游戏效果
游戏中:
游戏中止:
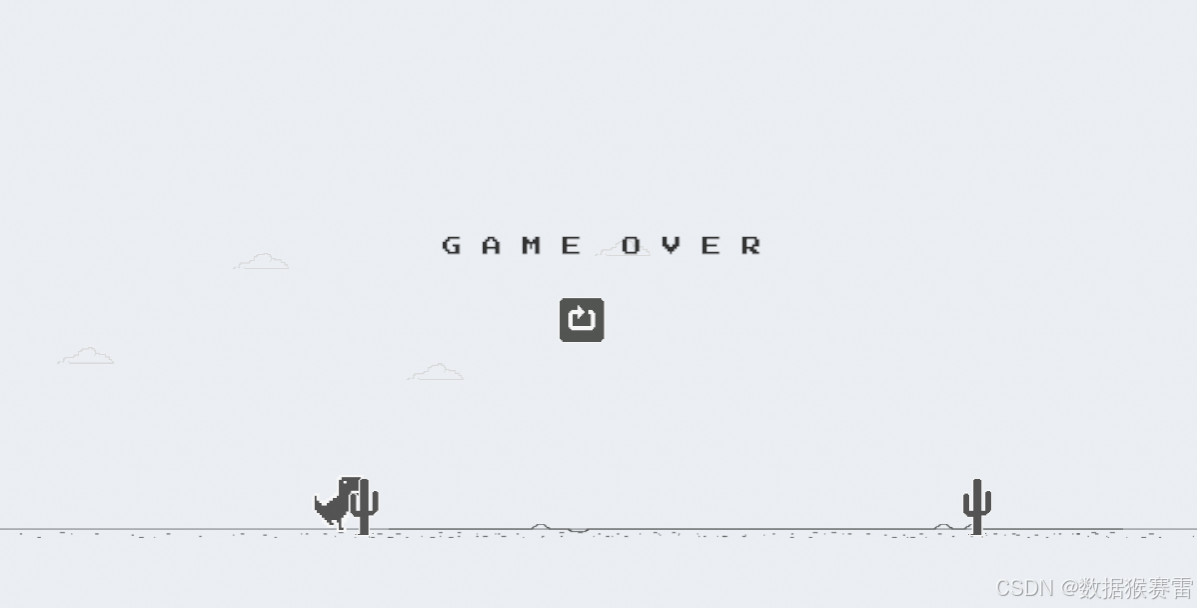
一、制作参考
如何制作游戏?【15分钟】教会你制作Unity小恐龙游戏!新手15分钟内马上学会!_ unity教学 _ 制作游戏 _ 游戏开发_哔哩哔哩_bilibili
二、图片资源
https://download.csdn.net/download/benben044/89522911?spm=1001.2014.3001.5501
三、创建场景
1、将资源都拖到Assets目录下

2、调整编辑布局
将Scene、Hierarchy、Game这3个模块分开,方便可以同时观察到三个模块的信息。
同时将Main Camera的color设置为白色,方便看到ground的颜色。
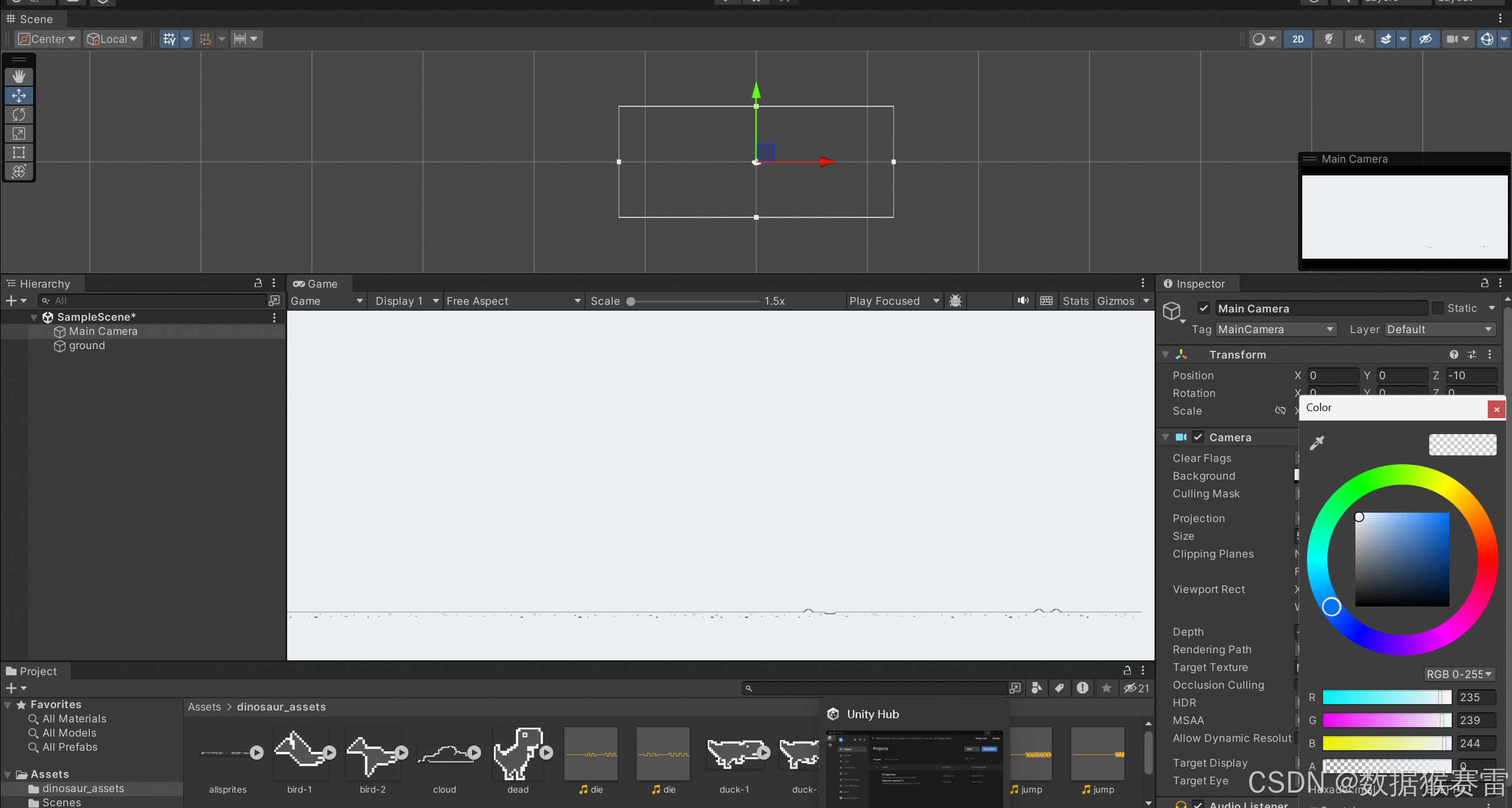
3、放dinosaur到scene
将assets中的run-2放到Scene中,就可以在Game中看到dinosaur的图案,同时将恐龙rename为Dinosaur。
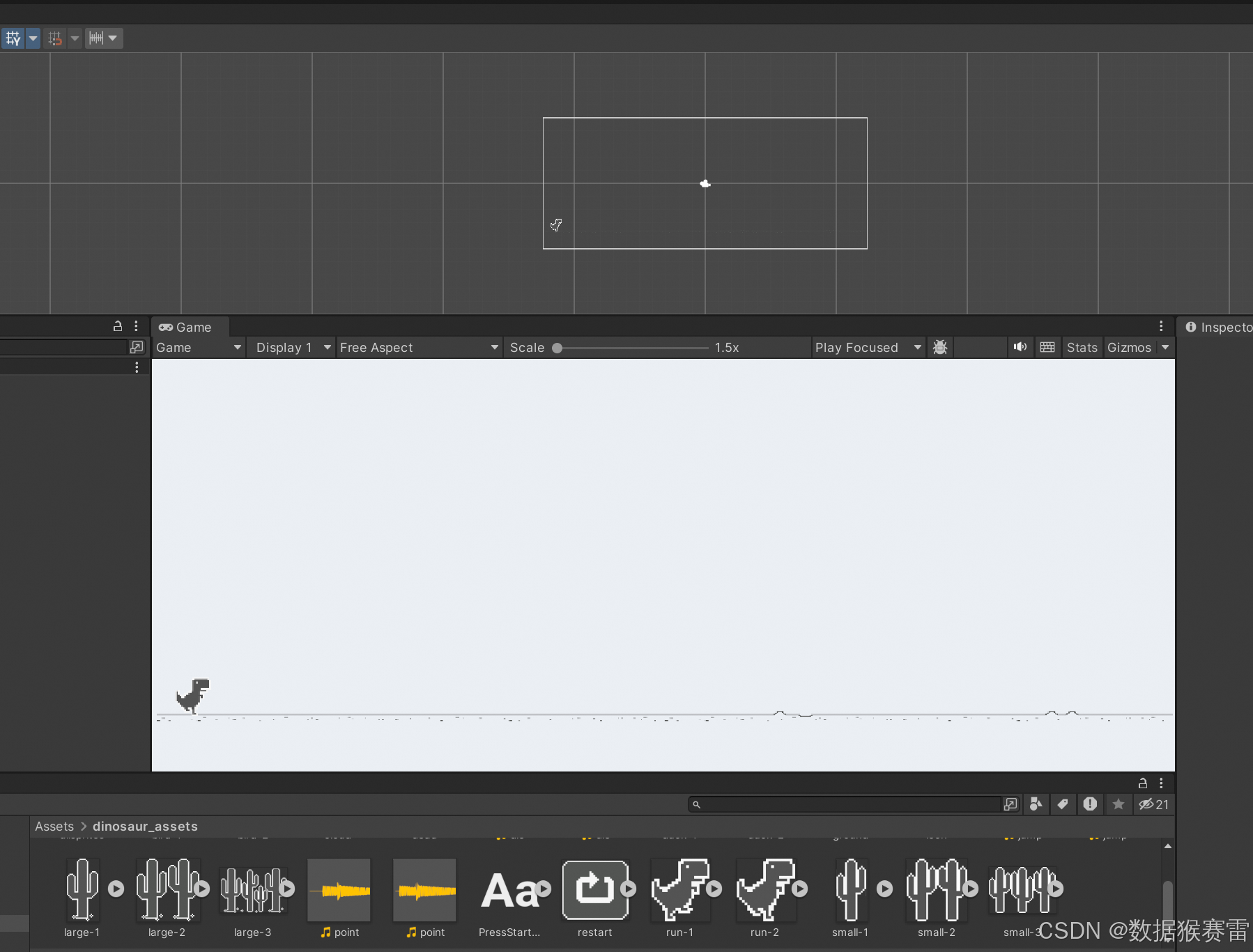
4、添加cloud到scene
同时给cloud进行重命名。
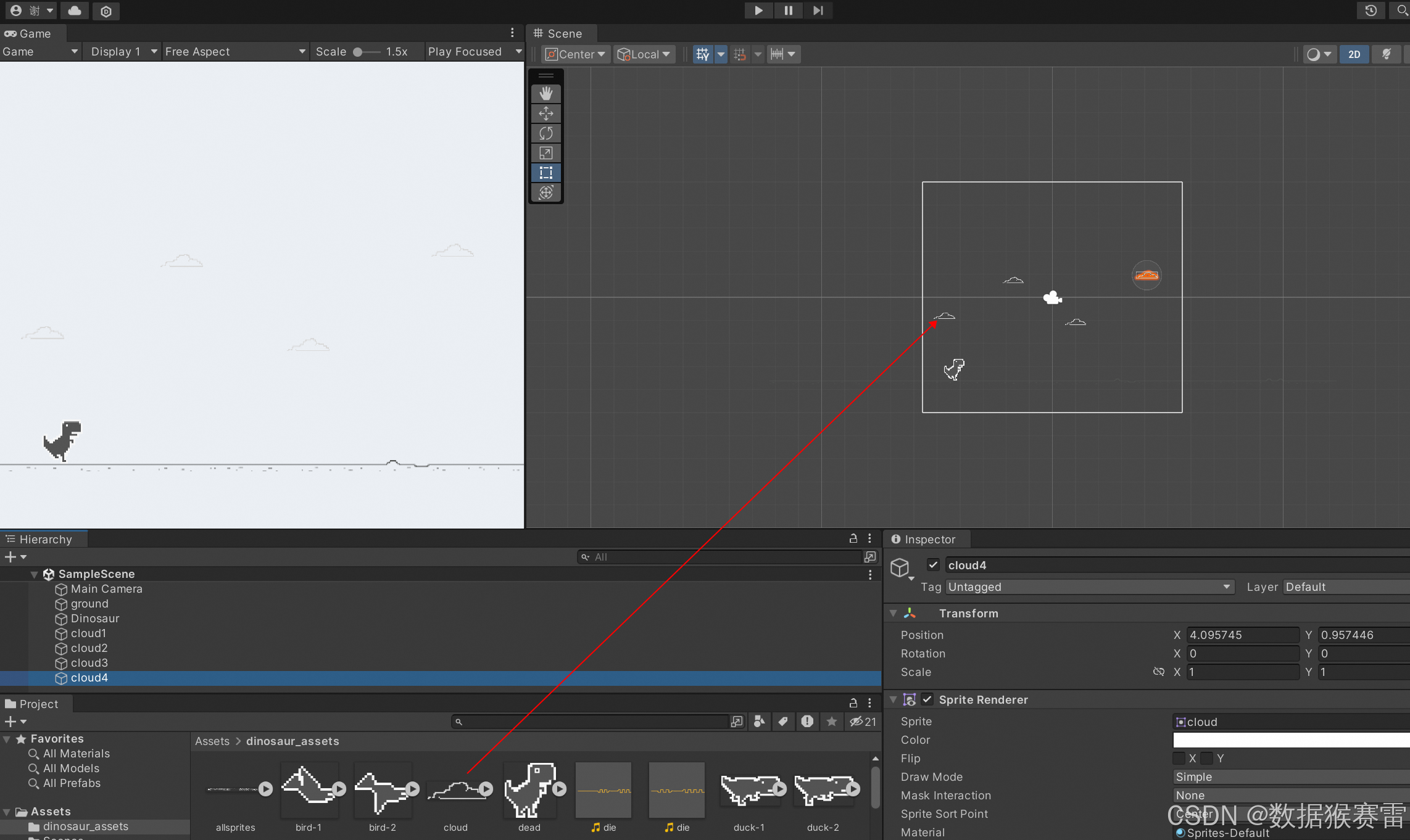
5、给dinosaur和ground添加组件
(1)给dinosaur添加Rigidbody 2d组件,使其具有物理属性,设置Gravity Scale为2,重力大一点下降速度会更快一点。
(2)再给dinosaur添加Box Collider 2d组件,使其具有碰撞属性
(3)接着给ground添加Box Collider 2d组件,使其具有碰撞属性
通过以上操作,dinosaur因为有物理属性所以会自然掉落,但是因为dinosaur和ground都有碰撞属性,所以dinosaur掉到地面后就停止掉落了。
