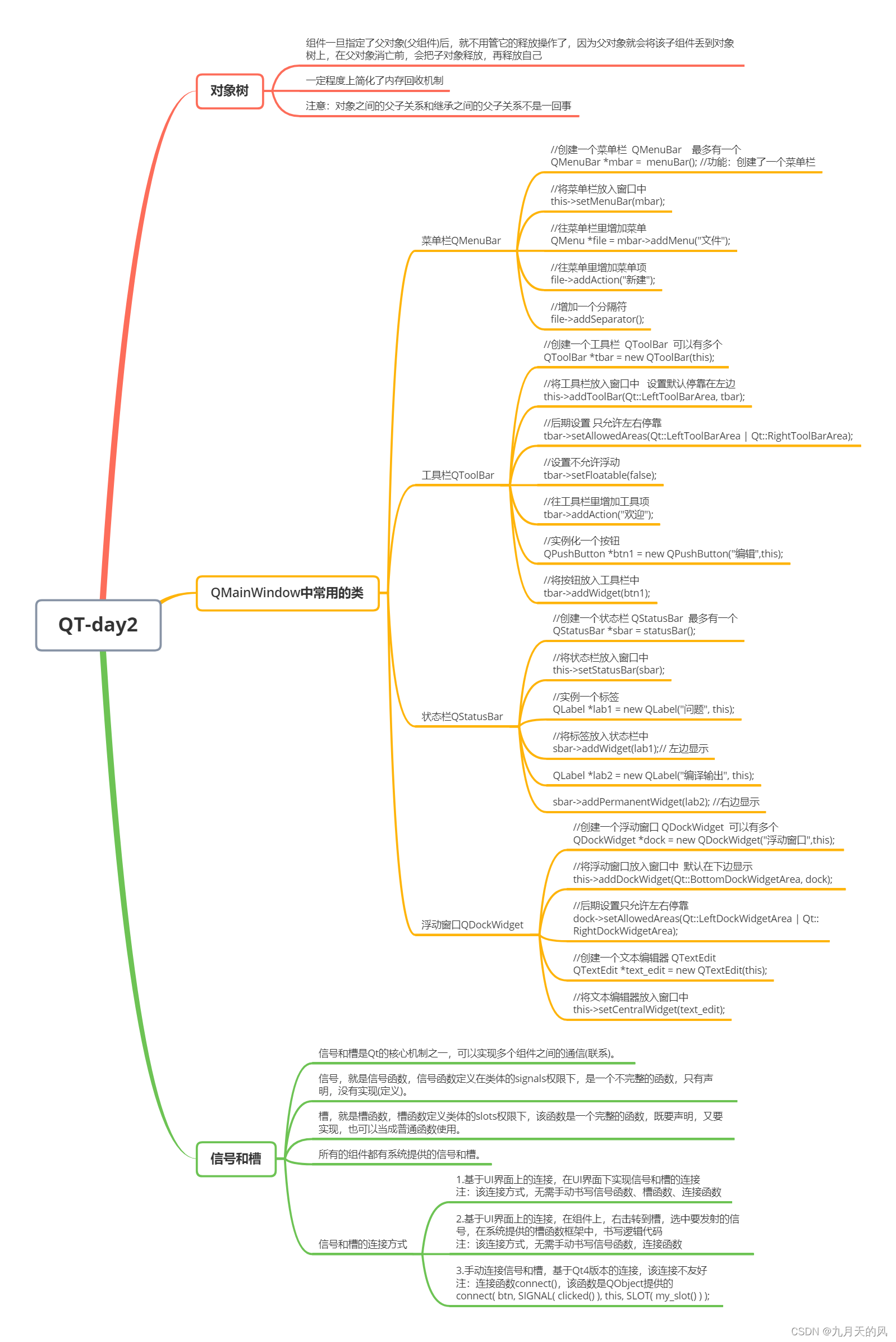
将登录按钮使用qt4版本的连接到自定义的槽函数中,在槽函数中判断ui界面上输入的账号是否为"admin",密码是否为"123456",如果账号密码匹配成功,则输出"登录成功",并关闭该界面,如果匹配失败,则输出登录失败,并将密码框中的内容清空
cpp
#include "myqq.h"
MyQQ::MyQQ(QWidget *parent)
:QWidget(parent)
,PNumLine(new QLineEdit(this))
,IdentifyLine(new QLineEdit(this))
,Msg(new QMessageBox(this))
{
//窗口相关设置
this->setWindowTitle("小黑的市集"); //重新设置窗口标题
this->setWindowIcon(QIcon(":/pictrue/xiaohei.jpeg")); //重新设置窗口图标
this->setFixedSize(500, 800); //重新设置窗口大小并固定
//手机区号前缀复选框
QComboBox *prefix = new QComboBox(this);
prefix->addItem("+86"); //中国大陆
prefix->addItem("+10"); //北京市
prefix->addItem("+21"); //上海市
prefix->resize(60, 30);
prefix->move(100, 200);
prefix->setStyleSheet("background-color:rgb(255,255,255)");
//手机号输入框
//QLineEdit *PNumLine = new QLineEdit(this); //创建行编辑器类对象
PNumLine->resize(240, 30); //重新设置大小
PNumLine->move(160, 200); //移动
PNumLine->setPlaceholderText("请输入手机号"); //占位
//验证码输入框
//QLineEdit *IdentifyLine = new QLineEdit(this); //创建行编辑器类对象
IdentifyLine->resize(300, 30); //重新设置大小
IdentifyLine->move(100, 250); //移动
IdentifyLine->setPlaceholderText("请输入验证码"); //占位
//登录按钮
QPushButton *LoginBtn = new QPushButton("登录", this); //创建一个按钮类
LoginBtn->resize(300, 30); //重新设置大小
LoginBtn->move(100, 320); //移动
LoginBtn->setStyleSheet("background-color:orange"); //设置按钮背景色
//密码登录按钮
QPushButton *PwdLoginBtn = new QPushButton("密码登录", this); //创建一个按钮类
PwdLoginBtn->resize(80, 30); //重新设置大小
PwdLoginBtn->move(100, 370); //移动
PwdLoginBtn->setStyleSheet("background-color:rgb(240, 240, 240)"); //设置按钮背景色
//无法登录按钮
QPushButton *NonLoginBtn = new QPushButton("无法登录?", this); //创建一个按钮类
