Microsoft OneNote是一款功能强大的笔记工具。学习如何使用 Java 更改样式,将您的 OneNote 体验提升到一个新的水平!在本指南中,我们将向您展示如何使用 Java 更改 OneNote 文档中的样式以增强可读性。本指南将为您提供分步说明,以实施样式更改和代码片段,从而提高您的工作效率。
**Aspose.Note**是一款处理Microsoft Office OneNote文件的类库。它允许开发人员在C#、VB.NET、ASP.NET web应用、web服务和Windows应用中中处理.one文件。它能够打开文件并操作OneNote元素,从文本、图像和属性到更多复杂元素,然后到处PNG、GIF、JPEG、BMP或PDF格式。
Aspose.Note 最新下载(qun:666790229)https://www.evget.com/product/3418/download
Java OneNote API 用于更改 OneNote 中的样式
Aspose.Note for Java OneNote API 提供了一种强大的方式以编程方式与 OneNote 文档进行交互。它允许开发人员自动执行任务、创建自定义工具以及将 OneNote 与其他 Java 应用程序集成。要使用 Java 更改 OneNote 中的样式,我们将使用 Aspose.Note for Java OneNote API 来访问和修改笔记的格式。
请下载API 的JAR或将以下pom.xml配置添加到基于 Maven 的 Java 应用程序。
<repository> <id>AsposeJavaAPI</id> <name>Aspose Java API</name> <url>https://releases.aspose.com/java/repo/</url> </repository> <dependency> <groupId>com.aspose</groupId> <artifactId>aspose-note</artifactId> <version>24.4</version> <classifier>jdk17</classifier> </dependency>
使用 Java 创建带有文本样式的 OneNote 页面标题
我们可以按照以下步骤以编程方式在 OneNote 文档中创建页面标题:
- 使用Document类创建一个新的 OneNote 文档。
- 使用Page类添加新页面。
- 使用RichText类指定标题文本、日期和时间。
- 设置RichText 类对象的ParagraphStyle属性来定义其字体名称,大小,颜色等。
- **最后,使用save()**方法保存文档。
以下代码示例显示如何使用 Java 在 OneNote 文档中创建带有样式的页面标题。
// initialize new Document Document doc = new Document(); // initialize new Page Page page = new Page(); // title text RichText titleText = new RichText().append("Title text."); // title text style ParagraphStyle titleTextStyle = new ParagraphStyle(); titleTextStyle.setFontName("Courier New"); titleTextStyle.setFontSize(20); // set title text style titleText.setParagraphStyle(titleTextStyle); // title date RichText titleDate = new RichText().append("Friday, 11 November 2011"); titleDate.setParagraphStyle(ParagraphStyle.getDefault()); // title time RichText titleTime = new RichText().append("12:34"); titleTime.setParagraphStyle(ParagraphStyle.getDefault()); Title title = new Title(); title.setTitleText(titleText); title.setTitleDate(titleDate); title.setTitleTime(titleTime); page.setTitle(title); // append page node doc.appendChildLast(page); // save the document doc.save("CreatePageTitle.one");
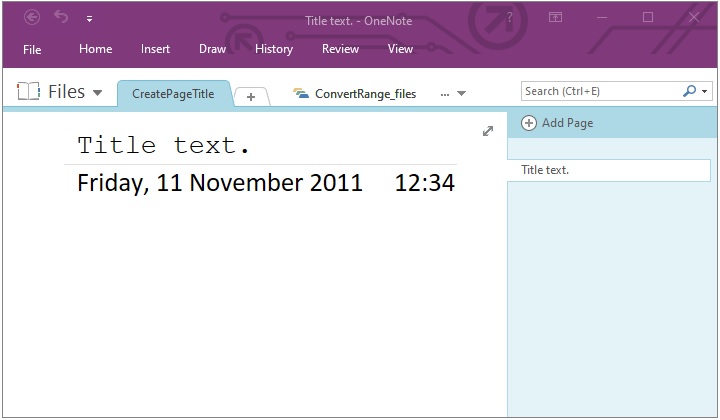
使用 Java 更改页面标题的文本样式
我们还可以按照以下步骤更改 OneNote 文档中页面标题的文本样式:
- 使用Document类加载现有的 OneNote 文档。
- 循环遍历文档中的所有页面标题。
- 修改每个标题的ParagraphStyle属性。
- 或者,修改每个标题的TextRuns 的Style属性。
- **最后,使用save()**方法保存文档。
以下代码示例显示如何使用 Java 更改 OneNote 文档中页面标题的文本样式。
// Load the document into Aspose.Note. Document document = new Document("CreatePageTitle.one"); // Change the style for (Title title : (Iterable<Title>) document.getChildNodes(Title.class)) { // Modify title paragraph style title.getTitleText().getParagraphStyle().setFontSize(38); title.getTitleText().getParagraphStyle().setBold(true); title.getTitleText().getParagraphStyle().setFontColor(Color.BLUE); // Alternatively modify text run style within the title for (TextRun richText : title.getTitleText().getTextRuns()) { richText.getStyle().setFontSize(50); richText.getStyle().setBold(true); richText.getStyle().setFontColor(Color.BLUE); } } // Save the document document.save("PageTitle.one");