在测试中如果发现Dinosaur掉到地面后脚没有落地,可以编辑Box Collider 2D中的Edit Collider,调整碰撞的范围。
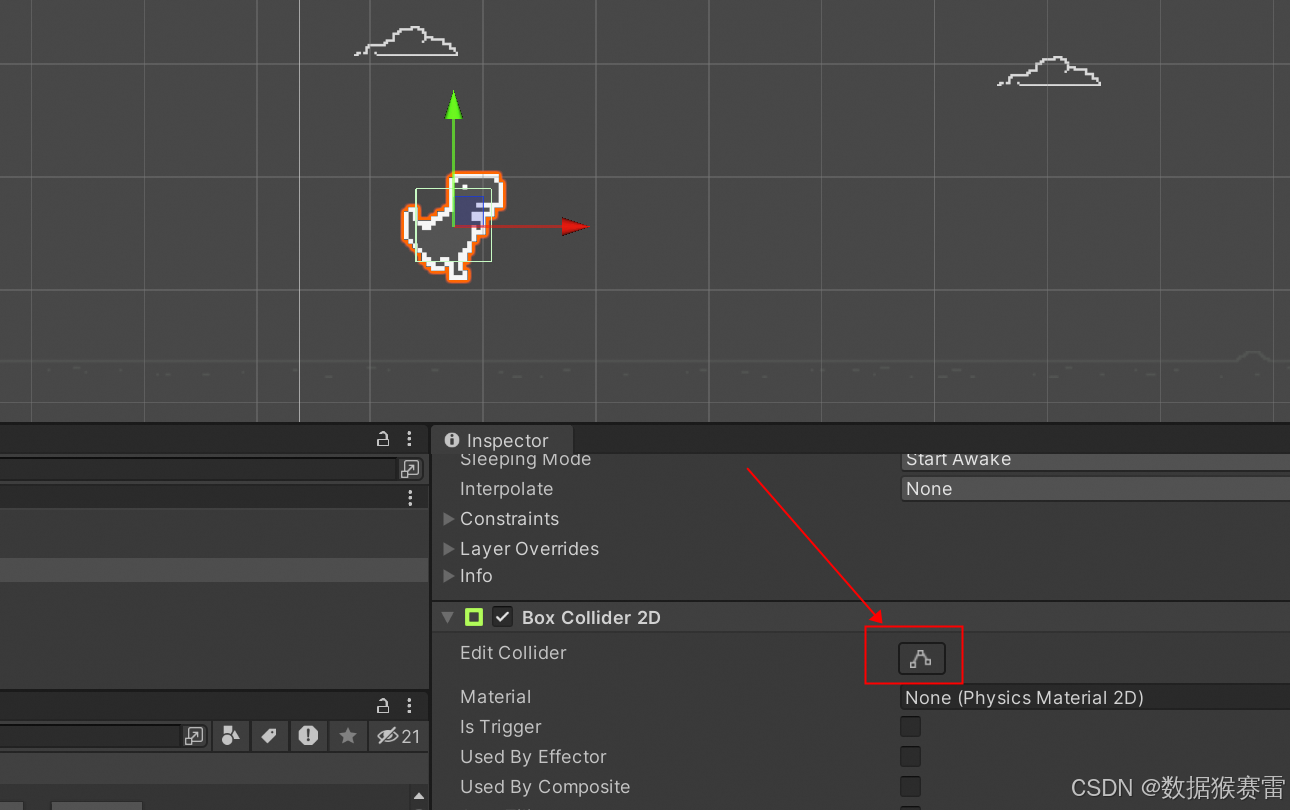
四、编辑C#脚本
1、创建恐龙脚本
cs
public class Dinosaur : MonoBehaviour
{
Rigidbody2D rb;
bool isJumping;
public float jump; // 当为public时即可在Inspector面板看到属性信息
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody2D>();
isJumping = false;
}
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown(KeyCode.Space) && isJumping == false) {
rb.velocity = new Vector2(0, jump);
isJumping = true;
}
}
private void OnCollisionEnter2D(Collision2D collision)
{
// 只有碰到底才能跳,否则在天空中就飞起来了
isJumping = false;
}
}
在Dinosaur的Dinosaur组件中设置jump为8。
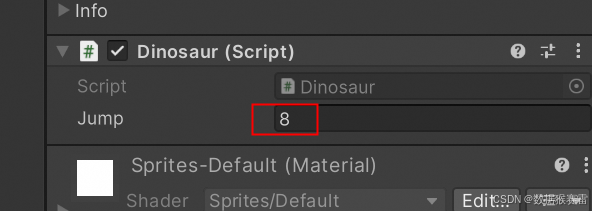
2、创建恐龙动画
首先,在Assets下创建Animations的目录。
在Window->Animation下创建动画组件,然后点击Scene下的Dinosaur,最后点击Create,在Assets下的Animations下创建DinosaurAnimation.anim文件,如下图所示:
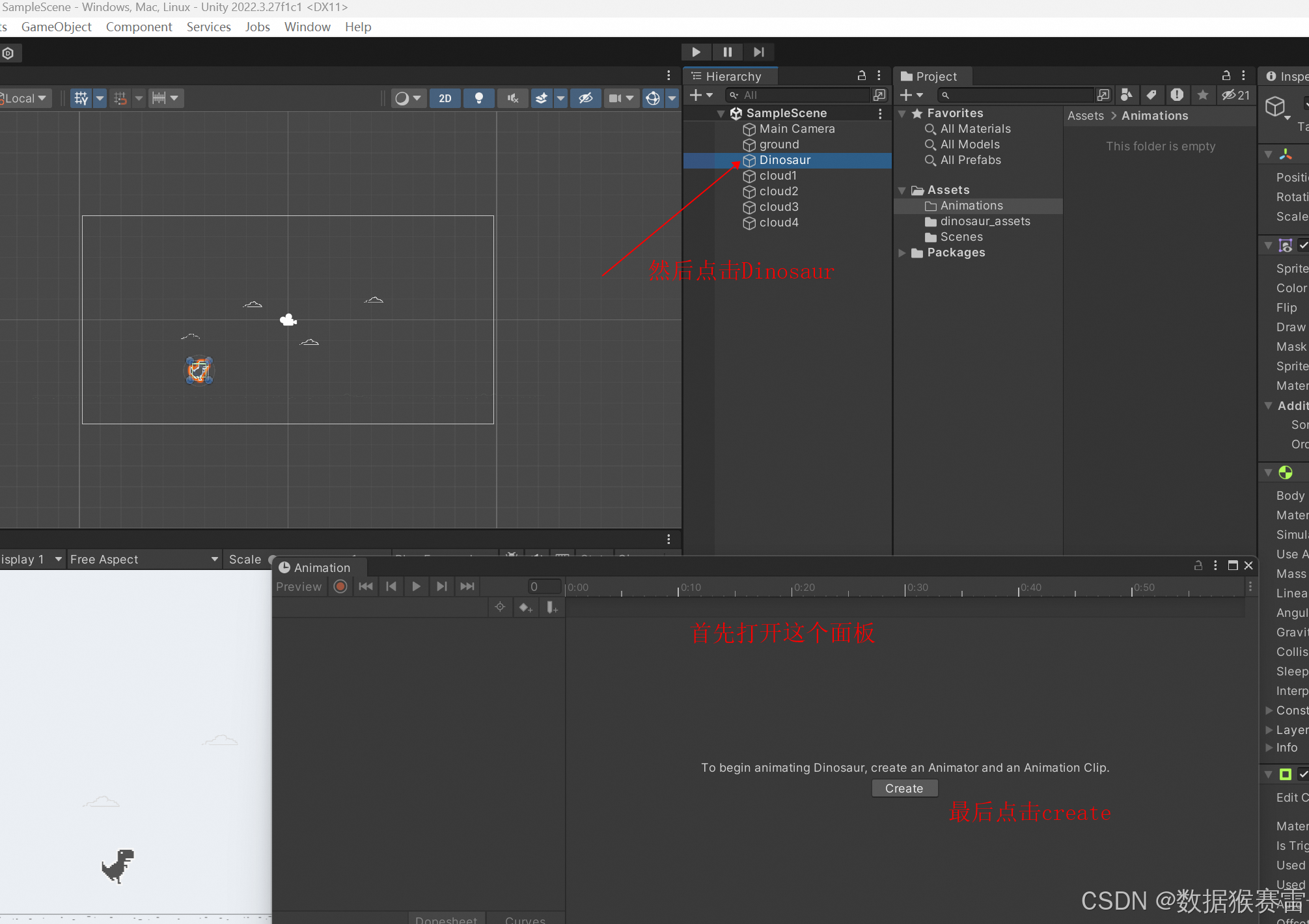
然后将dinosaur_assets下的run-1和run-2拖入animation编辑器,第1个点是run-1,第2个点是run-2,第3个点是run-1,循环的动作。
