点一下关注吧!!!非常感谢!!持续更新!!!
目前已经更新到了:
- Hadoop
- HDFS
- MapReduce
- Hive
- Flume
- Sqoop
- Zookeeper
- HBase 正在···
章节内容
上一节我们完成了:
- HBase Shell 的使用
- HBase 增、删、改、查等操作
- HBase列族相关的操作
背景介绍
这里是三台公网云服务器,每台 2C4G,搭建一个Hadoop的学习环境,供我学习。
之前已经在 VM 虚拟机上搭建过一次,但是没留下笔记,这次趁着前几天薅羊毛的3台机器,赶紧尝试在公网上搭建体验一下。
- 2C4G 编号 h121
- 2C4G 编号 h122
- 2C2G 编号 h123
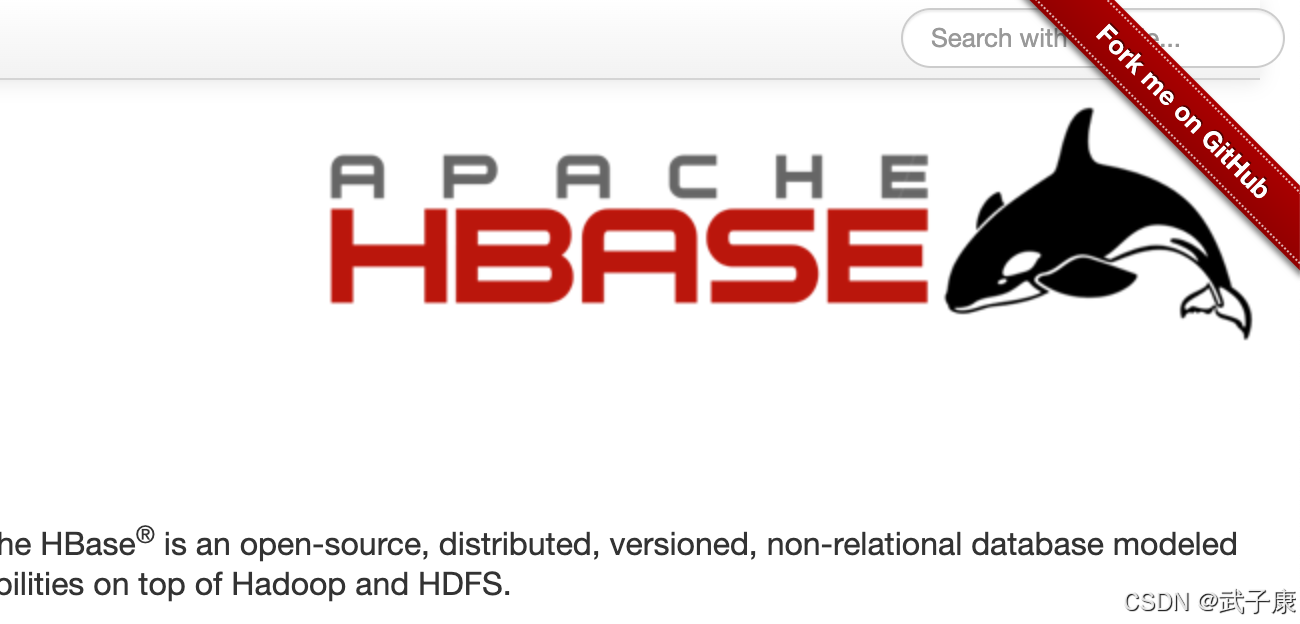
新建工程
新建一个Maven工程,这里就跳过了,不重复描述了。
POM
更新我们的POM文档,加入如下的依赖:
xml
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>1.3.1</version>
</dependency>
建立新表
java
public class Test01 {
public static void main(String[] args) throws IOException {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "h121.wzk.icu,h122.wzk.icu");
configuration.set("hbase.zookeeper.property.clientPort", "2181");
Connection connection = ConnectionFactory.createConnection(configuration);
HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
// 创建表描述器
HTableDescriptor descriptor = new HTableDescriptor(TableName.valueOf("test01"));
// 设置列族描述器
descriptor.addFamily(new HColumnDescriptor("base_info"));
// 建立表
admin.createTable(descriptor);
System.out.println("test01 表建立完毕");
admin.close();
connection.close();
}
}
运行上面的代码,可以得到如下的结果:
插入数据
java
public class Test02 {
public static void main(String[] args) throws IOException {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "h121.wzk.icu,h122.wzk.icu");
configuration.set("hbase.zookeeper.property.clientPort", "2181");
Connection connection = ConnectionFactory.createConnection(configuration);
HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
// 插入数据
Table table = connection.getTable(TableName.valueOf("test01"));
// 设定 row key
Put put = new Put(Bytes.toBytes("rk1"));
// 列族 列 值
put.addColumn(Bytes.toBytes("base_info"), Bytes.toBytes("name"), Bytes.toBytes("wuzikang"));
// 执行插入
table.put(put);
table.close();
System.out.println("rk1 base_info:name wuzikang 数据插入成功!");
admin.close();
connection.close();
}
}
运行可以获得如下的结果:
删除数据
java
public class Test03 {
public static void main(String[] args) throws IOException {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "h121.wzk.icu,h122.wzk.icu");
configuration.set("hbase.zookeeper.property.clientPort", "2181");
Connection connection = ConnectionFactory.createConnection(configuration);
HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
// 删除数据
Table table02 = connection.getTable(TableName.valueOf("test01"));
Delete delete = new Delete(Bytes.toBytes("rk1"));
table02.delete(delete);
table02.close();
System.out.println("rk1 数据删除成功!");
admin.close();
connection.close();
}
}
运行可以获得如下的结果:
获取列族
java
public class Test04 {
public static void main(String[] args) throws IOException {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "h121.wzk.icu,h122.wzk.icu");
configuration.set("hbase.zookeeper.property.clientPort", "2181");
