正则表达式-字符类
- 语法示例:
[abc]:代表a或者b,或者c字符中的一个。
[^abc]:代表除a,b,c以外的任何字符。
[a-z]:代表a-z的所有小写字符中的一个。
[A-Z]:代表A-Z的所有大写字符中的一个。
[0-9]:代表0-9之间的某一个数字字符。
[a-zA-Z0-9]:代表a-z或者A-Z或者0-9之间的任意一个字符。
[a-dm-p]:a 到 d 或 m 到 p之间的任意一个字符。
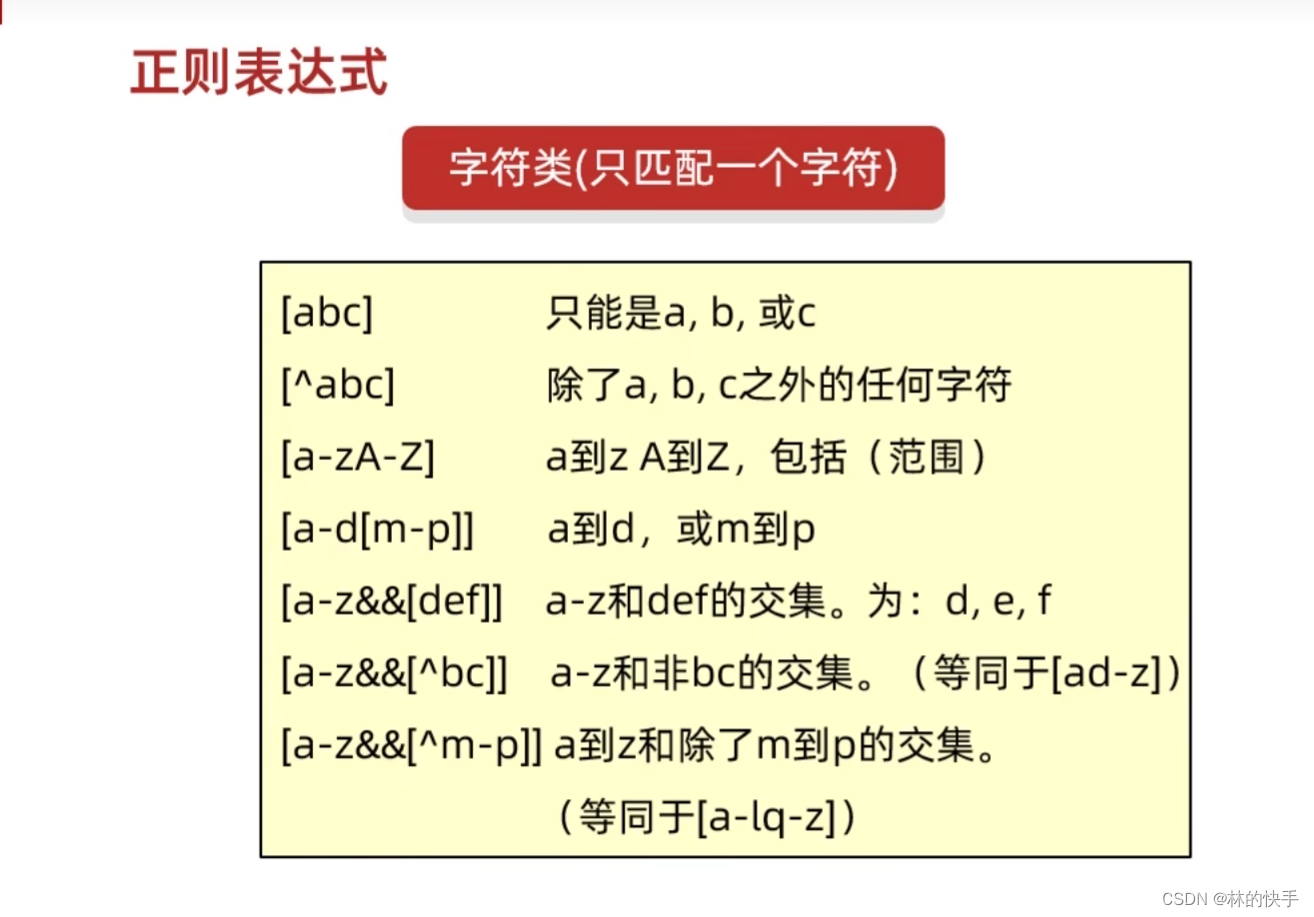
cpp
public static void main(String[] args) {
//public boolean matches(String regex):判断是否与正则表达式匹配,匹配返回true
// 只能是a b c
System.out.println("-----------1-------------");
System.out.println("a".matches("[abc]")); // true
System.out.println("z".matches("[abc]")); // false
// 不能出现a b c
System.out.println("-----------2-------------");
System.out.println("a".matches("[^abc]")); // false
System.out.println("z".matches("[^abc]")); // true
System.out.println("zz".matches("[^abc]")); //false
System.out.println("zz".matches("[^abc][^abc]")); //true
//先判断第一个z是不是不在abc范围之内,然后再判断第二个z是不是不在abc范围之内
// a到zA到Z(包括头尾的范围)
System.out.println("-----------3-------------");
System.out.println("a".matches("[a-zA-z]")); // true
System.out.println("z".matches("[a-zA-z]")); // true
System.out.println("aa".matches("[a-zA-z]"));//false
System.out.println("zz".matches("[a-zA-Z]")); //false