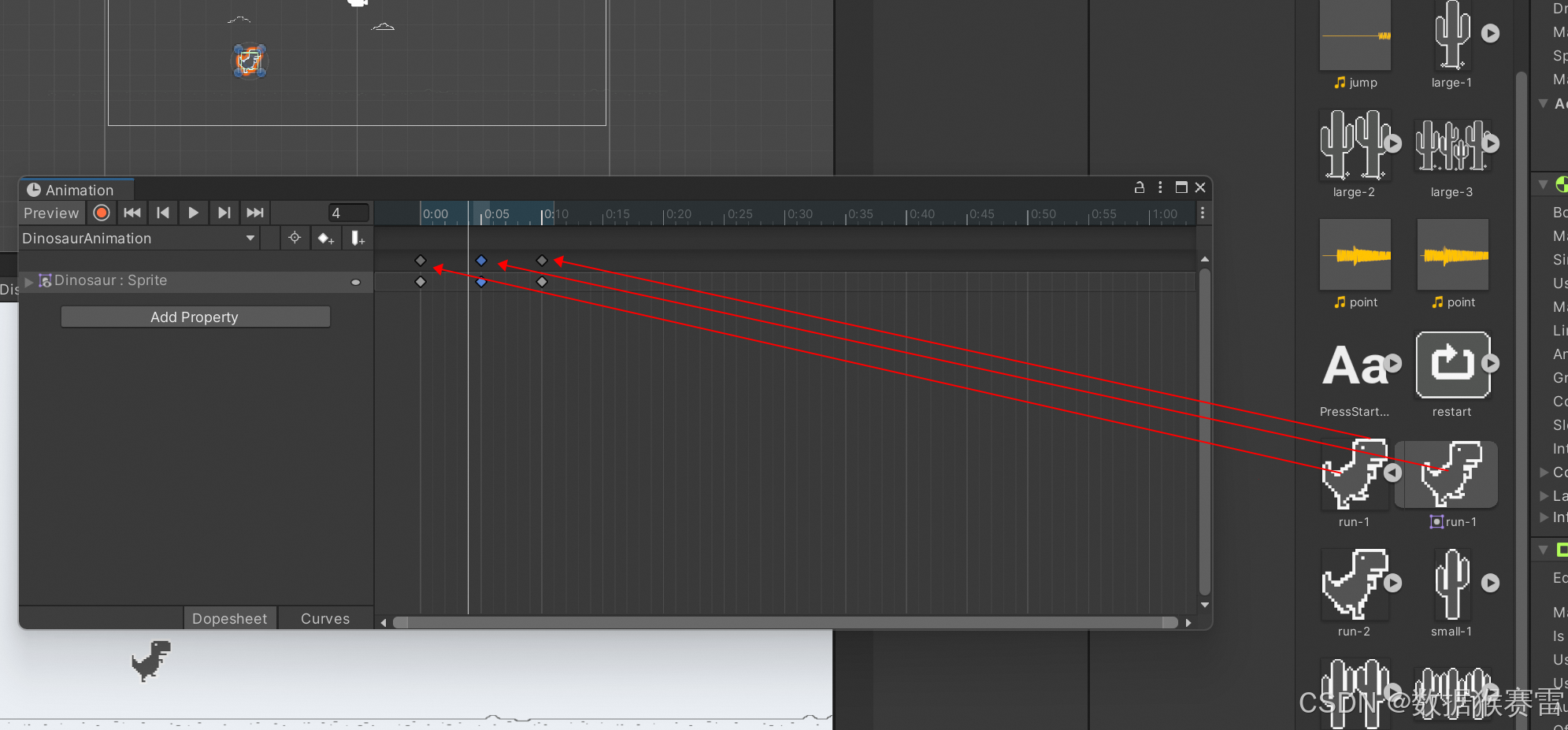
3、创建Movement脚本
在Assets下创建Movement的脚本,该脚本的功能是:当角色在run时,天上的云、地面都在向左移动。即,角色不动,参照物动,从而造成角色向右移动的假象。
cs
public class Movement : MonoBehaviour
{
public float movementSpeed;
public float startPosition;
public float endPosition;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
transform.position = new Vector2(transform.position.x - movementSpeed * Time.deltaTime, transform.position.y);
// 到dinosaur快要越界掉下去时,从开始处重新开始run
if (transform.position.x <= endPosition){
transform.position = new Vector2(startPosition, transform.position.y);
}
}
}
给ground,所有的cloud都加载该脚本,并且设置3个变量分别为5,5,-15。
4、加入仙人掌
将仙人掌放到Scene中,并且添加Box Collider 2D的脚本,这样恐龙就不能穿透它了。同时加入Movement的脚本,参数设置也是5,5,-15。
5、创建GameManager
在Scene中Create Empty,名为GameManager。
然后在Assets下创建GameManager的脚本,并把该脚本赋予GameManager的物体上。
脚本内容如下:
