p333-
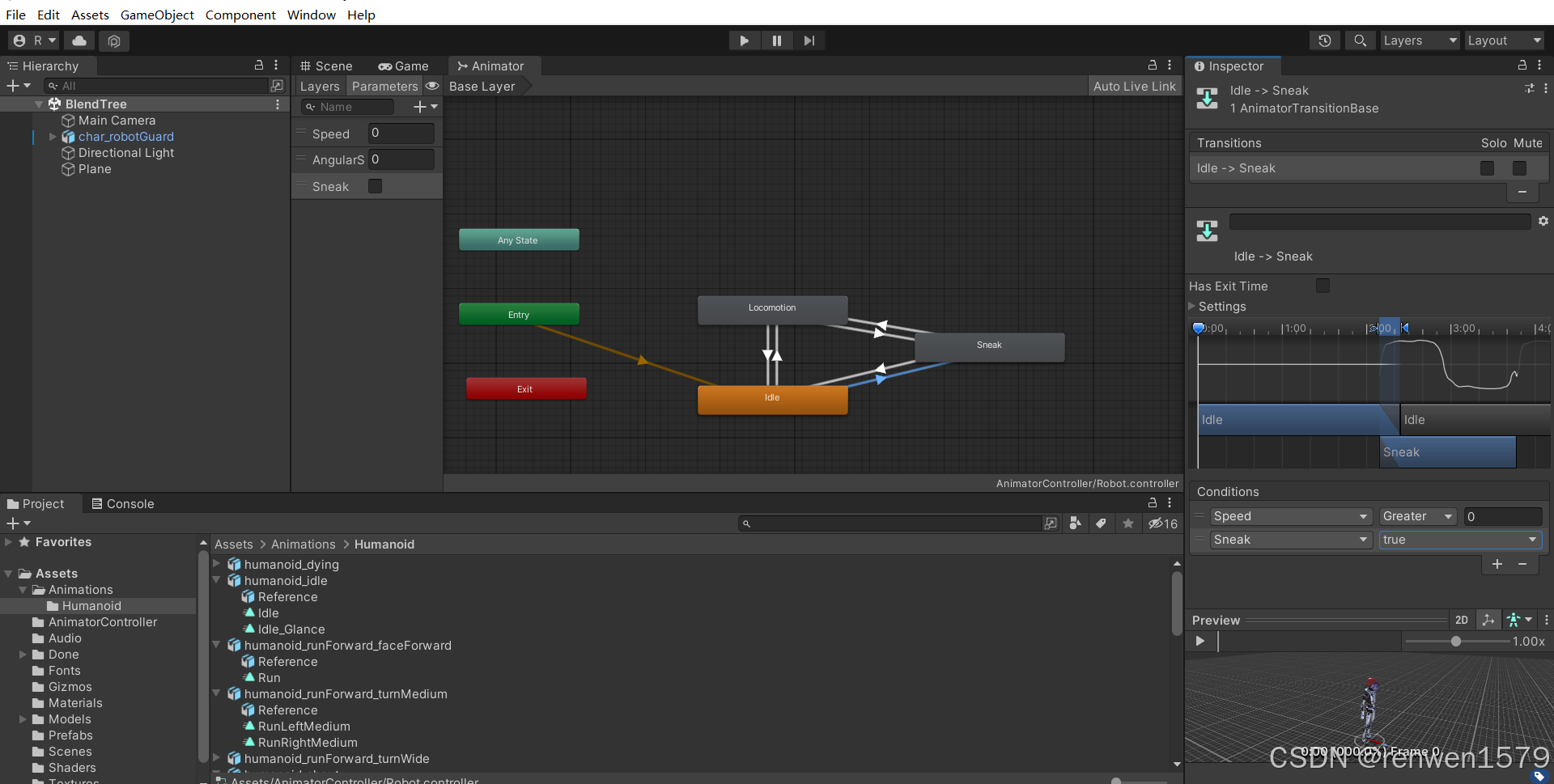
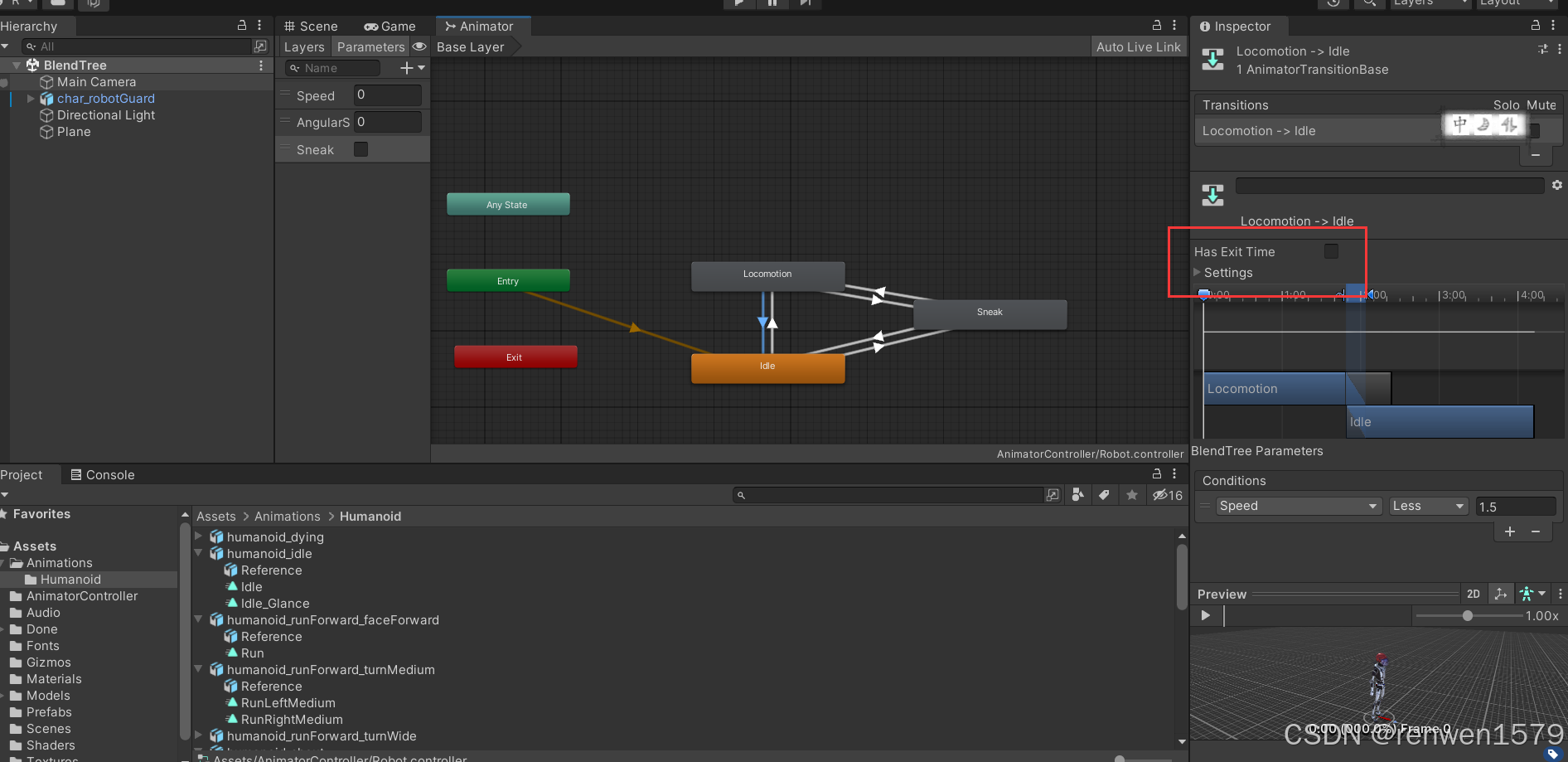
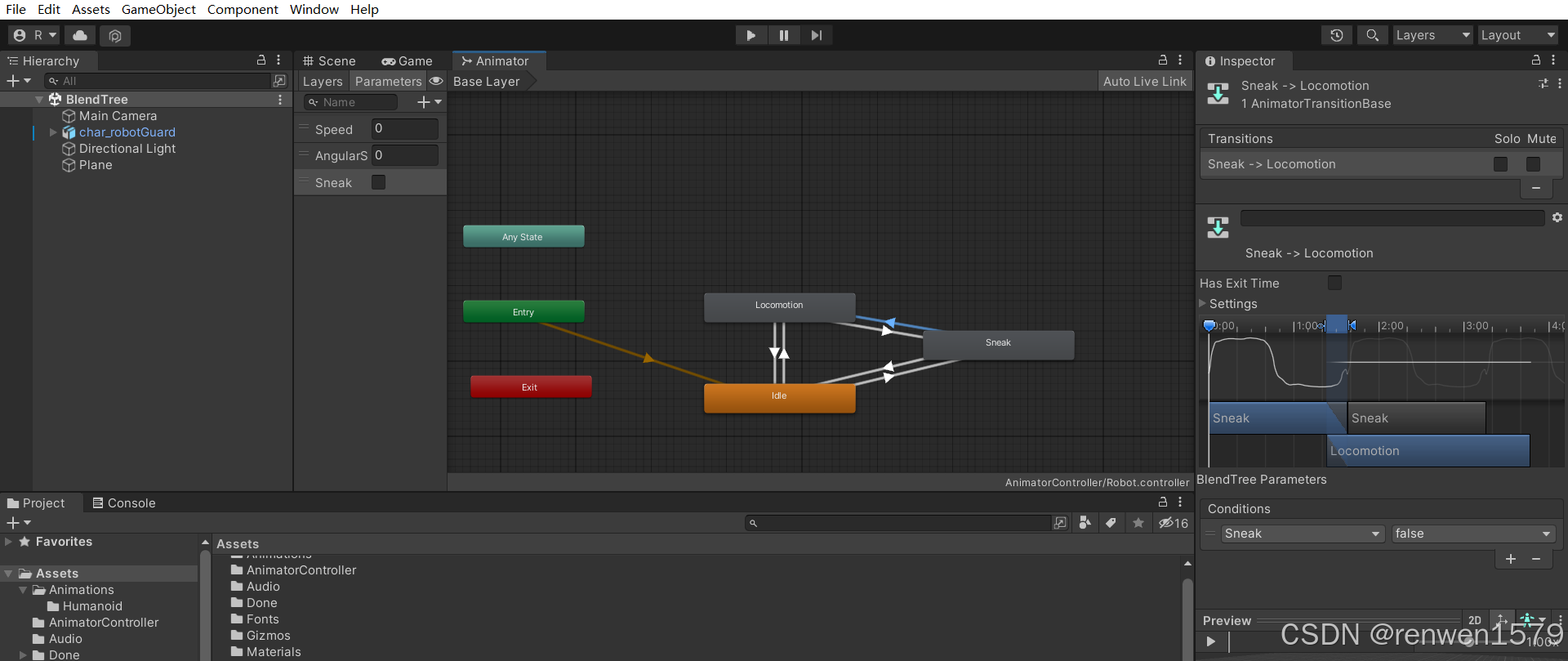
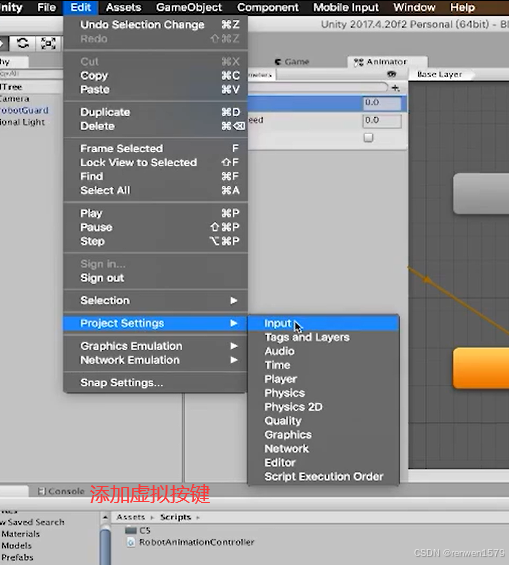
声音组件添加
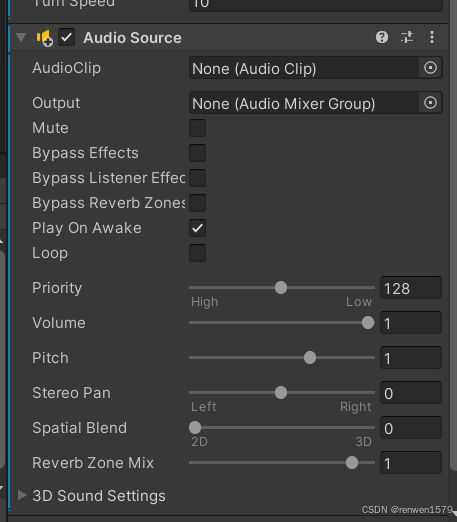
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RobotAnimationController : MonoBehaviour
{
Header("平滑过渡时间")
Range(0,3)
public float dampTime = 3f;
Header("移动速度")
public float speed = 3f;
Header("转身速度")
public float turnSpeed = 10f;
//虚拟轴
private float hor, ver;
//动画组件
private Animator ani;
//声音组件
private AudioSource aud;
//虚拟按键
private bool sneak;
private void Awake()
{
ani = GetComponent<Animator>();
aud = GetComponent<AudioSource>();
}
private void Update()
{
hor = Input.GetAxis("Horizontal");
ver = Input.GetAxis("Vertical");
//获取虚拟按键
sneak = Input.GetButton("Sneak");
//if (sneak) {
// ani.SetBool("Sneak", sneak);
//}
//设置动画参数
ani.SetBool("Sneak", sneak);
//只要按下一个方向键,就走
if (hor != 0 || ver != 0)
