Python语言修改控制台输出文字的颜色和背景颜色
格式
\033[显示模式;字体颜色;背景颜色m
显示模式
显示模式 | 格式 |
---|---|
将文本颜色和背景颜色重置为默认值,取消所有其他文本属性 | \033[0m |
高亮(加粗) | \033[1m |
低亮 | \033[2m |
斜体 | \033[3m |
下划线 | \033[4m |
闪烁 | \033[5m |
快速闪烁 | \033[6m |
反显 | \033[7m |
隐藏 | \033[8m |
删除线 | \033[9m |
python
for i in range(0, 10):
print(f'\033[{i}m' + f'字体{i}' + '\033[0m')
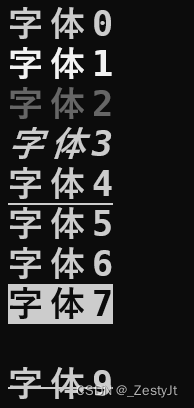
字体颜色
字体颜色 | 格式 |
---|---|
黑色 | \033[30m |
红色 | \033[31m |
绿色 | \033[32m |
黄色 | \033[33m |
蓝色 | \033[34m |
紫色 | \033[35m |
靓色 | \033[36m |
白色 | \033[37m |
自定义颜色 | \033[38m |
字体颜色重置为默认值 | \033[39m |
python
for i in range(0, 10):
print(f'\033[3{i}m' + f'字体颜色{i}' + '\033[0m')
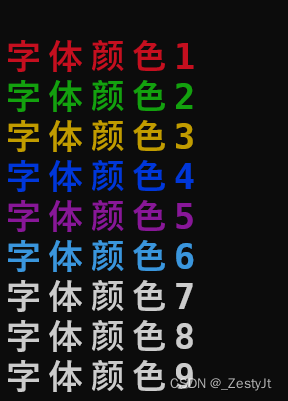
这里需要注意自定义颜色,它的格式为:
\033[38;5;颜色值m
颜色值可以取0-255之间的值
python
for i in range(0, 256):
if i % 10 == 0:
print()
print(f'\033[38;5;{i}m' + f'字体颜色' + '\033[0m', end = ' ')
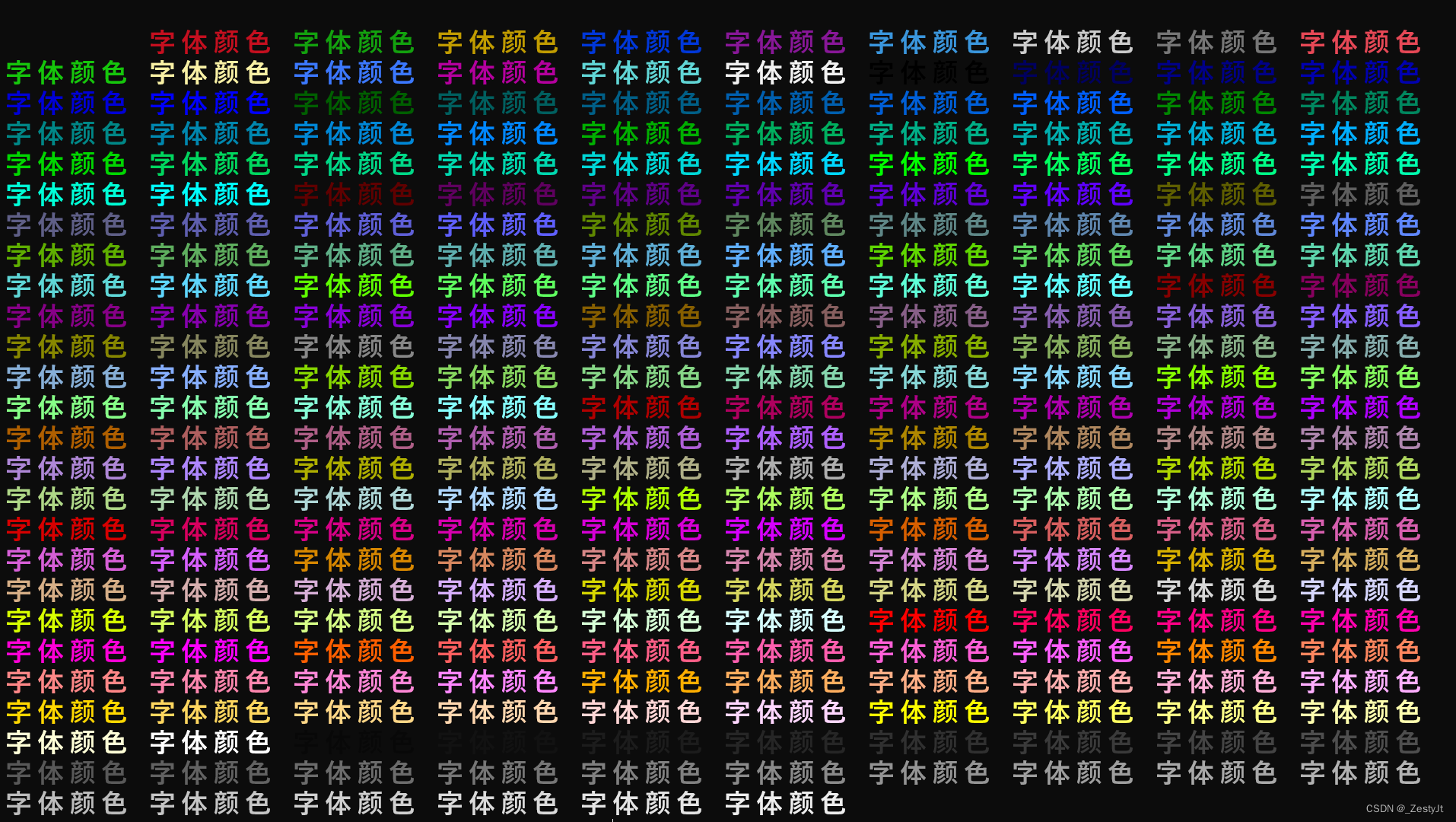
背景颜色
背景颜色 | 格式 |
---|---|
黑色 | \033[40m |
红色 | \033[41m |
绿色 | \033[42m |
黄色 | \033[43m |
蓝色 | \033[44m |
紫色 | \033[45m |
靓色 | \033[46m |
白色 | \033[47m |
自定义颜色 | \033[48m |
背景颜色重置为默认值 | \033[49m |
python
for i in range(0, 10):
print(f'\033[4{i}m' + f'背景颜色{i}' + '\033[0m')
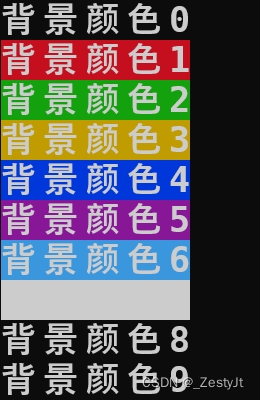
还是需要注意自定义颜色,它的格式为:
\033[48;5;颜色值m
颜色值可以取0-255之间的值
python
for i in range(0, 256):
if i % 10 == 0:
print()
print(f'\033[48;5;{i}m' + f'背景颜色' + '\033[0m', end = ' ')
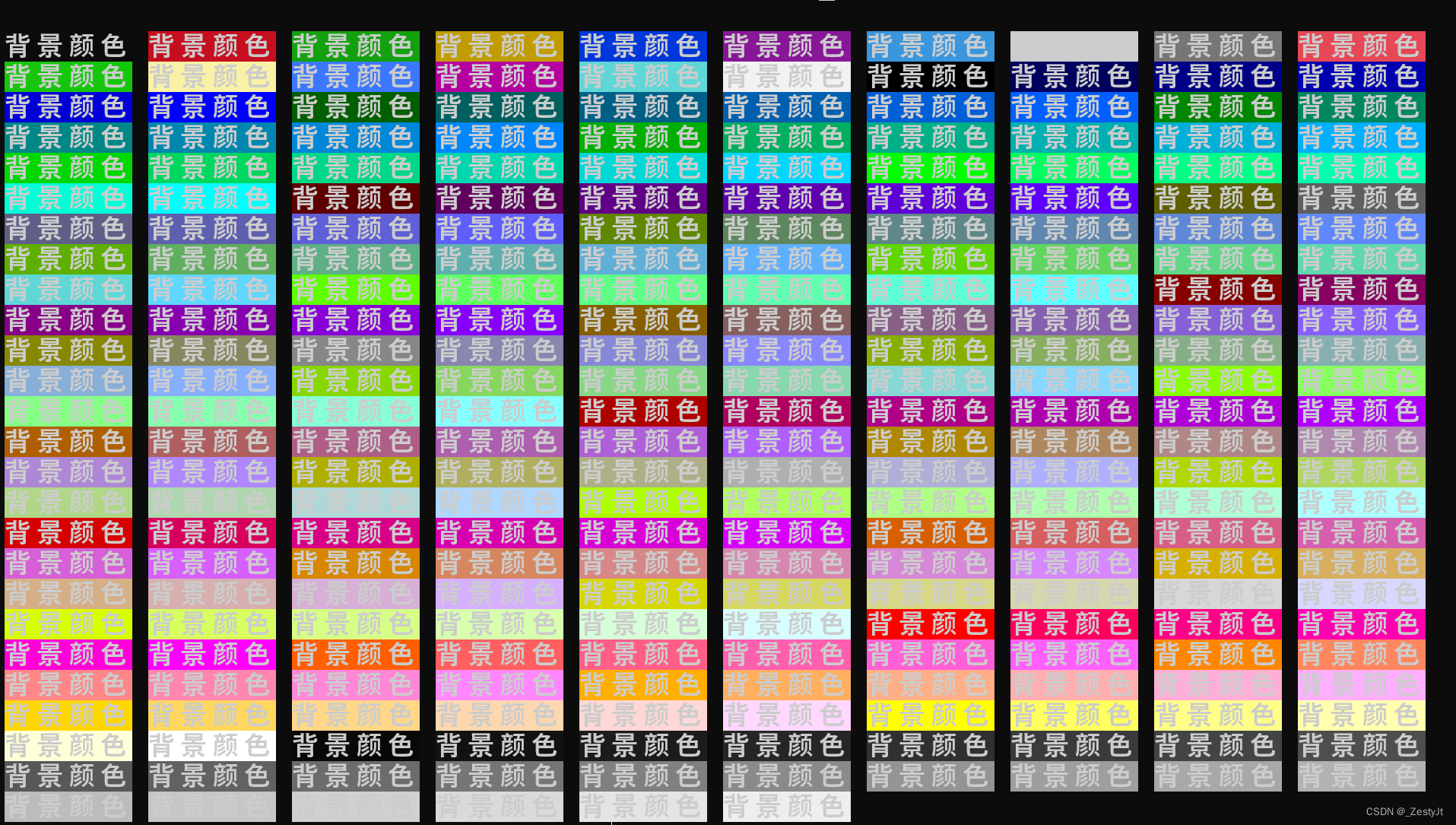
文字加效果显示类
写了一个给文字加入效果的类,可以指定颜色、显示模式、背景颜色。
python
import argparse
import subprocess
class DisplayMode:
def __init__(self):
self.display_mode = {
'reset': '\033[0m',
'highlight': '\033[1m',
'delight': '\033[2m',
'italic': '\033[3m',
'underline': '\033[4m',
'glitter': '\033[5m',
