一、绪论
Java系统中,分页查询的场景随处可见,本节介com.baomidou.mybatisplus.core.metadata.IPage;来分页的工具类
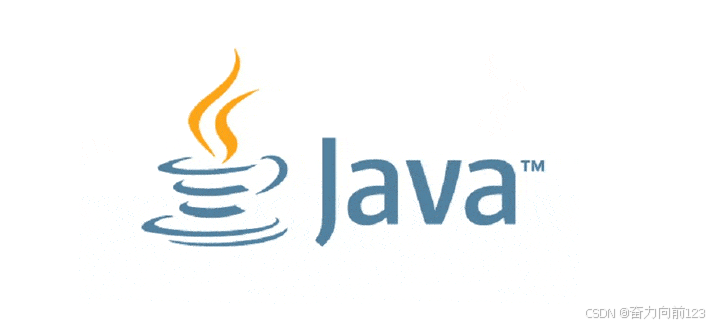
二、分页工具类
public class PageUtils implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 总记录数
*/
private int totalCount;
/**
* 每页记录数
*/
private int pageSize;
/**
* 总页数
*/
private int totalPage;
/**
* 当前页数
*/
private int currPage;
/**
* 列表数据
*/
private List<?> list;
/**
* 分页
* @param list 列表数据
* @param totalCount 总记录数
* @param pageSize 每页记录数
* @param currPage 当前页数
*/
public PageUtils(List<?> list, int totalCount, int pageSize, int currPage) {
this.list = list;
this.totalCount = totalCount;
this.pageSize = pageSize;
this.currPage = currPage;
this.totalPage = (int)Math.ceil((double)totalCount/pageSize);
}
/**
* 分页
*/
public PageUtils(IPage<?> page) {
this.list = page.getRecords();
this.totalCount = (int)page.getTotal();
this.pageSize = (int)page.getSize();
this.currPage = (int)page.getCurrent();
this.totalPage = (int)page.getPages();
}
public int getTotalCount() {
return totalCount;
}
public void setTotalCount(int totalCount) {
this.totalCount = totalCount;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public int getTotalPage() {
return totalPage;
}
public void setTotalPage(int totalPage) {
this.totalPage = totalPage;
}
public int getCurrPage() {
return currPage;
}
public void setCurrPage(int currPage) {
this.currPage = currPage;
}
public List<?> getList() {
return list;
}
public void setList(List<?> list) {
this.list = list;
}
}
java
public class PageUtils implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 总记录数
*/
private int totalCount;
/**
* 每页记录数
*/
private int pageSize;
/**
* 总页数
*/
private int totalPage;
/**
* 当前页数
*/
private int currPage;
/**
* 列表数据
*/
private List<?> list;
/**
* 分页
* @param list 列表数据
* @param totalCount 总记录数
* @param pageSize 每页记录数
* @param currPage 当前页数
*/
public PageUtils(List<?> list, int totalCount, int pageSize, int currPage) {
this.list = list;
this.totalCount = totalCount;
this.pageSize = pageSize;
this.currPage = currPage;
this.totalPage = (int)Math.ceil((double)totalCount/pageSize);
}
/**
* 分页
*/
public PageUtils(IPage<?> page) {
this.list = page.getRecords();
this.totalCount = (int)page.getTotal();
this.pageSize = (int)page.getSize();
this.currPage = (int)page.getCurrent();
this.totalPage = (int)page.getPages();
}
public int getTotalCount() {
return totalCount;
}
public void setTotalCount(int totalCount) {
this.totalCount = totalCount;
}
public int getPageSize() {
return pageSize;
}
public void setPageSize(int pageSize) {
this.pageSize = pageSize;
}
public int getTotalPage() {
return totalPage;
}
public void setTotalPage(int totalPage) {
this.totalPage = totalPage;
}
public int getCurrPage() {
return currPage;
}
public void setCurrPage(int currPage) {
this.currPage = currPage;
}
public List<?> getList() {
return list;
}
public void setList(List<?> list) {
this.list = list;
}
}
Ipage接口
/*
* Copyright (c) 2011-2021, baomidou (jobob@qq.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.baomidou.mybatisplus.core.metadata;
import java.io.Serializable;
import java.util.List;
import java.util.function.Function;
import static java.util.stream.Collectors.toList;
/**
* 分页 Page 对象接口
*
* @author hubin
* @since 2018-06-09
*/
public interface IPage<T> extends Serializable {
/**
* 获取排序信息,排序的字段和正反序
*
* @return 排序信息
*/
List<OrderItem> orders();
/**
* 自动优化 COUNT SQL【 默认:true 】
*
* @return true 是 / false 否
*/
default boolean optimizeCountSql() {
return true;
}
/**
* {@link com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor#isOptimizeJoin()}
* 两个参数都为 true 才会进行sql处理
*
* @return true 是 / false 否
* @since 3.4.4 @2021-09-13
*/
default boolean optimizeJoinOfCountSql() {
return true;
}
/**
* 进行 count 查询 【 默认: true 】
*
* @return true 是 / false 否
*/
default boolean searchCount() {
return true;
}
/**
* 计算当前分页偏移量
*/
default long offset() {
long current = getCurrent();
if (current <= 1L) {
return 0L;
}
return Math.max((current - 1) * getSize(), 0L);
}
/**
* 最大每页分页数限制,优先级高于分页插件内的 maxLimit
*
* @since 3.4.0 @2020-07-17
*/
default Long maxLimit() {
return null;
}
/**
* 当前分页总页数
*/
default long getPages() {
if (getSize() == 0) {
return 0L;
}
long pages = getTotal() / getSize();
if (getTotal() % getSize() != 0) {
pages++;
}
return pages;
