1、添加窗体
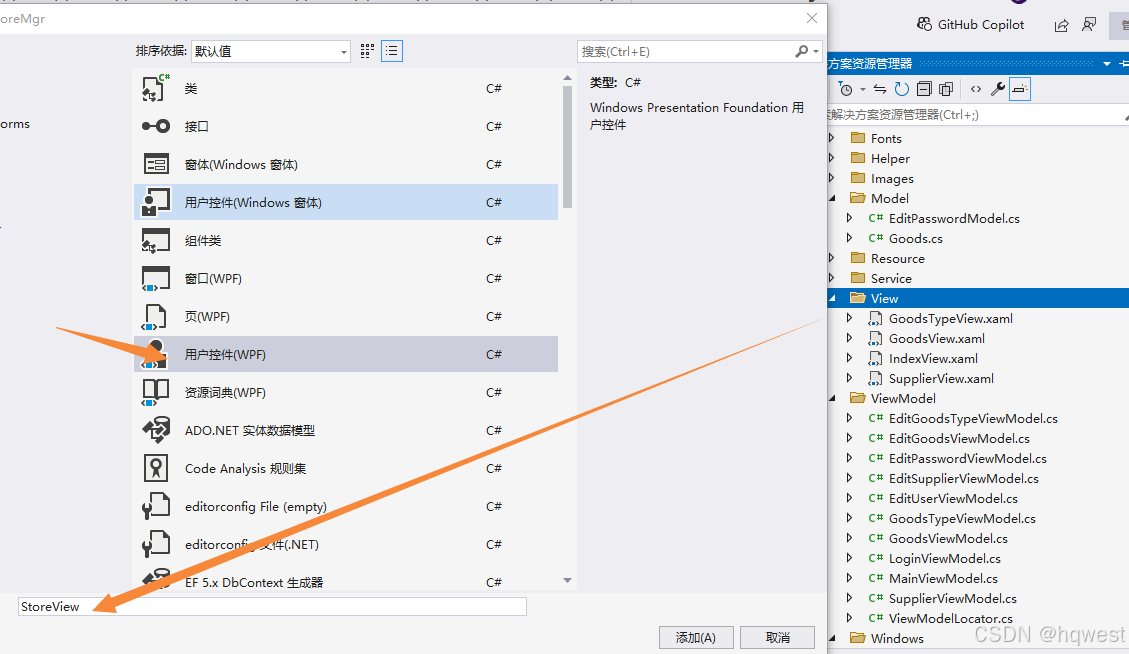
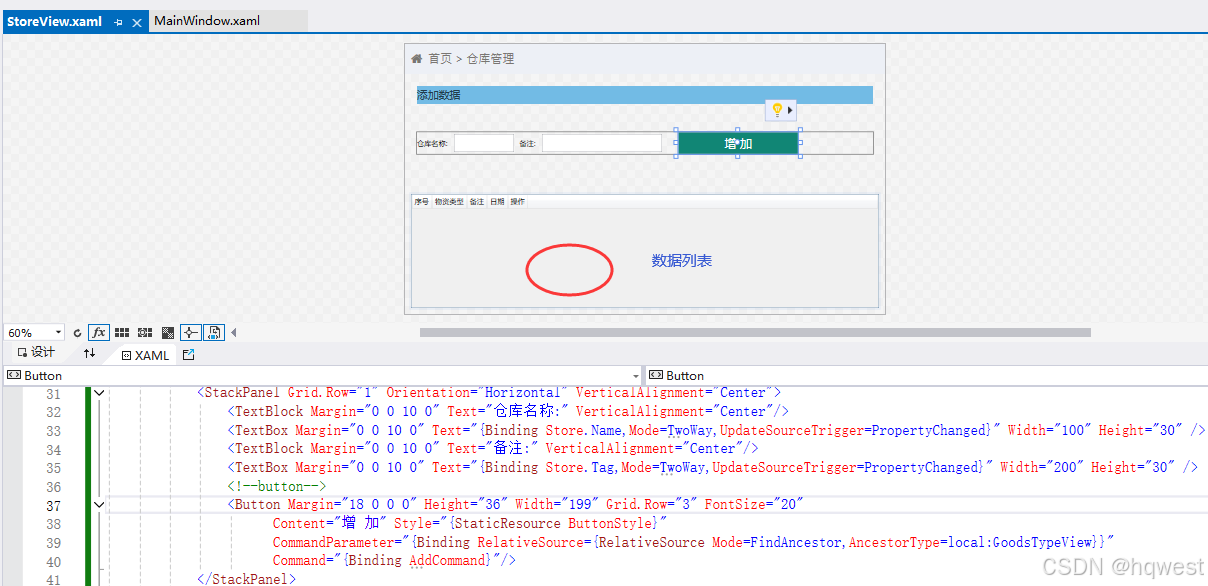
cs
<UserControl x:Class="West.StoreMgr.View.StoreView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:West.StoreMgr.View"
mc:Ignorable="d"
DataContext="{Binding Source={StaticResource Locator},Path=Store}"
d:DesignHeight="450" d:DesignWidth="800">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="50"/>
<RowDefinition/>
<RowDefinition/>
</Grid.RowDefinitions>
<!--标题-->
<StackPanel Background="#EDF0F6" Orientation="Horizontal">
<TextBlock Margin="10 0 0 0" Text="" FontSize="20" FontFamily="/Fonts/#FontAwesome" HorizontalAlignment="Left" VerticalAlignment="Center" Foreground="#797672"/>
<TextBlock Margin="10 0 0 0" Text="首页 > 仓库管理" FontSize="20" FontFamily="/Fonts/#FontAwesome" HorizontalAlignment="Left" VerticalAlignment="Center" Foreground="#797672"/>
</StackPanel>
<!--增加-->
<Grid Grid.Row="1" Margin="20">
<Grid.RowDefinitions>
<RowDefinition Height="30"/>
<RowDefinition/>
</Grid.RowDefinitions>
<Border Background="#72BBE5">
<TextBlock Text="添加数据" FontSize="18" VerticalAlignment="Center" Foreground="#1F3C4C" Margin="0 0 10 0"/>
</Border>
