目录
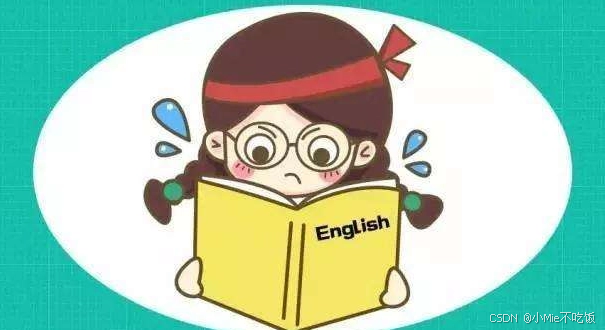
项目名称
随机单词生成器(Random Word Generator)
项目目标
- 学习C++基本语法和数据结构。
- 练习使用C++标准库中的随机数生成功能。
- 理解容器(如
std::vector
)的使用方法。 - 实践简单的用户界面设计。
功能描述
- 单词存储 :使用
std::vector
存储一组单词。 - 随机选择:从单词列表中随机选择一个单词。
- 用户交互:允许用户输入命令来获取随机单词或退出程序。
- 输出结果:显示选中的单词。
技术要点
- C++基础:了解C++的基本语法,如变量声明、控制流(if语句、循环)。
- 标准库 :熟悉
<iostream>
、<vector>
、<cstdlib>
、<ctime>
等头文件的使用。 - 随机数生成 :使用
std::rand()
和srand()
函数生成随机数。 - 容器操作 :学习如何向
std::vector
中添加元素,以及如何遍历容器。
(想学没环境的可以看看我以下文章)
Windows - C 语言开发环境 llvm-mingw + vscode (零基础超适用)
为什么很多人都无法解决 VSCode C 系列调试问题 (经验分享 有用)
示例代码
cpp
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
// 假设我们有一个简单的单词结构
struct Word {
std::string word;
std::string translation;
std::string example;
};
int main() {
// 初始化单词数据库
std::vector<Word> words = {
{"apple", "苹果", "I ate an apple yesterday."}, // 我昨天吃了一个苹果。
{"book", "书", "She is reading a book about history."}, // 她正在阅读一本关于历史的书籍。
{"cat", "猫", "The cat is sleeping on the couch."}, // 猫正在沙发上睡觉。
{"dog", "狗", "His dog is very friendly."}, // 他的狗非常友好。
{"elephant", "大象", "Elephants are the largest land animals."}, // 大象是陆地上最大的动物。
{"flower", "花", "She loves flowers and often buys some for her room."}, // 她喜欢花,经常买一些来装饰房间。
{"giraffe", "长颈鹿", "A giraffe can reach leaves high up in trees."}, // 长颈鹿能够到树上很高的叶子。
{"hat", "帽子", "Don't forget to take your hat; it's cold outside."}, // 不要忘记戴帽子;外面很冷。
{"ice", "冰", "The ice cream is melting in the hot sun."}, // 冰淇淋在炎热的阳光下融化了。
{"jacket", "夹克", "He wore a leather jacket to the party."}, // 他穿着皮夹克去参加派对。
{"kite", "风筝", "The children were flying kites in the park."}, // 孩子们在公园里放风筝。
{"lemon", "柠檬", "She squeezed some lemon into the tea."}, // 她挤了一些柠檬汁到茶里。
{"monkey", "猴子", "The monkey climbed up the tall tree."}, // 猴子爬上了高高的树。
{"nose", "鼻子", "She touched her nose to make sure she wasn't dreaming."}, // 她摸了摸鼻子,确定自己不是在做梦。
{"orange", "橙子", "Oranges are rich in vitamin C."}, // 橙子富含维生素C。
{"pencil", "铅笔", "I need a pencil to write this down."}, // 我需要一支铅笔来记下这个。
{"queen", "女王", "The queen was crowned in a grand ceremony."}, // 女王在一个盛大的仪式中被加冕。
{"rabbit", "兔子", "The rabbit hopped quickly across the road."}, // 兔子快速地跳过了马路。
{"snake", "蛇", "The snake slithered silently through the grass."}, // 蛇悄无声息地在草丛中滑行。
{"tree", "树", "The tree's branches provided shade for the picnickers."}, // 树的枝条为野餐者提供了阴凉。
{"umbrella", "伞", "She opened her umbrella to shield herself from the rain."}, // 她打开了伞以遮挡雨水。
{"violin", "小提琴", "He played a beautiful tune on his violin."}, // 他用小提琴演奏了一首美妙的旋律。
{"window", "窗户", "The window was open to let in the fresh air."}, // 窗户开着以让新鲜空气进来。
{"xylophone", "木琴", "The musician played a lively song on the xylophone."}, // 音乐家用木琴演奏了一首活泼的歌曲。
{"yacht", "游艇", "They sailed their yacht along the coast."}, // 他们沿着海岸航行他们的游艇。
{"zebra", "斑马", "The zebra's stripes are unique to each individual."} // 斑马的条纹对每个个体来说都是独一无二的。
};
// 随机种子
srand(static_cast<unsigned int>(time(nullptr)));
// 随机选择一个单词进行测试
int index = rand() % words.size();
Word currentWord = words[index];
// 显示单词和例句
std::cout << "Word: " << currentWord.word << std::endl;
std::cout << "Example: " << currentWord.example << std::endl;
// 这里可以添加更多的交互逻辑,如检查用户输入、播放发音等
return 0;
}
扩展建议
- 增加单词数量:扩充单词列表,包含更多单词。
- 用户界面:添加简单的文本菜单,允许用户选择获取更多单词或退出。
- 功能增强:添加功能,如显示单词释义、发音等。
- 错误处理:增加对用户输入的基本错误处理。
学习资源
- C++官方文档和在线教程。
- 编程社区和论坛,如Stack Overflow。
- 相关书籍,如《C++ Primer》。
(到底啦)