是函数原型:
cpp
constexpr bool contains( std::basic_string_view<CharT,Traits> sv ) const noexcept; // (1) (since C++23)
constexpr bool contains( CharT ch ) const noexcept; // (2) (since C++23)
constexpr bool contains( const CharT* s ) const; // (3) (since C++23)
示例代码
cpp
#include <iostream>
#include <string>
#include <string_view>
int main() {
std::string str = "hello world";
std::string_view sv = "world";
if (str.contains(sv)) {
std::cout << "The string contains the substring" << std::endl;
}
if (str.contains('o')) {
std::cout << "The string contains the character 'o'" << std::endl;
}
if (str.contains("hello")) {
std::cout << "The string contains the C-style string" << std::endl;
}
return 0;
}
优势
使用 contains
函数可以使代码更加清晰易懂,减少了不必要的代码量,提高了代码的可读性和可维护性。同时,由于其实现通常是内联的,性能上也不会有明显的损失。
禁止从 nullptr
构造 std::basic_string
和 std::basic_string_view
(P2166R1)
背景
在 C++23 之前,从 nullptr
构造 std::basic_string
和 std::basic_string_view
的行为是未定义的。这可能会导致程序崩溃或产生不可预期的结果。例如:
cpp
#include <iostream>
#include <string>
int main() {
std::string str(nullptr); // 未定义行为
std::cout << str << std::endl;
return 0;
}
改动
C++23 通过 P2166R1 提案,禁止了从 nullptr
构造 std::basic_string
和 std::basic_string_view
。具体来说,std::basic_string_view
的构造函数 basic_string_view( std::nullptr_t )
被删除:
cpp
basic_string_view( std::nullptr_t ) = delete; // (since C++23)
影响
这一改动提高了代码的安全性,避免了因从 nullptr
构造字符串而导致的潜在错误。如果尝试从 nullptr
构造字符串,编译器会报错,从而在编译阶段就发现问题。
std::basic_string_view
的显式范围构造函数 (P1989R2)
功能介绍
std::basic_string_view
是 C++17 引入的一个轻量级的非拥有型字符串表示,它设计用来提供对字符序列的引用。C++23 引入了显式范围构造函数,使得可以更方便地从一个范围构造 std::basic_string_view
。以下是构造函数原型:
cpp
constexpr basic_string_view() noexcept ; // (1) (since C++17)
constexpr basic_string_view( const basic_string_view& other ) noexcept = default ; // (2) (since C++17)
constexpr basic_string_view( const CharT* s, size_type count ) ; // (3) (since C++17)
constexpr basic_string_view( const CharT* s ) ; // (4) (since C++17)
template< class It, class End >
constexpr basic_string_view( It first, End last ) ; // (5) (since C++20)
template< class R >
constexpr explicit basic_string_view( R&& r ) ; // (6) (since C++23)
示例代码
cpp
#include <iostream>
#include <string>
#include <string_view>
int main() {
std::string str = "hello world";
std::basic_string_view sv(str.begin(), str.end());
std::cout << sv << std::endl;
return 0;
}
优势
显式范围构造函数提供了更多的灵活性,使得可以直接从迭代器范围构造 std::basic_string_view
,而不需要先构造一个临时的字符串对象。这在处理字符串切片和子字符串时非常有用。
std::basic_string::resize_and_overwrite
(P1072R10)
功能介绍
std::basic_string::resize_and_overwrite
是 C++23 引入的一个新函数,用于调整字符串的大小并覆盖其内容。该函数接受一个最大大小和一个操作函数作为参数,操作函数用于修改字符串的内容。以下是函数原型:
cpp
template< class Operation >
constexpr void resize_and_overwrite( size_type count, Operation op );
示例代码
cpp
#include <iostream>
#include <string>
int main() {
std::string str = "hello";
str.resize_and_overwrite(10, [](char* p, size_t count) {
for (size_t i = 0; i < count; ++i) {
p[i] = 'a';
}
return count;
});
std::cout << str << std::endl;
return 0;
}
优势
该函数避免了在需要修改字符串内容时,先初始化一个合适大小的 std::string
的开销。它允许直接在字符串的存储上进行操作,提高了性能。
用于高效切片的 std::basic_string::substr
右值引用重载 (P2438R2)
背景
在 C++23 之前,std::basic_string::substr
函数返回一个新的 std::string
对象,这可能会导致不必要的内存分配和复制。特别是当原字符串是一个右值(临时对象)时,这种开销是可以避免的。
改动
C++23 引入了 std::basic_string::substr
的右值引用重载,允许在原字符串是右值时,直接移动其资源,而不是进行复制。这提高了切片操作的效率。
示例代码
cpp
#include <iostream>
#include <string>
std::string getString() {
return "hello world";
}
int main() {
std::string sub = getString().substr(6);
std::cout << sub << std::endl;
return 0;
}
优势
右值引用重载的 substr
函数在处理临时字符串时,避免了不必要的复制,提高了性能。特别是在处理大型字符串时,这种优化效果更为明显。
总结
C++23 在字符串处理方面引入的这些新特性和改动,使得代码更加简洁、安全和高效。contains
函数提高了代码的可读性,禁止从 nullptr
构造字符串增强了代码的安全性,显式范围构造函数提供了更多的灵活性,resize_and_overwrite
函数避免了不必要的初始化开销,而 substr
的右值引用重载则提高了切片操作的性能。这些改动将有助于开发者编写更加优质的 C++ 代码。