目录
[2.1 初始化通讯录](#2.1 初始化通讯录)
[2.2 添加通讯录数据](#2.2 添加通讯录数据)
[2.3 查找通讯录数据](#2.3 查找通讯录数据)
[2.4 删除通讯录数据](#2.4 删除通讯录数据)
[2.5 修改通讯录数据](#2.5 修改通讯录数据)
[2.6 展示通讯录数据编辑](#2.6 展示通讯录数据编辑)
[2.7 销毁通讯录数据](#2.7 销毁通讯录数据)
一、前言
上一篇我们讲了如何实现动态顺序表,这一篇就基于顺序表实现通讯录系统,我们知道顺序表的底层逻辑其实就是数组,同样的,通讯录系统也只是在顺序表之上进行了一层封装,相当于把每一个用户的所以数据都当做了数组中的一个元素,在通过顺序表的增删查改功能实现通讯录相对应的功能。
每个数组元素中都包含这样整个的用户数据
功能要求:
1.能够保存用户信息:名字、性别、年龄、电话、地址等
2.增加联系人信息
3.删除指定联系人
4.查找制定联系人
5.修改指定联系人
6.显示联系⼈信息
二、各个功能的实现
2.1 初始化通讯录
这里其实和顺序表的初始化是一样的,只要把数组置空,有效数据,空间容量归0就行了,
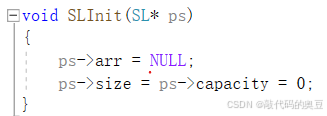
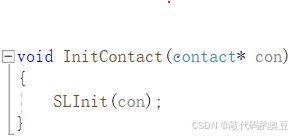
2.2 添加通讯录数据
添加通讯录数据同样需要用到写顺序表时用到的添加数据函数(毕竟通讯录是基于顺序表建立的系统),我这里用到的是尾插
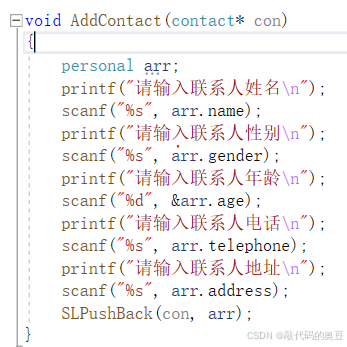
2.3 查找通讯录数据
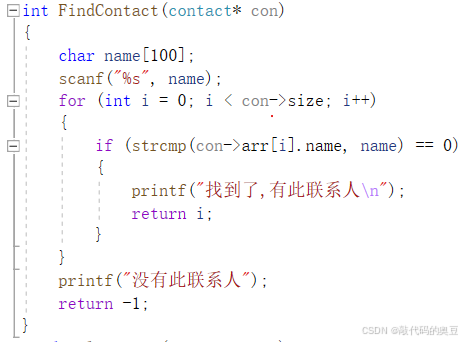
2.4 删除通讯录数据
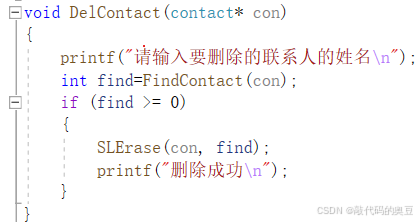
2.5 修改通讯录数据
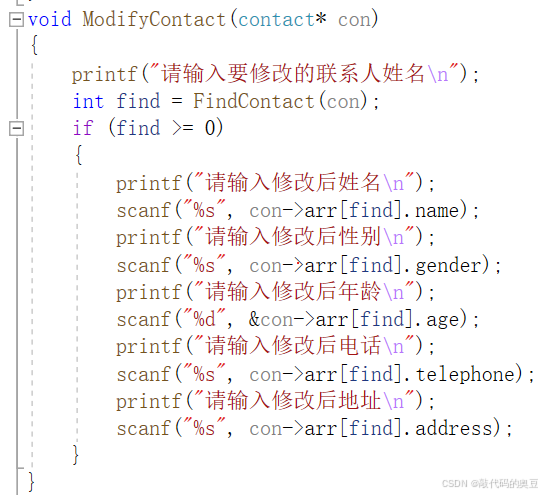
2.6 展示通讯录数据
2.7 销毁通讯录数据
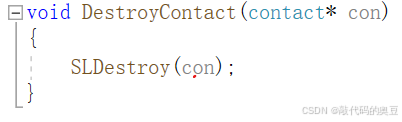
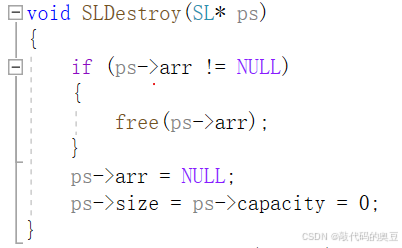
三、添加菜单和测试
作为一个系统肯定是要给别人用的,我们还需要加一个系统使用者提示,方便使用对应功能,
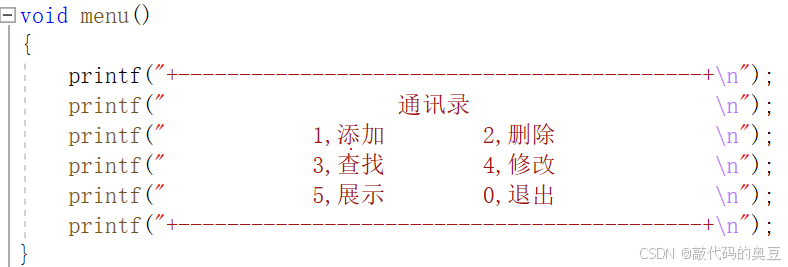
用switch语句对应数字对应到对应功能。
大功告成后还是需要进行测试的,避免有错误。
四、完整源码
sxb.h
#include<stdio.h>
#include<stdlib.h>
#include<assert.h>
#include"contact.h"
typedef personal SLDataType;
// 动态顺序表 -- 按需申请
typedef struct SeqList
{
SLDataType* arr;
int size; // 有效数据个数
int capacity; // 空间容量
}SL;
//初始化和销毁
void SLInit(SL* ps);
void SLDestroy(SL* ps);
//扩容
void SLCheckCapacity(SL* ps);
//头部插入删除 / 尾部插入删除
void SLPushBack(SL* ps, SLDataType x);
void SLPopBack(SL* ps);
void SLPushFront(SL* ps, SLDataType x);
void SLPopFront(SL* ps);
void SLprint(SL* ps);
//指定位置之前插入/删除数据
void SLInsert(SL* ps, int pos, SLDataType x);
void SLErase(SL* ps, int pos);
sxb.c
#include"sxb.h"
void SLInit(SL* ps)
{
ps->arr = NULL;
ps->size = ps->capacity = 0;
}
void SLDestroy(SL* ps)
{
if (ps->arr != NULL)
{
free(ps->arr);
}
ps->arr = NULL;
ps->size = ps->capacity = 0;
}
void SLCheckCapacity(SL* ps)
{
if (ps->capacity == ps->size)
{
