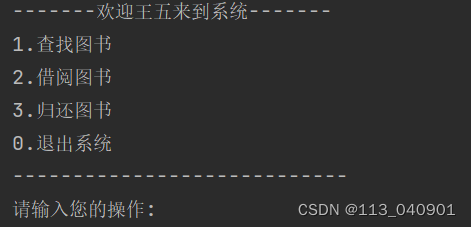
一、框架
1. 创建类
用户:管理员AdminUser 普通用户NormalUser 继承抽象类User
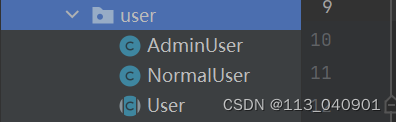
书:书Book 书架BookList
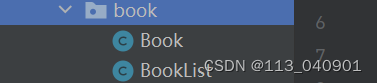
操作对象:书Book
2. 知识点
主要涉及的知识点:数据类型 变量 if for 数组 方法 类和对象 封装继承多态 抽象类和接口
3.利用数组放置图书
Test.Java

BookList.java
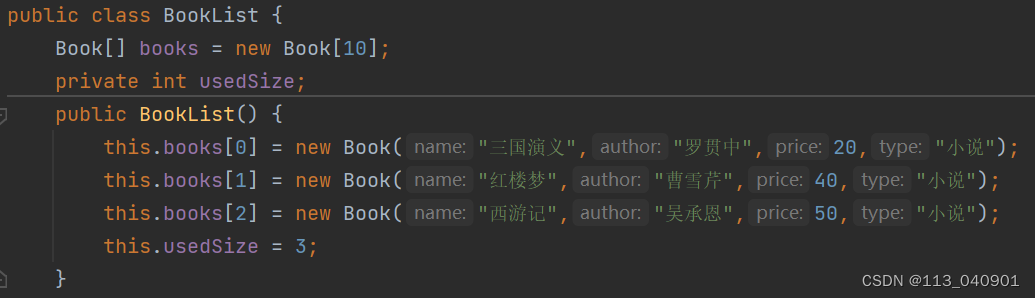
方法调用
BookList.java
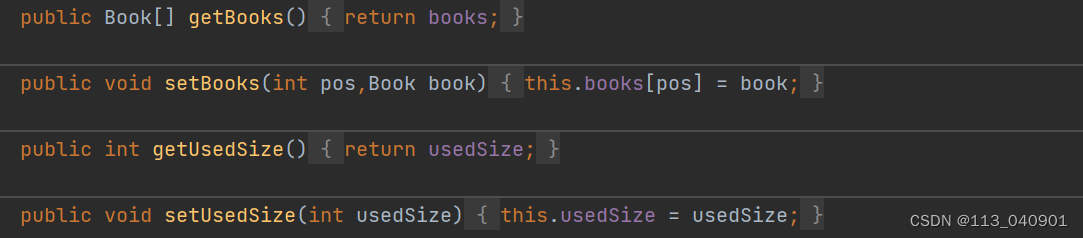
Book.java
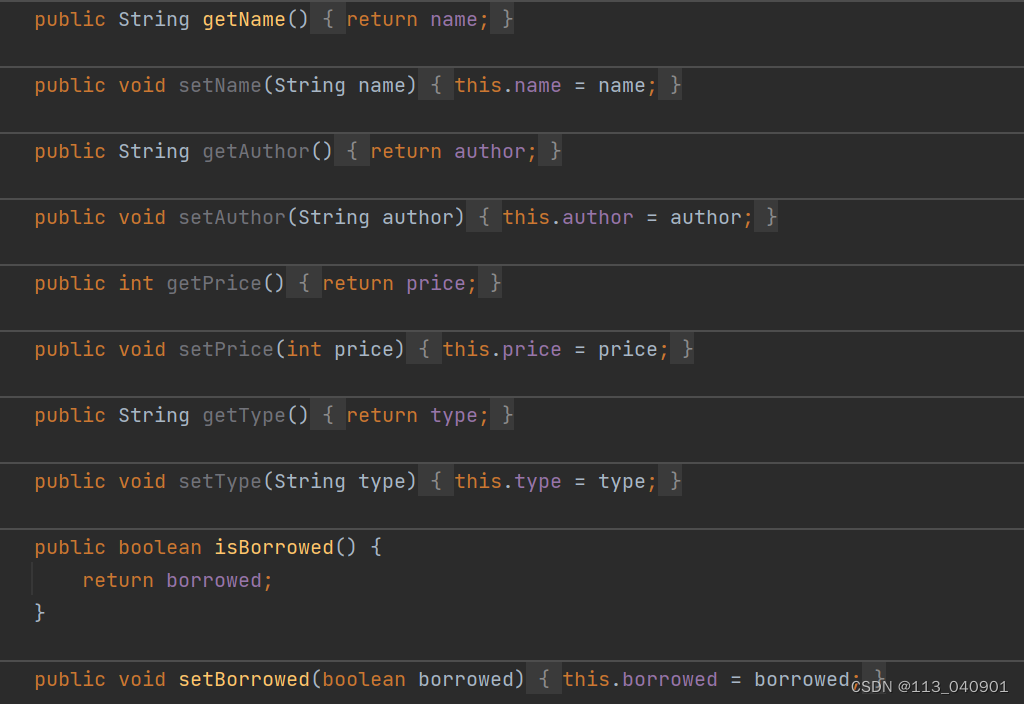
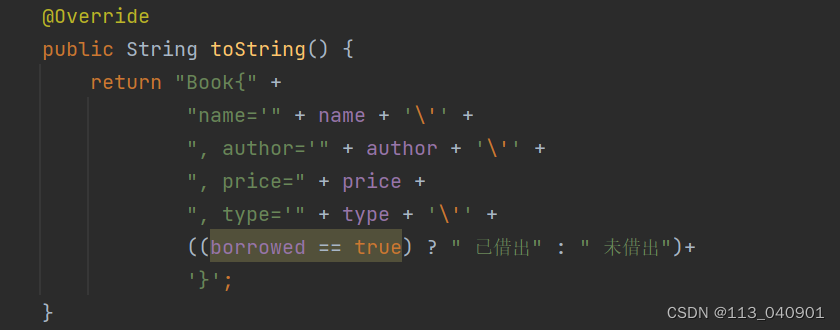
4.利用向上转型实现用户身份的选择
User.java
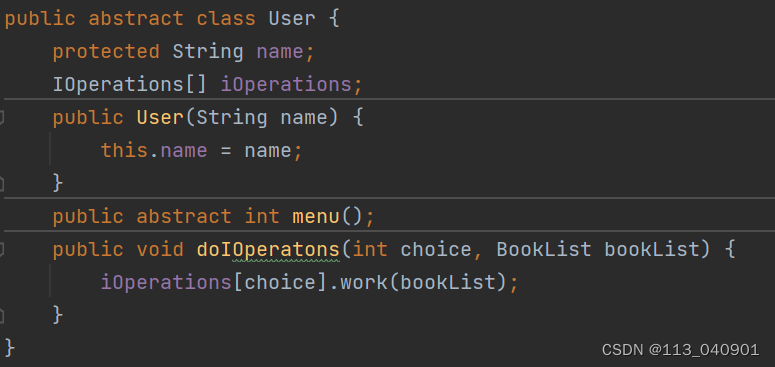
添加图书操作AddIOperation等类实现接口IOperations
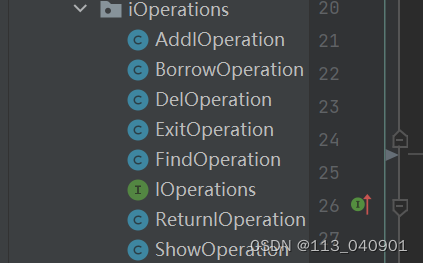
IOperations.java

每个功能操作均需重写接口IOperations的work方法--此处以添加操作为例

NormalUser.java
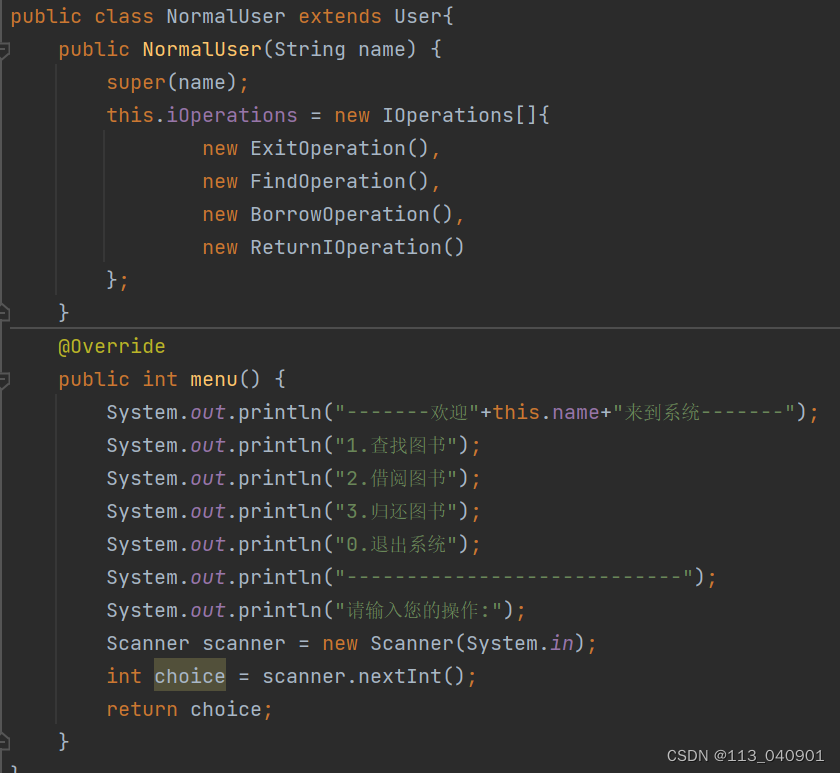
AdminUser.java
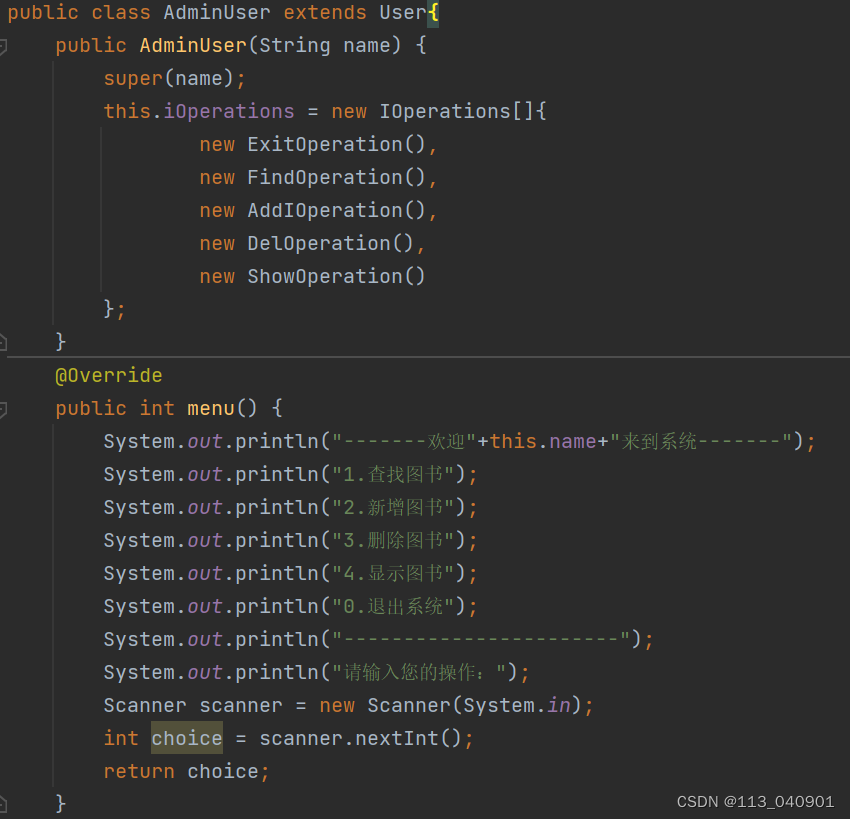
Test.java
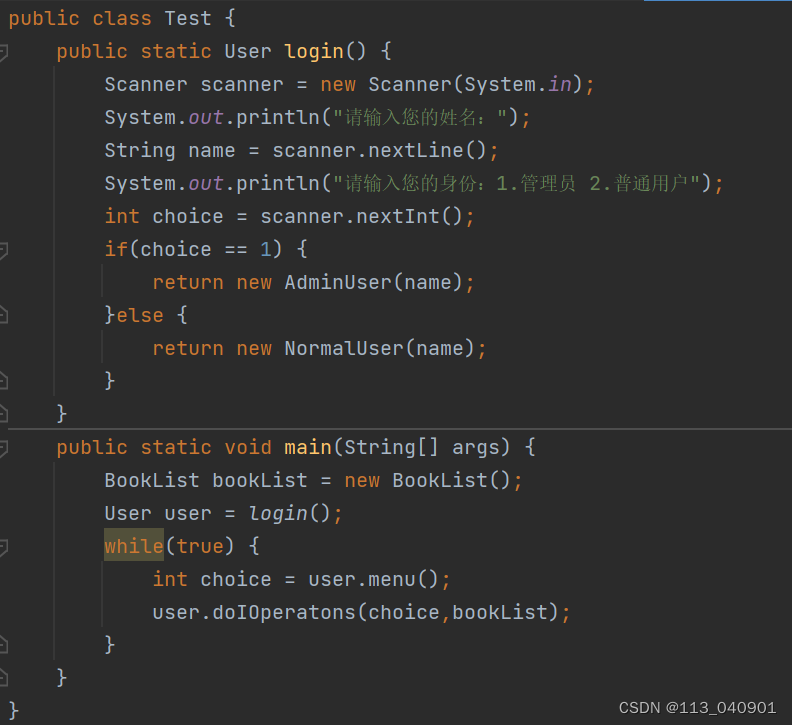
二、功能的实现------重写Work()方法
1.退出操作ExitOperation
java
public class ExitOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("退出系统");
System.exit(0);
}
}
2.展示操作ShowOperation
java