System.out.println("zz".matches("[a-zA-Z][a-zA-Z]")); //true
System.out.println("0".matches("[a-zA-Z]"));//false
System.out.println("0".matches("[a-zA-Z0-9]"));//true
// [a-d[m-p]] a到d,或m到p
System.out.println("-----------4-------------");
System.out.println("a".matches("[a-d[m-p]]"));//true
System.out.println("d".matches("[a-d[m-p]]")); //true
System.out.println("m".matches("[a-d[m-p]]")); //true
System.out.println("p".matches("[a-d[m-p]]")); //true
System.out.println("e".matches("[a-d[m-p]]")); //false
System.out.println("0".matches("[a-d[m-p]]")); //false
// [a-z&&[def]] a-z和def的交集。为:d,e,f
//细节:如果要求二个范围的交集,那么需要写符号&&
//如果写成一个&,那么此时的&就不再是交集了,而是一个简简单单的&符号
System.out.println("----------5------------");
System.out.println("a".matches("[a-z&[def]]")); //true
System.out.println("d".matches("[a-z&&[def]]")); //true
System.out.println("0".matches("[a-z&&[def]]")); //false
// [a-z&&[^bc]] a-z和非bc的交集。(等同于[ad-z])
System.out.println("-----------6------------_");
System.out.println("a".matches("[a-z&&[^bc]]"));//true
System.out.println("b".matches("[a-z&&[^bc]]")); //false
System.out.println("0".matches("[a-z&&[^bc]]")); //false
