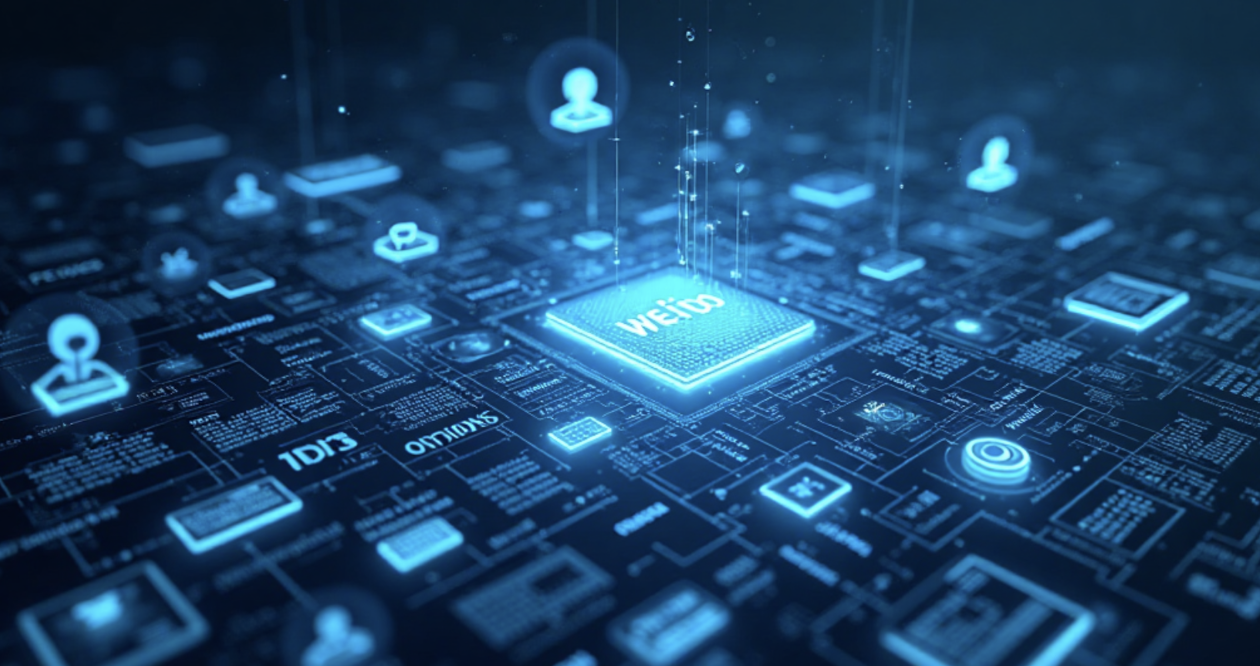
一、关键数据分析:微博热帖背后的隐含网络
微博每天产生数百万条内容,这些内容天然包含了大量非结构化文本信息,包括人物、品牌、事件、观点等实体以及它们之间的复杂关系。为了实现"自动识别+归类分析",我们采用如下实体-关系抽取流程:
🧩 目标数据结构化示例:
发帖用户 | 内容摘要 | 评论情感 | 实体1 | 关系 | 实体2 |
---|---|---|---|---|---|
用户A | 小米汽车上市首日大涨 | 正面 | 小米 | 发布 | 汽车 |
用户B | 华为和荣耀又要打擂台? | 中性 | 华为 | 对比 | 荣耀 |
我们从微博热搜中抽取:
- 原始发帖文本
- 评论区信息
- 实体关系三元组(如"华为-竞争-荣耀")
- 情感倾向(正面/负面/中性)
二、核心技术路线图谱
🧠 本项目技术模块如下图所示:
plain
┌────────────┐
│ 爬虫代理模块 │────┐
└────────────┘ │
▼
┌────────────┐
│ 请求配置模块 │(含cookie/user-agent)
└────────────┘
│
▼
┌────────────┐
│ 微博页面采集 │(热搜 + 评论)
└────────────┘
│
▼
┌─────────────────┐
│ 中文NLP抽取模块 │(实体识别+关系抽取+情感分析)
└─────────────────┘
│
▼
┌─────────────────┐
│ 数据结构化&可视化 │(保存至CSV/图谱生成)
└─────────────────┘
三、完整代码演变:从采集到结构化抽取
以下为主要实现代码,已集成爬虫代理设置、实体识别与关系抽取,适合初学者调试和项目集成。
python
import requests
from bs4 import BeautifulSoup
from fake_useragent import UserAgent
import time
import random
import jieba
import re
import csv
import spacy
from lxml import etree
# ========== 1. 代理配置(亿牛云代理 www.16yun.cn)==========
proxy_host = "proxy.16yun.cn" # 代理域名
proxy_port = "3100" # 代理端口
proxy_user = "16YUN" #用户名
proxy_pass = "16IP" #密码
proxies = {
"http": f"http://{proxy_user}:{proxy_pass}@{proxy_host}:{proxy_port}",
"https": f"http://{proxy_user}:{proxy_pass}@{proxy_host}:{proxy_port}"
}
# ========== 2. 请求头设置 ==========
ua = UserAgent()
headers = {
"User-Agent": ua.random,
"Cookie": "YOUR_WEIBO_COOKIE" # 登录后的Cookie,提高访问成功率
}
# ========== 3. 微博热搜采集 ==========
def get_hot_posts():
url = "https://s.weibo.com/top/summary?cate=realtimehot"
response = requests.get(url, headers=headers, proxies=proxies)
response.encoding = 'utf-8'
soup = BeautifulSoup(response.text, "html.parser")
links = soup.select("td.td-02 a")