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class GameManager : MonoBehaviour
{
public GameObject cactus; // 仙人掌
public GameObject cactusSpawnPostition; //仙人掌出现的位置
public float spawnTime; // 仙人掌出现的时间
float timer; // 计时器
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
timer += Time.deltaTime;
if (timer >= spawnTime)
{
Instantiate(cactus, cactusSpawnPostition.transform);
timer = 0;
}
}
}
意思是:当超过spawnTime时,就让cactus在cactusSpawnPosition出现。
6、创建cactusSpawnPosition
这个就是上个脚本中仙人掌每次自动生成的位置。
修改该对象的tag为红色菱形,这样在scene中更容易被辨认出来。
然后在scene中确定位置,如下图所示:
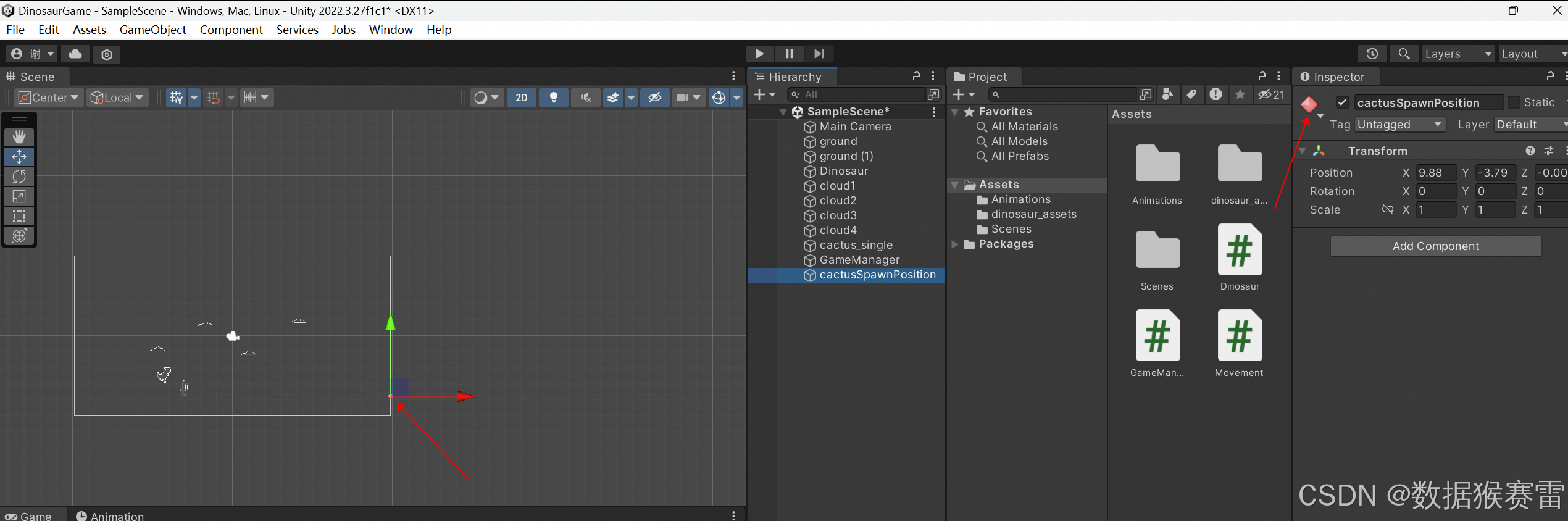
接着拖动hierachy中的cactus到Assets成为prefab预制体,修改器transform中的的Position全是0。
然后,设置GameManager的参数如下:
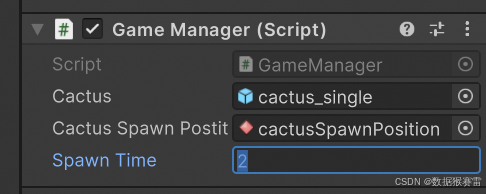
7、给仙人掌添加tag
点击Asset中的cactus_single,然后在其Tag旁边的Untagged点击一下,选择Add Tag如下图:
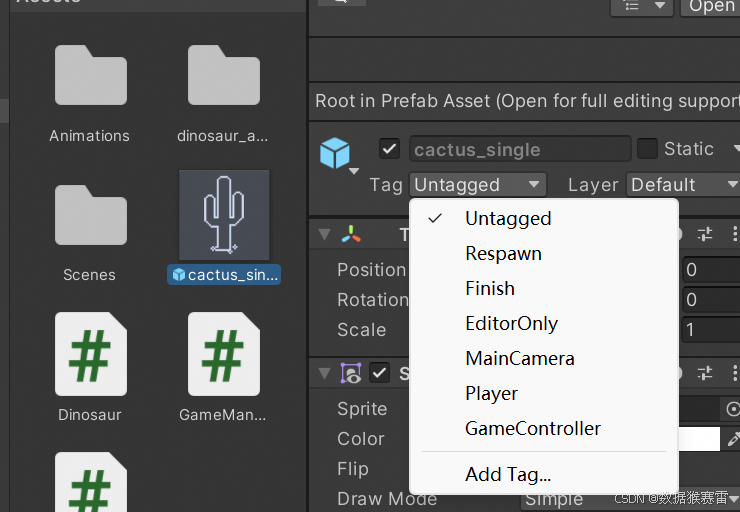
添加Cacuts的tag如下:
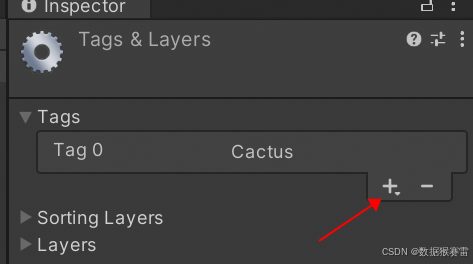