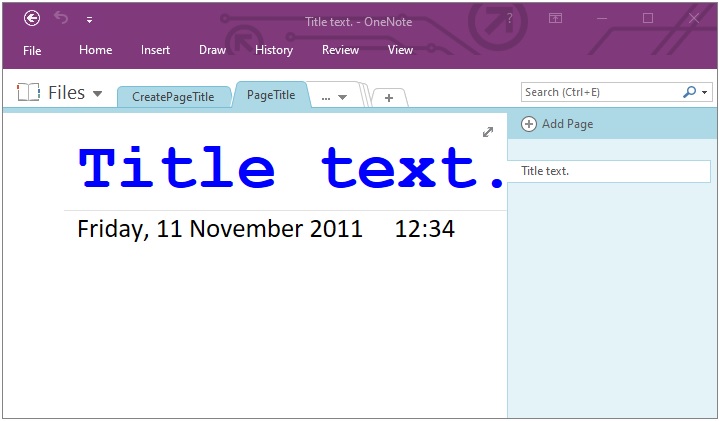
使用 Java 更改 OneNote 段落的文本样式
我们可以按照以下步骤更改 OneNote 文档中段落的文本样式:
- 使用Document类加载 OneNote 文档。
- 使用GgtChildNodes() 方法获取特定或所有RichText节点。
- 修改RichText 节点的TextRuns的 Style属性,例如 FontColor、Highlight、FontSize 等。
- **最后,使用save()**方法保存文档。
以下代码示例展示如何使用 Java 更改 OneNote 文档中段落的文本样式。
// Load the document into Aspose.Note. Document document = new Document("D:\\Files\\Aspose.one"); // Get all the pages List<Page> pages = document.getChildNodes(Page.class); // Get a particular RichText node(s) List<RichText> richTextNodes = pages.get(3).getChildNodes(RichText.class); if (richTextNodes != null && richTextNodes.size() > 3) { for (int i = 3; i < richTextNodes.size(); i++) { RichText richText = richTextNodes.get(i); // Apply formatting style for (TextRun run : richText.getTextRuns()) { // Set font color run.getStyle().setFontColor(Color.YELLOW); // Set highlight color run.getStyle().setHighlight(Color.BLUE); // Set font size run.getStyle().setFontSize(14); } } } // Save the document document.save("D:\\Files\\ParagraphStyle.one");
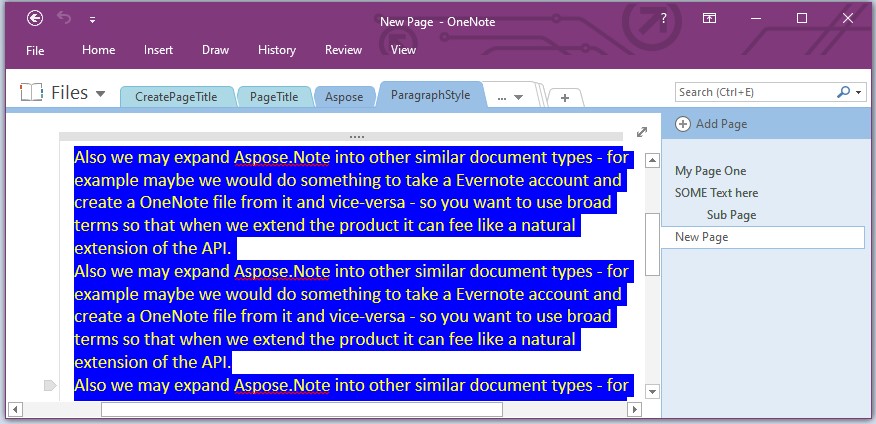
使用 Java 在 OneNote 中设置默认段落样式
我们还可以按照以下步骤在 OneNote 文档中设置默认段落样式:
- 使用Document类创建一个新文档。
- 使用Page类创建一个新页面。
- 初始化Outline 和OutlineElement类对象。
- 创建一个RichText 类对象并指定ParagraphStyle。
- 之后,附加子元素。
- **最后,使用save()**方法保存文档。
下面的代码示例展示如何使用 Java 设置 OneNote 文档中段落的默认段落样式。
// Create a new document Document document = new Document(); // Create a new page Page page = new Page(); // Create a new outline Outline outline = new Outline(); // Create an outline element OutlineElement outlineElem = new OutlineElement(); // Create style ParagraphStyle defaultStyle = new ParagraphStyle() .setFontName("Courier New") .setFontSize(20); RichText text = new RichText() .append("DefaultParagraphFontAndSize") .append(System.lineSeparator()) .append("OnlyDefaultParagraphFont", new TextStyle().setFontSize(14)) .append(System.lineSeparator()) .append("OnlyDefaultParagraphFontSize", new TextStyle().setFontName("Verdana")); text.setParagraphStyle(defaultStyle); // Append elements outlineElem.appendChildLast(text); outline.appendChildLast(outlineElem); page.appendChildLast(outline); document.appendChildLast(page); // Save the document document.save("SetDefaultParagraphStyle.one");
结论
在本文中,我们探讨了如何使用 Java 更改 OneNote 文档中页面标题或段落的文本样式。通过利用Aspose.Note for Java OneNote API,您可以轻松地将此类功能集成到 Java 应用程序中。那么,让我们深入研究并开始自定义 OneNote 以更好地满足您的需求!