<StackPanel Grid.Row="1" Orientation="Horizontal" VerticalAlignment="Center">
<TextBlock Margin="0 0 10 0" Text="仓库名称:" VerticalAlignment="Center"/>
<TextBox Margin="0 0 10 0" Text="{Binding Store.Name,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}" Width="100" Height="30" />
<TextBlock Margin="0 0 10 0" Text="备注:" VerticalAlignment="Center"/>
<TextBox Margin="0 0 10 0" Text="{Binding Store.Tag,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}" Width="200" Height="30" />
<!--button-->
<Button Margin="18 0 0 0" Height="36" Width="199" Grid.Row="3" FontSize="20"
Content="增 加" Style="{StaticResource ButtonStyle}"
CommandParameter="{Binding RelativeSource={RelativeSource Mode=FindAncestor,AncestorType=local:GoodsTypeView}}"
Command="{Binding AddCommand}"/>
</StackPanel>
</Grid>
<!--浏览-->
<Grid Grid.Row="2" Margin="10 0 10 10">
<DataGrid ItemsSource="{Binding StoreList}" CanUserAddRows="False" AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="序号" Binding="{Binding Id}"/>
<DataGridTextColumn Header="物资类型" Binding="{Binding Name}"/>
<DataGridTextColumn Header="备注" Binding="{Binding Tag}"/>
<DataGridTextColumn Header="日期" Binding="{Binding InsertDate}"/>
