174. Dungeon Game
The demons had captured the princess and imprisoned her in the bottom-right corner of a dungeon. The dungeon consists of m x n rooms laid out in a 2D grid. Our valiant knight was initially positioned in the top-left room and must fight his way through dungeon to rescue the princess.
The knight has an initial health point represented by a positive integer. If at any point his health point drops to 0 or below, he dies immediately.
Some of the rooms are guarded by demons (represented by negative integers), so the knight loses health upon entering these rooms; other rooms are either empty (represented as 0) or contain magic orbs that increase the knight's health (represented by positive integers).
To reach the princess as quickly as possible, the knight decides to move only rightward or downward in each step.
Return the knight's minimum initial health so that he can rescue the princess.
Note that any room can contain threats or power-ups, even the first room the knight enters and the bottom-right room where the princess is imprisoned.
Example 1:
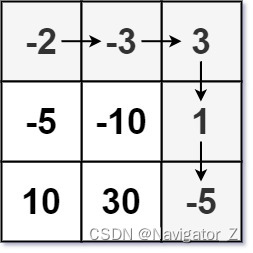
Input: dungeon = [[-2,-3,3],[-5,-10,1],[10,30,-5]]
Output: 7
Explanation: The initial health of the knight must be at least 7 if he follows the optimal path: RIGHT-> RIGHT -> DOWN -> DOWN.
Example 2:
Input: dungeon = [[0]]
Output: 1
Constraints:
- m == dungeon.length
- n == dungeon[i].length
- 1 <= m, n <= 200
- -1000 <= dungeon[i][j] <= 1000
From: LeetCode
Link: 174. Dungeon Game
Solution:
Ideas:
1. Dynamic Programming Table (DP Table):
- Create a 2D array dp where dp[i][j] stores the minimum initial health required to reach the princess starting from cell (i, j).
2. Base Case Initialization:
- For the princess's cell (m-1, n-1), set dp[m-1][n-1] to the maximum of 1 and 1 - dungeon[m-1][n-1].
3. Filling the DP Table:
- Last Column: Calculate minimum health for each cell in the last column based on the cell below it.
- Last Row: Calculate minimum health for each cell in the last row based on the cell to the right.
- Other Cells: For each cell (i, j), compute the minimum health based on the minimum of the right and below cells, adjusted by the current cell's value. If the resulting health is less than or equal to 0, set it to 1.
4. Result:
- The value in dp[0][0] represents the minimum initial health required for the knight to rescue the princess starting from the top-left corner.
Code:
c
int calculateMinimumHP(int** dungeon, int dungeonSize, int* dungeonColSize) {
int m = dungeonSize;
int n = dungeonColSize[0];
int dp[m][n];
dp[m-1][n-1] = dungeon[m-1][n-1] > 0 ? 1 : 1 - dungeon[m-1][n-1];
for (int i = m - 2; i >= 0; i--) {
dp[i][n-1] = dp[i+1][n-1] - dungeon[i][n-1];
if (dp[i][n-1] <= 0) dp[i][n-1] = 1;
}
for (int j = n - 2; j >= 0; j--) {
dp[m-1][j] = dp[m-1][j+1] - dungeon[m-1][j];
if (dp[m-1][j] <= 0) dp[m-1][j] = 1;
}
for (int i = m - 2; i >= 0; i--) {
for (int j = n - 2; j >= 0; j--) {
int min_health_on_exit = dp[i+1][j] < dp[i][j+1] ? dp[i+1][j] : dp[i][j+1];
dp[i][j] = min_health_on_exit - dungeon[i][j];
if (dp[i][j] <= 0) dp[i][j] = 1;
}
}
return dp[0][0];
}