{
//设置Speed,角色动起来
ani.SetFloat("Speed", 5.6f, dampTime, Time.deltaTime);
//将向量转换成四元数
Quaternion targetQua = Quaternion.LookRotation(new Vector3(hor, 0, ver));
//lerp过去
transform.rotation = Quaternion.Lerp(transform.rotation, targetQua, Time.deltaTime * turnSpeed);
}
else {
//停下来
ani.SetFloat("Speed", 0f);
}
//判断当前是否是Locomotion状态
bool isLocomotion=ani.GetCurrentAnimatorStateInfo(0).IsName("Locomotion");
if (isLocomotion)
{
//判断脚步声音是不是已经开始播放了
if (!aud.isPlaying) {
//播放声音
aud.Play();
}
}
else {
//停止声音
aud.Stop();
}
}
}
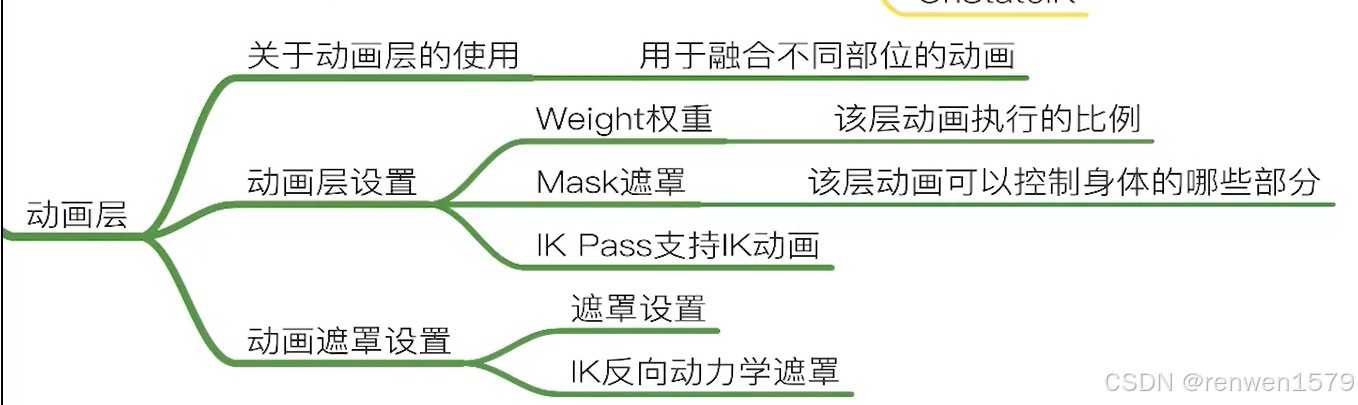
边走路边喊叫 动画分层的应用
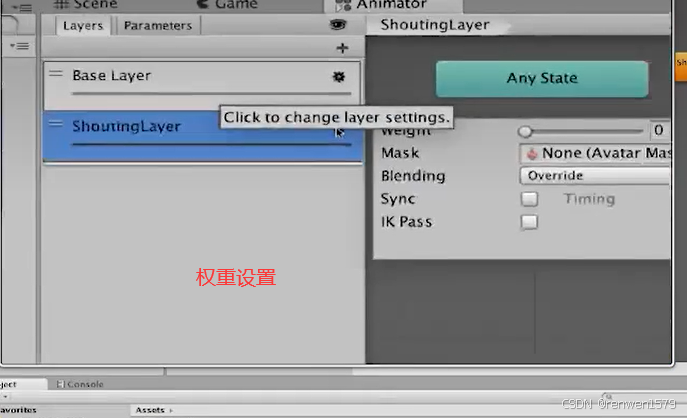
Mask
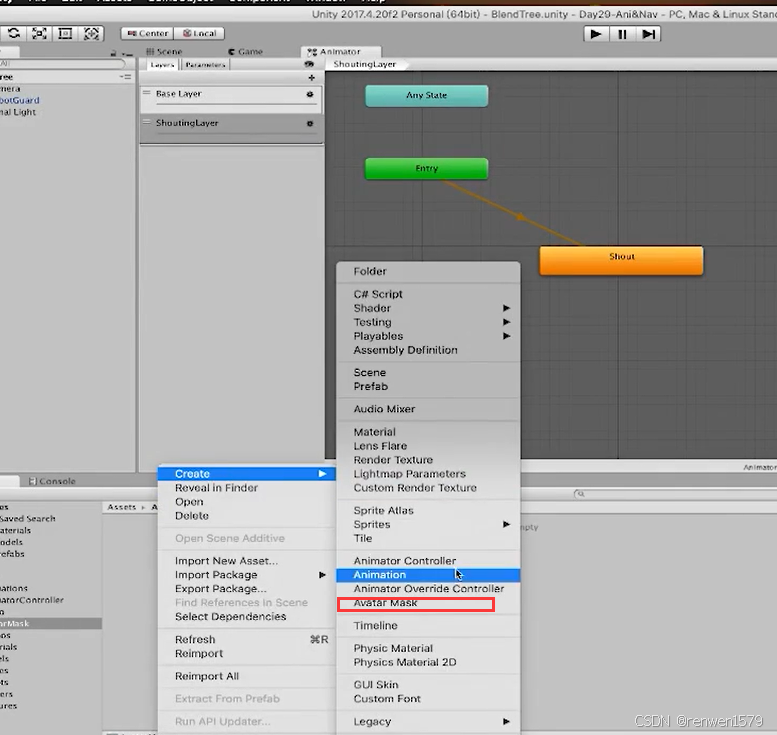
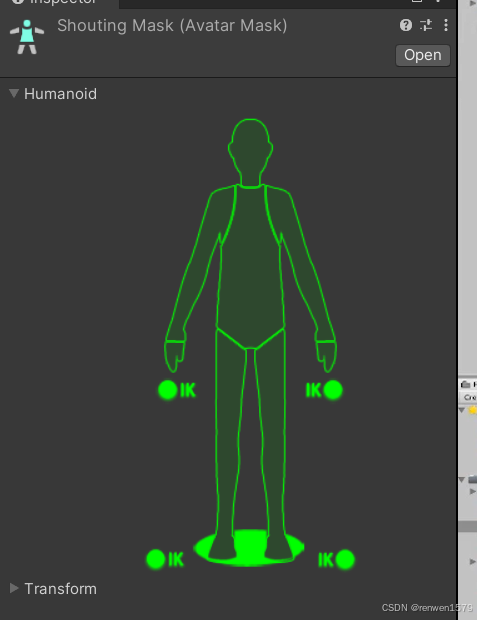
模型正对我们
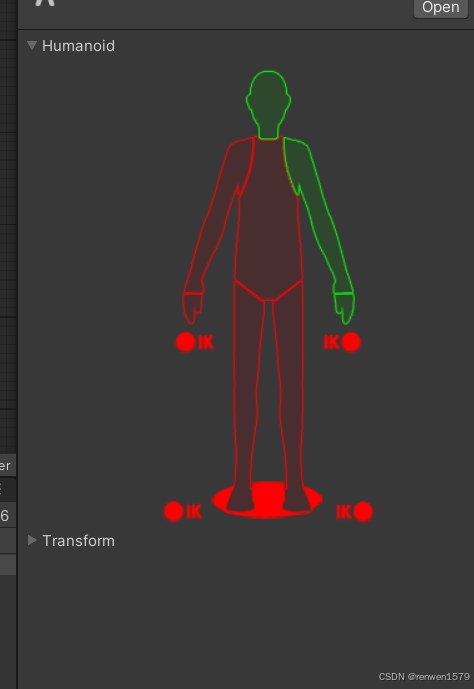
非人型遮罩------手动勾选
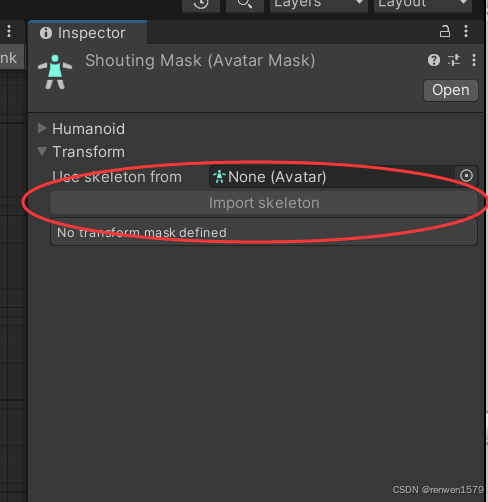
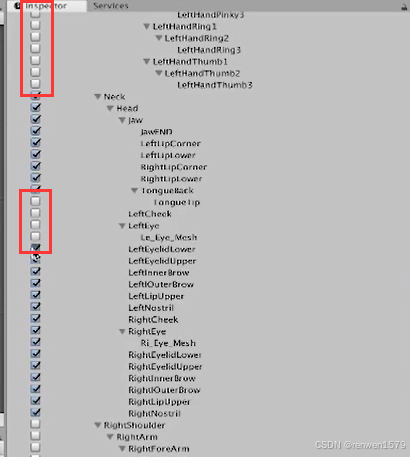
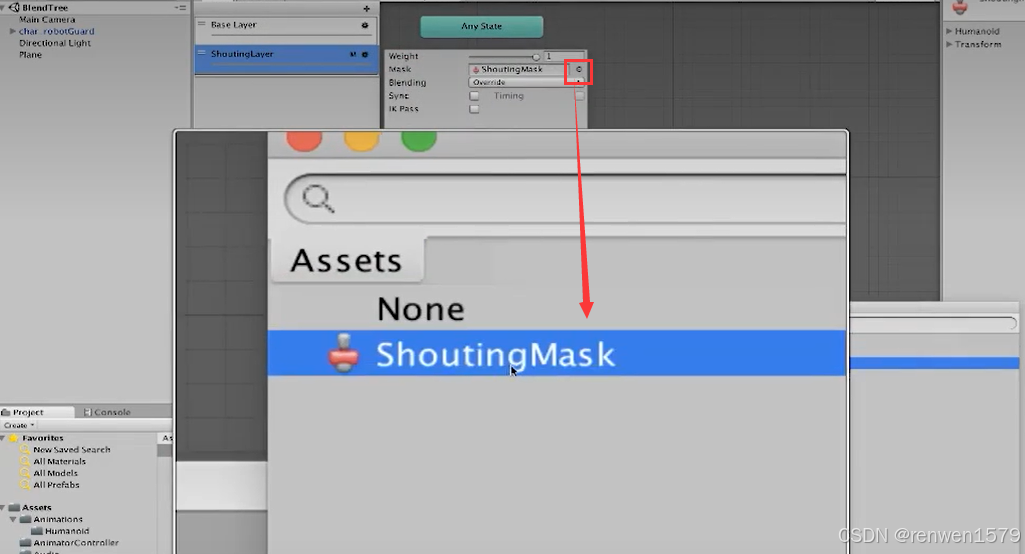
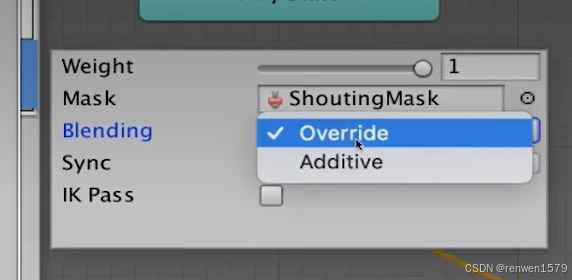
