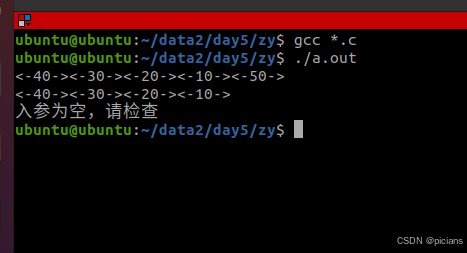
主程序
#include "fun.h"
int main(int argc, const char *argv[])
{
double_p H=create_head();
insert_head(H,10);
insert_head(H,20);
insert_head(H,30);
insert_head(H,40);
insert_tail(H,50);
show_link(H);
del_tail(H);
show_link(H);
free_link(H);
H=NULL;
show_link(H);
return 0;
}
源程序
#include "fun.h"
//创建头节点
double_p create_head()
{
double_p H=(double_p)malloc(sizeof(node));
if(H==NULL)
{
printf("节点申请失败\n");
return NULL;
}
H->prior=H;
H->next=H;
H->len=0;
return H;
}
//创建新节点
double_p create_new(typdata data)
{
double_p new=(double_p)malloc(sizeof(node));
if(new==NULL)
{
printf("节点申请失败\n");
return NULL;
}
new->prior=NULL;
new->next=NULL;
new->data=data;
return new;
}
//判空
int empty_link(double_p H)
{
if(H==NULL)
{
printf("入参为空,请检查\n");
return -1;
}
return H->len==0;
}
//头插
void insert_head(double_p H,typdata data)
{
if(H==NULL)
{
printf("入参为空,请检查\n");
return;
}
double_p new=create_new(data);
new->next=H->next;
H->next=new;
new->next->prior=new;
new->prior=H;
H->len++;
}
//遍历
void show_link(double_p H)
{
if(H==NULL)
{
printf("入参为空,请检查\n");
return;
}
if(empty_link(H))
{
printf("链表已空,无需遍历\n");
return;
}
double_p p=H;
while(p->next!=H)
{
p=p->next;
printf("<-%d->",p->data);
}
putchar(10);
}
//尾插
void insert_tail(double_p H,typdata data)
{
if(H==NULL)
{
printf("入参为空,请检查\n");
return;
}
double_p p=H;
while(p->next!=H)
{
p=p->next;
}
double_p new=create_new(data);
new->next=p->next;
p->next=new;
new->prior=p;
H->prior=new;
H->len++;
}
//尾删
void del_tail(double_p H)
{
if(H==NULL)
{
printf("入参为空,请检查\n");
return;
}
if(empty_link(H))
{
printf("链表已空,无需删除\n");
return;
}
double_p p=H;
while(p->next->next!=H)
{
p=p->next;
}
free(p->next);
p->next=NULL;
p->next=H;
H->prior=p;
H->len--;
}
//释放链表
void free_link(double_p H)
{
if(H==NULL)
{
printf("入参为空,请检查\n");
}
while(H->next != H)
{
del_tail(H);
}
free(H);
H=NULL;
}
头文件
#ifndef _FUN_H
#define _FUN_H
#include <myhead.h>
typedef int typdata;
typedef struct node
{
union
{
typdata data;
int len;
};
struct node * prior;
struct node *next;
}node,*double_p;
double_p create_head();//创建头节点
double_p create_new(typdata data);//创建新节点
int empty_link(double_p H);//判空
void insert_head(double_p H,typdata data);//头插
void show_link(double_p);//遍历
void insert_tail(double_p H,typdata data);//尾插
void del_tail(double_p H);//尾删
void free_link(double_p H);//释放链表
#endif
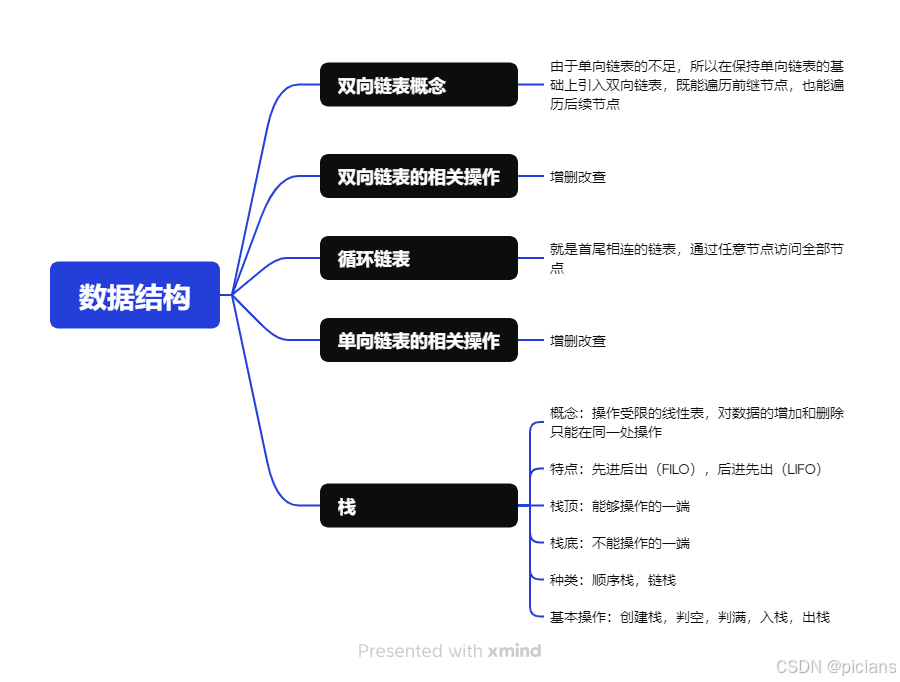