public class ShowOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("展示书籍");
for (int i = 0; i < bookList.getUsedSize(); i++) {
System.out.println(bookList.getBooks()[i].toString());
}
}
}
3.查找操作FindOperation
java
public class FindOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("查找书籍");
System.out.println("请输入您要查找的图书名字:");
Scanner scanner = new Scanner(System.in);
String name = scanner.nextLine();
for (int i = 0; i < bookList.getUsedSize(); i++) {
if(bookList.getBooks()[i].getName().equals(name)) {
System.out.println(bookList.getBooks()[i].toString());
return;
}
}
System.out.println("没有这本书");
}
}
4.借阅操作BorrowOperation
java
public class BorrowOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("借阅书籍");
System.out.println("请输入你要借阅的图书名字:");
Scanner scanner = new Scanner(System.in);
String name = scanner.nextLine();
for (int i = 0; i < bookList.getUsedSize(); i++) {
if (bookList.getBooks()[i].getName().equals(name)) {
bookList.getBooks()[i].setBorrowed(true);
System.out.println("借阅成功");
return;
}
}
System.out.println("没有找到这本书");
}
}
5.归还操作ReturnOPeration
java
public class ReturnIOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("归还书籍");
System.out.println("请输入你要归还的图书名字:");
Scanner scanner = new Scanner(System.in);
String name = scanner.nextLine();
for (int i = 0; i < bookList.getUsedSize(); i++) {
if (bookList.getBooks()[i].getName().equals(name)) {
if(bookList.getBooks()[i].isBorrowed()) {
bookList.getBooks()[i].setBorrowed(false);
System.out.println("归还成功");
return;
}else {
System.out.println("这本书已经归还了,无需再次归还");
return;
}
}
}
System.out.println("没有找到这本书");
}
}
6.添加功能AddOperation
java
public class AddIOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("增添书籍");
//判满
int currentSize = bookList.getUsedSize();
if (currentSize == bookList.getBooks().length) {
System.out.println("书架已满,添加失败");
return;
}
System.out.println("请输入图书名字:");
Scanner scanner = new Scanner(System.in);
String name = scanner.nextLine();
System.out.println("请输入图书的作者:");
String author = scanner.nextLine();
System.out.println("请输入图书的价格:");
int price = scanner.nextInt();
scanner.nextLine();
System.out.println("请输入图书的类型:");