override覆盖上一层的动画
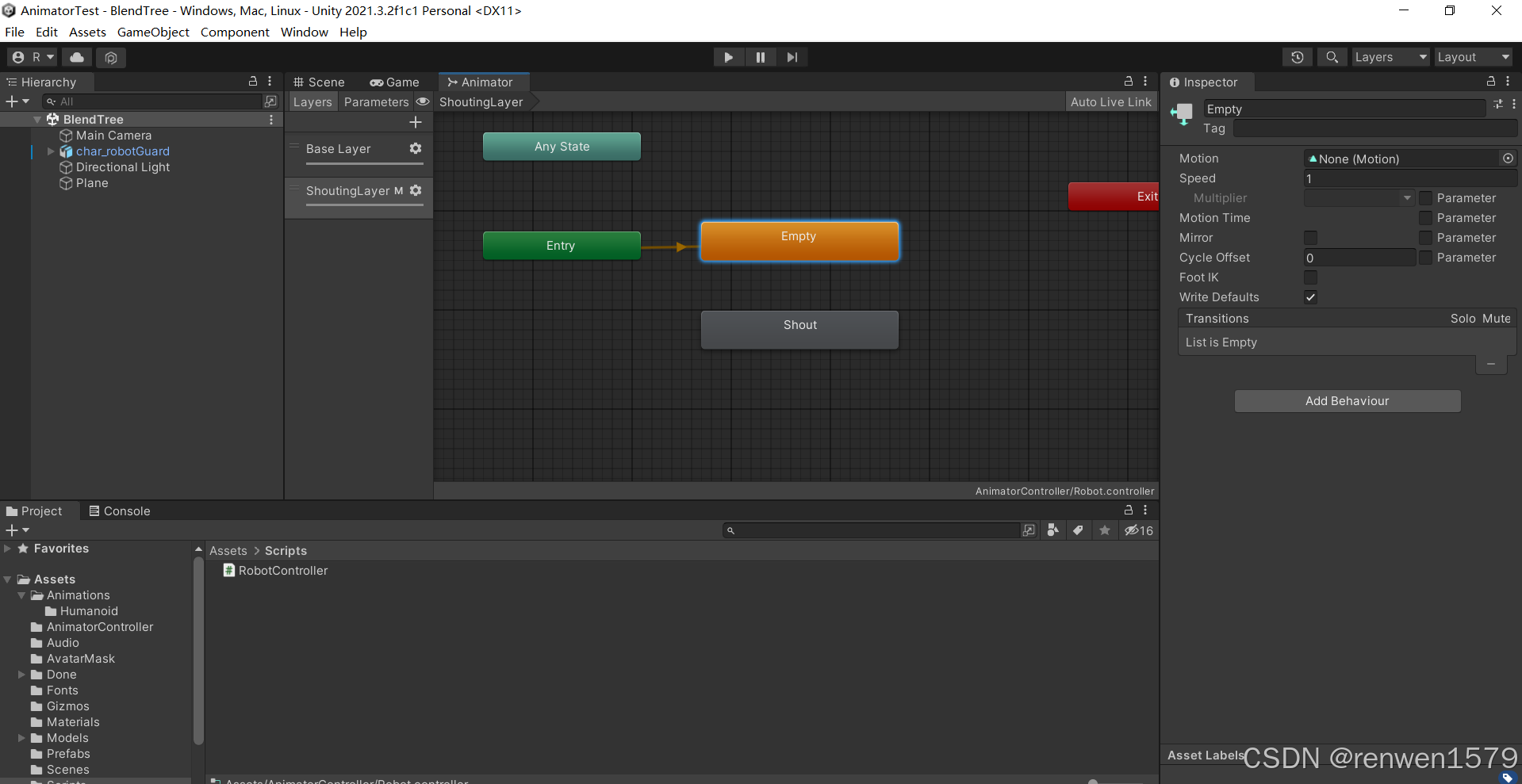
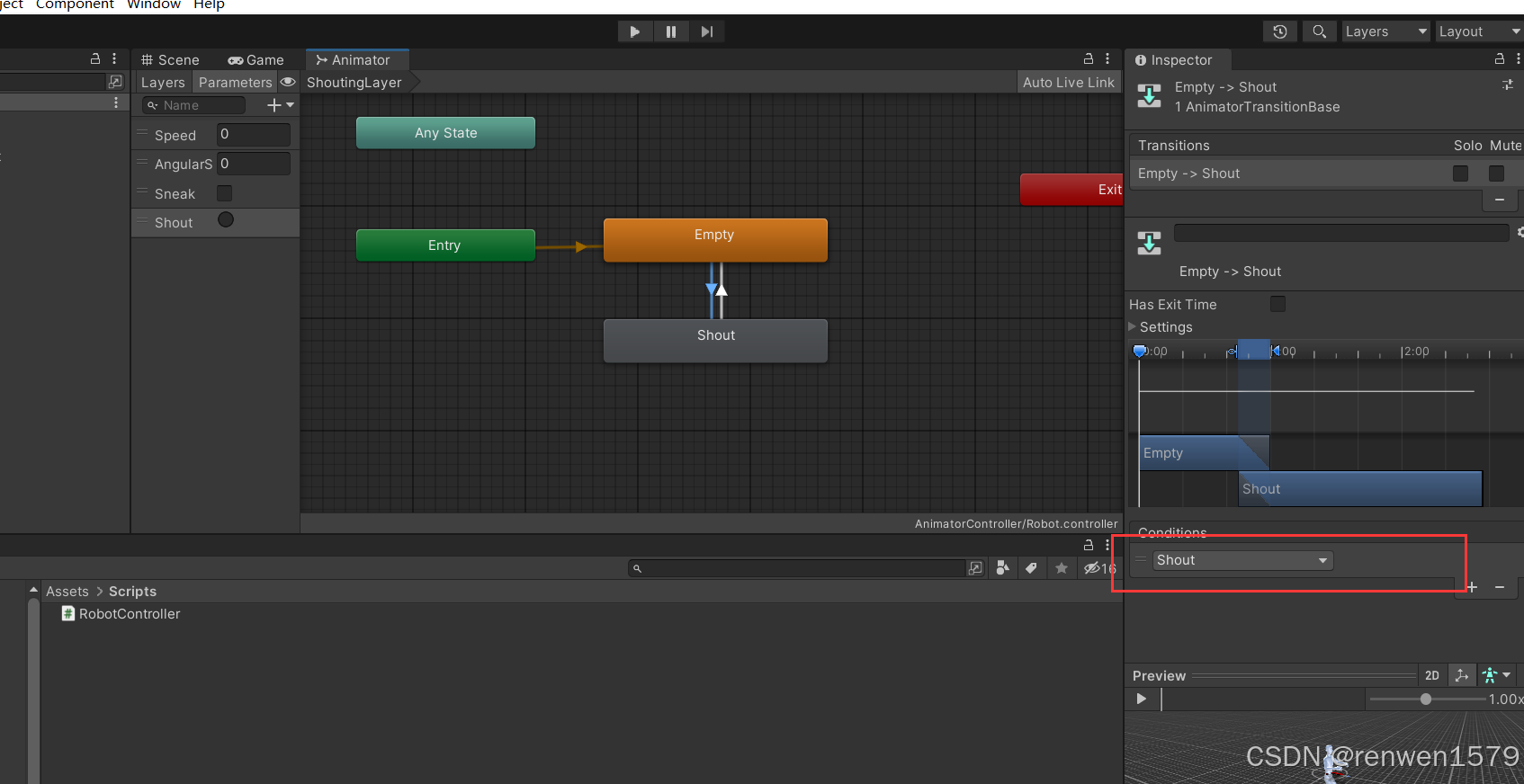
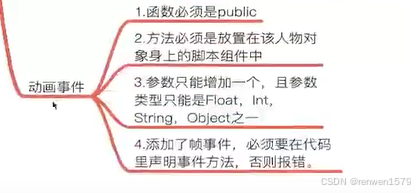
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RobotController : MonoBehaviour
{
Header("平滑过渡时间")
Range(0, 3)
public float dampTime = 3f;
Header("移动速度")
public float speed = 3f;
Header("转身速度")
public float turnSpeed = 10f;
//虚拟轴
private float hor, ver;
//动画组件
private Animator ani;
//声音组件
private AudioSource aud;
Header("喊叫声音片段")
public AudioClip shoutClip;
//虚拟按键
private bool sneak,shout;
private void Awake()
{
ani = GetComponent<Animator>();
aud = GetComponent<AudioSource>();
}
private void Update()
{
hor = Input.GetAxis("Horizontal");
ver = Input.GetAxis("Vertical");
//获取虚拟按键
sneak = Input.GetButton("Sneak");
shout = Input.GetButtonDown("Shout");
//if (sneak) {
// ani.SetBool("Sneak", sneak);
//}
//设置动画参数
ani.SetBool("Sneak", sneak);
if (shout) {
//触发参数shout