titles_links = [(a.text.strip(), "https://s.weibo.com" + a.get("href")) for a in links if a.get("href")]
return titles_links[:5] # 取前5条热点
# ========== 4. 实体+关系抽取(简单版)==========
def extract_entities(text):
# 简单规则模拟实体对和关系,后续可引入深度学习模型或Spacy中文模型
patterns = [
(r"(小米|华为|荣耀|苹果|比亚迪).{1,5}(发布|对比|上市|合作|竞争).{1,5}(手机|汽车|品牌|产品)", "三元组")
]
results = []
for pattern, label in patterns:
match = re.search(pattern, text)
if match:
results.append((match.group(1), match.group(2), match.group(3)))
return results
# ========== 5. 评论情感分析模拟 ==========
def analyze_sentiment(text):
if any(word in text for word in ["好", "赞", "厉害", "支持"]):
return "正面"
elif any(word in text for word in ["差", "垃圾", "不好"]):
return "负面"
return "中性"
# ========== 6. 主流程 ==========
def run():
hot_posts = get_hot_posts()
results = []
for title, link in hot_posts:
time.sleep(random.uniform(1, 3))
print(f"正在抓取:{title}")
resp = requests.get(link, headers=headers, proxies=proxies)
if resp.status_code != 200:
print("请求失败")
continue
soup = BeautifulSoup(resp.text, "html.parser")
texts = soup.get_text()
entity_relations = extract_entities(texts)
sentiment = analyze_sentiment(texts)
for e1, rel, e2 in entity_relations:
results.append([title, e1, rel, e2, sentiment])
# ========== 7. 写入CSV文件 ==========
with open("weibo_entity_relations.csv", "w", newline="", encoding="utf-8") as f:
writer = csv.writer(f)
writer.writerow(["微博标题", "实体1", "关系", "实体2", "情感"])
writer.writerows(results)
print("数据采集完成 🎉")
if __name__ == "__main__":
run()
四、技术演变模式可视化
👣 版本1.0:正则规则抽取
- 优点:简单快速,无需训练
- 缺点:容易漏识/误识,缺乏上下文理解能力
👣 版本2.0(可扩展):BERT-BiLSTM-CRF或Prompt式实体关系识别
- 支持微调中文预训练模型
- 可用开源库如
LTP
,HanLP
,Spacy-zh
,BERT4NER
等
五、总结
💡 本文用一套「微博热帖 → 文本抽取 → 实体关系 → 情感标注」的完整流程,验证了中文非结构化文本的NLP实战价值。