Connection connection = ConnectionFactory.createConnection(configuration);
HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
// 获取某个列族信息
HTable table = (HTable) connection.getTable(TableName.valueOf("test01"));
Get get = new Get(Bytes.toBytes("rk1"));
get.addFamily(Bytes.toBytes("base_info"));
// 执行查询
Result result = table.get(get);
Cell[] cells = result.rawCells();
for (Cell cell : cells) {
String cf = Bytes.toString(CellUtil.cloneFamily(cell));
String column = Bytes.toString(CellUtil.cloneQualifier(cell));
String value = Bytes.toString(CellUtil.cloneValue(cell));
String rowKey = Bytes.toString(CellUtil.cloneRow(cell));
System.out.println("rowKey: " + rowKey + ", " + cf + ", " + column + ", " + value);
}
table.close();
admin.close();
connection.close();
}
}
运行可以获得如下的结果:
扫描全表
java
public class Test05 {
public static void main(String[] args) throws IOException {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "h121.wzk.icu,h122.wzk.icu");
configuration.set("hbase.zookeeper.property.clientPort", "2181");
Connection connection = ConnectionFactory.createConnection(configuration);
HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
HTable table = (HTable) connection.getTable(TableName.valueOf("test01"));
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
Cell[] cells = result.rawCells();
for (Cell cell : cells) {
String cf = Bytes.toString(CellUtil.cloneFamily(cell));
String column = Bytes.toString(CellUtil.cloneQualifier(cell));
String value = Bytes.toString(CellUtil.cloneValue(cell));
String rowkey = Bytes.toString(CellUtil.cloneRow(cell));
System.out.println("rowKey: " + rowkey + ", " + cf + ", " + column + ", " + value);
}
}
table.close();
admin.close();
connection.close();
}
}
运行可以获得如下结果:
Scan+Row
java
public class Test06 {
public static void main(String[] args) throws IOException {
Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", "h121.wzk.icu,h122.wzk.icu");
configuration.set("hbase.zookeeper.property.clientPort", "2181");
Connection connection = ConnectionFactory.createConnection(configuration);
HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
HTable table = (HTable) connection.getTable(TableName.valueOf("test01"));
Scan scan = new Scan();
scan.setStartRow("rk1".getBytes());
scan.setStopRow("rk2".getBytes());
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
Cell[] cells = result.rawCells();
for (Cell cell : cells) {
String cf = Bytes.toString(CellUtil.cloneFamily(cell));
String column = Bytes.toString(CellUtil.cloneQualifier(cell));
String value = Bytes.toString(CellUtil.cloneValue(cell));
String rowkey = Bytes.toString(CellUtil.cloneRow(cell));
System.out.println("rowKey: " + rowkey + ", " + cf + ", " + column + ", " + value);
}
}
table.close();
admin.close();
connection.close();
}
}