介绍:
基于Pygame的糖果从屏幕顶部下落的游戏代码。这个游戏包括了一个可以左右移动的篮子来接住下落的糖果,接住糖果会增加得分。
代码:
python
import pygame
import random
import os
# 初始化pygame和设置屏幕大小
pygame.init()
screen_width, screen_height = 800, 600
screen = pygame.display.set_mode((screen_width, screen_height))
# 设置颜色
WHITE = (255, 255, 255)
RED = (255, 0, 0)
ORANGE = (255, 128, 0)
BLACK = (0, 0, 0)
# 糖果和篮子的尺寸
candy_width, candy_height = 30, 30
basket_width, basket_height = 100, 50
# 初始化篮子的位置
basket_x = screen_width // 2
basket_y = screen_height - basket_height
# 糖果列表
candies = []
# 初始化得分
score = 0
# 最高得分
highest_score = 0
# 设置游戏运行时间(毫秒)
game_duration = 30000
# 难度和对应的下落速度
DIFFICULTIES = {
'Easy': 10,
'Medium': 20,
'Hard': 30
}
# 按钮的位置和大小
button_width = 150
button_height = 50
button_x_offset = 100
button_y = screen_height // 2 - (button_height // 2)
# 字体
font = pygame.font.Font(None, 36)
# 难度选择函数
def select_difficulty():
selected_difficulty = None
clock = pygame.time.Clock() # 添加一个时钟对象来控制帧率
while not selected_difficulty:
for event in pygame.event.get():
if event.type == pygame.QUIT:
return None
elif event.type == pygame.MOUSEBUTTONDOWN:
mouse_x, mouse_y = pygame.mouse.get_pos()
if button_x_offset <= mouse_x < button_x_offset + button_width and button_y <= mouse_y < button_y + button_height:
selected_difficulty = 'Easy'
elif button_x_offset + button_width + 10 <= mouse_x < button_x_offset + 2 * button_width + 10 and button_y <= mouse_y < button_y + button_height:
selected_difficulty = 'Medium'
elif button_x_offset + 2 * button_width + 20 <= mouse_x < button_x_offset + 3 * button_width + 20 and button_y <= mouse_y < button_y + button_height:
selected_difficulty = 'Hard'
# 在循环内渲染难度选择界面
screen.fill(WHITE)
# 绘制按钮
pygame.draw.rect(screen, RED, (button_x_offset, button_y, button_width, button_height))
pygame.draw.rect(screen, RED, (button_x_offset + button_width + 10, button_y, button_width, button_height))
pygame.draw.rect(screen, RED, (button_x_offset + 2 * button_width + 20, button_y, button_width, button_height))