ani.SetTrigger("Shout");
//播放喊叫声音
AudioSource.PlayClipAtPoint(shoutClip,transform.position);
}
//只要按下一个方向键,就走
if (hor != 0 || ver != 0)
{
//设置Speed,角色动起来
ani.SetFloat("Speed", 5.6f, dampTime, Time.deltaTime);
//将向量转换成四元数
Quaternion targetQua = Quaternion.LookRotation(new Vector3(hor, 0, ver));
//lerp过去
transform.rotation = Quaternion.Lerp(transform.rotation, targetQua, Time.deltaTime * turnSpeed);
}
else
{
//停下来
ani.SetFloat("Speed", 0f);
}
//判断当前是否是Locomotion状态
bool isLocomotion = ani.GetCurrentAnimatorStateInfo(0).IsName("Locomotion");
if (isLocomotion)
{
//判断脚步声音是不是已经开始播放了
if (!aud.isPlaying)
{
//播放声音
aud.Play();
}
}
else
{
//停止声音
aud.Stop();
}
}
}
AudioListener挂载在角色身上
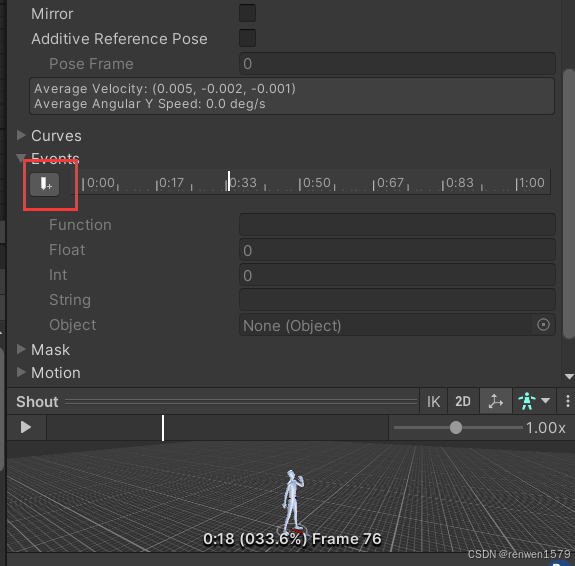
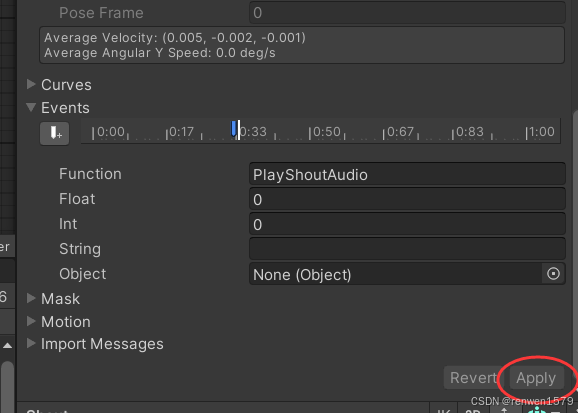
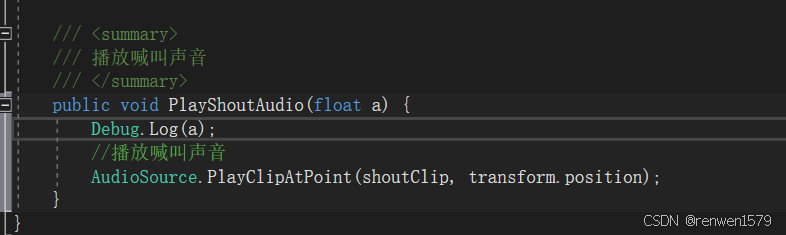
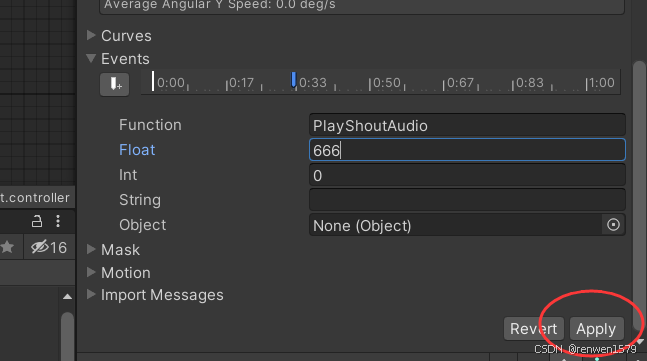
参数不能超过1个
IK方向动力学
Inverse kinematics
反应的是一种由手部带到肩部的运动形式,在这个运动中,运动以手部这个自由端为起始,当手部进行运动时会自然的带动固定端肩部的运动。