'glitter_quick': '\033[6m',
'invert': '\033[7m',
'hide': '\033[8m',
'delete': '\033[9m',
}
def __call__(self, mode):
return self.display_mode[mode]
class FontColor:
def __init__(self):
self.font_colors = {
'black': '\033[30m',
'red': '\033[31m',
'green': '\033[32m',
'yellow': '\033[33m',
'blue': '\033[34m',
'purple': '\033[35m',
'cyan': '\033[36m',
'white': '\033[37m',
'custom': '\033[38;5;{color}m'
}
def __call__(self, mode, color=None):
if mode == 'custom' and color is None:
print(self.font_colors['red'] + '自定义背景字体没有设置' + DisplayMode()('reset'))
elif mode == 'custom' and color is not None:
return self.font_colors[mode].replace('{color}', str(color))
else:
return self.font_colors[mode]
class BackgroundColors:
def __init__(self):
self.background_colors = {
'black': '\033[40m',
'red': '\033[41m',
'green': '\033[42m',
'yellow': '\033[43m',
'blue': '\033[44m',
'purple': '\033[45m',
'cyan': '\033[46m',
'white': '\033[47m',
'custom': '\033[48;5;{color}m'
}
def __call__(self, mode, color=None):
if mode == 'custom' and color is None:
print(self.background_colors['red'] + '自定义背景颜色没有设置' + DisplayMode()('reset'))
elif mode == 'custom' and color is not None:
return self.background_colors[mode].replace('{color}', str(color))
else:
return self.background_colors[mode]
def print_text(text, display_mode=None, font_color=None, bg_color=None, end=None, **kwargs):
"""
彩色输出控制台内容
:param text: 输出的内容
:param display_mode: 显示模式
reset: 重置样式,
highlight: 高亮,
delight: 低亮,
italic: 斜体,
underline: 下划线,
glitter: 闪烁,
glitter_quick: 快速闪烁,
invert: 反显,
hide: 隐藏(不显示),
delete: 删除线
:param font_color: 字体颜色
black: 黑色,
red: 红色,
green: 绿色,
yellow: 黄色,
blue: 蓝色,
purple: 紫色,
cyan: 靓色,
