目录
- [1- 思路](#1- 思路)
-
- [哈希表 + 滑动窗口](#哈希表 + 滑动窗口)
- [2- 实现](#2- 实现)
-
- [⭐438. 找到字符串中所有字母异位词------题解思路](#⭐438. 找到字符串中所有字母异位词——题解思路)
- [3- ACM 实现](#3- ACM 实现)
- 原题链接:438. 找到字符串中所有字母异位词
1- 思路
哈希表 + 滑动窗口
思路
- 哈希表:通过数组维护一个哈希表
- 滑动窗口:通过控制数组的下标,来实现滑动窗口
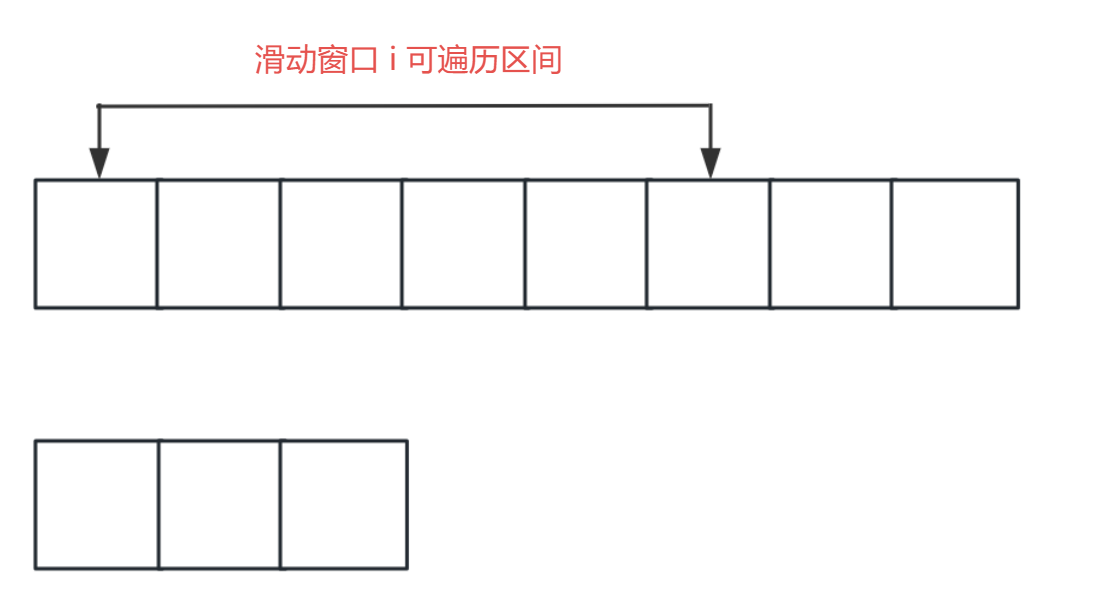
2- 实现
⭐438. 找到字符串中所有字母异位词------题解思路
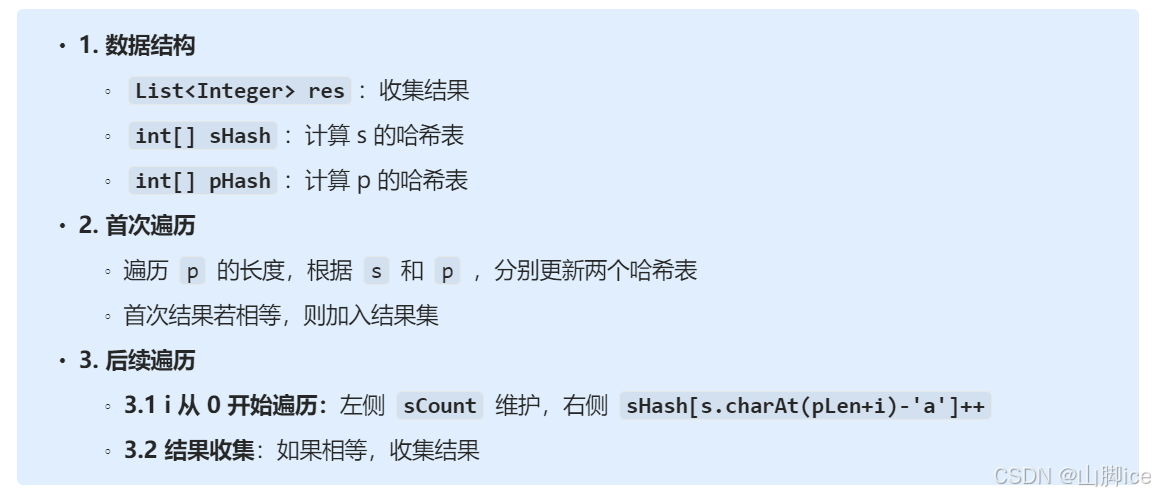
java
class Solution {
public List<Integer> findAnagrams(String s, String p) {
//1. 数据结构
List<Integer> res = new ArrayList<>();
int[] sHash = new int[26];
int[] pHash = new int[26];
int sLen = s.length();
int pLen = p.length();
if(sLen<pLen){
return res;
}
// 2.首次遍历
for(int i = 0 ; i < pLen;i++){
sHash[s.charAt(i)-'a']++;
pHash[p.charAt(i)-'a']++;
}
if(Arrays.equals(sHash,pHash)){
res.add(0);
}
// 3.循环遍历
for(int i = 0; i <sLen-pLen;i++){
// 3.1左侧维护
sHash[s.charAt(i)-'a']--;
sHash[s.charAt(pLen+i)-'a']++;
if(Arrays.equals(sHash,pHash)){
res.add(i+1);
}
}
return res;
}
}
3- ACM 实现
java
public class findAnagrams {
public static List<Integer> findA(String s,String p){
//1. 数据结构
List<Integer> res = new ArrayList<>();
int[] sCount = new int[26];
int[] pCount = new int[26];
int sLen = s.length();
int pLen = p.length();
if(sLen<pLen){
return res;
}
// 2.首次遍历
for(int i = 0 ; i < pLen;i++){
sCount[s.charAt(i)-'a']++;
pCount[p.charAt(i)-'a']++;
}
if(Arrays.equals(sCount,pCount)){
res.add(0);
}
// 3.再次遍历
for(int i =0;i<sLen-pLen;i++){
sCount[s.charAt(i)-'a']--;
sCount[s.charAt(i+pLen)-'a']++;
if(Arrays.equals(sCount,pCount)){
res.add(i+1);
}
}
return res;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("输入字符串1");
String str1 = sc.nextLine();
System.out.println("输入字符串2");
String str2 = sc.nextLine();
System.out.println("结果是"+findA(str1,str2));
}
}