文章目录
1、什么lua
"Lua"的英文全称是"Lightweight Userdata Abstraction Layer",意思是"轻量级用户数据抽象层"。
2、创建SpringBoot工程
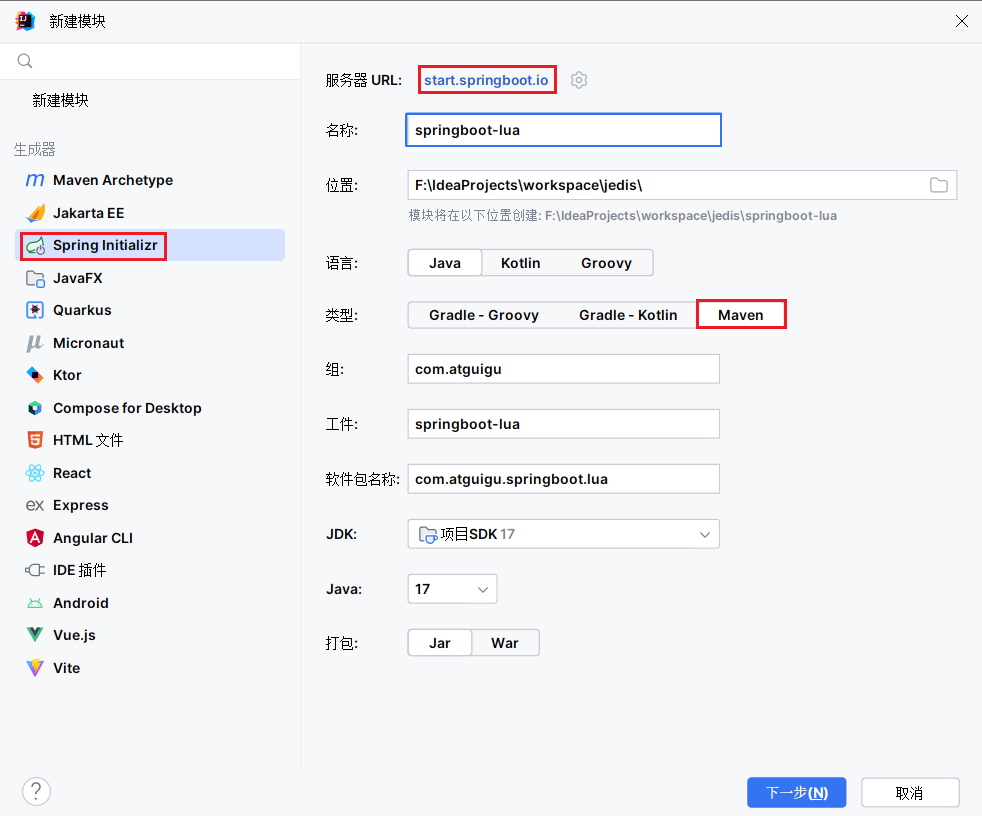
3、引入相关依赖
xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.0.5</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<!-- Generated by https://start.springboot.io -->
<!-- 优质的 spring/boot/data/security/cloud 框架中文文档尽在 => https://springdoc.cn -->
<groupId>com.atguigu</groupId>
<artifactId>springboot-lua</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>springboot-lua</name>
<description>springboot-lua</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
4、创建LUA脚本
bash
local current = redis.call('GET',KEYS[1])
if current == ARGV[1]
then redis.call('SET',KEYS[1],ARGV[2])
return true
else
redis.call('SET',KEYS[1],ARGV[3])
return true
end
return false
5、创建配置类
java
package com.atguigu.lua.config;
import org.springframework.context.annotation.Bean;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.script.RedisScript;
import org.springframework.data.redis.serializer.StringRedisSerializer;
import org.springframework.stereotype.Component;
@Component
public class AppRedisConfiguration {
//简单序列化
@Bean
public RedisTemplate<String,String> redisTemplate(RedisConnectionFactory factory) {
RedisTemplate<String,String> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(factory);
// 设置键序列化方式
redisTemplate.setKeySerializer(new StringRedisSerializer());
// 设置简单类型值的序列化方式
redisTemplate.setValueSerializer(new StringRedisSerializer());
// 设置默认序列化方式
redisTemplate.setDefaultSerializer(new StringRedisSerializer());
redisTemplate.afterPropertiesSet();
return redisTemplate;
}
//加载lua脚本,设置返回值类型
@Bean
public RedisScript<Boolean> script() {
Resource scriptSource = new ClassPathResource("lua/test.lua");
return RedisScript.of(scriptSource, Boolean.class);
}
}
6、创建启动类
java
package com.atguigu.lua;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
// Generated by https://start.springboot.io
// 优质的 spring/boot/data/security/cloud 框架中文文档尽在 => https://springdoc.cn
@SpringBootApplication
public class SpringbootLuaApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootLuaApplication.class, args);
}
}
7、创建测试类
java
package com.atguigu.lua;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.script.RedisScript;
import java.util.List;
@SpringBootTest
class SpringbootLuaApplicationTests {
@Autowired
private RedisTemplate redisTemplate;
@Autowired
private RedisScript redisScript;
@Test
void contextLoads() {
redisTemplate.execute(redisScript, List.of("str"),"spring","mybatis","springboot");
}
}
bash
127.0.0.1:6379> keys *
1) "balance"
2) "str"
127.0.0.1:6379> get str
"springboot"
127.0.0.1:6379>
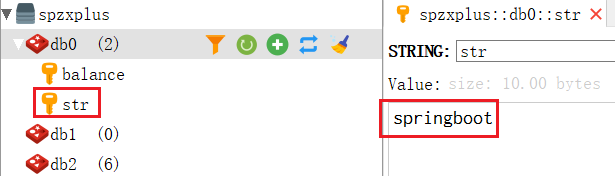