单例模式
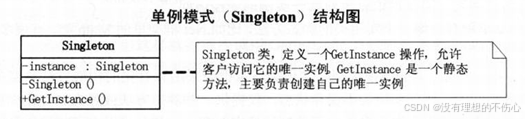
C++
饿汉
cpp
/* HM hungry man 饿汉 */
#include <iostream>
using namespace std;
class Singleton
{
private:
Singleton() { cout << "单例对象创建!" << endl; };
Singleton(const Singleton &);
Singleton &operator=(const Singleton &);
~Singleton() { cout << "单例对象销毁!" << endl; };
static Singleton *myInstance;
public:
static Singleton *getInstance()
{
return myInstance;
}
static void releaseInstance()
{
delete myInstance;
}
};
Singleton *Singleton::myInstance = new Singleton();
int main()
{
Singleton *ct1 = Singleton::getInstance();
Singleton *ct2 = Singleton::getInstance();
Singleton *ct3 = Singleton::getInstance();
Singleton::releaseInstance();
return 0;
}
懒汉
cpp
/* LM lazy man 懒汉 */
#include <iostream>
using namespace std;
class Singleton
{
private:
Singleton() { cout << "单例对象创建!" << endl; };
Singleton(const Singleton &);
Singleton &operator=(const Singleton &);
~Singleton() { cout << "单例对象销毁!" << endl; };
public:
static Singleton *getInstance()
{
static Singleton myInstance;
return &myInstance;
}
};
int main()
{
Singleton *ct1 = Singleton::getInstance();
Singleton *ct2 = Singleton::getInstance();
Singleton *ct3 = Singleton::getInstance();
return 0;
}
C
饿汉
c
/* HM hungry man 饿汉 */
#include <stdio.h>
// 定义单例结构体
typedef struct
{
int initialized;
} Singleton;
// 静态全局变量,初始化单例对象
static Singleton singleton = {1};
// 单例对象的访问函数
Singleton *getSingleton(void)
{
static Singleton *ptr = &singleton; // 静态局部变量,初始化单例对象指针
return ptr;
}
int main()
{
Singleton *ct1 = getSingleton();
Singleton *ct2 = getSingleton();
Singleton *ct3 = getSingleton();
// 注意:在C语言中,单例对象的生命周期通常与程序相同,
// 不需要显式释放,因为程序结束时资源会自动释放。
// 但是,如果单例对象持有需要释放的资源,应该在适当的地方释放。
return 0;
}
懒汉
c
/* LM lazy man 懒汉 */
#include <stdio.h>
typedef struct
{
int initialized;
} Singleton;
static Singleton *getSingleton(void)
{
static Singleton instance = {0}; // 静态局部变量,只初始化一次
static Singleton *ptr = NULL; // 静态局部指针,用于返回单例对象
if (ptr == NULL)
{
ptr = &instance; // 第一次调用时初始化指针
instance.initialized = 1; // 标记单例对象已初始化
printf("单例对象创建!\n");
}
return ptr;
}
int main()
{
Singleton *ct1 = getSingleton();
Singleton *ct2 = getSingleton();
Singleton *ct3 = getSingleton();
// 如果需要显示单例对象的生命周期结束,可以在这里释放资源或做一些清理工作
// 但请注意,C语言中单例对象的销毁通常是在程序结束时自动发生的
return 0;
}