1、完成基本的三层架构
1.1创建Account表

创建实体类 Account
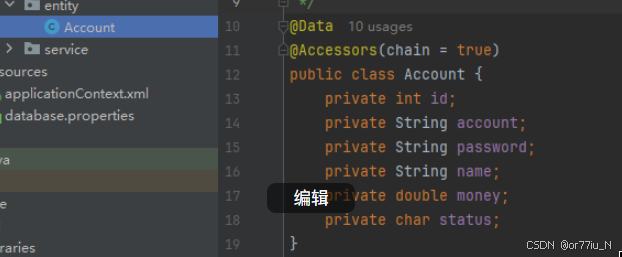
1.2 Service层写入 AccountService 接口
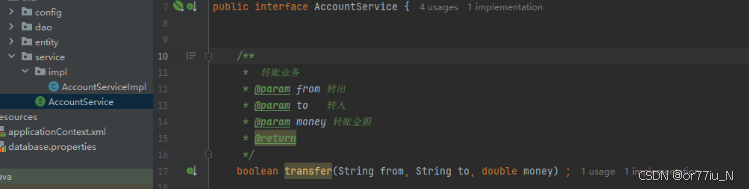
Service层 下写 impl 包定义 AccountServiceImpl 类 实现接口 AccountService
java
@Service
@Transactional
@RequiredArgsConstructor
public class AccountServiceImpl implements AccountService {
private final AccountDao accountDao;
// @Transactional 事务注解可以写再方法上,也可以写类上
@Override
public boolean transfer(String from, String to, double money) {
//获取转入账号信息
Account toAccount = accountDao.getAccountByAccount(to);
if (toAccount == null) {
System.out.println("账号不存在");
return false;
}
if (toAccount.getStatus() == 1) {
System.out.println("被禁用");
return false;
}
//获取转出账号信息
Account fromAccount = accountDao.getAccountByAccount(from);
if (fromAccount.getMoney() <= money) {
System.out.println("转出账号余额不足");
return false;
}
//实现转账
accountDao.addMoney(to, money);
accountDao.subMoney(from, money);
System.out.println("转账成功");
return true;
}
}
1.3 dao层写入 AccountDao 接口
dao层 下写 impl 包定义 AccountDaoImpl 类 实现接口 AccountDao
java
@Repository
@RequiredArgsConstructor
public class AccountDaoImpl implements AccountDao {
private final JdbcTemplate jdbcTemplate;
@Override
public Account getAccountByAccount(String account) {
String sql = "select * from account where account=?";
return jdbcTemplate.queryForObject(sql, new BeanPropertyRowMapper<>(Account.class),account);
}
@Override
public int addMoney(String account, double money) {
String sql = "update account set money=money+? where account=?";
return jdbcTemplate.update(sql,money,account);
}
@Override
public int subMoney(String account, double money) {
// int i=1/0;
String sql = "update account set money=money-? where account=?";
return jdbcTemplate.update(sql,money,account);
}
}
2、事务配置类
2.1跟dao层同级创建config包,config包下创建SpringConfig类
java
//@Configuration 该类式一个配置类,相当于一个applicationContext.xml配置文件
@Configuration
//@ComponentScan 表示组件扫描(会扫描basePackages包下(包含子包)所有注解)
//相当于配置文件中得<context:component-scan/>
@ComponentScan(basePackages = "com.cxd")
//@PropertySource 表示properties配置文件得资源路径
//相当于 <context:property-placeholder location="classpath*:database.properties"/>
@PropertySource(value = "classpath:database.properties")
