一、阿里云对象存储
1.引入maven
官网:https://help.aliyun.com/zh/oss/developer-reference/java-installation?spm=a2c4g.11186623.0.i12
bash
<dependency>
<groupId>com.aliyun.oss</groupId>
<artifactId>aliyun-sdk-oss</artifactId>
<version>3.15.1</version>
</dependency>
如果使用的是Java 9及以上的版本,则需要添加JAXB相关依赖。添加JAXB相关依赖示例代码如下:
bash
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>javax.activation</groupId>
<artifactId>activation</artifactId>
<version>1.1.1</version>
</dependency>
<!-- no more than 2.3.3-->
<dependency>
<groupId>org.glassfish.jaxb</groupId>
<artifactId>jaxb-runtime</artifactId>
<version>2.3.3</version>
</dependency>
2.Endpoint地址获取
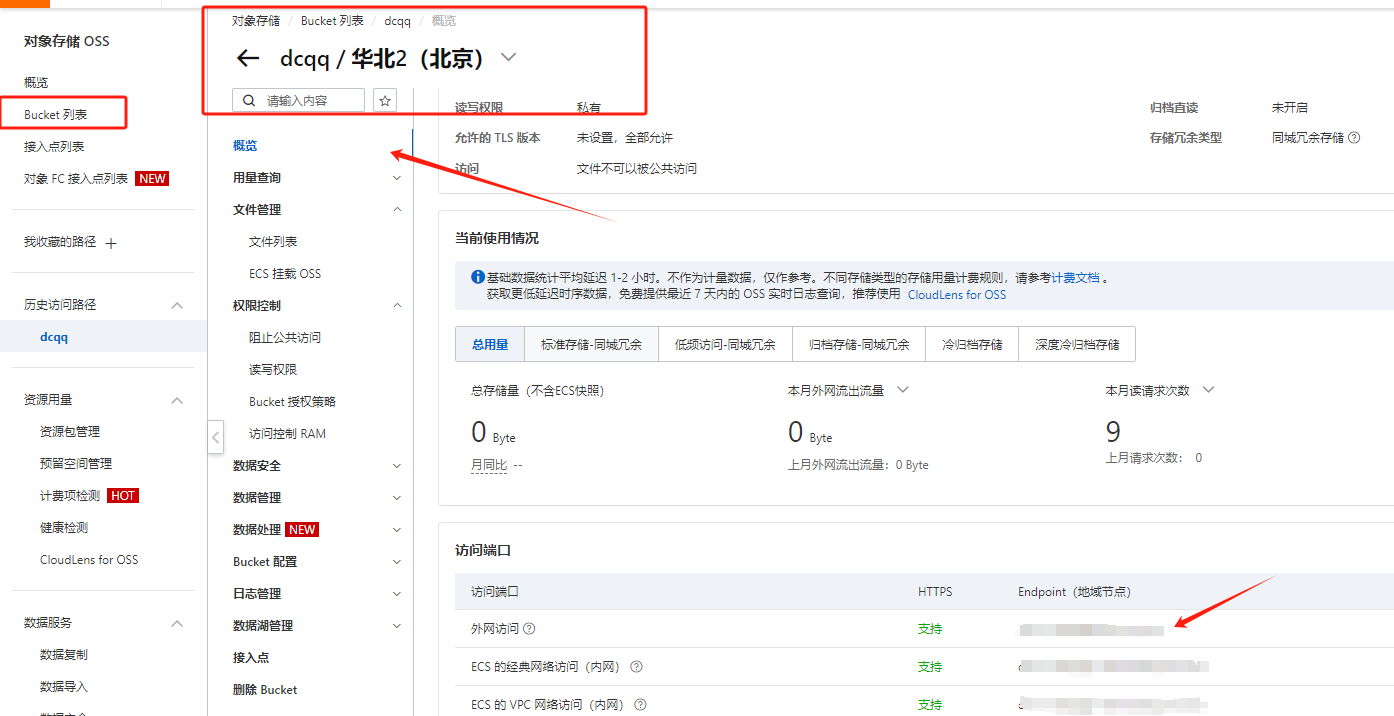
3.环境变量配置
以windows为例,配置之后重启idea工具(我这配置之后没生效,重启之后生效)
4.工具类
java
package com.dcqq.common.utils;
import com.aliyun.oss.OSS;
import com.aliyun.oss.OSSClientBuilder;
import com.aliyun.oss.common.auth.CredentialsProviderFactory;
import com.aliyun.oss.common.auth.EnvironmentVariableCredentialsProvider;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
public class AliYunOssUtil {
// Endpoint以华东1(杭州)为例,其它Region请按实际情况填写。
private static String endpoint = "https://oss-cn-beijing.aliyuncs.com";
// 填写Bucket名称,例如examplebucket。
private static String bucketName = "examplebucket";
private static String url = "https://"+bucketName+".oss-cn-beijing.aliyuncs.com/";
/**
* 文件上传
* @param bytes
* @param objectName 阿里云存储文件的位置,包括文件名,不包含存储桶bucket名称,如:upload/1.png ,注意:upload前面不能有斜杠
* @return
* @throws Exception
*/
public static String upload(byte[] bytes,String objectName) throws Exception {
// 从环境变量中获取访问凭证。运行本代码示例之前,请确保已设置环境变量OSS_ACCESS_KEY_ID和OSS_ACCESS_KEY_SECRET
EnvironmentVariableCredentialsProvider credentialsProvider = CredentialsProviderFactory.newEnvironmentVariableCredentialsProvider();
//创建OSSClient实例
OSS ossClient = new OSSClientBuilder().build(endpoint, credentialsProvider);
ossClient.putObject(bucketName, objectName, new ByteArrayInputStream(bytes));
return url+objectName;
}
public static void main(String[] args) throws Exception {
File file=new File("C:\\图片\\1.png");
FileInputStream fileInputStream=new FileInputStream(file);
byte[] fileBytes = new byte[(int) file.length()];
fileInputStream.read(fileBytes);
upload(fileBytes,"upload/1.png");
}
}