// [a-z&&[^m-p]] a到z和除了m到p的交集。(等同于[a-1q-z])
System.out.println("-----------7-------------");
System.out.println("a".matches("[a-z&&[^m-p]]")); //true
System.out.println("m".matches("[a-z&&[^m-p]]")); //false
System.out.println("0".matches("[a-z&&[^m-p]]")); //false
}
结果
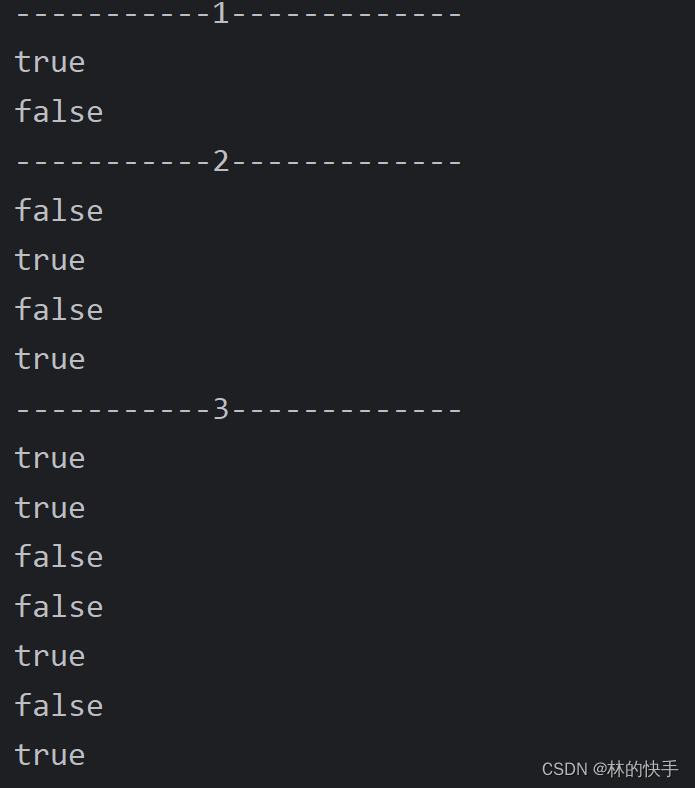
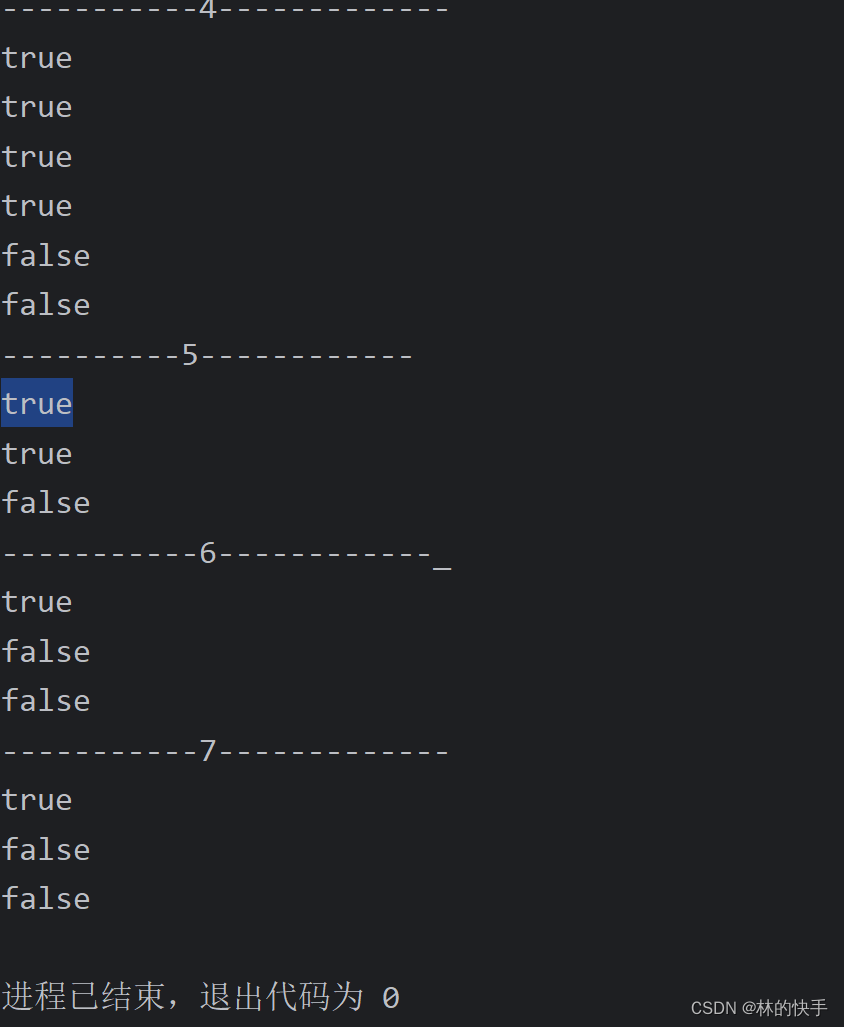
正则表达式-预定义字符
语法示例:
"." : 匹配任何字符。
"\d":任何数字[0-9]的简写;
"\D":任何非数字[^0-9]的简写;
"\s": 空白字符:[ \t\n\x0B\f\r] 的简写
"\S": 非空白字符:[^\s] 的简写
"\w":单词字符:[a-zA-Z_0-9]的简写
"\W":非单词字符:[^\w]
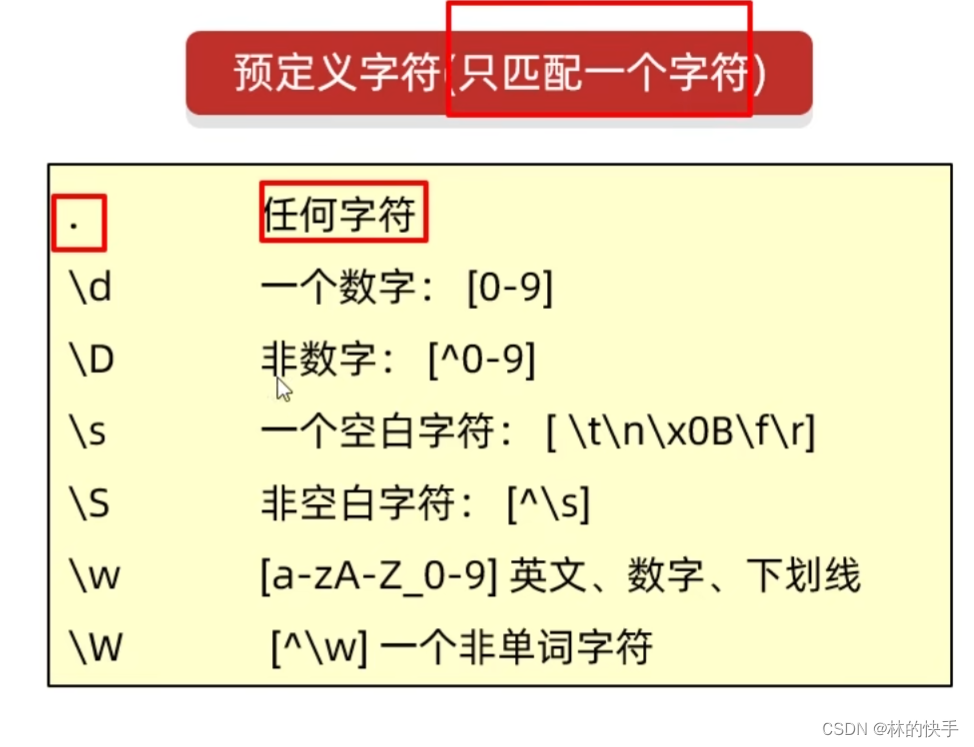
cpp
package zzbds;
public class zzbdsdemo2 {
public static void main(String[] args) {
//.表示任意一个字符
System.out.println("你".matches("..")); //false
System.out.println("你".matches(".")); //true
System.out.println("你a".matches(".."));//true
// \\d 表示任意的一个数字
// \\d只能是任意的一位数字
// 简单来记:两个\表示一个\
System.out.println("a".matches("\\d")); // false
System.out.println("3".matches("\\d")); // true
System.out.println("333".matches("\\d")); // false
//\\w只能是一位单词字符[a-zA-Z_0-9]
System.out.println("z".matches("\\w")); // true