在Tag栏位选择刚刚创建的Cactus,如下:
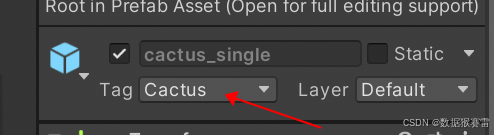
8、删除不用的仙人掌
如果仙人掌已经在movement中endPosition的左边,就可以destory它。
所以,如果越界后,是仙人掌就destroy它,否则类似ground、cloud就循环从头开始。
所以优化Movement脚本如下:
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement : MonoBehaviour
{
public float movementSpeed;
public float startPosition;
public float endPosition;
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
transform.position = new Vector2(transform.position.x - movementSpeed * Time.deltaTime, transform.position.y);
// 到dinosaur快要越界掉下去时,从开始处重新开始run
if (transform.position.x <= endPosition){
if(gameObject.tag == "Cactus")
{
Destroy(gameObject);
}
else
{
transform.position = new Vector2(startPosition, transform.position.y);
}
}
}
}
9、创建GameOver和Restart
(1)GameOver
在Hierarchy中选择UI -> Legacy -> Text如下:
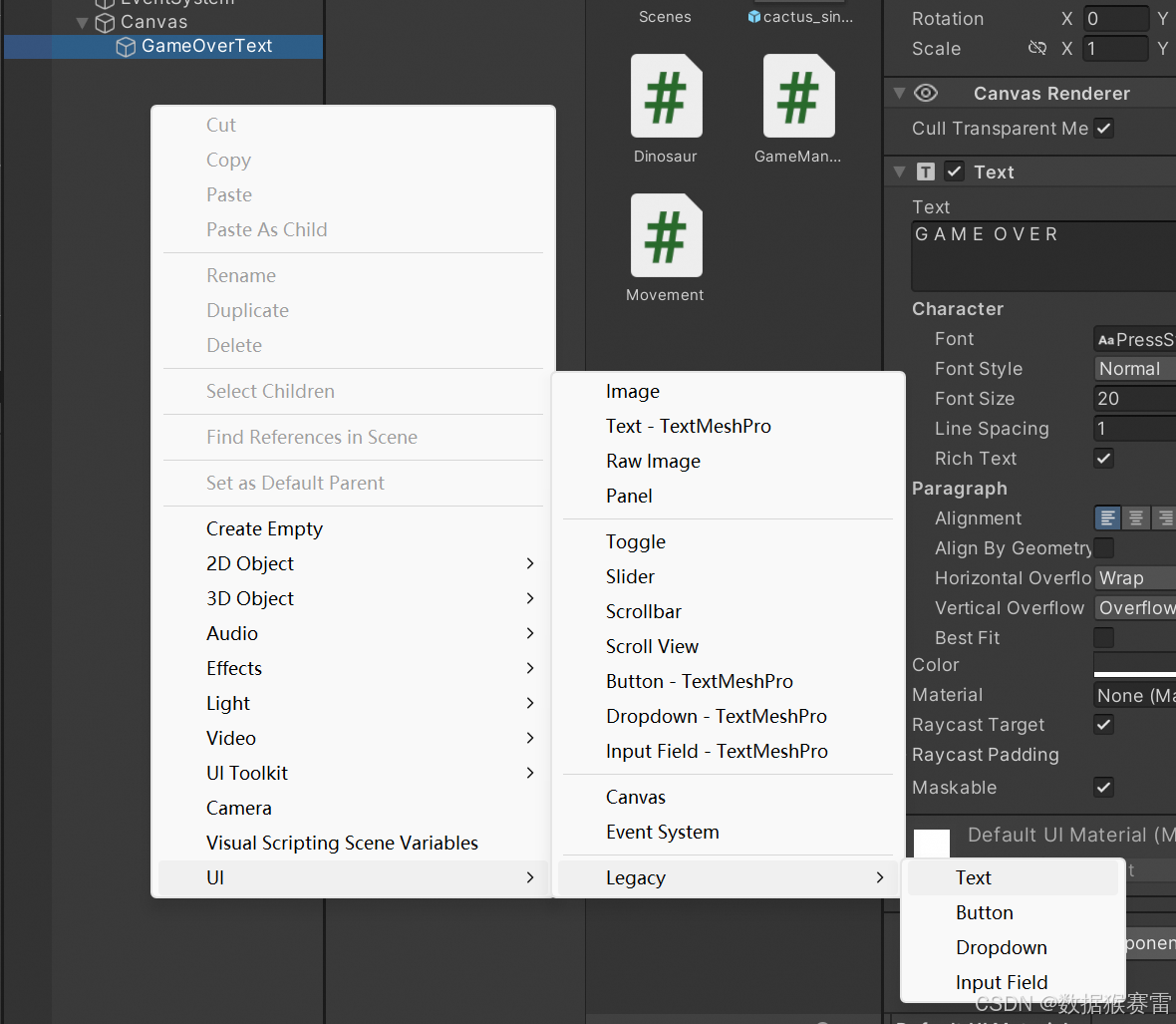
编写文字:GAME OVER
并且选择好FFT格式的字体。
(2)Restart