<DataGridTemplateColumn Header="操作">
<DataGridTemplateColumn.CellTemplate>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<Button Content="编辑"
Command="{Binding RelativeSource={RelativeSource Mode=FindAncestor,AncestorType=local:StoreView},Path=DataContext.EditCommand}"
CommandParameter="{Binding RelativeSource={RelativeSource Mode=Self}}"
Tag="{Binding}"
Style="{StaticResource DataGridButtonStyle}" />
<Button Content="删除"
Command="{Binding RelativeSource={RelativeSource Mode=FindAncestor,AncestorType=local:StoreView},Path=DataContext.DeleteCommand}"
CommandParameter="{Binding RelativeSource={RelativeSource Mode=Self}}"
Tag="{Binding}"
Style="{StaticResource DataGridButtonStyle}" />
</StackPanel>
</DataTemplate>
</DataGridTemplateColumn.CellTemplate>
</DataGridTemplateColumn>
</DataGrid.Columns>
</DataGrid>
</Grid>
</Grid>
</UserControl>
2、添加viewmodel
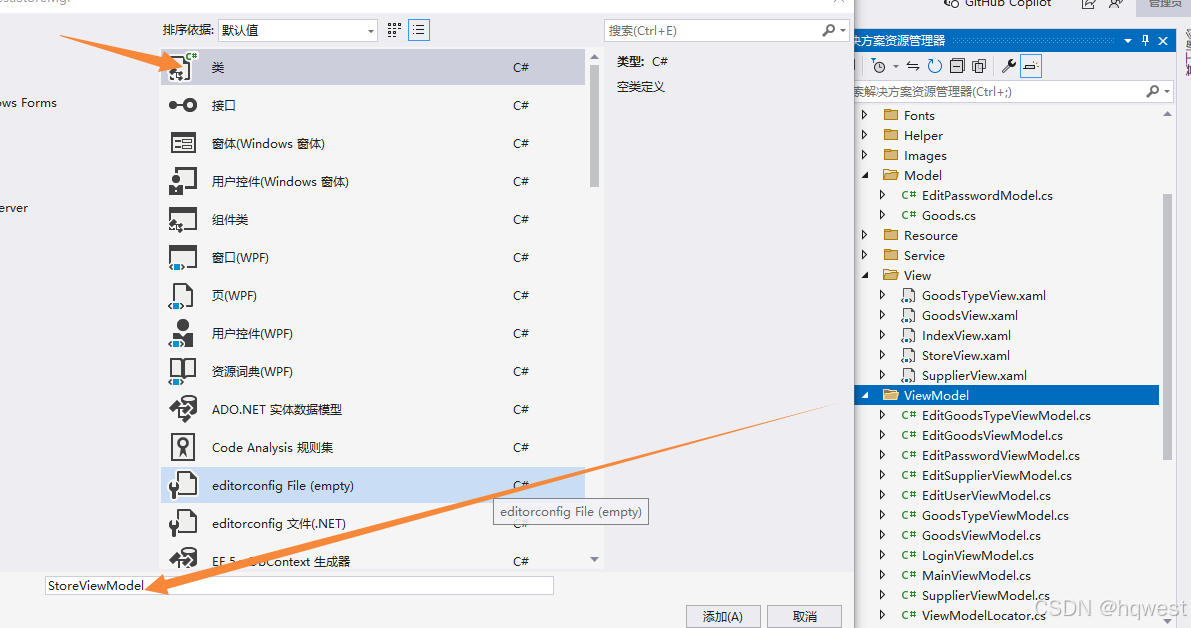
cs
using GalaSoft.MvvmLight;
using GalaSoft.MvvmLight.Command;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Controls;
using West.StoreMgr.Helper;
using West.StoreMgr.Service;
using CommonServiceLocator;