System.out.println("2".matches("\\w")); // true
System.out.println("21".matches("\\w")); // false
System.out.println("你".matches("\\w"));//false
// 非单词字符 [^\w]
System.out.println("你".matches("\\W")); // true
System.out.println("---------------------------------------------");
// 以上正则匹配只能校验单个字符。
/*
1. X? : 0次或1次
2. X* : 0次到多次
3. X+ : 1次或多次
4. X{n} : 恰好n次
5. X{n,} : 至少n次
6. X{n,m}: n到m次(n和m都是包含的)
*/
// 必须是数字 字母 下划线 至少 6位
System.out.println("2442fsfsf".matches("\\w{6,}"));//true
System.out.println("244f".matches("\\w{6,}"));//false
// 必须是数字和字符 必须是4位
System.out.println("23dF".matches("[a-zA-Z0-9]{4}"));//true
System.out.println("23 F".matches("[a-zA-Z0-9]{4}"));//false
System.out.println("23dF".matches("[\\w&&[^_]]{4}"));//true
System.out.println("23_F".matches("[\\w&&[^_]]{4}"));//false
}
}
正则表达式-数量词
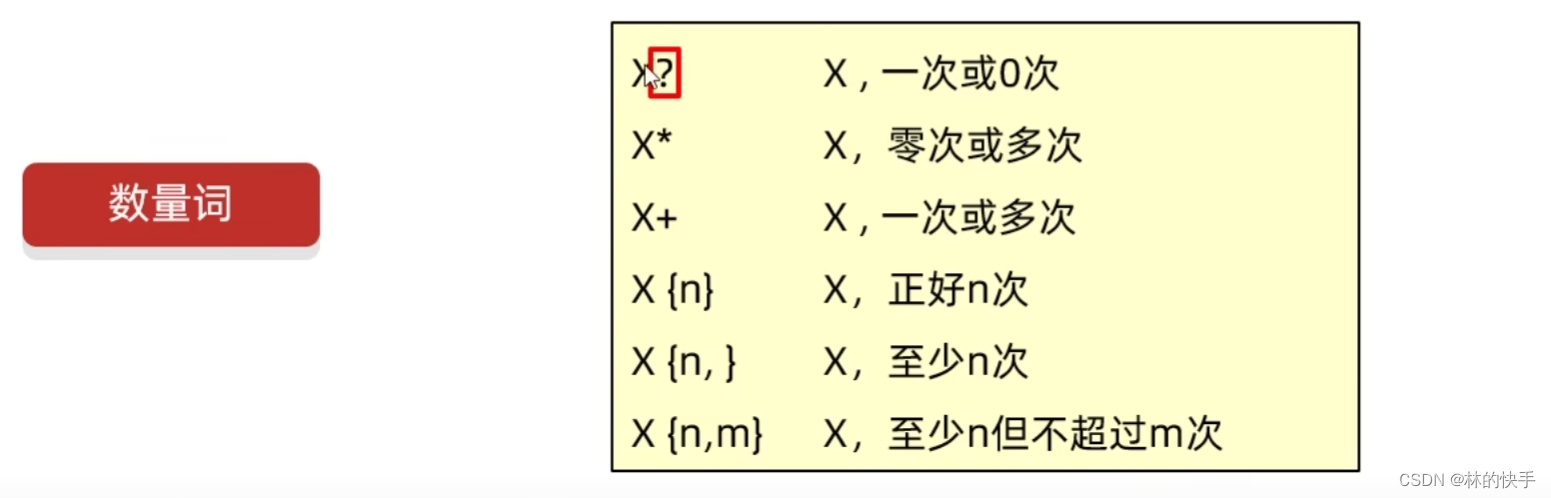
cpp
package zzbds;
public class zzbdsdemo3 {
public static void main(String[] args) {
// 必须是数字 字母 下划线 至少 6位
System.out.println("2442fsfsf".matches("\\w{6,}"));//true
System.out.println("244f".matches("\\w{6,}"));//false
// 必须是数字和字符 必须是4位
System.out.println("23dF".matches("[a-zA-Z0-9]{4}"));//true
