在Spring MVC中,域对象(Domain Object)通常指的是与业务逻辑相关的模型对象,它们代表了应用程序中的核心数据结构。例如,在一个电商应用中,Product、User、Order等类可以被视为域对象。这些对象通常与数据库中的表相对应,并包含业务逻辑和数据验证等功能。
使用ServletAPI向request域对象共享数据
在Spring MVC中,使用HttpServletRequest对象可以直接操作请求范围的属性。通过request.setAttribute()方法设置的属性可以在同一请求的后续处理过程中使用,但它们不会在重定向或后续请求中保持。
java
@RequestMapping("/testServletAPI")
public String testServletAPI(HttpServletRequest request){
request.setAttribute("testScope", "hello,servletAPI");
return "success";
}
在这个例子中,testScope属性被设置为"hello, servletAPI",并且可以在返回的视图中访问。假设你有一个名为success.jsp的视图,你可以在该视图中使用EL表达式来访问这个属性:
html
<html>
<body>
<h1>${testScope}</h1> <!-- 输出: hello, servletAPI -->
</body>
</html>
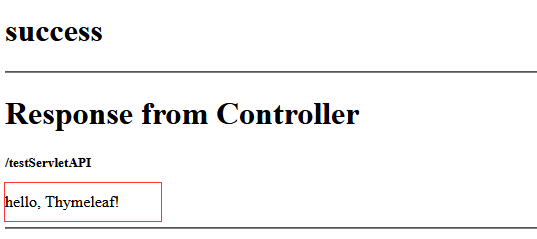
使用ModelAndView向request域对象共享数据
在Spring MVC中,使用ModelAndView对象可以方便地将数据传递到视图中。ModelAndView不仅可以设置模型数据,还可以指定视图名称。通过这种方式,你可以将数据放入请求域中,以便在视图中使用。
java
@RequestMapping("/testModelAndView")
public ModelAndView testModelAndView(){
/**
* ModelAndView有Model和View的功能
* Model主要用于向请求域共享数据
* View主要用于设置视图,实现页面跳转
*/
ModelAndView mav = new ModelAndView();
//向请求域共享数据
mav.addObject("testScope1", "hello,ModelAndView");
//设置视图,实现页面跳转
mav.setViewName("success");
return mav;
}
html
<h5>/testModelAndView</h5>
<p th:text="${testScope1}"></p>
<hr>
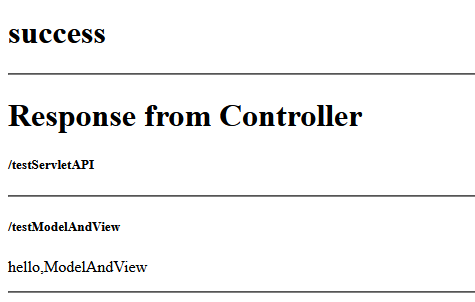
也可以通过这种方式
java
/**
* ModelAndView有Model和View的功能
* Model主要用于向请求域共享数据
* View主要用于设置视图,实现页面跳转
*/
@RequestMapping("/test/mvc")
public ModelAndView testMAV(){
ModelAndView mav = new ModelAndView();
//像请求域中共享数据
mav.addObject("name", "miaow");
//设计逻辑视图,就是跳转的页面
mav.setViewName("success");
return mav;
}
html
<h5>/test/mvc</h5>
<a th:href="@{/test/mvc}">获取ModelAndView中的请求域中共享数据 </a>
<p th:text="${name}"></p>
<hr>
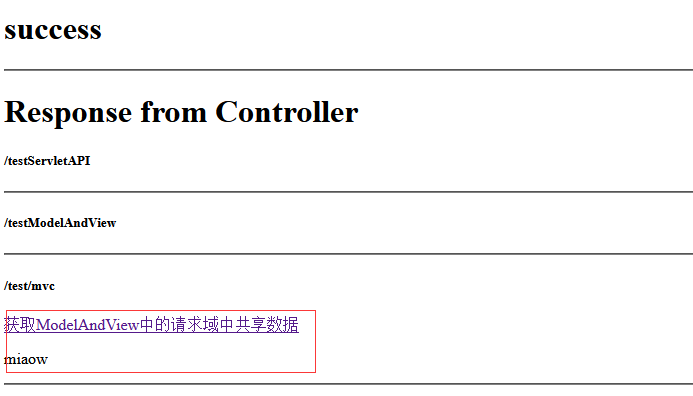
使用Model向request域对象共享数据
在Spring MVC中,使用Model对象可以方便地向请求域共享数据。Model是一个用于传递数据到视图的接口,允许你将属性添加到请求范围中。
java
@RequestMapping("/testModel")
public String testModel(Model model){
model.addAttribute("testScope3", "hello,Model");
return "success";
}
html
<h5>/testModel</h5>
<p th:text="${testScope3}"></p>
<hr>
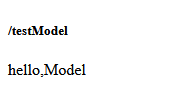
使用map向request域对象共享数据
在Spring MVC中,你可以使用Map对象向请求域共享数据。通过将数据放入Map中,你可以将多个属性传递到视图中。
java
@RequestMapping("/testMap")
public String testMap(Map<String, Object> map){
map.put("testScope4", "hello,Map");
return "success";
}
html
<h5>/testMap</h5>
<p th:text="${testScope4}"></p>
<hr>
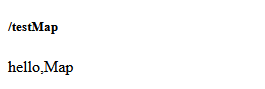
使用ModelMap向request域对象共享数据
在Spring MVC中,ModelMap是一个用于传递模型数据到视图的对象。它是Model接口的一个实现,允许你将属性添加到请求域中。
java
@RequestMapping("/testModelMap")