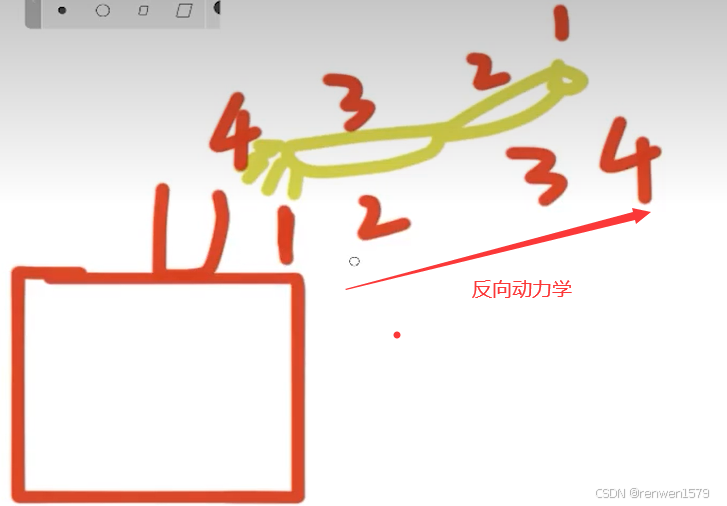
比如别人拉起你的手
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RobotController : MonoBehaviour
{
Header("平滑过渡时间")
Range(0, 3)
public float dampTime = 3f;
Header("移动速度")
public float speed = 3f;
Header("转身速度")
public float turnSpeed = 10f;
//虚拟轴
private float hor, ver;
//动画组件
private Animator ani;
//声音组件
private AudioSource aud;
Header("喊叫声音片段")
public AudioClip shoutClip;
//虚拟按键
private bool sneak,shout;
private void Awake()
{
ani = GetComponent<Animator>();
aud = GetComponent<AudioSource>();
}
private void Update()
{
hor = Input.GetAxis("Horizontal");
ver = Input.GetAxis("Vertical");
//获取虚拟按键
sneak = Input.GetButton("Sneak");
shout = Input.GetButtonDown("Shout");
//if (sneak) {
// ani.SetBool("Sneak", sneak);
//}
//设置动画参数
ani.SetBool("Sneak", sneak);
if (shout) {
//触发参数shout
ani.SetTrigger("Shout");
}
//只要按下一个方向键,就走
if (hor != 0 || ver != 0)
{
//设置Speed,角色动起来