System.out.println("23 F".matches("[a-zA-Z0-9]{4}"));//false
System.out.println("23dF".matches("[\\w&&[^_]]{4}"));//true
System.out.println("23_F".matches("[\\w&&[^_]]{4}"));//false
}
}
正则表达式-逻辑运算符
语法示例:
&&:并且
| :或者
\ :转义字符
cpp
package zzbds;
public class zzbdsdemo5 {
public static void main(String[] args) {
// \ 转义字符 改变后面那个字符原本的含义
//练习:以字符串的形式打印一个双引号
//"在Java中表示字符串的开头或者结尾
//此时\表示转义字符,改变了后面那个双引号原本的含义
//把他变成了一个普普通通的双引号而已。
System.out.println("\"");
// \表示转义字符
//两个\的理解方式:前面的\是一个转义字符,改变了后面\原本的含义,把他变成一个普普通通的\而已。
System.out.println("c:Users\\moon\\IdeaProjects\\basic-code\\myapi\\src\\com\\itheima\\a08regexdemo\\RegexDemo1.java");
}
}
cpp
public class zzbdsdemo4 {
public static void main(String[] args) {
String str = "had";
//1.要求字符串是小写辅音字符开头,后跟ad
String regex = "[a-z&&[^aeiou]]ad";
System.out.println("1." + str.matches(regex));
//2.要求字符串是aeiou中的某个字符开头,后跟ad
regex = "[a|e|i|o|u]ad";//这种写法相当于:regex = "[aeiou]ad";
System.out.println("2." + str.matches(regex));
}
}
练习1
需求:
请编写正则表达式验证用户输入的手机号码是否满足要求。
请编写正则表达式验证用户输入的邮箱号是否满足要求。
请编写正则表达式验证用户输入的电话号码是否满足要求。
验证手机号码 13112345678 13712345667 13945679027 139456790271
