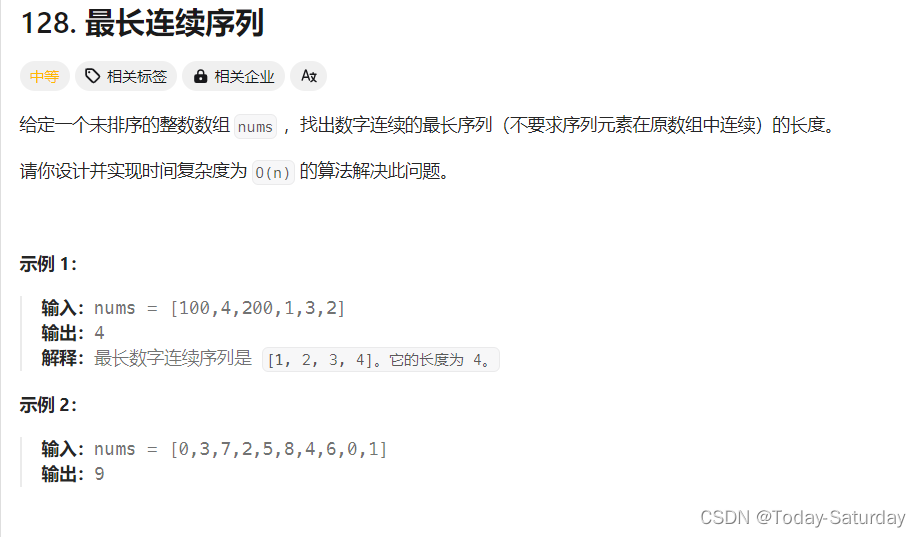
java
package com.lsy.leetcodehot100;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Set;
public class _Hot3_最长连续序列 {
public static int longestConsecutive(int[] nums) {
//创建set去重
//对重复的数字进行去重
Set<Integer> set = new HashSet<>();
//将数字存入set
for(int num:nums){
set.add(num);
}
//记录当前遍历的序列的最长长度
int max = 0;
for (int num : set) {
//记录当前的遍历的数字序列最后一个元素
int current = num;
//检查current是否是起始数字,如果在的话说明不是,如果不在说明是
if(!set.contains(current-1)){
//如果不在,就继续遍历元素,看后面还有没有连续的数字
while(set.contains(current+1)){
//有就自增记录当前的元素
current++;
}
}
//如果 num 是 5 而 cur 是 8,那么这个序列是 [5, 6, 7, 8],长度为 8 - 5 + 1 = 4
//如果重新计算的序列长度大于当前的max,就更新max的长度为最长长度
max = Math.max(max,current-num+1);
}
return max;
}
public static void main(String[] args) {
int[] nums= {1,3,2,0,5,6,9,8,7,3,0,3,5};
int max = longestConsecutive(nums);
System.out.println(max);
}
}