1:拷贝图片
#include <stdio.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, const char *argv[])
{
//在子进程拥有和父进程一样的拷贝文件(确保拷贝文件为空)
int fp_w = open("cpy.jpg",O_WRONLY|O_CREAT|O_TRUNC,0664);
if(fp_w<0)
{
perror("open");
return -1;
}
//关闭创建的拷贝文件
close(fp_w);
//创建一个子进程
pid_t pid = fork();
//在父进程中打开原文件和拷贝文件
int fp_r = open("photo1.jpg",O_RDONLY);
if(fp_r<0)
{
perror("open");
return -1;
}
fp_w=open("cpy.jpg",O_WRONLY);
if(fp_w<0)
{
perror("open");
return -1;
}
//计算原文件大小,并返回字节大小
off_t size = lseek(fp_r,0,SEEK_END);
//pid>0进入父进程
if(pid>0)
{
//让原文件和目标文件都偏移到起始位置
lseek(fp_r,0,SEEK_SET);
lseek(fp_w,0,SEEK_SET);
char c;
//一个字节一个字节循环拷贝,且只拷贝一半
for(int i=0;i<size/2;i++)
{
read(fp_r,&c,1);
write(fp_w,&c,1);
}
printf("前半部分拷贝完成!\n");
}
//进入子进程
else if(pid == 0)
{
execl("./cp2out","./cp2out",NULL);
}
else
{
perror("fork");
return -1;
}
close(fp_w);
close(fp_r);
return 0;
}
结果图片:
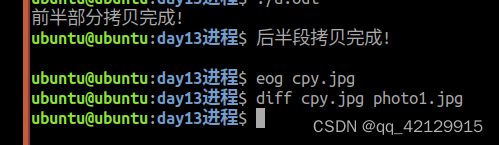
2:数组的翻转
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
static char buf[] = "1234567";
void* callBack(void* arg)
{
int len = strlen(buf);
for(int i=0 ;i<len;i++)
{
printf("%c",buf[i]);
}
putchar(10);
return NULL;
}
void* callBack1(void* arg)
{
int len = strlen(buf);
for(int i=0;i<len/2;i++)
{
buf[i] = buf[i] ^ buf[len-1-i];
buf[len-1-i] = buf[i] ^ buf[len-1-i];
buf[i] = buf[i] ^ buf[len-1-i];
}
// for(int i=0;i<len;i++)
// {
// printf("%c",buf[i]);
// }
// putchar(10);
}
int main(int argc, const char *argv[])
{
pthread_t A;
pthread_t B;
if(pthread_create(&A,NULL,callBack1,NULL)!=0)
{
perror("pthread_create ");
return -1;
}
if(pthread_create(&B,NULL,callBack,NULL)!=0)
{
perror("pthread_create ");
return -1;
}
pthread_join(B,NULL);
pthread_join(A,NULL);
return 0;
}