验证座机电话号码 020-2324242 02122442 027-42424 0712-3242434
验证邮箱号码 3232323@qq.com zhangsan@itcast.cnn dlei0009@163.com dlei0009@pci.com.cn
cs
package zzbds;
/*
需求:
请编写正则表达式验证用户输入的手机号码是否满足要求。
请编写正则表达式验证用户输入的邮箱号是否满足要求。
请编写正则表达式验证用户输入的电话号码是否满足要求。
验证手机号码 13112345678 13712345667 13945679027 139456790271
验证座机电话号码 020-2324242 02122442 027-42424 0712-3242434
验证邮箱号码 3232323@qq.com zhangsan@itcast.cnn dlei0009@163.com dlei0009@pci.com.cn
*/
public class zzbdsdemo6 {
public static void main(String[] args) {
//拿着一个正确的数据,从左到右以此去写
//13112345678
//分3个部分
//第一部分: 1表示手机号码只能以1开头
//第二部分:[3-9]表示手机号码的第二位只能是3-9
//第三部分:\\d{9}表示任意数字可以出现9次,也只能出现9次
String regex1 = "1[3-9]\\d{9}";
System.out.println("13112345678".matches(regex1));//true
System.out.println("13712345667".matches(regex1));//true
System.out.println("13945679027".matches(regex1));//true
System.out.println("139456790271".matches(regex1));//false
System.out.println("-----------------------------");
//座机号码
//分3个部分
//020-2324242 02122442 027-42424 0712-3242434 我们自己规定范围5-10
//第一部分:区号 0开头 写一个0即可,-前面的至少2位或者3位任意数字\\d{2,3}
//第二部分:表示- ,写一个-即可, -?表示0次或一次