}
/**
* 内部什么也不干
* <p>只是为了 json 反序列化时不报错</p>
*/
default IPage<T> setPages(long pages) {
// to do nothing
return this;
}
/**
* 分页记录列表
*
* @return 分页对象记录列表
*/
List<T> getRecords();
/**
* 设置分页记录列表
*/
IPage<T> setRecords(List<T> records);
/**
* 当前满足条件总行数
*
* @return 总条数
*/
long getTotal();
/**
* 设置当前满足条件总行数
*/
IPage<T> setTotal(long total);
/**
* 获取每页显示条数
*
* @return 每页显示条数
*/
long getSize();
/**
* 设置每页显示条数
*/
IPage<T> setSize(long size);
/**
* 当前页
*
* @return 当前页
*/
long getCurrent();
/**
* 设置当前页
*/
IPage<T> setCurrent(long current);
/**
* IPage 的泛型转换
*
* @param mapper 转换函数
* @param <R> 转换后的泛型
* @return 转换泛型后的 IPage
*/
@SuppressWarnings("unchecked")
default <R> IPage<R> convert(Function<? super T, ? extends R> mapper) {
List<R> collect = this.getRecords().stream().map(mapper).collect(toList());
return ((IPage<R>) this).setRecords(collect);
}
/**
* 老分页插件不支持
* <p>
* MappedStatement 的 id
*
* @return id
* @since 3.4.0 @2020-06-19
*/
default String countId() {
return null;
}
}
java
/*
* Copyright (c) 2011-2021, baomidou (jobob@qq.com).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.baomidou.mybatisplus.core.metadata;
import java.io.Serializable;
import java.util.List;
import java.util.function.Function;
import static java.util.stream.Collectors.toList;
/**
* 分页 Page 对象接口
*
* @author hubin
* @since 2018-06-09
*/
public interface IPage<T> extends Serializable {
/**
* 获取排序信息,排序的字段和正反序
*
* @return 排序信息
*/
List<OrderItem> orders();
/**
* 自动优化 COUNT SQL【 默认:true 】
*
* @return true 是 / false 否
*/
default boolean optimizeCountSql() {
return true;
}
/**
* {@link com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor#isOptimizeJoin()}
* 两个参数都为 true 才会进行sql处理
*
* @return true 是 / false 否
* @since 3.4.4 @2021-09-13
*/
default boolean optimizeJoinOfCountSql() {
return true;
}
/**
* 进行 count 查询 【 默认: true 】
*
* @return true 是 / false 否
*/
default boolean searchCount() {
return true;
}
/**
* 计算当前分页偏移量
*/
default long offset() {
long current = getCurrent();
if (current <= 1L) {
return 0L;
}
return Math.max((current - 1) * getSize(), 0L);
}
/**
* 最大每页分页数限制,优先级高于分页插件内的 maxLimit
*
* @since 3.4.0 @2020-07-17
*/
default Long maxLimit() {
return null;
}
/**
* 当前分页总页数
*/
default long getPages() {
if (getSize() == 0) {
return 0L;
}
long pages = getTotal() / getSize();
if (getTotal() % getSize() != 0) {
pages++;
}
return pages;
}
/**
* 内部什么也不干
* <p>只是为了 json 反序列化时不报错</p>
*/
default IPage<T> setPages(long pages) {
// to do nothing
return this;
}
/**
* 分页记录列表
*
* @return 分页对象记录列表
*/
List<T> getRecords();
/**
* 设置分页记录列表
*/
IPage<T> setRecords(List<T> records);
/**
* 当前满足条件总行数
*
* @return 总条数
*/
long getTotal();
/**
* 设置当前满足条件总行数
*/
IPage<T> setTotal(long total);
/**
* 获取每页显示条数
*
* @return 每页显示条数
*/
long getSize();
/**
* 设置每页显示条数
*/
IPage<T> setSize(long size);
/**
* 当前页
*
* @return 当前页
*/
long getCurrent();
/**
* 设置当前页
*/
IPage<T> setCurrent(long current);
/**
* IPage 的泛型转换
*
* @param mapper 转换函数
* @param <R> 转换后的泛型
* @return 转换泛型后的 IPage
*/
@SuppressWarnings("unchecked")
default <R> IPage<R> convert(Function<? super T, ? extends R> mapper) {
List<R> collect = this.getRecords().stream().map(mapper).collect(toList());
return ((IPage<R>) this).setRecords(collect);
}
/**
* 老分页插件不支持
* <p>
* MappedStatement 的 id
*
* @return id
* @since 3.4.0 @2020-06-19
*/
default String countId() {
return null;
}
}
三、运用
/**
* 分页查询权限信息
* @param param
* @return
*/
public PageUtils queryPage(Map<String, Object> param) {
IPage<TMenu> page = new Query<TMenu>().getPage(param);
QueryWrapper<TMenu> wrapper = new QueryWrapper<>();
if (param.containsKey("leftId")) {
wrapper.eq("left_id", param.get("leftId").toString());
}
wrapper.eq("state", "0");
wrapper.orderByAsc("sort");
IPage<TMenu> tMenuIPage = tMenuMapper.selectPage(page, wrapper);
return new PageUtils(tMenuIPage);
}
java
/**
* 分页查询权限信息
* @param param
* @return
*/
public PageUtils queryPage(Map<String, Object> param) {
IPage<TMenu> page = new Query<TMenu>().getPage(param);
QueryWrapper<TMenu> wrapper = new QueryWrapper<>();
if (param.containsKey("leftId")) {
wrapper.eq("left_id", param.get("leftId").toString());
}
wrapper.eq("state", "0");
wrapper.orderByAsc("sort");
IPage<TMenu> tMenuIPage = tMenuMapper.selectPage(page, wrapper);
return new PageUtils(tMenuIPage);
}