ani.SetFloat("Speed", 5.6f, dampTime, Time.deltaTime);
//将向量转换成四元数
Quaternion targetQua = Quaternion.LookRotation(new Vector3(hor, 0, ver));
//lerp过去
transform.rotation = Quaternion.Lerp(transform.rotation, targetQua, Time.deltaTime * turnSpeed);
}
else
{
//停下来
ani.SetFloat("Speed", 0f);
}
//判断当前是否是Locomotion状态
bool isLocomotion = ani.GetCurrentAnimatorStateInfo(0).IsName("Locomotion");
if (isLocomotion)
{
//判断脚步声音是不是已经开始播放了
if (!aud.isPlaying)
{
//播放声音
aud.Play();
}
}
else
{
//停止声音
aud.Stop();
}
}
/// <summary>
/// 播放喊叫声音
/// </summary>
public void PlayShoutAudio(float a) {
Debug.Log(a);
//播放喊叫声音
AudioSource.PlayClipAtPoint(shoutClip, transform.position);
}
}
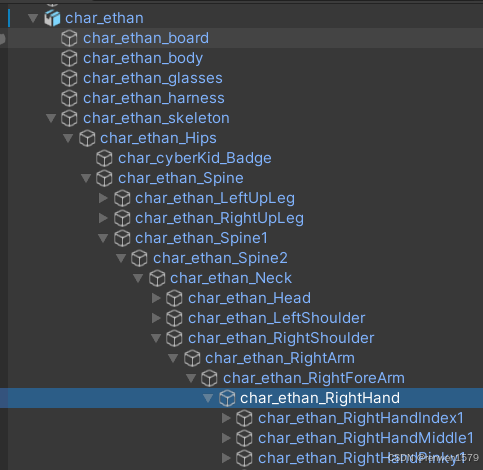
找到关节
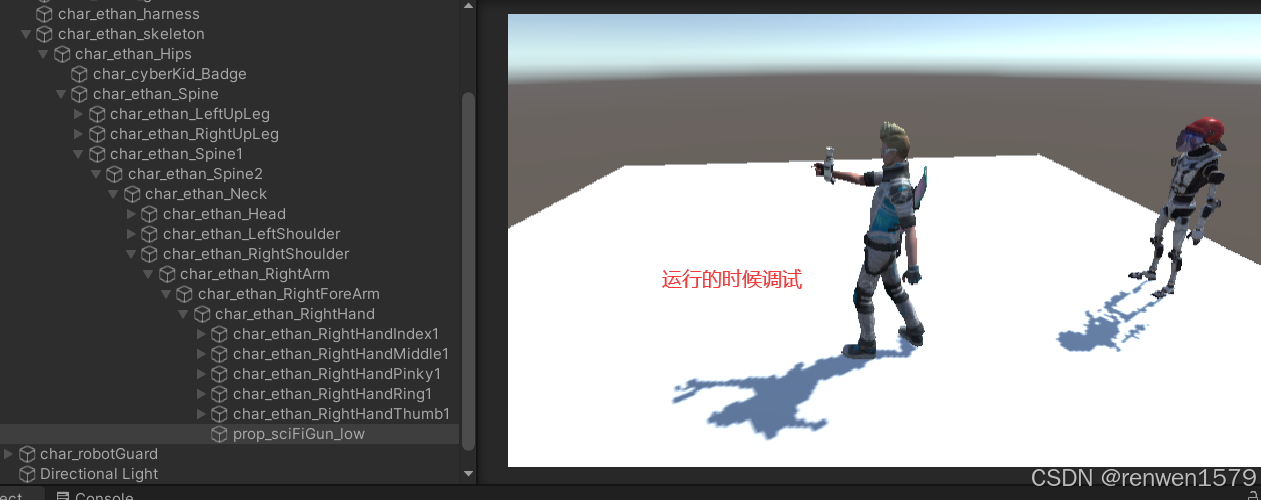
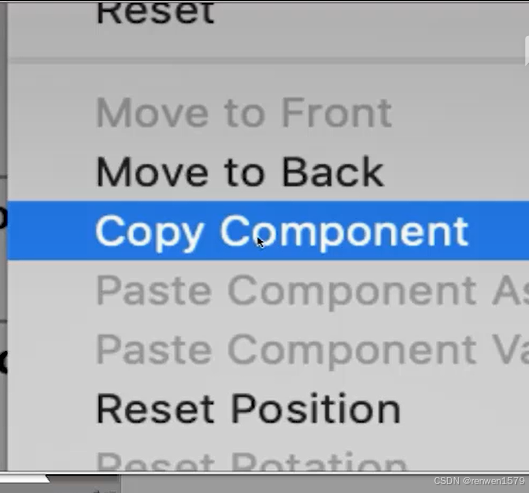
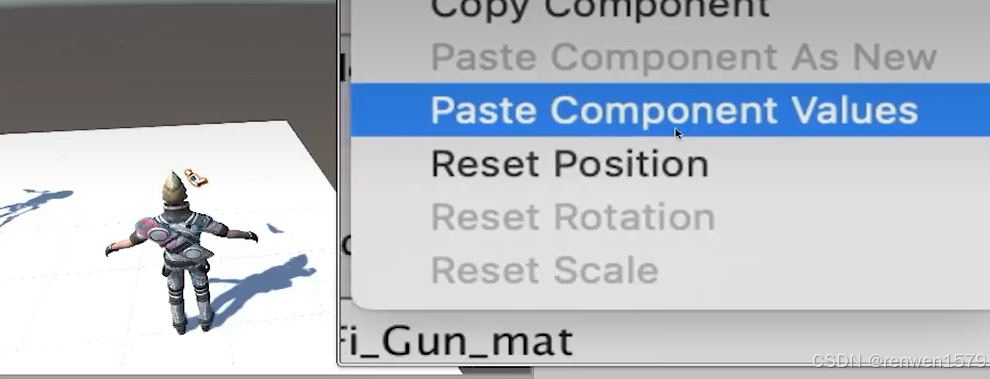
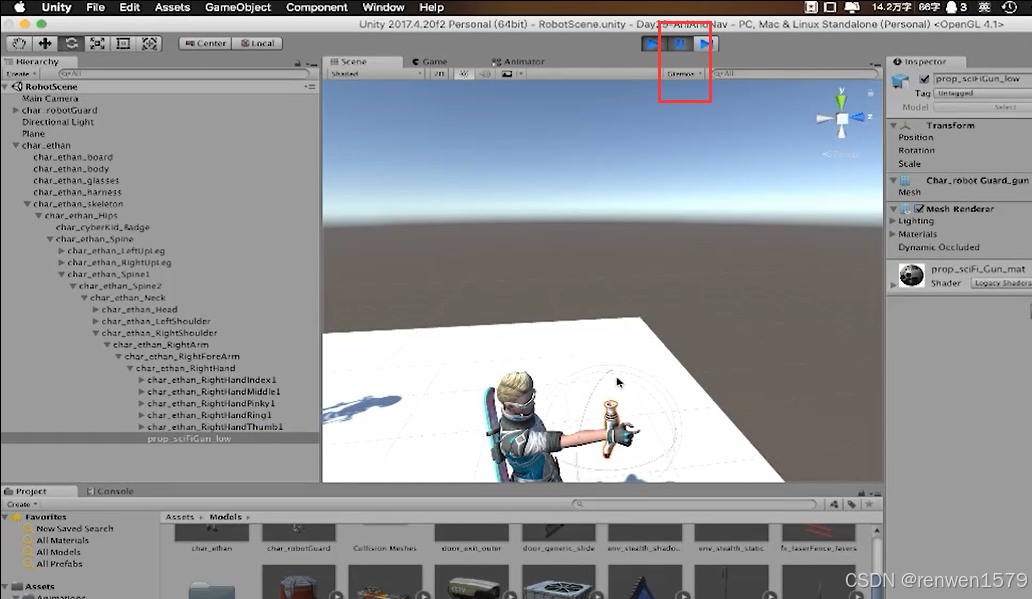
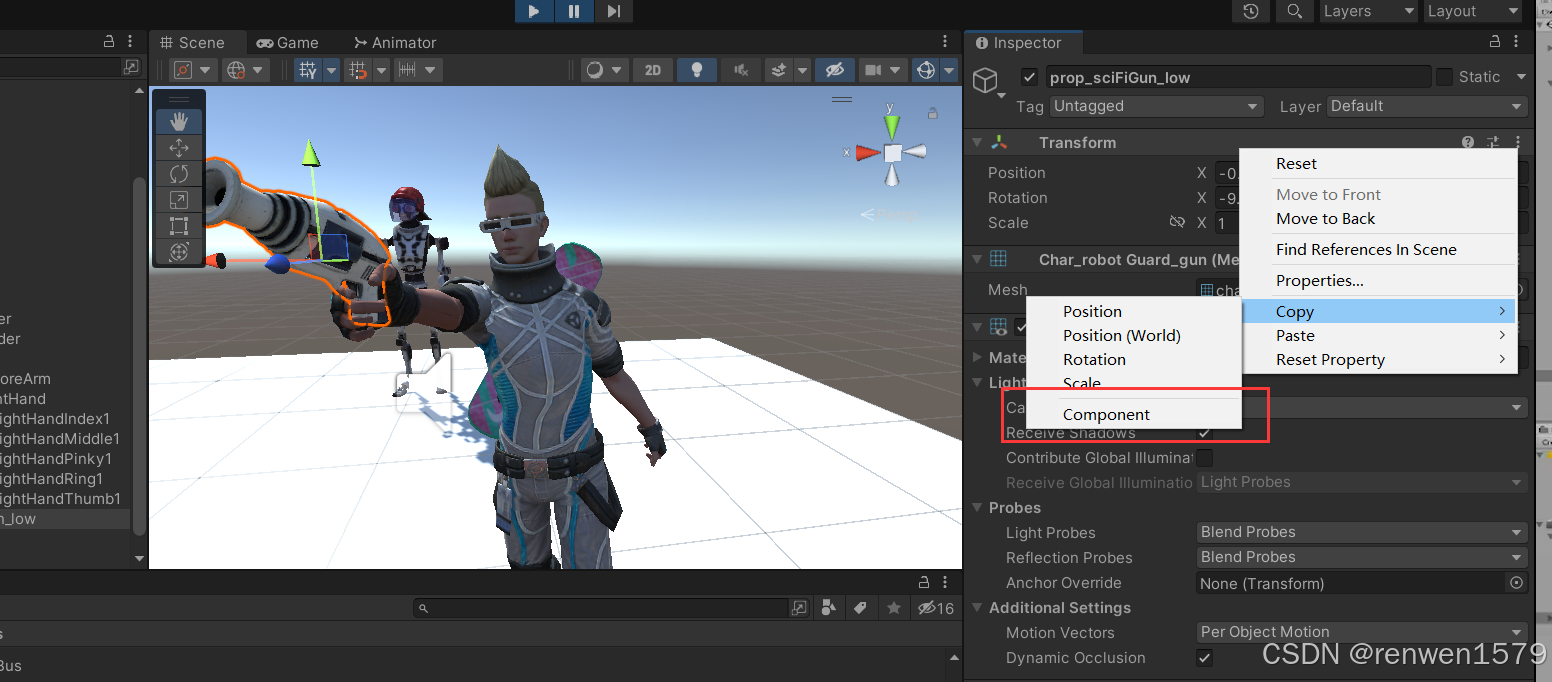