using West.StoreMgr.Windows;
using static West.StoreMgr.Windows.MsgBoxWindow;
namespace West.StoreMgr.ViewModel
{
/// <summary>
/// 仓库设置viewmodel
/// </summary>
public class StoreViewModel : ViewModelBase
{
public StoreViewModel()
{
StoreList = new StoreService().Select();
}
private Store store = new Store();
public Store Store
{
get { return store; }
set { store = value; RaisePropertyChanged(); }
}
private List<Store> storeList = new List<Store>();
public List<Store> StoreList
{
get { return storeList; }
set { storeList = value; RaisePropertyChanged(); }
}
//增加
public RelayCommand<UserControl> AddCommand
{
get
{
var command = new RelayCommand<UserControl>((view) =>
{
if (string.IsNullOrEmpty(Store.Name) == true)
{
MsgWinHelper.ShowError("不能为空!");
return;
}
Store.InsertDate = DateTime.Now;
var service = new StoreService();
int count = service.Insert(Store);
if (count > 0)
{
StoreList = service.Select();
MsgWinHelper.ShowMessage("添加成功!");
Store = new Store();
}
else
{
MsgWinHelper.ShowError("添加失败!");
}
});
return command;
}
}
//修改
public RelayCommand<Button> EditCommand
{
get
{
var command = new RelayCommand<Button>((view) =>
{
var old = view.Tag as Store;