public String testModelMap(ModelMap modelMap){
modelMap.addAttribute("testScope5", "hello,ModelMap");
return "success";
}
html
<h5>/testModelMap</h5>
<p th:text="${testScope5}"></p>
<hr>
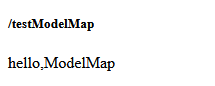
向session域共享数据
在Spring MVC中,向会话(session)域共享数据可以通过使用@SessionAttributes注解或直接使用HttpSession对象来实现。
@SessionAttributes
@SessionAttributes注解可以将特定的模型属性存储在HTTP会话中,这样在用户的多个请求之间可以共享数据。
java
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.SessionAttributes;
@Controller
@SessionAttributes("user")
public class UserController {
@ModelAttribute("user")
public User createUserModel() {
return new User(); // 创建一个新的User对象
}
@RequestMapping("/saveUser")
public String saveUser(@ModelAttribute("user") User user, Model model) {
user.setName("John Doe"); // 设置用户属性
model.addAttribute("message", "User saved successfully!");
return "success"; // 返回视图名称
}
@RequestMapping("/showUser")
public String showUser(@ModelAttribute("user") User user, Model model) {
model.addAttribute("message", "Current User: " + user.getName());
return "success"; // 返回视图名称
}
}
java
public class User {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
创建一个名为success.html的Thymeleaf模板文件,用于显示传递的数据。
java
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Session Example</title>
</head>
<body>
<h1>Response from Controller</h1>
<p th:text="${message}">This will be replaced by the message value.</p>
</body>
</html>
使用HttpSession
你也可以直接使用HttpSession对象来共享数据。
java
@RequestMapping("/testSession")
public String testSession(HttpSession session){
session.setAttribute("testSessionScope", "hello,session");
return "success";
}
html
<h5>/testSession</h5>
<p th:text="'Hello,session的值 ' + ${session.testSessionScope} + '!'"></p>
<hr>
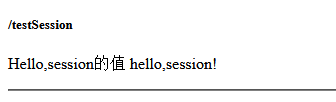
向application域共享数据
在Spring MVC中,向应用程序(application)域共享数据通常是通过ServletContext来实现的。应用程序域的数据在整个应用程序的生命周期内都是可用的,适合于存储全局共享的数据。
java
@RequestMapping("/testApplication")
public String testApplication(HttpSession session){
ServletContext application = session.getServletContext();
application.setAttribute("testApplicationScope", "hello,application");
return "success";
}
html
<h5>/testApplication</h5>
<p th:text="'Hello,application的值 ' + ${application.testApplicationScope} + '!'"></p>
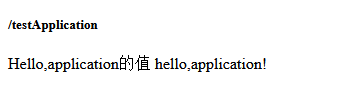
附上控制层代码:
html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>成功跳转页面</title>
</head>
<body>
<h1>success</h1>
<hr>
<h1>Response from Controller</h1>
<h5>/testServletAPI</h5>