int newcapacity = ps->capacity == 0 ? 4 : 2 * ps->capacity;
SLDataType* tem = (SLDataType*)realloc(ps->arr, newcapacity * sizeof(SLDataType));
if (tem == NULL)
{
perror("realloc fail");
exit(1);
}
ps->arr = tem;
ps->capacity = newcapacity;
}
}
void SLPushFront(SL* ps, SLDataType x)
{
assert(ps);
SLCheckCapacity(ps);
int i;
for (i = ps->size; i > 0; i--)
{
ps->arr[i] = ps->arr[i - 1];
}
ps->arr[0] = x;
ps->size++;
}
void SLPushBack(SL* ps, SLDataType x)
{
assert(ps);
SLCheckCapacity(ps);
ps->arr[ps->size++] = x;
}
void SLPopFront(SL* ps)
{
assert(ps);
SLCheckCapacity(ps);
int i;
for (i = 0; i < ps->size; i++)
{
ps->arr[i] = ps->arr[i + 1];
}
ps->size--;
}
void SLPopBack(SL* ps)
{
assert(ps);
SLCheckCapacity(ps);
ps->size--;
}
void SLprint(SL* ps)
{
int i;
for (i = 0; i < ps->size; i++)
{
printf("%d ", ps->arr[i]);
}
printf("\n");
}
void SLInsert(SL* ps, int pos, SLDataType x)
{
assert(ps);
SLCheckCapacity(ps);
int i = 0;
for (i = ps->size; i > pos; i--)
{
ps->arr[i] = ps->arr[i - 1];
}
ps->arr[pos] = x;
ps->size++;
}
void SLErase(SL* ps, int pos)
{
assert(ps);
SLCheckCapacity(ps);
int i = 0;
for (i = pos; i < ps->size - 1; i++)
{
ps->arr[i] = ps->arr[i + 1];
}
ps->size--;
}
contact.h
//前置声明
typedef struct SeqList contact;
//用户数据
typedef struct PersonInfo
{
char name[100];//姓名
char gender[5];//性别
int age;//年龄
char telephone[11];//电话
char address[100];//地址
}personal;
//初始化通讯录
void InitContact(contact* con);
//添加通讯录数据
void AddContact(contact* con);
//删除通讯录数据
void DelContact(contact* con);
//展示通讯录数据
void ShowContact(contact* con);
//查找通讯录数据
int FindContact(contact* con);
//修改通讯录数据
void ModifyContact(contact* con);
//销毁通讯录数据
void DestroyContact(contact* con);
contact.c
#include"contact.h"
#include"sxb.h"
#include<string.h>
void InitContact(contact* con)
{
SLInit(con);
}
void AddContact(contact* con)
{
personal arr;
printf("请输入联系人姓名\n");
scanf("%s", arr.name);
printf("请输入联系人性别\n");
scanf("%s", arr.gender);
printf("请输入联系人年龄\n");