if (old == null) return;
var model = ServiceLocator.Current.GetInstance<EditStoreViewModel>();
model.Store = old;
var window = new EditStoreWindow();
window.ShowDialog();
StoreList = new StoreService().Select();
});
return command;
}
}
//删除
public RelayCommand<Button> DeleteCommand
{
get
{
var command = new RelayCommand<Button>((view) =>
{
if (MsgWinHelper.ShowQuestion("您确定要删除该仓库吗?") == CustomMessageBoxResult.OK)
{
var old = view.Tag as Store;
if (old == null) return;
var service = new StoreService();
int count = service.Delete(old);
if (count > 0)
{
StoreList = service.Select();
MsgWinHelper.ShowMessage("操作成功!");
}
else
{
MsgWinHelper.ShowError("操作失败!");
}
}
});
return command;
}
}
}
}
3、修改仓库窗体
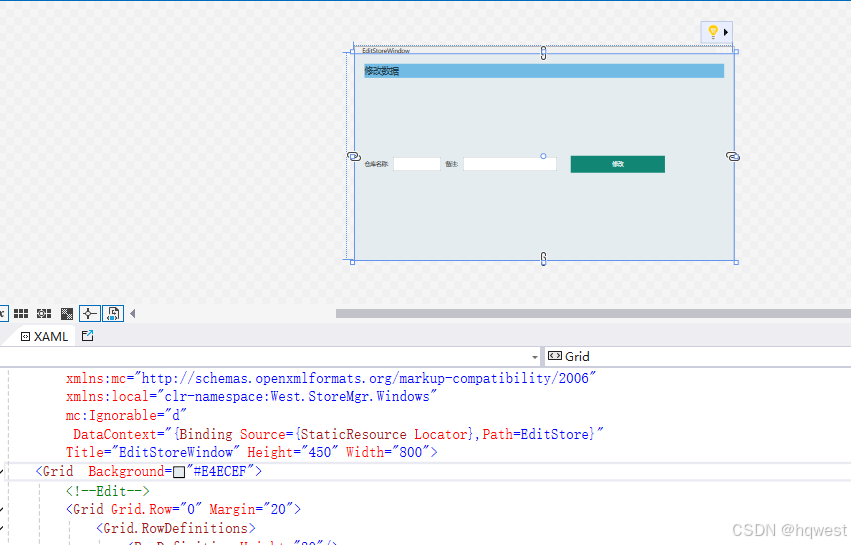
cs
<Window x:Class="West.StoreMgr.Windows.EditStoreWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:West.StoreMgr.Windows"
mc:Ignorable="d"
DataContext="{Binding Source={StaticResource Locator},Path=EditStore}"
Title="EditStoreWindow" Height="450" Width="800">
<Grid Background="#E4ECEF">
<!--Edit-->
<Grid Grid.Row="0" Margin="20">
<Grid.RowDefinitions>
<RowDefinition Height="30"/>
