一.修改第三人称模板的 Charactor
1.随鼠标将四处看的功能的输入注释掉。
cpp
void ARunGANCharacter::SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent)
{
// Set up action bindings
if (UEnhancedInputComponent* EnhancedInputComponent = CastChecked<UEnhancedInputComponent>(PlayerInputComponent)) {
//Jumping
EnhancedInputComponent->BindAction(JumpAction, ETriggerEvent::Triggered, this, &ACharacter::Jump);
EnhancedInputComponent->BindAction(JumpAction, ETriggerEvent::Completed, this, &ACharacter::StopJumping);
//Moving
EnhancedInputComponent->BindAction(MoveAction, ETriggerEvent::Triggered, this, &ARunGANCharacter::Move);
//Looking
//EnhancedInputComponent->BindAction(MoveAction, ETriggerEvent::Triggered, this, &ARunGANCharacter::Look);
}
2.在Tick里,注释掉计算左右方向的部分。只让它获得向前的方向。再加个每帧都朝,正前方输入的逻辑。
cpp
void ARunGANCharacter::Tick(float DeltaSeconds)
{
Super::Tick(DeltaSeconds);
GetController()->SetControlRotation(FMath::RInterpConstantTo(GetControlRotation(),DesireRotation,GetWorld()->GetRealTimeSeconds(),10.f));
//
const FRotator Rotation = Controller->GetControlRotation();
const FRotator YawRotation(0, Rotation.Yaw, 0);
// get forward vector
const FVector ForwardDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::X);
// get right vector
//const FVector RightDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::Y);
//
AddMovementInput(ForwardDirection, 1);
}
3.修改按下输入,调用的Move里的逻辑。让它如果转向,就按自己输入的X方向。旋转90度。
如果不转向,按照人物控制当前的朝向,计算左右的向量,并添加左右输入。
相当于一直向前跑,不转向可以左右调整。
cpp
if (bTurn)
{
//GEngine->AddOnScreenDebugMessage(-1,5.0f,FColor::Red,TEXT("Turn"));
FRotator NewRotation = FRotator(0.f,90.f*(MovementVector.X), 0.f);
FQuat QuatA = FQuat(DesireRotation);
FQuat QuatB = FQuat(NewRotation);
DesireRotation = FRotator(QuatA * QuatB);
bTurn = false;
}
else
{
// find out which way is forward
const FRotator Rotation = Controller->GetControlRotation();
const FRotator YawRotation(0, Rotation.Yaw, 0);
// get forward vector
//const FVector ForwardDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::X);
// get right vector
const FVector RightDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::Y);
//ForwardDirection = FVector(0,0,0);
// add movement
//AddMovementInput(ForwardDirection, 0); //
AddMovementInput(RightDirection, MovementVector.X);
/* if (Controller->IsLocalPlayerController())
{
APlayerController* const PC = CastChecked<APlayerController>(Controller);
}*/
//GetCharacterMovement()->bOrientRotationToMovement = false;
//
}
4.这里转向的方向DesireRotation,会被一直赋值到控制器(Controller->GetControlRotation)。进而影响我们 向前方向。因为Tick里一直在修正。左右会在,Move回调函数里添加,也受控制器的影响。
cpp
void ARunGANCharacter::Tick(float DeltaSeconds)
{
Super::Tick(DeltaSeconds);
GetController()->SetControlRotation(FMath::RInterpConstantTo(GetControlRotation(),DesireRotation,GetWorld()->GetRealTimeSeconds(),10.f));
//
const FRotator Rotation = Controller->GetControlRotation();
const FRotator YawRotation(0, Rotation.Yaw, 0);
// get forward vector
