目录
- 题目
- [1- 思路](#1- 思路)
- [2- 实现](#2- 实现)
-
- [⭐236. 二叉树的最近公共祖先------题解思路](#⭐236. 二叉树的最近公共祖先——题解思路)
- [3- ACM实现](#3- ACM实现)
题目
- 原题连接:236. 二叉树的最近公共祖先
1- 思路
模式识别
- 模式1:二叉树最近公共祖先 ------> 递归 + 判断
递归思路,分情况判断:
- 1.参数及返回值
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q)
- 2.递归终止条件
- 遇到
null
、root == p
、root = q
的情况,此时递归结束。
- 遇到
- 3.单层递归逻辑 4 种情况
- 递归 left 赋值
- 递归 right 赋值
- ① 左右都为 null、此时返回 null
- ② 左不
**null**
、右**null**
返回左 - ③ 左
**null**
、右不**null**
返回右 - ④ 直接返回 root
2- 实现
⭐236. 二叉树的最近公共祖先------题解思路
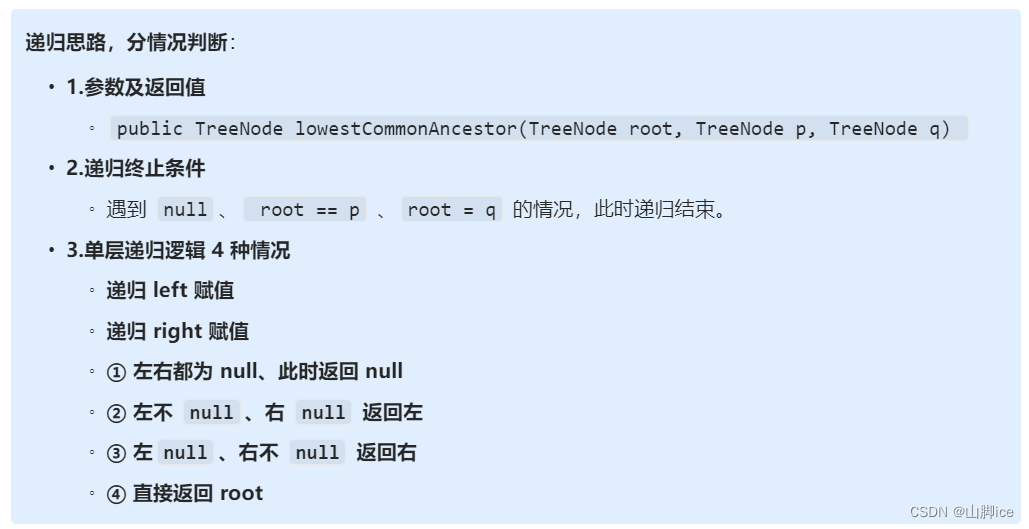
java
class Solution {
public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if(root==null || root==p || root==q){
return root;
}
TreeNode left = lowestCommonAncestor(root.left,p,q);
TreeNode right = lowestCommonAncestor(root.right,p,q);
if(left==null && right==null){
return null;
}else if(left!=null && right==null){
return left;
}else if(left==null && right!=null){
return right;
}else{
return root;
}
}
}
3- ACM实现
java
public class lowestCommonAncestor {
static class TreeNode{
int val;
TreeNode left;
TreeNode right;
TreeNode(){}
TreeNode(int x){
val = x;
}
}
public static TreeNode build(Integer[] nums){
TreeNode root = new TreeNode(nums[0]);
Queue<TreeNode> queue =new LinkedList<>();
queue.offer(root);
int index = 1;
while(!queue.isEmpty() && index < nums.length){
TreeNode node = queue.poll();
if(nums[index]!=null && index<nums.length){
node.left = new TreeNode(nums[index]);
queue.offer(node.left);
}
index++;
if(nums[index]!=null && index<nums.length){
node.right = new TreeNode(nums[index]);
queue.offer(node.right);
}
index++;
}
return root;
}
public static TreeNode findOne(TreeNode root,int val){
if(root==null) return root;
if(root.val == val) return root;
TreeNode left = findOne(root.left,val);
if(left!=null) return left;
return findOne(root.right,val);
}
public static TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if(root==null || root==p || root==q){
return root;
}
TreeNode left = lowestCommonAncestor(root.left,p,q);
TreeNode right = lowestCommonAncestor(root.right,p,q);
if(left==null && right==null){
return null;
}else if(left!=null && right==null){
return left;
}else if(left==null && right!=null){
return right;
}else{
return root;
}
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("输入二叉树构造数组");
String input = sc.nextLine();
input = input.replace("[","");
input = input.replace("]","");
String[] parts = input.split(",");
Integer[] nums = new Integer[parts.length];
for(int i = 0 ; i <parts.length;i++){
if(!parts[i].equals("null")){
nums[i] = Integer.parseInt(parts[i]);
}else{
nums[i] = null;
}
}
TreeNode root = build(nums);
System.out.println("输入val1");
TreeNode p = findOne(root,sc.nextInt());
System.out.println("输入val2");
TreeNode q = findOne(root,sc.nextInt());
TreeNode res = lowestCommonAncestor(root,p,q);
System.out.println("公共父节点值为"+res.val);
}
}