<RowDefinition/>
</Grid.RowDefinitions>
<Border Background="#72BBE5">
<TextBlock Text="修改数据" FontSize="18" VerticalAlignment="Center" Foreground="#1F3C4C" Margin="0 0 10 0"/>
</Border>
<StackPanel Grid.Row="1" Orientation="Horizontal" VerticalAlignment="Center">
<TextBlock Margin="0 0 10 0" Text="仓库名称:" VerticalAlignment="Center"/>
<TextBox Margin="0 0 10 0" Text="{Binding Store.Name,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}" Width="100" Height="30" />
<TextBlock Margin="0 0 10 0" Text="备注:" VerticalAlignment="Center"/>
<TextBox Margin="0 0 10 0" Text="{Binding Store.Tag,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}" Width="200" Height="30" />
<!--button-->
<Button Margin="18 0 0 0" Height="36" Width="199" Grid.Row="3"
Content="修改" Style="{StaticResource ButtonStyle}"
CommandParameter="{Binding RelativeSource={RelativeSource Mode=FindAncestor,AncestorType=local:EditStoreWindow}}"
Command="{Binding EditCommand}"/>
</StackPanel>
</Grid>
</Grid>
</Window>
4、运行效果
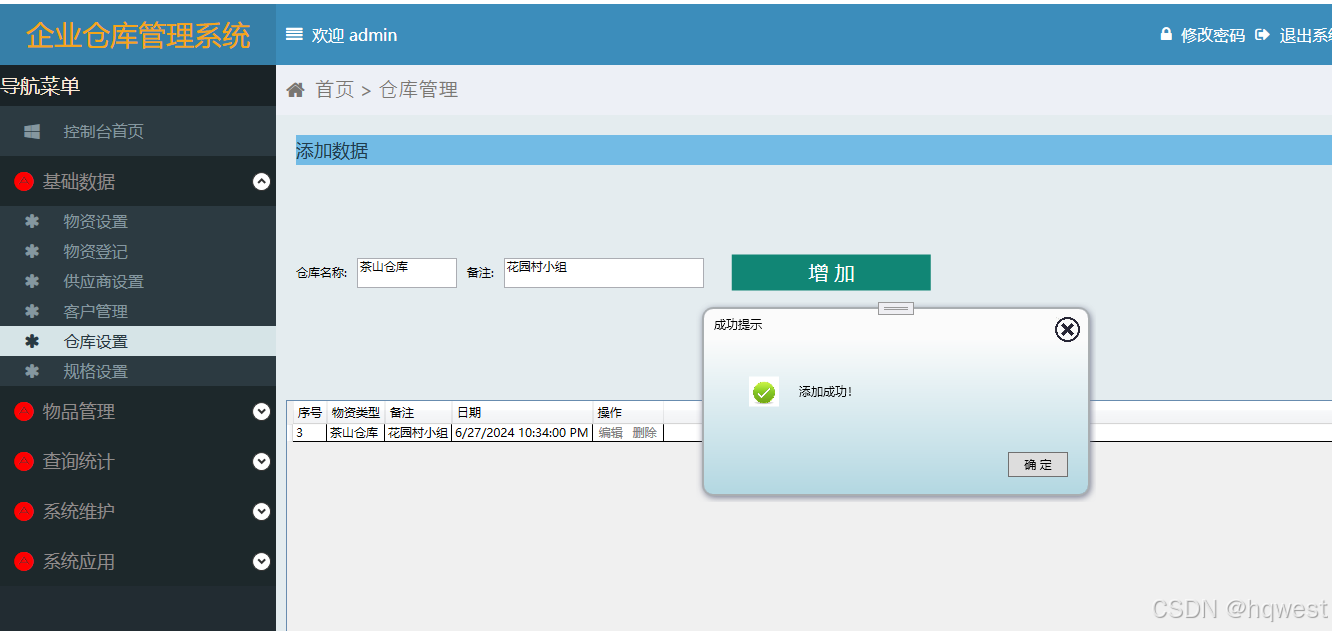
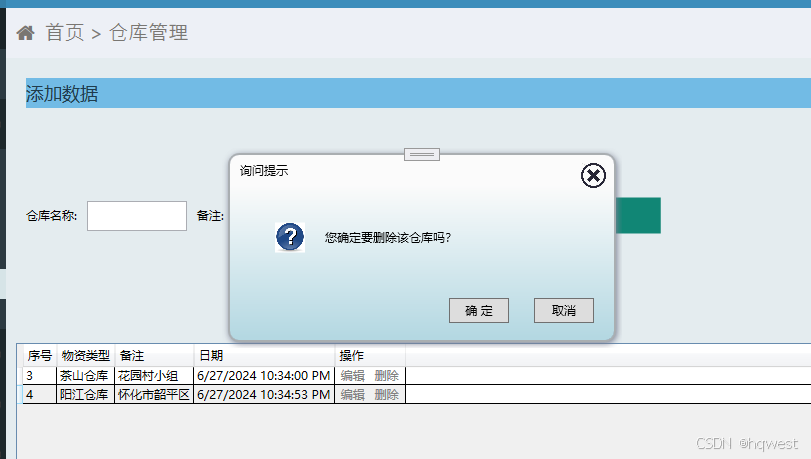
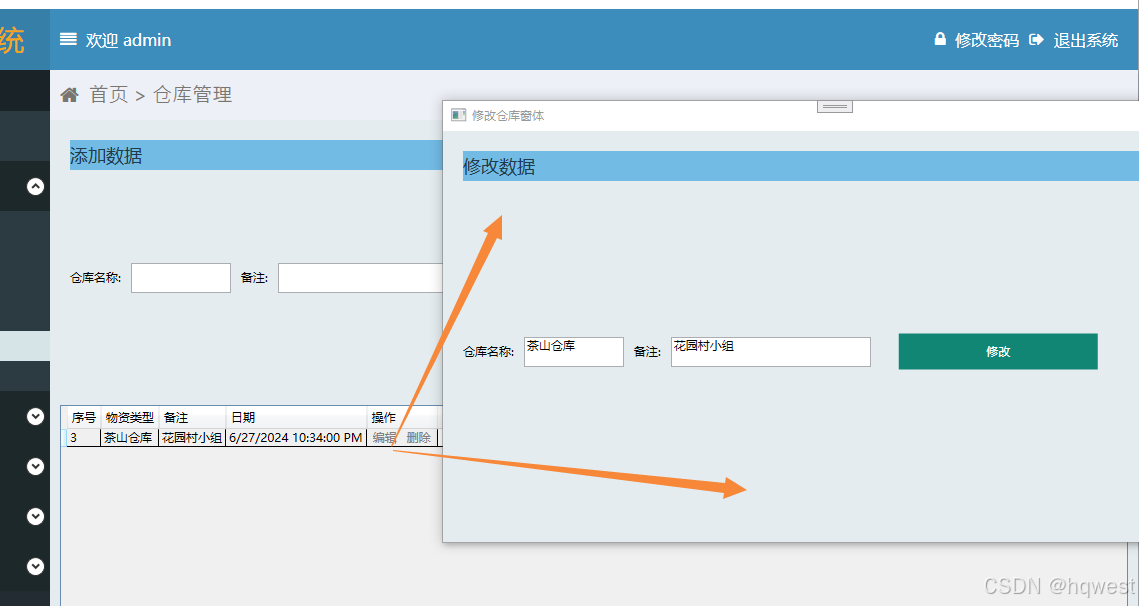
原创不易,打字不易,截图不易,多多点赞,送人玫瑰,留有余香,财务自由明日实现。