# 绘制按钮文本
easy_text = font.render("Easy", True, WHITE)
screen.blit(easy_text, (button_x_offset + (button_width - easy_text.get_width()) // 2,
button_y + (button_height - easy_text.get_height()) // 2))
medium_text = font.render("Medium", True, WHITE)
screen.blit(medium_text, (button_x_offset + button_width + 10 + (button_width - medium_text.get_width()) // 2,
button_y + (button_height - medium_text.get_height()) // 2))
hard_text = font.render("Hard", True, WHITE)
screen.blit(hard_text, (button_x_offset + 2 * button_width + 20 + (button_width - hard_text.get_width()) // 2,
button_y + (button_height - hard_text.get_height()) // 2))
pygame.display.flip() # 更新屏幕显示
# 控制帧率
clock.tick(60)
return selected_difficulty
# 加载或设置历史最高分数
def load_highest_score():
global highest_score
file_path = os.path.join(os.getcwd(), 'highest_score.txt')
try:
with open(file_path, 'r') as file:
highest_score = int(file.read().strip())
except FileNotFoundError:
highest_score = 0
def save_highest_score():
global highest_score
file_path = os.path.join(os.getcwd(), 'highest_score.txt')
with open(file_path, 'w') as file:
file.write(str(highest_score))
def check_restart_button(event):
button_rect = pygame.Rect(screen_width - 100, screen_height - 50, 100, 50)
return event.type == pygame.MOUSEBUTTONDOWN and event.button == 1 and button_rect.collidepoint(event.pos)
# 选择难度
difficulty = select_difficulty()
if difficulty is None:
pygame.quit()
# 根据难度设置下落速度
speed = DIFFICULTIES[difficulty]
# 游戏主循环
clock = pygame.time.Clock()
running = True
game_over = False
load_highest_score() # 加载历史最高分数
game_start_time = pygame.time.get_ticks() # 记录游戏开始时间
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEMOTION:
basket_x = event.pos[0]
basket_x = max(0, min(basket_x, screen_width - basket_width))
elif check_restart_button(event):
game_start_time = pygame.time.get_ticks()
candies.clear()
score = 0
# 计算已经过去的时间(毫秒)
elapsed_time = pygame.time.get_ticks() - game_start_time
