- webpack5主要是内部效率的优化
- 对比webpack4,没有太多使用上的改动
基本配置
- 拆分配置和merge
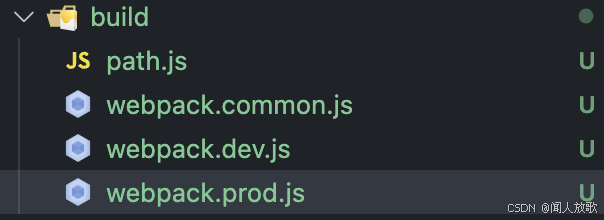
javascript
module.exports = merge(commonConfig, { /** options **/})
- 启动本地服务
在dev中添加配置
javascript
devServer: {
static: {
directory: distPath,
},
port: 8089,
hot: true,
compress: true,
open: true, // 自动打开浏览器
proxy: [{
context: ['/api'],
target: 'http://localhost:3000',
secure: false,
changeOrigin: true,
pathRewrite: {'^/api' : ''},
}],
},
在package.json script中添加命令
javascript
"scripts": {
"start": "webpack serve --config build/webpack.dev.js",
},
- 处理ES6
使用babel-loader
javascript
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
},
}
],
},
- 处理样式
javascript
module: {
rules: [
{
test: /\.css$/,
use: ['style-loader', 'css-loader', 'postcss-loader'],
},
{
test: /\.sass$/,
use: ['style-loader', 'css-loader', 'postcss-loader', 'sass-loader'],
}
],
},
- 处理图片
开发环境:
javascript
module: {
rules: [
{
test: /\.(png|jpg|jpeg|gif)$/,
use: ['file-loader'],
},
],
},
生产环境
javascript
module: {
rules: [
{
// 小于5 * 1024大小,图片转base64
test: /\.(png|png|jpg|jpeg|gif)/,
use: [{
loader: "url-loader",
options: {
limit: 1024 * 5,
name: "img/[name].[hash:8].[ext]",
outputPath: "img",
}
}
],
},
],
},
- 模块化
javascript
// webpack.common.js
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './src/index.js',
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env'],
},
},
},
{
test: /\.css$/,
use: ['style-loader', 'css-loader'],
},
],
},
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html',
}),
],
};
javascript
// webpack.dev.js
const { merge } = require('webpack-merge');
const common = require('./webpack.common.js');
module.exports = merge(common, {
mode: 'development',
devtool: 'inline-source-map',
devServer: {
contentBase: './dist',
port: 3000,
},
});
javascript
// webpack.prod.js
const { merge } = require('webpack-merge');
const common = require('./webpack.common.js');
const TerserPlugin = require('terser-webpack-plugin');
const MiniCssExtractPlugin = require('mini-css-extract-plugin');
const CssMinimizerPlugin = require('css-minimizer-webpack-plugin');
module.exports = merge(common, {
mode: 'production',
devtool: 'source-map',
output: {
filename: '[name].[contenthash].js',
path: path.resolve(__dirname, 'dist'),
clean: true, // 清理 /dist 文件夹
},
module: {
rules: [
{
test: /\.css$/,
use: [MiniCssExtractPlugin.loader, 'css-loader'],
},
],
},
plugins: [
new MiniCssExtractPlugin({
filename: '[name].[contenthash].css',
}),
],
optimization: {
minimizer: [
new TerserPlugin(),
new CssMinimizerPlugin(),
],
splitChunks: {
chunks: 'all',
},
},
});
高级配置
- 多入口
javascript
module.exports = {
entry: {
index: path.join(srcPath, "index.js"),
other: path.join(srcPath, "other.js"),
},
output: {
path: distPath,
filename: "[name].[contenthash].js",
clean: true,
},
plugins: [
new HtmlWebpackPlugin({
template: path.join(srcPath, "index.html"),
filename: "index.html",
chunks: ["index", "common", "vendor"], // 与entry中的index对应
}),
new HtmlWebpackPlugin({
template: path.join(srcPath, "other.html"),
filename: "other.html",
chunks: ["other", "common", "vendor"],
}), // 与entry中的other对应
],
};
- 抽离CSS文件
javascript
module.exports = merge(commonConfig, {
mode: "production",
module: {
rules: [
{
test: /\.scss$/,
use: [MiniCssExtractPlugin.loader, 'css-loader', 'postcss-loader', 'sass-loader'],
},
{
test: /\.css$/i,
use: [MiniCssExtractPlugin.loader, "css-loader", "postcss-loader"],
}
],
},
plugins: [
new webpack.CleanPlugin(),
new DefinePlugin({ "ENV": JSON.stringify("production") }),
new MiniCssExtractPlugin({ filename: "css/[name].[contenthash:8].css", chunkFilename: "css/[name].[contenthash:8].css" })
]
});
- 抽离公共代码
javascript
module.exports = merge(commonConfig, {
mode: "production",
optimization: {
splitChunks: {
chunks: "all",
cacheGroups: {
vendor: {
name: 'vendor',
test: /[\\/]node_modules[\\/]/,
minSize: 0,
minChunks: 1,
priority: 1
},
common: {
name: 'common',
minSize: 0,
minChunks: 2,
priority: 2,
}
}
}
}
});
- 懒加载
引用动态数据
javascript
setTimeout(() => import(./dynamic-data.js).then(res => {
console.log(res.default.message);
}))
- 处理JSX
.babelrc
javascript
{
"preset": ["@babel/preset-env"],
"plugins": []
}
webpack.config.js
javascript
rules: [
{
test: /\.js$/,
loader: ['babel-loader'],
include: srcPath,
exclude: /node_modules/
}
]
--
- 处理Vue
使用vue-loader
javascript
{
test: /\.vue$/,
loader: ['vue-loader'],
include: srcPath
}