scanf("%d", &arr.age);
printf("请输入联系人电话\n");
scanf("%s", arr.telephone);
printf("请输入联系人地址\n");
scanf("%s", arr.address);
SLPushBack(con, arr);
}
int FindContact(contact* con)
{
char name[100];
scanf("%s", name);
for (int i = 0; i < con->size; i++)
{
if (strcmp(con->arr[i].name, name) == 0)
{
printf("找到了,有此联系人\n");
return i;
}
}
printf("没有此联系人");
return -1;
}
void DelContact(contact* con)
{
printf("请输入要删除的联系人的姓名\n");
int find=FindContact(con);
if (find >= 0)
{
SLErase(con, find);
printf("删除成功\n");
}
}
void ShowContact(contact* con)
{
printf("姓名 性别 年龄 电话 地址\n");
for (int i = 0; i < con->size; i++)
{
printf("%5s %5s %5d %5s %6s\n", con->arr[i].name,con->arr[i].gender,con->arr[i].age, con->arr[i].telephone, con->arr[i].address);
}
}
void ModifyContact(contact* con)
{
printf("请输入要修改的联系人姓名\n");
int find = FindContact(con);
if (find >= 0)
{
printf("请输入修改后姓名\n");
scanf("%s", con->arr[find].name);
printf("请输入修改后性别\n");
scanf("%s", con->arr[find].gender);
printf("请输入修改后年龄\n");
scanf("%d", &con->arr[find].age);
printf("请输入修改后电话\n");
scanf("%s", con->arr[find].telephone);
printf("请输入修改后地址\n");
scanf("%s", con->arr[find].address);
}
}
void DestroyContact(contact* con)
{
SLDestroy(con);
}
test.c
#include"contact.h"
void menu()
{
printf("+-------------------------------------------+\n");
printf(" 通讯录 \n");
printf(" 1,添加 2,删除 \n");
printf(" 3,查找 4,修改 \n");
printf(" 5,展示 0,退出 \n");
printf("+-------------------------------------------+\n");
}
void mycontact()
{
personal p1;
int c=1;
InitContact(&p1);
while (c)
{
printf("请输入:");
scanf("%d", &c);
switch (c)
{
case 0:break;
case 1:
AddContact(&p1);
break;
case 2:
DelContact(&p1);
break;
case 3:
FindContact(&p1);
break;
case 4:
ModifyContact(&p1);
break;
case 5:
ShowContact(&p1);
break;
default:
printf("输入错误");
}
}
printf("退出通讯录");
DestroyContact(&p1);
}
int main()
{
menu();
mycontact();
return 0;
}
本篇内容就到这里了,希望对各位有帮助,如果有错误欢迎指出。
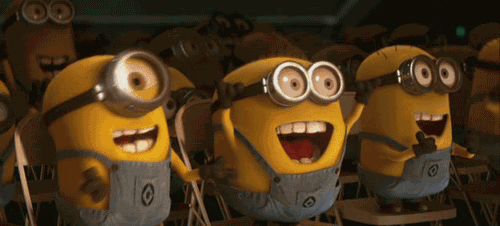