# 将毫秒转换为秒
elapsed_seconds = elapsed_time / 1000
# 生成新的糖果(限制生成频率)
if random.random() < 0.02 and len(candies) < 10:
candy_x = random.randint(0, screen_width - candy_width)
candy_y = 0 - candy_height # 开始时稍微在屏幕外
candies.append([candy_x, candy_y])
# 移动糖果
for candy in candies:
candy[1] += speed
# 检查糖果是否超出屏幕底部
if candy[1] > screen_height:
candies.remove(candy)
# 检查是否接到糖果(在糖果掉出屏幕之前)
if (basket_x < candy[0] < basket_x + basket_width and
basket_y - candy_height < candy[1] < basket_y):
score += 1
candies.remove(candy)
# 在游戏结束时保存最高分数
if not candies and score > highest_score:
highest_score = score
save_highest_score()
# 绘制游戏元素
screen.fill(WHITE) # 填充背景色
for candy_x, candy_y in candies:
pygame.draw.rect(screen, (255, 0, 0), (candy_x, candy_y, candy_width, candy_height))
pygame.draw.rect(screen, (100, 50, 0), (basket_x, basket_y, basket_width, basket_height)) # 绘制篮子矩形
# 显示得分
score_text = font.render(f"Score: {score}", True, BLACK)
highest_score_text = font.render(f"Highest score: {highest_score}", True, BLACK)
screen.blit(score_text, (10, 10))
screen.blit(highest_score_text, (10, 50))
time = game_duration - elapsed_time
# 获取秒数的后三位
last_three_digits = time % 1000
# 转换为字符串以便显示
time1 = int(time/1000)
time_str = str(last_three_digits).zfill(3)
if time > 0:
screen.blit(font.render(f"remainder: {time1}.{time_str}", True, RED), (300, 10))
# 绘制"重新开始"按钮
pygame.draw.rect(screen, BLACK, (screen_width - 100, screen_height - 50, 100, 50))
pygame.draw.rect(screen, WHITE, (screen_width - 98, screen_height - 48, 96, 46), 2)
screen.blit(font.render("restart", True, ORANGE), (screen_width - 85, screen_width - 300))
# 检查游戏是否应该结束
if time < 0:
font1 = pygame.font.Font(None, 52)
screen.blit(font1.render("game over", True, RED), (300, 300))
candies.clear()
score = 0
# 更新屏幕显示
pygame.display.flip()
# 控制游戏循环的速度
clock.tick(60)
# 退出pygame
pygame.quit()
选择难度页面示例:
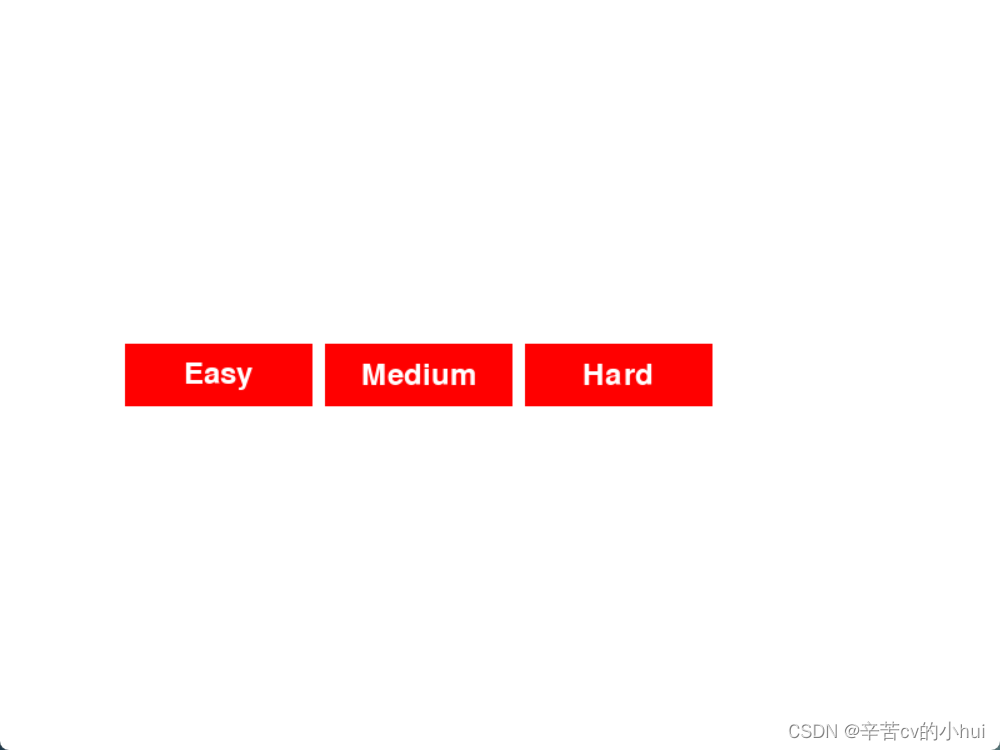
游戏页面:
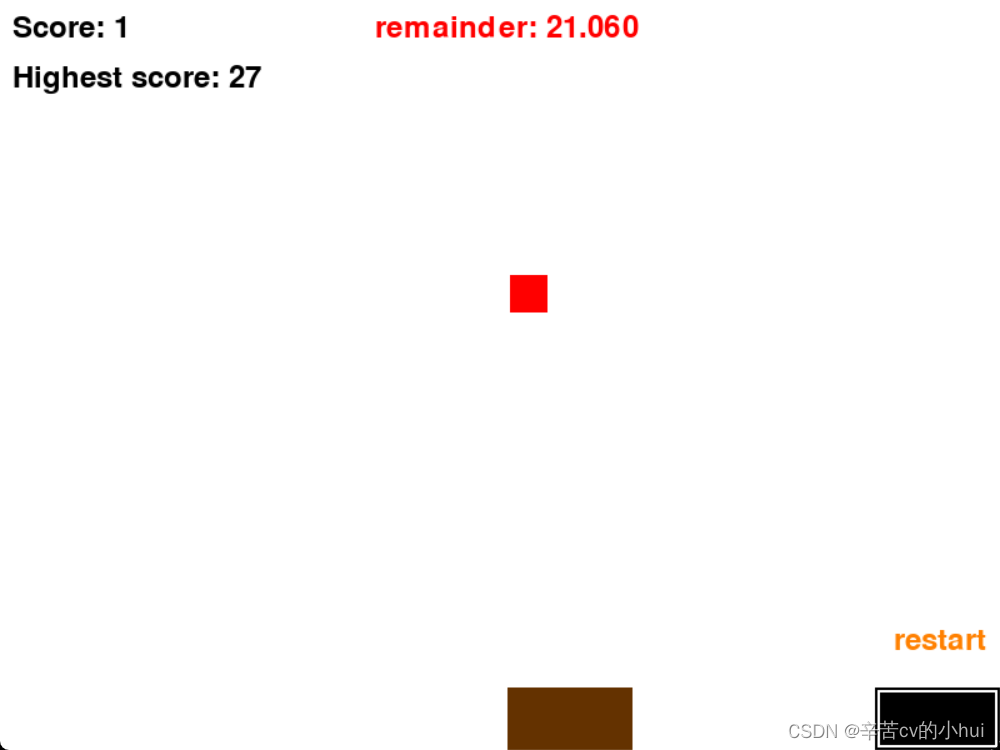