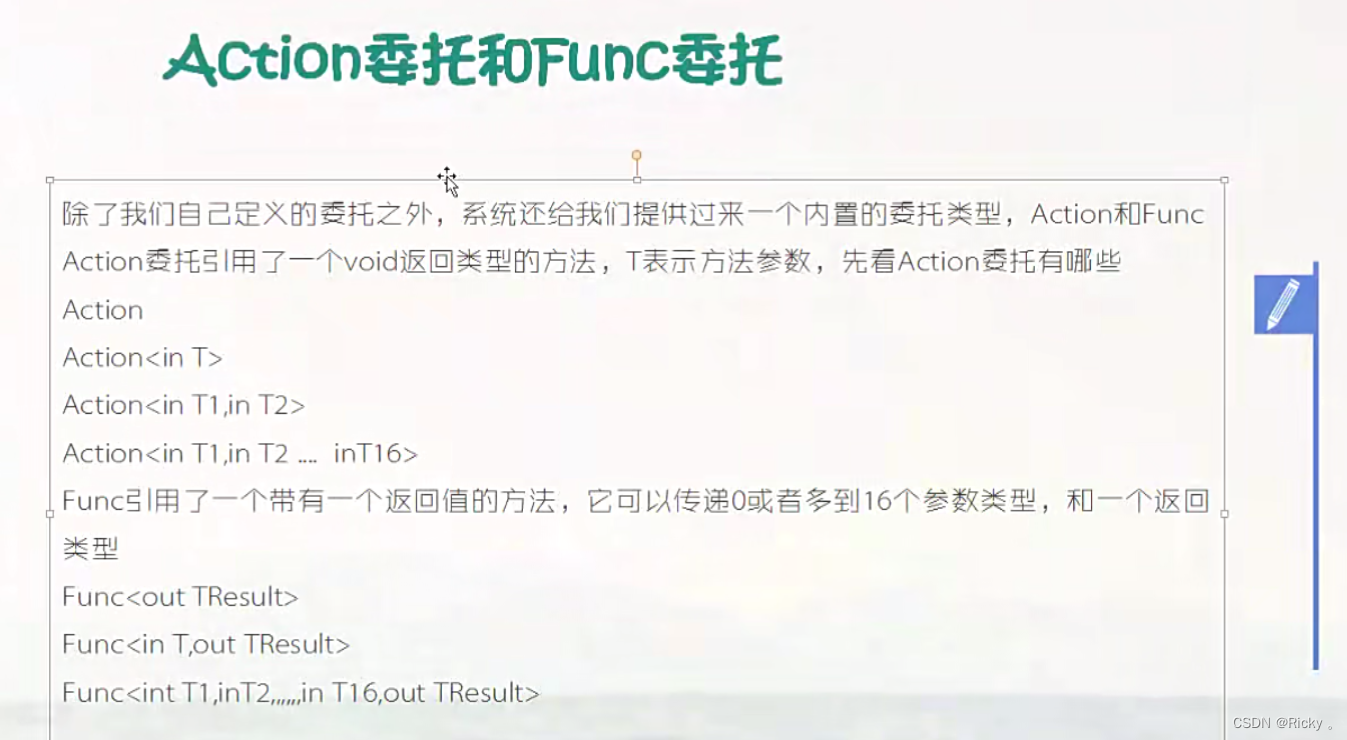
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Action委托
{
internal class Program
{
static void PrintString()
{
Console.WriteLine("hello world.");
}
static void PrintInt(int i)
{
Console.WriteLine(i);
}
static void PrintString(string str)
{
Console.WriteLine(str);
}
static void PrintDoubleInt(int i1,int i2)
{
Console.WriteLine(i1 + i2);
}
static void Main(string[] args)
{
Action a = PrintString;//Action是系统内置(预定义)的一个委托类型,它可以指向一个没有返回值,没有参数的方法
Action<int> b=PrintInt;//定义了一个委托类型,这个类型可以指向一个没有返回值,有一个int参数的方法
Action<string> c = PrintString;//定义了一个委托类型,这个类型可以指向一个没有返回值,有一个string参数的方法,因为叫PrintString的方法有两个,在这里系统会自动寻找匹配的方法
Action<int, int> d = PrintDoubleInt;
d(34, 23);
Console.ReadKey();
//Action可以通过泛型去指定Action指向的方法的多个参数的类型,参数的类型跟Action后面声明的委托类型是对应着的
}
}
}
cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Func委托
{
internal class Program
{
static int Test1()
{
return 1;
}
static int Test2(string str)
{
Console.WriteLine(str);
return 100;
}
static int Test3(int i,int j)
{
return i + j;
}
static void Main(string[] args)
{
//Func<int> a = Test1;//func中的泛型类型制定的是 方法的返回值类型
//Console.WriteLine(a());
Func<string,int> b=Test2;//func后面可以跟很多类型,最后一个类型是返回值类型,前面的类型是参数类型,参数类型必须跟指向的方法的参数类型按照顺序对应
Func<int,int,int> a=Test3;//func后面必须指定一个返回值类型,参数类型可以有0-16个,先写参数类型,最后一个是返回值类型
int res = a(1, 5);
Console.WriteLine(res);
Console.ReadKey();
}
}
}