//第三部分:-后面的第一位不能是0开头,第2位开始可以是任意数字,-后面号码总长度:5-10位
String regex2 = "0\\d{2,3}-?[1-9]\\d{4,9}";
System.out.println("020-2324242".matches(regex2));
System.out.println("02122442".matches(regex2));
System.out.println("027-42424".matches(regex2));
System.out.println("0712-3242434".matches(regex2));
System.out.println("-----------------------------");
//邮箱号码
//分3个部分
//3232323@qq.com zhangsan@itcast.cnn dlei0009@163.com dlei0009@pci.com.cn
//第一部分:@左边 \\w+ 表示任意的字母数字下划线,至少出现一次就可以了
//第二部分:@ 只能出现一次
//第三部分 3.1 .的左边 [\\w&&[^_]]{2,6} 任意的字母加数字,总共出现2-6次(此时不能出现下划线)
//3.2 写一个.即可 表示只能出现一次. [.]{1}
//3.3 .后面的大小写字母都可以,但要是字母,只能出现2~3次 [a-zA-Z]{2,3}
// 我们可以把3.2和3.3看成一组,这一组可以出现1次或者二次
String regex3 ="\\w+@[\\w&&[^_]]{2,6}([.]{1}[a-zA-Z]{2,3}){1,2}";
System.out.println("3232323@qq.com".matches(regex3));
System.out.println("zhangsan@itcast.cnn".matches(regex3));
System.out.println("dlei0009@163.com".matches(regex3));
System.out.println("lei0009@pci.com.cn".matches(regex3));
}
}
结果
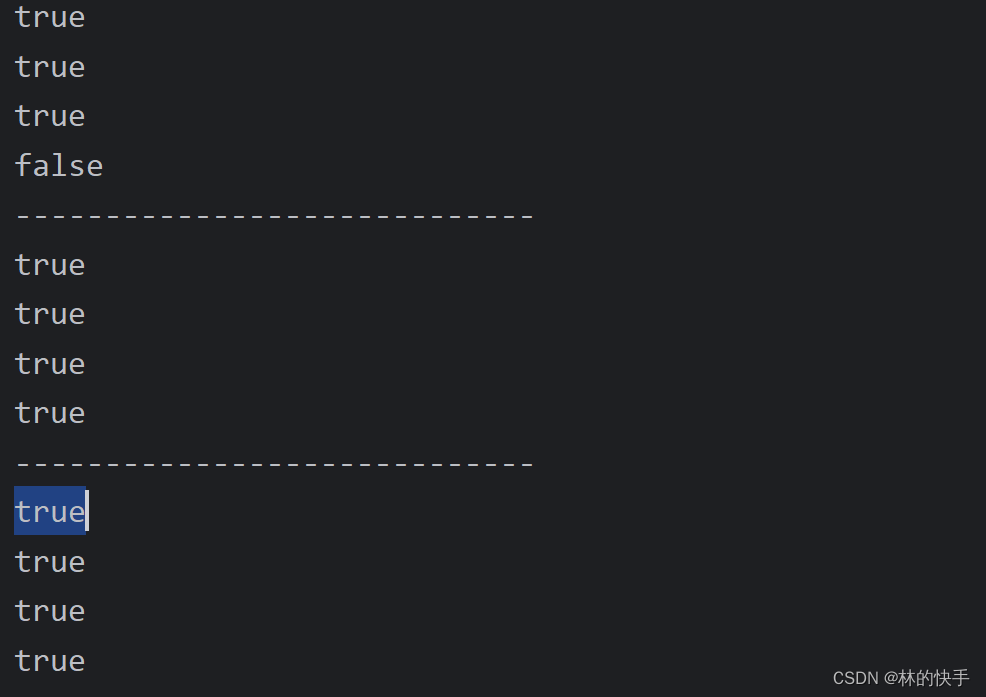
练习2
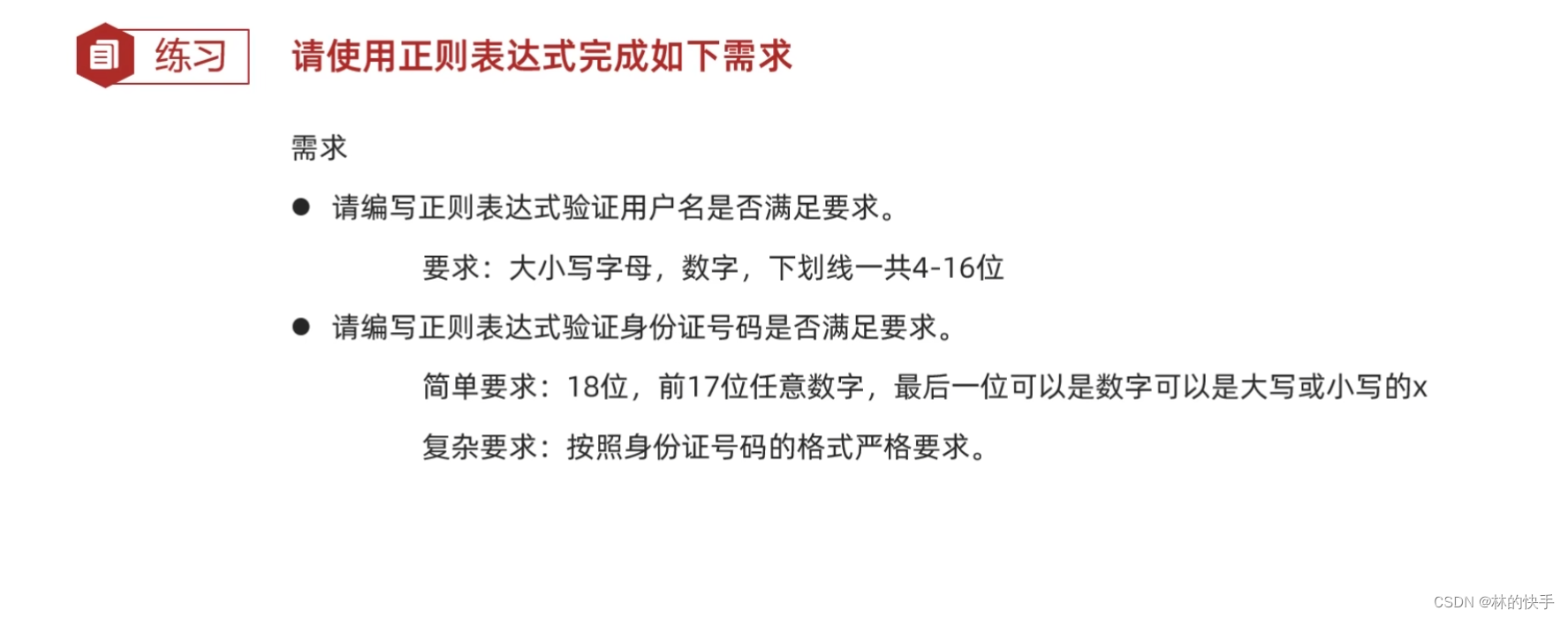
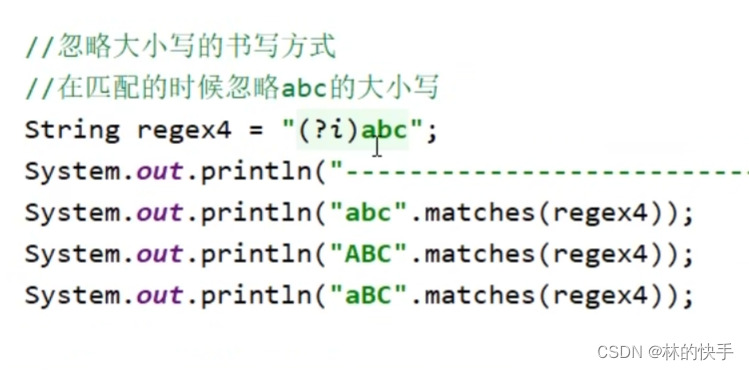