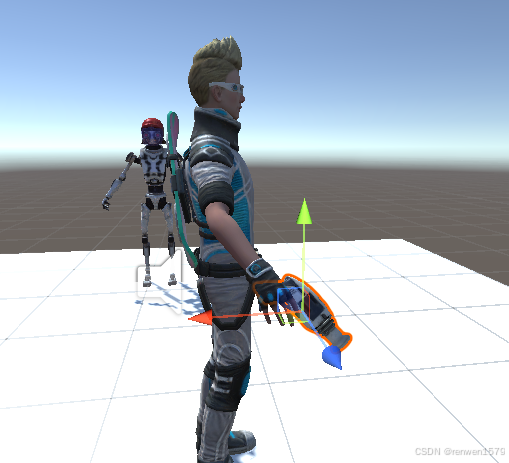
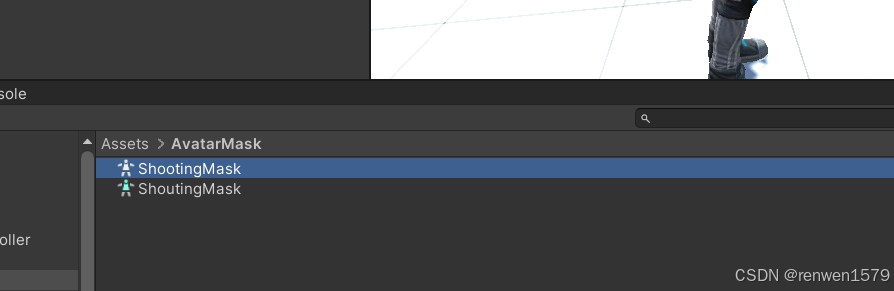
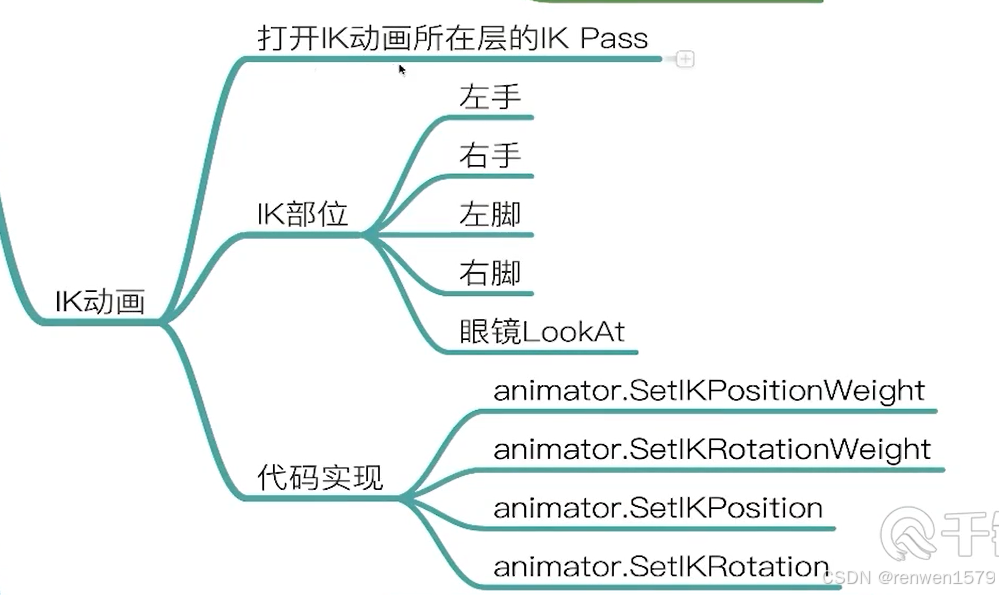
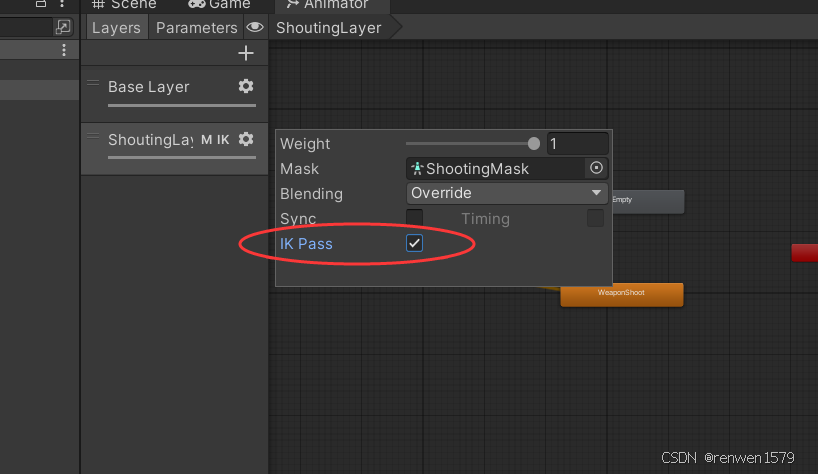
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EthanIKController : MonoBehaviour
{
private Animator ani;
Header("IK动画的开关")
public bool ikActive = false;
Header("射击敌人的最大角度")
public float fireMaxAngle = 100;
Header("IK指向的目标")
public Transform target;
Header("IK指向的目标身高")
public float targetHeight = 1.8f;
private void Awake()
{
ani = GetComponent<Animator>();
}
private void OnAnimatorIK(int layerIndex)
{
//获取玩家指向敌人的方向向量
Vector3 dir=target.position - transform.position;
//计算夹角
float angle=Vector3.Angle(dir, transform.forward);
if (angle < fireMaxAngle / 2)
{
ikActive = true;
}
else {
ikActive = false;
}
if (ikActive)
{
//设置IK权重
ani.SetIKPositionWeight(AvatarIKGoal.RightHand, 1);
//设置IK位置
ani.SetIKPosition(AvatarIKGoal.RightHand, target.position+Vector3.up*targetHeight);