<p th:text="${testScope}">This will be replaced by the testScope value.</p> <!-- 输出: hello, Thymeleaf! -->
<hr>
<h5>/testModelAndView</h5>
<p th:text="${testScope1}"></p>
<hr>
<h5>/test/mvc</h5>
<a th:href="@{/test/mvc}">获取ModelAndView中的请求域中共享数据 </a>
<p th:text="${name}"></p>
<hr>
<h5>/testModel</h5>
<p th:text="${testScope3}"></p>
<hr>
<h5>/testMap</h5>
<p th:text="${testScope4}"></p>
<hr>
<h5>/testModelMap</h5>
<p th:text="${testScope5}"></p>
<hr>
<h5>/testSession</h5>
<p th:text="'Hello,session的值 ' + ${session.testSessionScope} + '!'"></p>
<hr>
<h5>/testApplication</h5>
<p th:text="'Hello,application的值 ' + ${application.testApplicationScope} + '!'"></p>
</body>
</html>
java
@Controller
public class FieldController {
@RequestMapping("/testServletAPI")
public String testServletAPI(HttpServletRequest request) {
request.setAttribute("testScope", "hello, Thymeleaf!");
return "success"; // 返回视图名称
}
@RequestMapping("/testModelAndView")
public ModelAndView testModelAndView(){
/**
* ModelAndView有Model和View的功能
* Model主要用于向请求域共享数据
* View主要用于设置视图,实现页面跳转
*/
ModelAndView mav = new ModelAndView();
//向请求域共享数据
mav.addObject("testScope1", "hello,ModelAndView");
//设置视图,实现页面跳转
mav.setViewName("success");
return mav;
}
/**
* ModelAndView有Model和View的功能
* Model主要用于向请求域共享数据
* View主要用于设置视图,实现页面跳转
*/
@RequestMapping("/test/mvc")
public ModelAndView testMAV(){
ModelAndView mav = new ModelAndView();
//像请求域中共享数据
mav.addObject("name", "miaow");
//设计逻辑视图,就是跳转的页面
mav.setViewName("success");
return mav;
}
@RequestMapping("/testModel")
public String testModel(Model model){
model.addAttribute("testScope3", "hello,Model");
return "success";
}
@RequestMapping("/testMap")
public String testMap(Map<String, Object> map){
map.put("testScope4", "hello,Map");
return "success";
}
@RequestMapping("/testModelMap")
public String testModelMap(ModelMap modelMap){
modelMap.addAttribute("testScope5", "hello,ModelMap");
return "success";
}
@RequestMapping("/testSession")
public String testSession(HttpSession session){
session.setAttribute("testSessionScope", "hello,session");
return "success";
}
@RequestMapping("/testApplication")
public String testApplication(HttpSession session){
ServletContext application = session.getServletContext();
application.setAttribute("testApplicationScope", "hello,application");
return "success";
}
}
总结
- Request域:适合在单个请求中共享数据,使用Model、ModelMap、Map或ModelAndView。
- Session域:适合在多个请求之间共享数据,使用@SessionAttributes或HttpSession。
- Application域:适合在整个应用程序中共享数据,使用ServletContext。