在Cavas下再创建Button,位于UI -> Legacy -> Button,重命名为RestartButton。
然后将Button的图标替换为已有的素材,在调整下size。
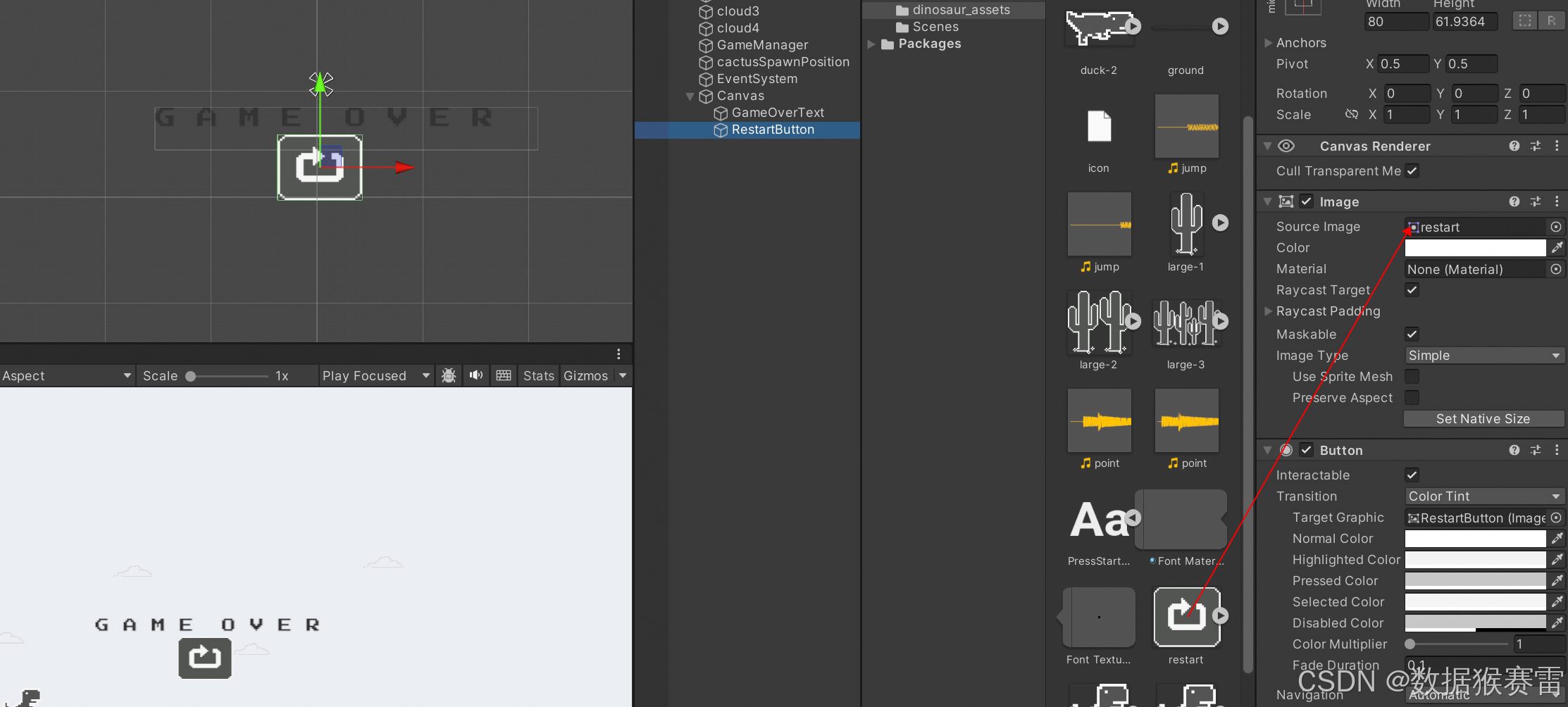
(3)创建Panel
将上面创建的2个UI组件都装到该Panel下,同时调整Panel->Color->A值为0。
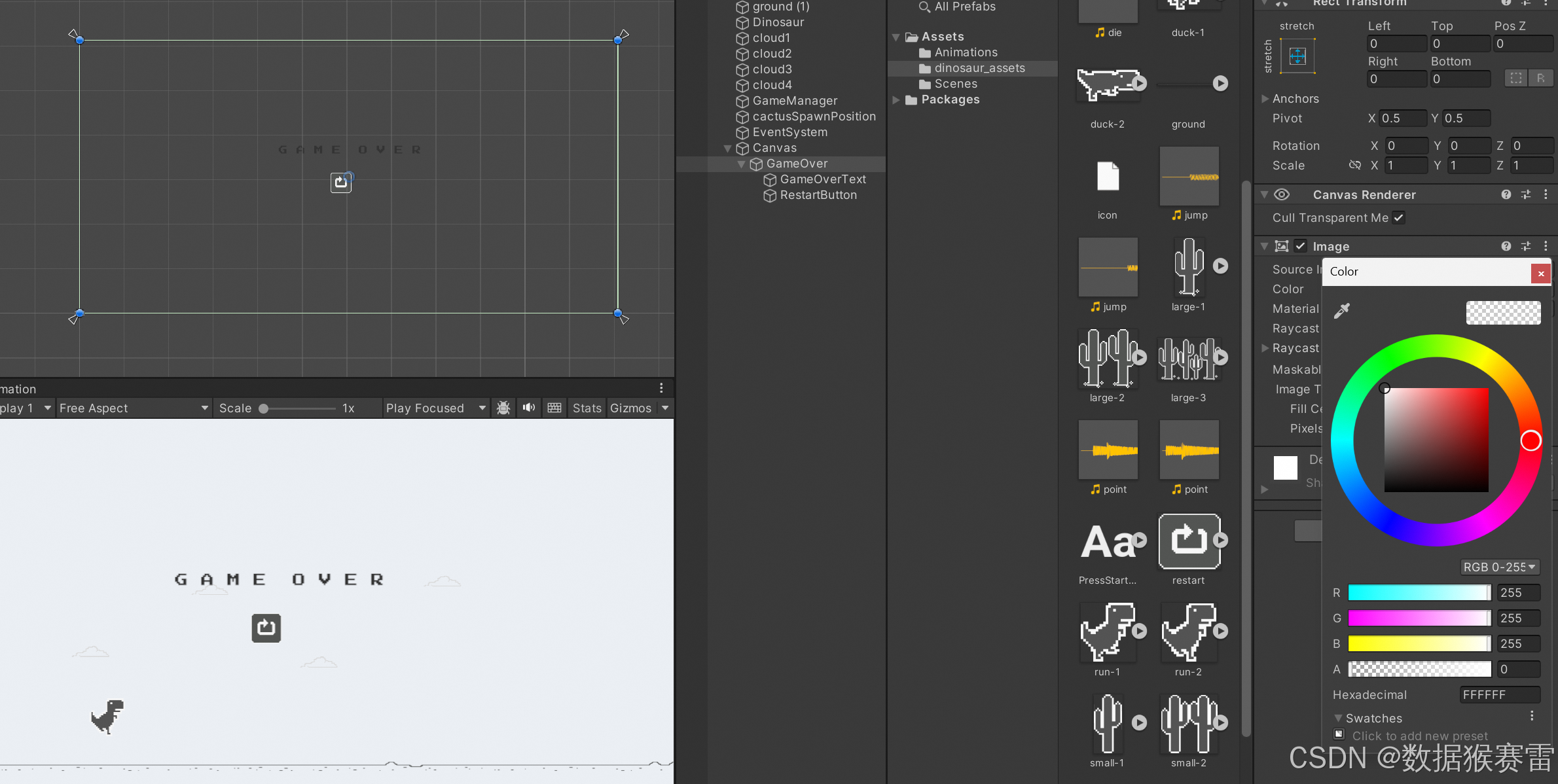
这样,同时控制Panel的Active状态,就可以控制Panel的出现和消失。
只有当触发GameOver时该Panel才会出现,否则该Panel的Active为false。
10、 保存场景
在File->Save As中,在Assets->Scenes下保存场景为lv1,level1的意思。
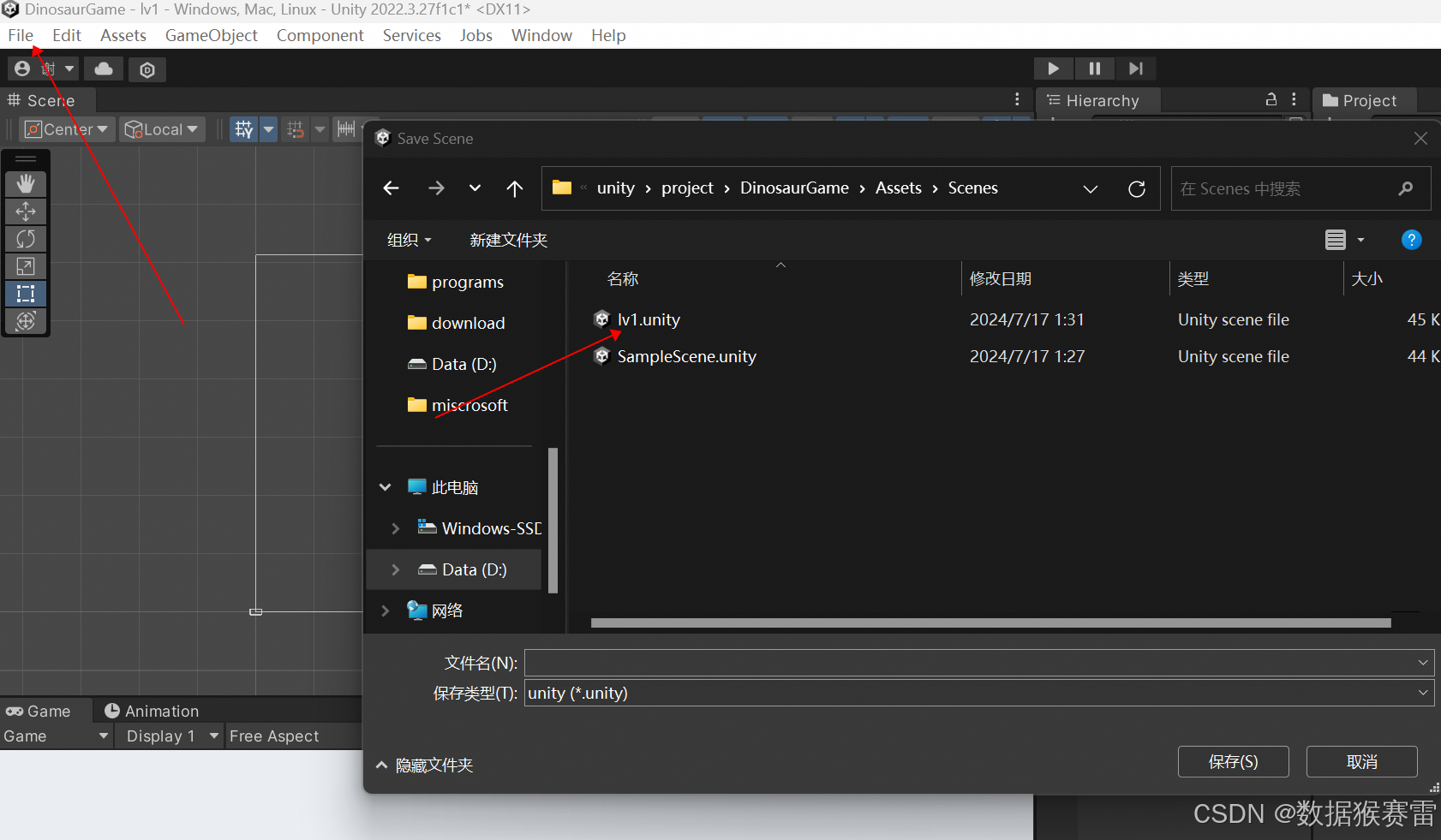
11、在GameManager中加入GameOver和Restart
- 创建GameOverScene变量,对应GameOver的Panel
- Start()中加入控制速度的Time.scaleTime=1
- 在多个函数中设置GameOver的Panel的Active为false
- 在GameOver()中,设置Time.scaleTime=0,同时出现GameOver的Panel
- 在Restart()中,重新加载lv1的scene,同时GameOver的Panel的Active为false
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class GameManager : MonoBehaviour
{
public GameObject cactus; // 仙人掌
public GameObject cactusSpawnPostition; //仙人掌出现的位置
public float spawnTime; // 仙人掌出现的时间