white: 白色,
custom: 自定义颜色,传入参数fc=颜色,来指定颜色,值在0-255
:param bg_color:
black: 黑色,
red: 红色,
green: 绿色,
yellow: 黄色,
blue: 蓝色,
purple: 紫色,
cyan: 靓色,
white: 白色,
custom: 自定义颜色,传入参数bg=颜色,来指定颜色,值在0-255
:param kwargs:
fc指定字体自定义颜色,bg指定背景颜色自定义颜色,值在0-255
Example
print_text('Hello World', 'italic', fc=44, bg=23)
"""
if display_mode is None:
display_mode = ''
else:
display_mode = DisplayMode()(display_mode)
if font_color is None or font_color == 'custom':
if kwargs.__contains__('fc'):
fc = kwargs['fc']
font_color = FontColor()('custom', fc)
else:
font_color = ''
else:
font_color = FontColor()(font_color)
if bg_color is None or bg_color == 'custom':
if kwargs.__contains__('bg'):
bg = kwargs['bg']
bg_color = BackgroundColors()('custom', bg)
else:
bg_color = ''
else:
bg_color = BackgroundColors()(bg_color)
text = str(text)
if end is None:
print(display_mode + font_color + bg_color + text + DisplayMode()('reset'))
else:
print(display_mode + font_color + bg_color + text + DisplayMode()('reset'), end=end)
def pct(text, **kwargs):
"""
彩色输出控制台内容
:param text: 输出的内容
:param 显示模式
%
reset: 重置样式,
highlight: 高亮,
delight: 低亮,
italic: 斜体,
underline: 下划线,
glitter: 闪烁,
glitter_quick: 快速闪烁,
invert: 反显,
hide: 隐藏(不显示),
delete: 删除线
:param: 字体颜色
$
black: 黑色, 1
red: 红色, 2
green: 绿色, 3
yellow: 黄色, 4
blue: 蓝色, 5
purple: 紫色, 6
cyan: 靓色, 7
white: 白色, 8
custom: 自定义颜色,传入参数fc=颜色,来指定颜色,值在0-255, 9
:param: 背景颜色
&
black: 黑色, 1
red: 红色, 2
green: 绿色, 3
yellow: 黄色, 4
blue: 蓝色, 5
purple: 紫色, 6
cyan: 靓色, 7
white: 白色, 8
custom: 自定义颜色,bg=颜色,来指定颜色,值在0-255, 9
:param kwargs:
fc指定字体自定义颜色,bg指定背景颜色自定义颜色,值在0-255
Example
text = '''
%hl+$4+Warning:^- %i+&9+this method is discarded.^-
'''
"""
display_mode = DisplayMode()