//@EnableTransactionManagement 开启事务管理
//相当于配置文件中得 <tx:annotation-driven/> 事务管理驱动
@EnableTransactionManagement
public class SpringConfig {
//@Value写在属性上,表示读取配置文件中值注入到属性中
@Value("${jdbc.driver}")
private String driver;
@Value("${jdbc.url}")
private String url;
@Value("${jdbc.username}")
private String username;
@Value("${jdbc.password}")
private String password;
/**
* @Bean 写在配置类中得方法上 相当于配置文件中得 <bean></bean>标签
* 其中得返回子就是<bean class=""></bean> class属性
* bean在容器中得名字就是方法名
* 数据源
*/
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource =
new DriverManagerDataSource();
dataSource.setDriverClassName(driver);
dataSource.setUrl(url);
dataSource.setUsername(username);
dataSource.setPassword(password);
return dataSource;
}
@Bean
public JdbcTemplate getJdbcTemplate(DataSource dataSource) {
return new JdbcTemplate(dataSource);
}
@Bean
//事务管理器
public DataSourceTransactionManager transactionManager(DataSource dataSource) {
return new DataSourceTransactionManager(dataSource);
}
}
2.2数据库的连接
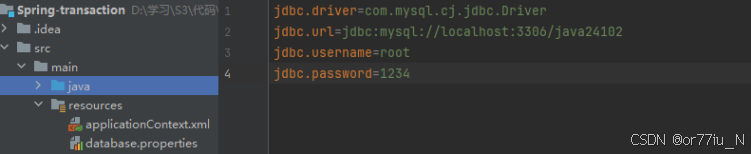
3、测试类
java
//@ContextConfiguration(locations = "classpath:applicationContext.xml")
@ContextConfiguration(classes = SpringConfig.class)
@RunWith(SpringJUnit4ClassRunner.class)
public class Test01 {
@Autowired
AccountDao accountDao;
@Autowired
AccountService accountService;
@Test
public void test02() {
accountService.transfer("888888","666666",100);
}
}
最后测试类,查看数据库数据
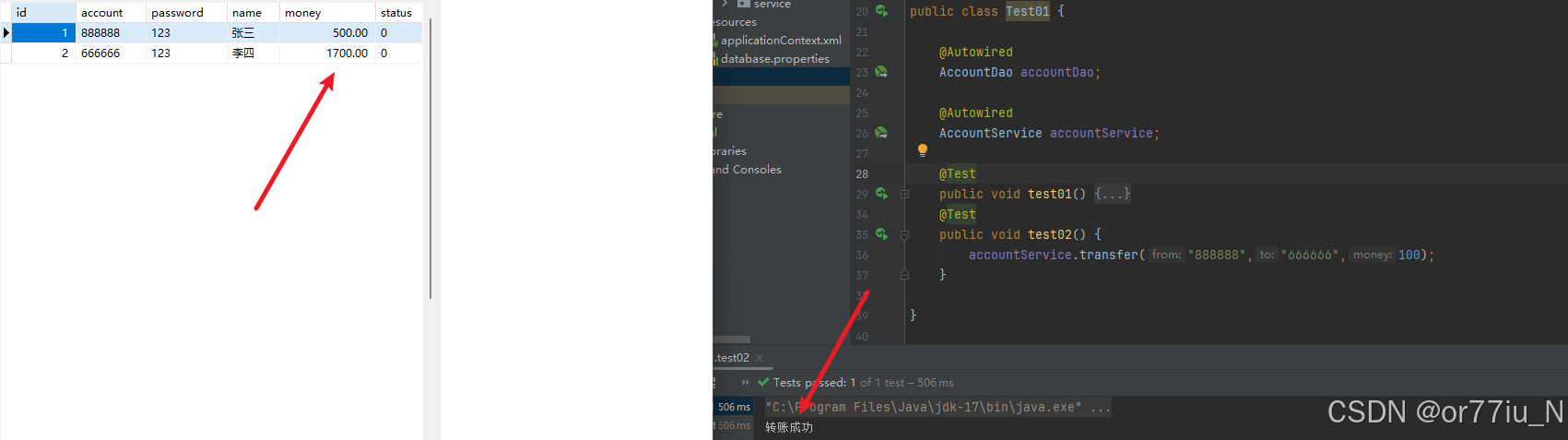
4、用到的jar包
java
@Repository
@RequiredArgsConstructor
public class AccountDaoImpl implements AccountDao {
private final JdbcTemplate jdbcTemplate;
@Override
public Account getAccountByAccount(String account) {
String sql = "select * from account where account=?";
return jdbcTemplate.queryForObject(sql, new BeanPropertyRowMapper<>(Account.class),account);
}
@Override
public int addMoney(String account, double money) {
String sql = "update account set money=money+? where account=?";
return jdbcTemplate.update(sql,money,account);
}
@Override
public int subMoney(String account, double money) {
// int i=1/0;
String sql = "update account set money=money-? where account=?";
return jdbcTemplate.update(sql,money,account);
}
}