float timer; // 计时器
public GameObject GameOverScene;
// Start is called before the first frame update
void Start()
{
Time.timeScale = 1.0f;
GameOverScene.SetActive(false);
}
// Update is called once per frame
void Update()
{
timer += Time.deltaTime;
if (timer >= spawnTime)
{
Instantiate(cactus, cactusSpawnPostition.transform);
timer = 0;
}
}
public void GameOver()
{
Time.timeScale = 0; // 将游戏暂停
GameOverScene.SetActive(true);
}
public void Restart()
{
SceneManager.LoadScene("lv1");
GameOverScene.SetActive(false);
}
}
编写完代码后,在Inspector中配置GameOverScene的值。
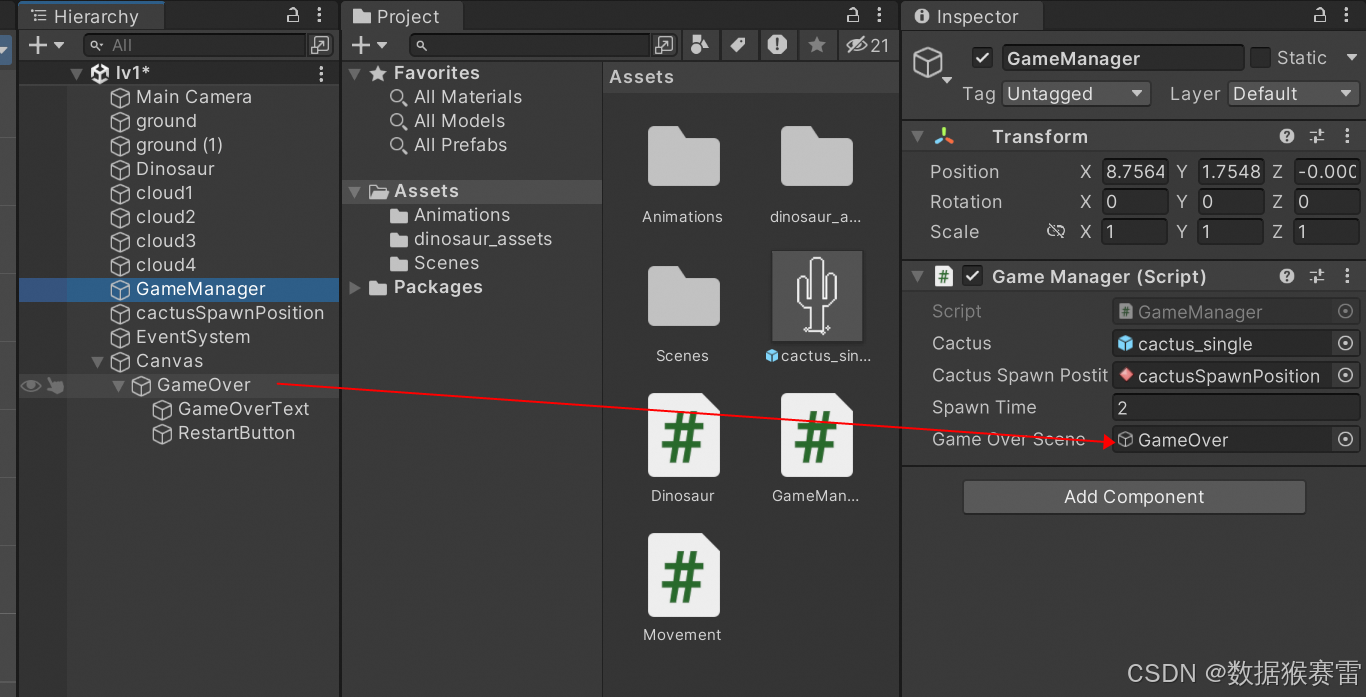
12、在Dinosaur脚本中加入游戏暂停的触发条件
主要是在OnCollisionEnter2D中加入触发条件。
cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Dinosaur : MonoBehaviour
{
Rigidbody2D rb;
bool isJumping;
public float jump; // 当为public时即可在Inspector面板看到属性信息
public GameManager gm;
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody2D>();