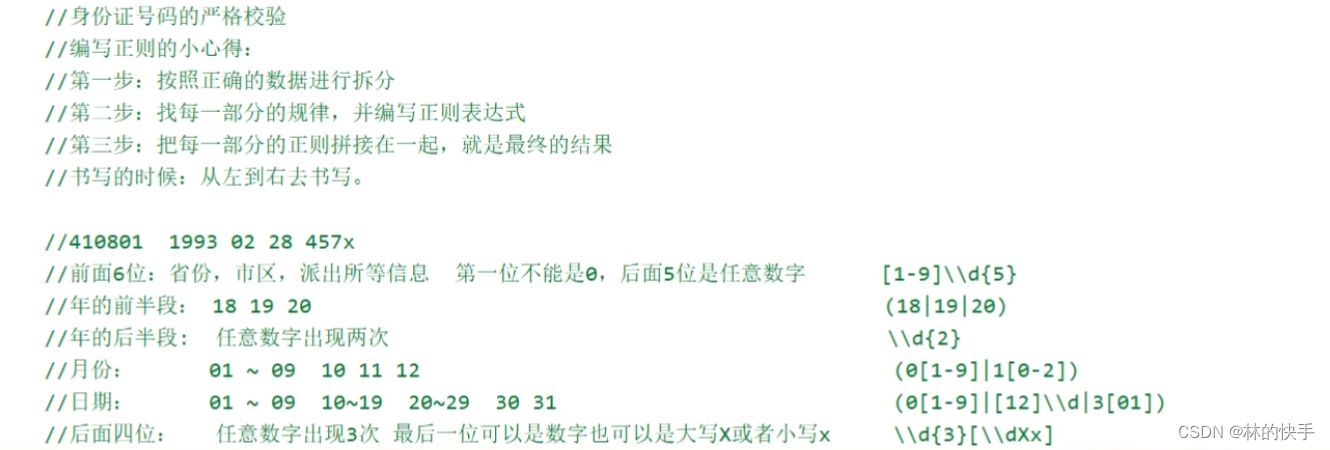
cpp
package zzbds;
import java.util.Scanner;
public class zzbdsdem7 {
public static void main(String[] args) {
//要求 :大小写字母,数字,下划线一共4-16位
Scanner sc = new Scanner(System.in);
System.out.println("请输入用户名");
String username = sc.next();
boolean flag1 = cheakusername(username);
if (flag1) {
System.out.println("满足");
} else {
System.out.println("不满足");
}
System.out.println("请输入身份证号码");
String peronID = sc.next();
boolean flag2 = cheakPersonID(peronID);
if (flag2) {
System.out.println("满足");
} else {
System.out.println("不满足");
}
}
private static boolean cheakPersonID(String peronID) {
String regex ="[1-9]\\d{5}(18|19|20)\\d{2}(0[1-9]|1[0-2])(0[1-9]|[12]\\d|3[01])\\d{3}(\\d|X|x)";
return peronID.matches(regex);
}
/*private static boolean cheakPersonID(String peronID) {
String regex = "[1-9]\\d{16}[xX\\d]";
return peronID.matches(regex);
}*/
private static boolean cheakusername(String username) {
String regex = "\\w{4,16}";
return username.matches(regex);
}
}
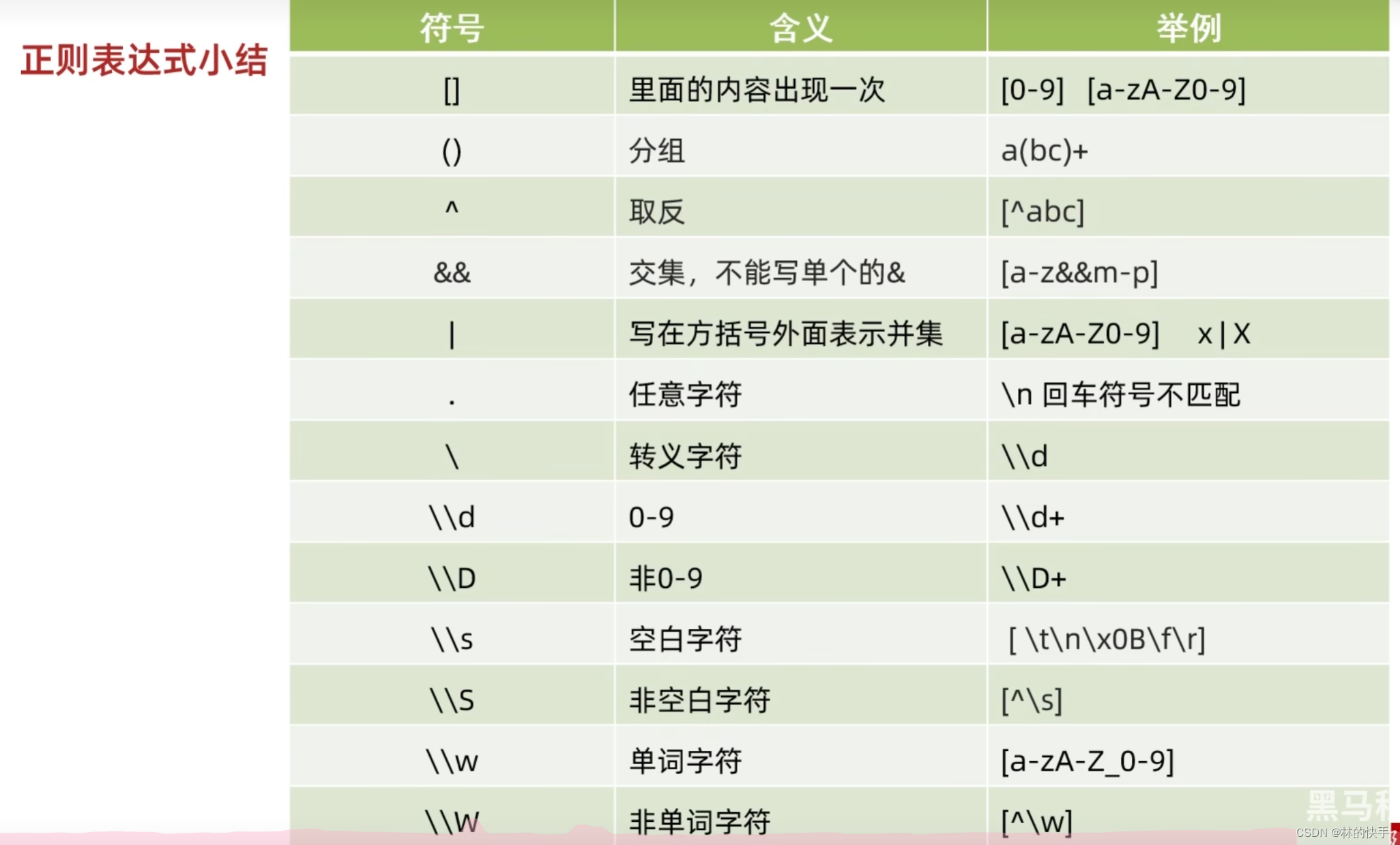
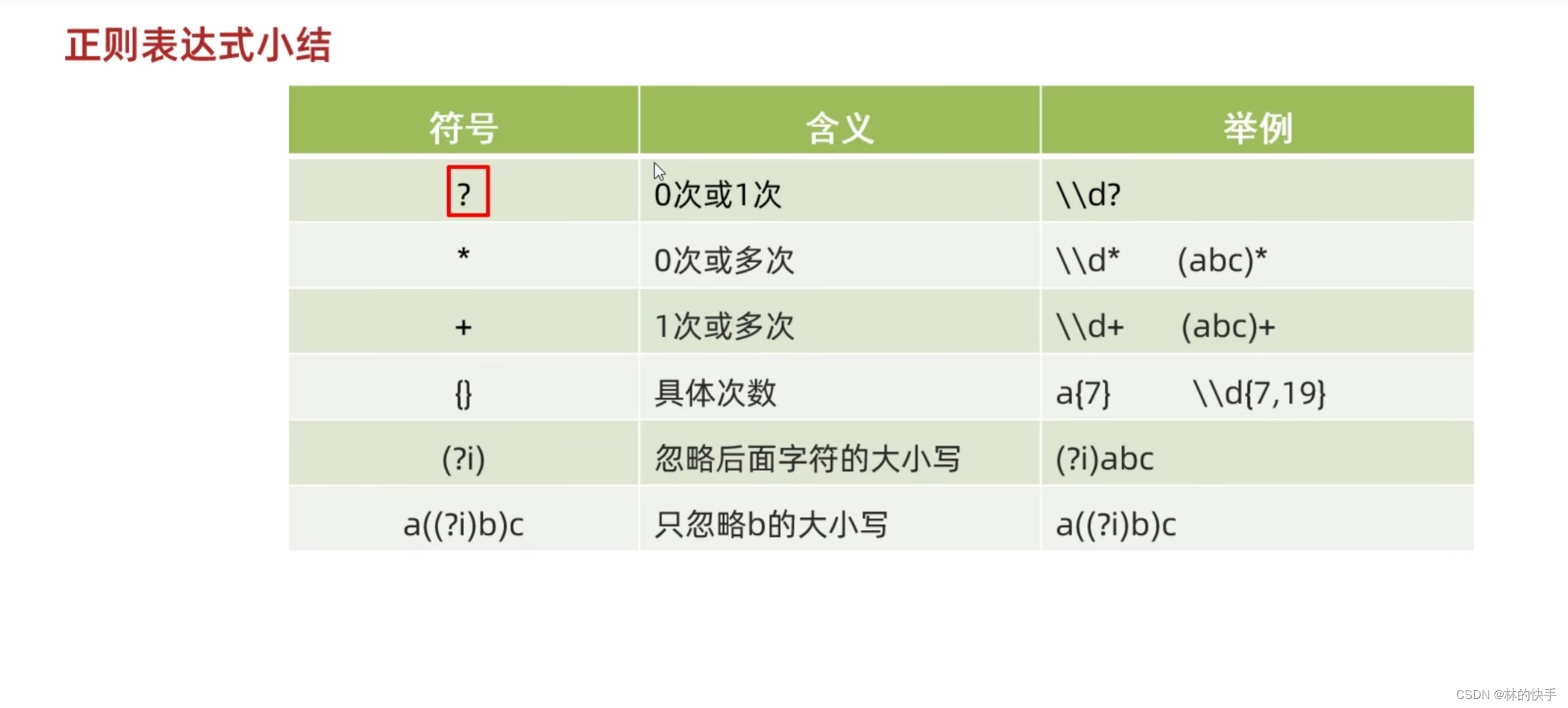
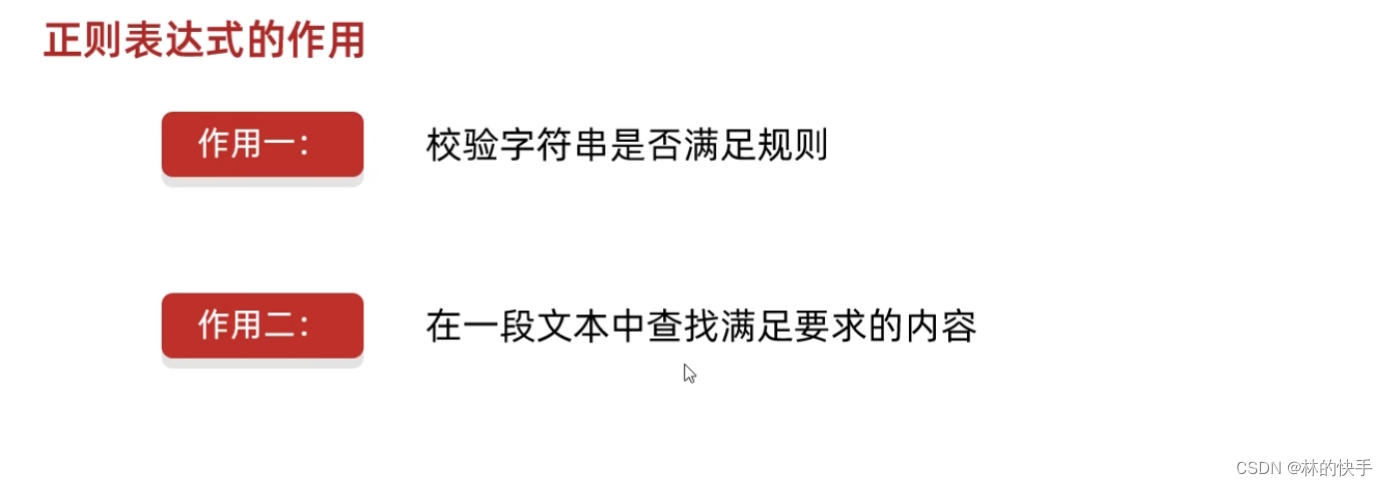