const FVector ForwardDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::X);
// get right vector
//const FVector RightDirection = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::Y);
//
AddMovementInput(ForwardDirection, 1);
}
人物逻辑就写好了,一直跑,Turn为True时转90.其余时间,相当于一直按W,你自己决定要不要A,D,斜向跑。
二.写碰撞,碰撞时,能实现转向。
创建TurnBox C++ Actor类。在里面,添加UBoxComponent组件,添加碰撞的回调函数。内容也很简单,如果是 角色碰撞,让它的装箱变量变为True。
cpp
#include "TurnBox.generated.h"
class UBoxComponent;
UCLASS()
class RUNGAN_API ATurnBox : public AActor
{
GENERATED_BODY()
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = Box,meta = (AllowPrivateAccess = "true"))
UBoxComponent* Box;
public:
// Sets default values for this actor's properties
ATurnBox();
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
UFUNCTION()
void CharacterOverlapStart(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult);
// UPrimitiveComponent, OnComponentBeginOverlap, UPrimitiveComponent*, OverlappedComponent, AActor*, OtherActor, UPrimitiveComponent*, OtherComp, int32, OtherBodyIndex, bool, bFromSweep, const FHitResult &, SweepResult);
UFUNCTION()
void CharacterOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex);
//UPrimitiveComponent*, OverlappedComponent, AActor*, OtherActor, UPrimitiveComponent*, OtherComp, int32, OtherBodyIndex);
};
这里实例化组件,就不写了。把角色的头文件,包含进去。绑定回调,回调里判断逻辑。
cpp
// Called when the game starts or when spawned
void ATurnBox::BeginPlay()
{
Super::BeginPlay();
Box->OnComponentBeginOverlap.AddDynamic(this,&ATurnBox::CharacterOverlapStart);
Box->OnComponentEndOverlap.AddDynamic(this, &ATurnBox::CharacterOverlapEnd);
}
// Called every frame
void ATurnBox::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
void ATurnBox::CharacterOverlapStart(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (ARunGANCharacter* InCharacter = Cast<ARunGANCharacter>(OtherActor))
{
InCharacter->bTurn = true;
}
}
void ATurnBox::CharacterOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex)
{
if (ARunGANCharacter* InCharacter = Cast<ARunGANCharacter>(OtherActor))
{
InCharacter->bTurn = false;
}
}
三.开始写路的逻辑,随机生成。
1.准备好道路资源
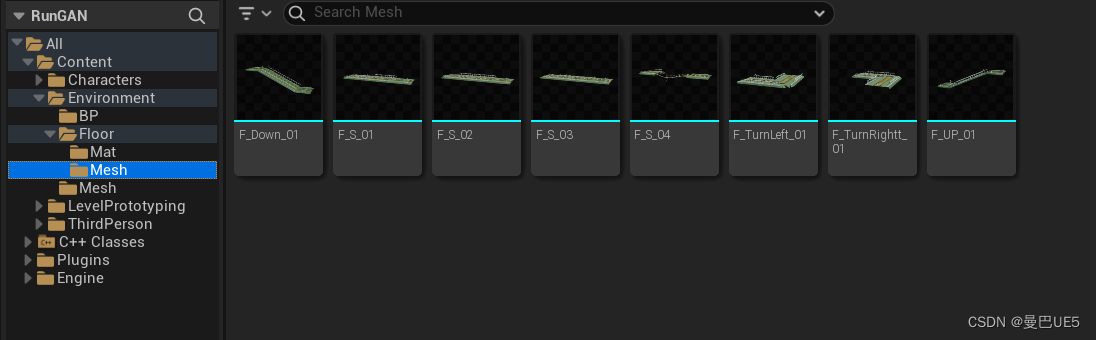
2.这里将道路,分为直道,转弯道,上下道。使用了UE创建枚举的方式。
cpp
#pragma once
#include"CoreMinimal.h"
#include "RunGANType.generated.h"
UENUM()
enum class FRoadType :uint8 //只需要一个字节,更高效 0-255
{
StraitFloor,
TurnFloor,
UPAndDownFloor,
MAX,
};
3.然后我们创建道路类,写上通用逻辑。在头文件,将道路类型加上,并前项声明 指针 指向的组件类。
cpp
#include "../../RunGANType.h"
#include "RunRoad.generated.h"
class UBoxComponent;
class USceneComponent;
class UStaticMeshComponent;
class UArrowComponent;
UCLASS()
class RUNGAN_API ARunRoad : public AActor
{
GENERATED_BODY()
//场景组件
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J", meta = (AllowPrivateAccess = "true"))
USceneComponent* SceneComponent;
//根组件
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J", meta = (AllowPrivateAccess = "true"))