isJumping = false;
}
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown(KeyCode.Space) && isJumping == false) {
rb.velocity = new Vector2(0, jump);
isJumping = true;
}
}
private void OnCollisionEnter2D(Collision2D collision)
{
// 只有碰到底才能跳,否则在天空中就飞起来了
isJumping = false;
if(collision.gameObject.tag == "Cactus")
{
// 撞到仙人掌,游戏暂停
gm.GameOver();
}
}
}
编写完代码后,配置GameManager的变量。
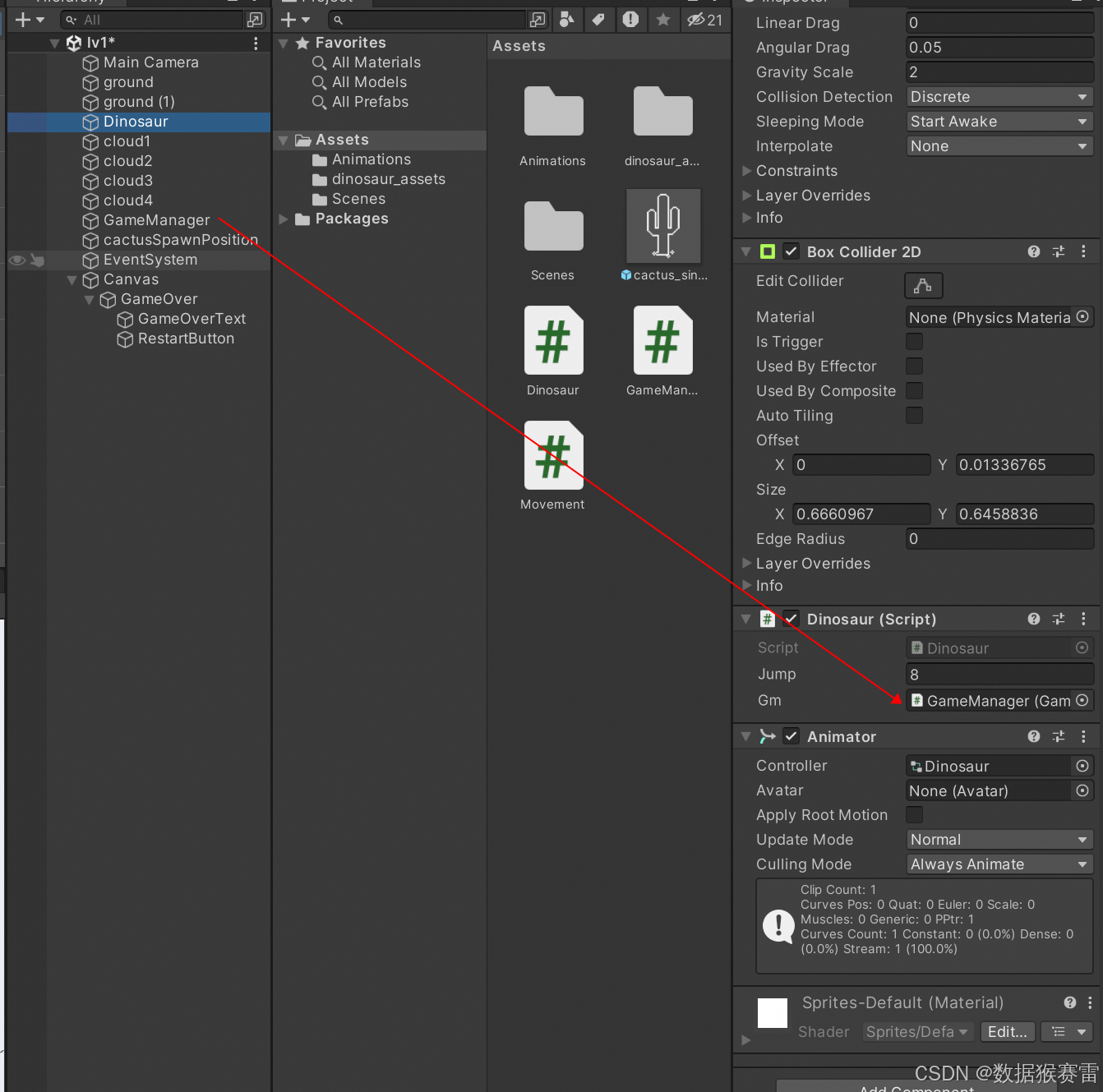
至此,该游戏制作完成!
五、总结
1、检测碰撞:OnCollisionEnter2D,这个是和Update平级的函数
2、物体移动时,transform.position = new Vector2(x, y),其中x是transform.position.x - Time.deltaTime * speed.
3、时间是float类型的数据
4、实例化预设体用Instantiate函数,参数1是实例化的对象,参数2是transform信息,可以用某个物体的transform信息作为参数2
5、检测碰撞的物体时,可以给对方物体一个tag,然后通过collision.gameobject.tag == <tag>判断和该物体是否相撞
6、加载场景使用SceneManager.LoadScene("<场景名称>")
7、如果有多个UI需要一起控制,则统一放到Panel中,然后控制该Panel即可
8、控制暂停、重启,可使用时间变量Time.timeScale