String type = scanner.nextLine();
Book newBook = new Book(name,author,price,type);
//判断书架有没有这本书
for (int i = 0; i < bookList.getBooks().length; i++) {
if(newBook.equals(bookList.getBooks()[i])) {
System.out.println("该书已存在,添加无效");
return;
}
}
bookList.setBooks(currentSize,newBook);
bookList.setUsedSize(currentSize+1);
System.out.println("添加成功");
}
}
7.删除操作DelOperation
java
public class DelOperation implements IOperations{
@Override
public void work(BookList bookList) {
System.out.println("删除书籍");
System.out.println("请输入您要删除的图书名字");
Scanner scanner = new Scanner(System.in);
int pos = -1;
String name = scanner.nextLine();
int currentSize = bookList.getUsedSize();
int i = 0;
for (; i < currentSize; i++) {
if(name.equals(bookList.getBooks()[i].getName())) {
pos = i;
break;
}
}
if (i == currentSize) {
System.out.println("没有你想删除的图书");
return;
}
int j = pos;
for (;j < bookList.getUsedSize()-1; j++) {
Book book = bookList.getBooks()[j+1];
bookList.setBooks(j,book);
}
bookList.setBooks(j,null);
bookList.setUsedSize(currentSize-1);
System.out.println("删除成功");
}
}