USceneComponent* RunRoadRootComponent;
//碰撞
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J",meta = (AllowPrivateAccess = "true"))
UBoxComponent* BoxComponent;
//模型
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J",meta = (AllowPrivateAccess = "true"))
UStaticMeshComponent* RoadMesh;
//方向
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J",meta = (AllowPrivateAccess = "true"))
UArrowComponent* SpawnPointMiddle;
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J",meta = (AllowPrivateAccess = "true"))
UArrowComponent* SpawnPointRight;
UPROPERTY(VisibleAnywhere,BlueprintReadOnly,Category = "C_J",meta = (AllowPrivateAccess = "true"))
UArrowComponent* SpawnPointLeft;
//地板类型
UPROPERTY(EditDefaultsOnly,Category = "TowerType") //EditDefaultsOnly:蓝图可以编译,但是在主编译器不显示所以不可以编译
FRoadType RoadType;
public:
// Sets default values for this actor's properties
ARunRoad();
FTransform GetAttackToTransform(const FVector& MyLocation);
protected:
// Called when the game starts or when spawned
virtual void BeginPlay() override;
public:
// Called every frame
virtual void Tick(float DeltaTime) override;
UFUNCTION()
void CharacterOverlapStart(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult);
// UPrimitiveComponent, OnComponentBeginOverlap, UPrimitiveComponent*, OverlappedComponent, AActor*, OtherActor, UPrimitiveComponent*, OtherComp, int32, OtherBodyIndex, bool, bFromSweep, const FHitResult &, SweepResult);
UFUNCTION()
void CharacterOverlapEnd(UPrimitiveComponent* OverlappedComponent, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex);
4.实现逻辑
cpp
#include"Components/BoxComponent.h"
#include"Components/SceneComponent.h"
#include"Components/StaticMeshComponent.h"
#include"Components/ArrowComponent.h"
CreateDefaultSubobject<T>实例化组件,SetupAttachment添加组件,Root根组件,Father在根组件下,其余在Father组件下。
cpp
ARunRoad::ARunRoad()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = false;
//generateBox = CreateDefaultSubobject<UBoxComponent>(TEXT("GenerateBox"));
//实例化
SceneComponent = CreateDefaultSubobject<USceneComponent>(TEXT("Father"));
RunRoadRootComponent = CreateDefaultSubobject<USceneComponent>(TEXT("Root"));
RootComponent = RunRoadRootComponent;
RoadMesh = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("RoadMesh"));
BoxComponent = CreateDefaultSubobject<UBoxComponent>(TEXT("Box"));
SpawnPointMiddle = CreateDefaultSubobject<UArrowComponent>(TEXT("SpawnPointMiddle"));
SpawnPointRight = CreateDefaultSubobject<UArrowComponent>(TEXT("SpawnPointRight"));
SpawnPointLeft = CreateDefaultSubobject<UArrowComponent>(TEXT("SpawnPointLeft"));
//附加顺序
SceneComponent->SetupAttachment(RootComponent);
RoadMesh->SetupAttachment(SceneComponent);
BoxComponent->SetupAttachment(SceneComponent);
SpawnPointMiddle->SetupAttachment(SceneComponent);
SpawnPointRight->SetupAttachment(SceneComponent);
SpawnPointLeft->SetupAttachment(SceneComponent);
}
这样初步就将路结构搭建好了。后续开始写GameMode生成每一个路面。