font_color = FontColor()
bg_color = BackgroundColors()
text = text.replace('^-', display_mode('reset'))
text = text.replace('%hl+', display_mode('highlight'))
text = text.replace('%dl+', display_mode('delight'))
text = text.replace('%i+', display_mode('italic'))
text = text.replace('%u+', display_mode('underline'))
text = text.replace('%g+', display_mode('glitter'))
text = text.replace('%gq+', display_mode('glitter_quick'))
text = text.replace('%in+', display_mode('invert'))
text = text.replace('%h+', display_mode('hide'))
text = text.replace('%d+', display_mode('delete'))
colors = [
{"name": "black", "chinese": "黑色", "id": 1, "description": "黑色, 1"},
{"name": "red", "chinese": "红色", "id": 2, "description": "红色, 2"},
{"name": "green", "chinese": "绿色", "id": 3, "description": "绿色, 3"},
{"name": "yellow", "chinese": "黄色", "id": 4, "description": "黄色, 4"},
{"name": "blue", "chinese": "蓝色", "id": 5, "description": "蓝色, 5"},
{"name": "purple", "chinese": "紫色", "id": 6, "description": "紫色, 6"},
{"name": "cyan", "chinese": "靓色", "id": 7, "description": "靓色, 7"},
{"name": "white", "chinese": "白色", "id": 8, "description": "白色, 8"},
{"name": "custom", "chinese": "自定义颜色", "id": 9,
"description": "自定义颜色,传入参数fc=颜色,来指定颜色,值在0-255"}
]
bg = 256
if kwargs.__contains__('bg'):
bg = kwargs['bg']
fc = 256
if kwargs.__contains__('fc'):
fc = kwargs['fc']
for i in range(9):
text = text.replace(f'${i + 1}+', font_color(colors[i]['name'], fc))
for i in range(9):
text = text.replace(f'&{i + 1}+', bg_color(colors[i]['name'], bg))
print(text)
if __name__ == '__main__':
pct('%hl+$4+Warning:^- %i+&9+this method is discarded.^-')
print_text('Hello World', 'italic', fc=44, bg=23)
运行结果