NonLoginBtn->resize(80, 30); //重新设置大小
NonLoginBtn->move(320, 370); //移动
NonLoginBtn->setStyleSheet("background-color:rgb(240, 240, 240)"); //设置按钮背景色
//其他登录方式标签
QLabel *OtherLabel = new QLabel("--------- 其他方式登录 ---------", this); //创建一个标签类
OtherLabel->resize(200, 30); //重新设置大小
OtherLabel->move(150, 650); //移动
//QQ登录按钮
QPushButton *QQLoginBtn = new QPushButton(this); //创建一个按钮类
QQLoginBtn->resize(48, 48);
QQLoginBtn->move(150, 700); //移动
QQLoginBtn->setIcon(QIcon(":/pictrue/QQ-square-fill.png")); //填充图片
QQLoginBtn->setIconSize(this->size()); //图标设置为按钮大小
//微信登录按钮
QPushButton *WcLoginBtn = new QPushButton(this); //创建一个按钮类
WcLoginBtn->resize(48, 48);
WcLoginBtn->move(226, 700); //移动
WcLoginBtn->setIcon(QIcon(":/pictrue/110-wechat2.png")); //填充图片
WcLoginBtn->setIconSize(this->size()); //图标设置为按钮大小
//微博登录按钮
QPushButton *WbLoginBtn = new QPushButton(this); //创建一个按钮类
WbLoginBtn->resize(48, 48);
WbLoginBtn->move(302, 700); //移动
WbLoginBtn->setIcon(QIcon(":/pictrue/weibo-square-fill.png")); //填充图片
WbLoginBtn->setIconSize(this->size()); //图标设置为按钮大小
//背景动图设置
QLabel *BgdLabel = new QLabel(this); //创建一个标签类对象
QMovie *mv = new QMovie(":/pictrue/qq.gif"); //创建一个动图类对象
BgdLabel->resize(500, 180); //重新设置大小
BgdLabel->setMovie(mv); //填充动图
mv->start(); //开始
//消息对话框设置
Msg->setWindowTitle("提示");
//连接登录按钮的单击信号和当前窗口自定义的槽
connect(LoginBtn, SIGNAL(clicked()), this, SLOT(my_slot()));
}
MyQQ::~MyQQ()
{}
void MyQQ::my_slot()
{
if(PNumLine->text() == "admin" && IdentifyLine->text() == "123456"){
Msg->setText("登录成功");
Msg->open();
Msg->exec();
this->close();
}else{
IdentifyLine->clear();
Msg->setText("登录失败");
Msg->open();
Msg->exec();
}
}