//设置眼睛的IK权重
ani.SetLookAtWeight(1);
//设置眼睛的IK位置
ani.SetLookAtPosition(target.position+Vector3.up * targetHeight);
}
else {
//设置IK权重为0
ani.SetIKPositionWeight(AvatarIKGoal.RightHand, 0);
//设置眼睛权重为0
ani.SetLookAtWeight(0);
}
}
}
Curve
p341
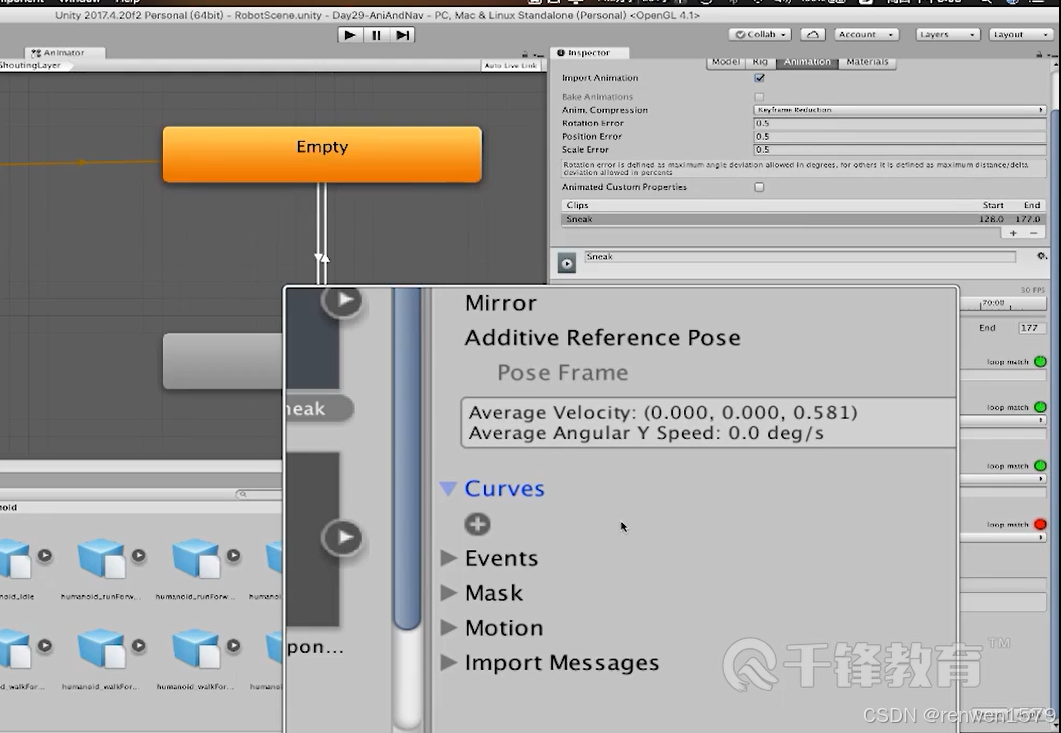
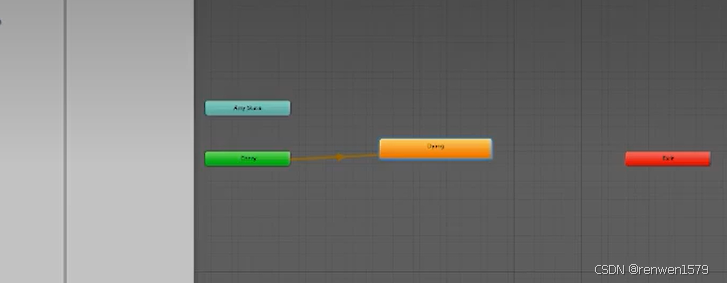
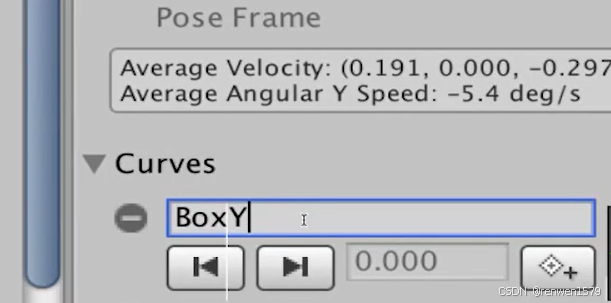
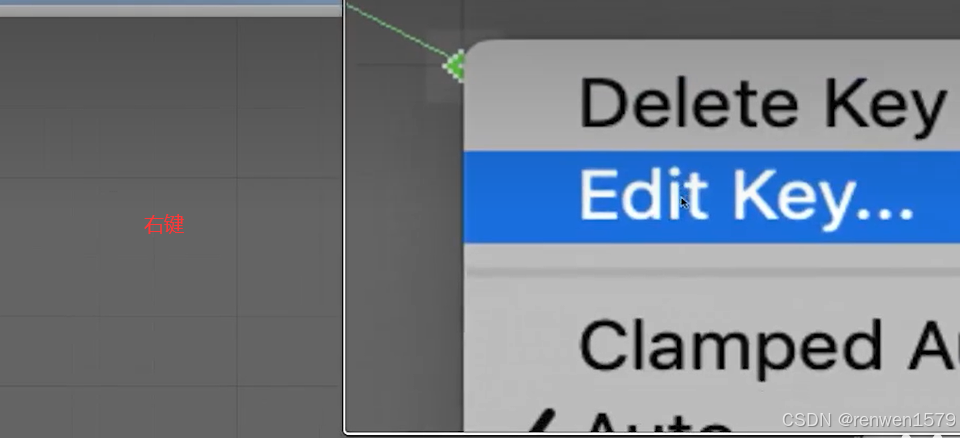
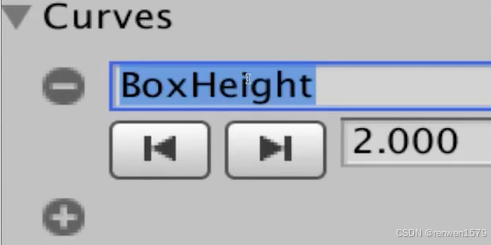
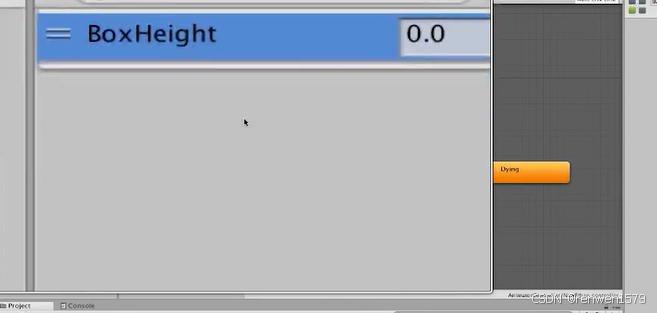
绑定到一起只需要名称一致
p342
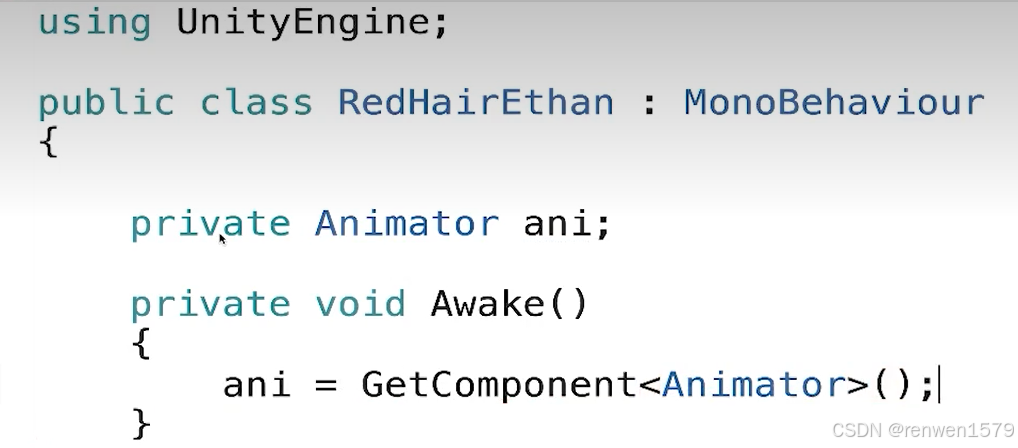
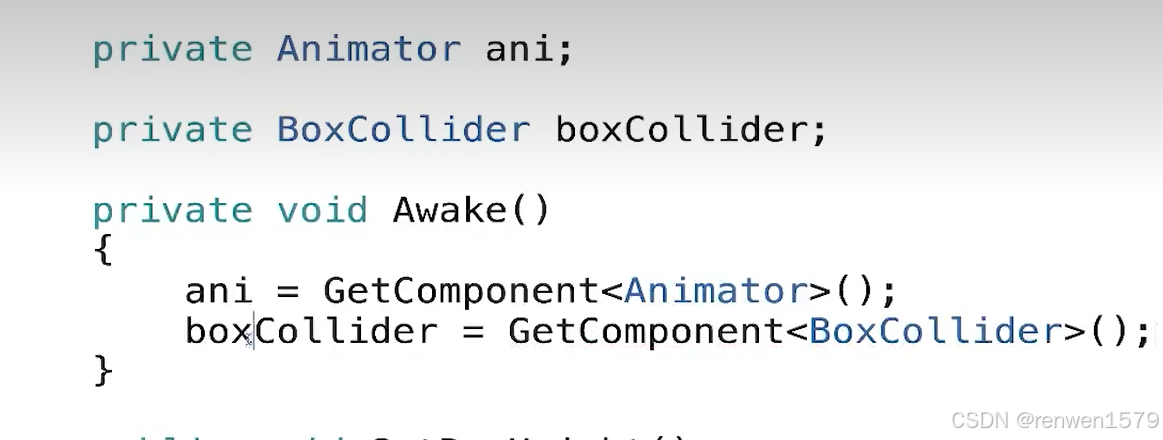
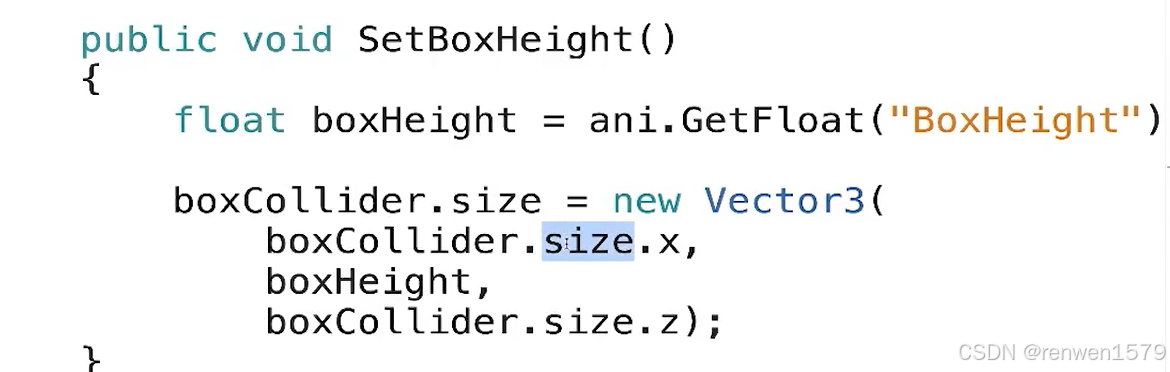
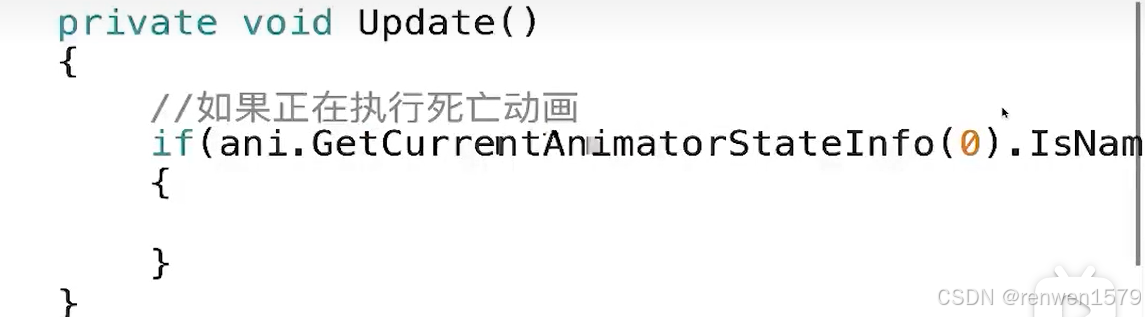
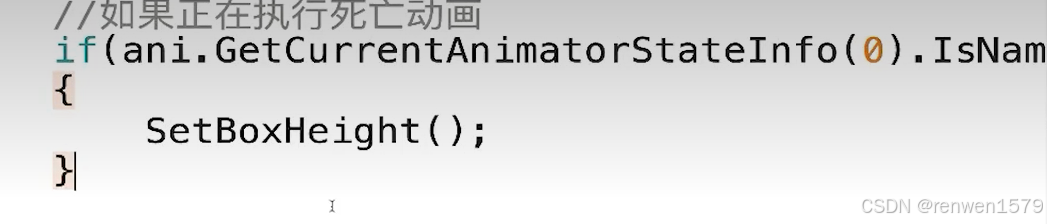
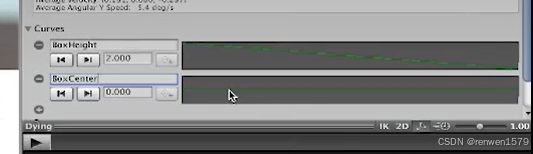
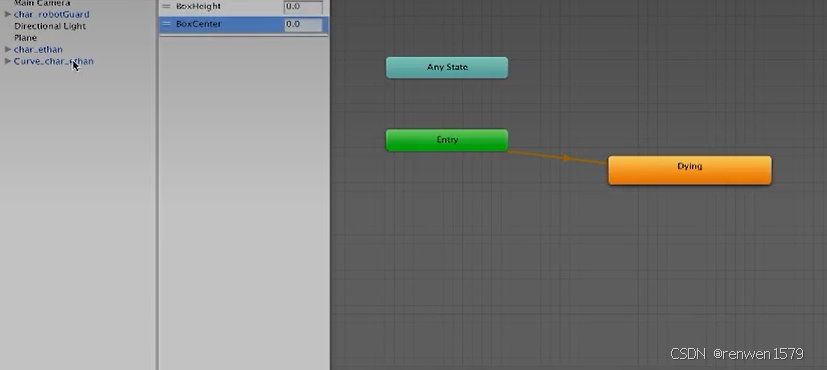

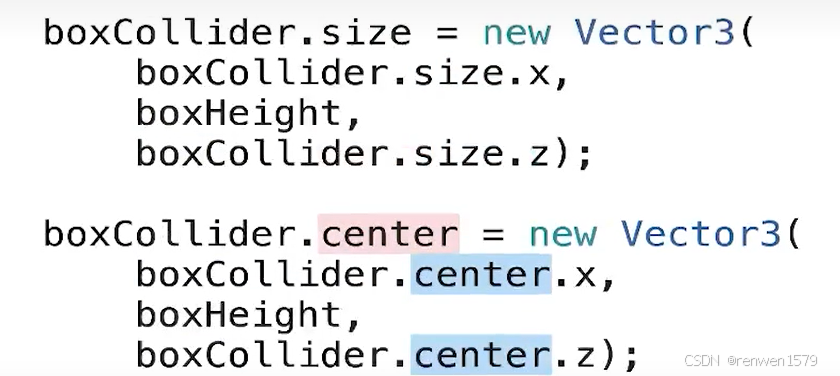
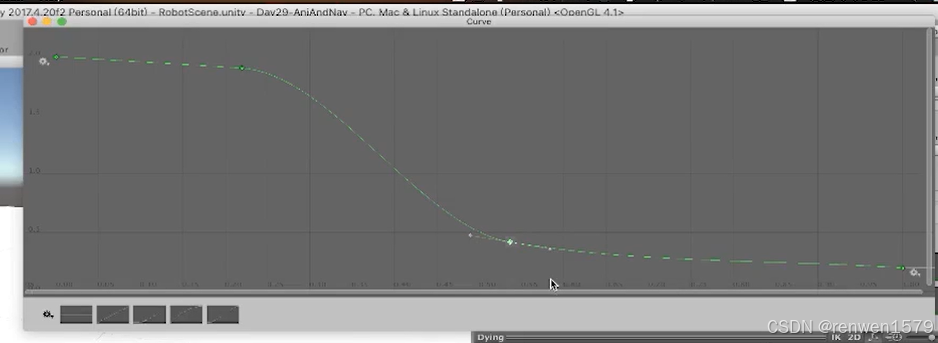
开始慢后期快
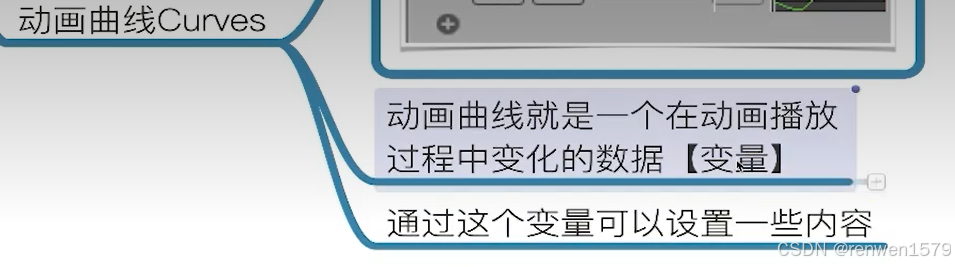