一.面向对象
1.概念
1.1面向过程 C
吃饭:动作为核心
起身--》开门--》大量的逻辑判断
1.2面向对象 C++/Java/Python/Go
目标:吃饭
人(忽略)吃饭
站在人类的角度思考问题
2.什么是对象?
Object-->东西(万事万物皆是对象。)
2.1类
类:抽象,一个设计,对象的抽象
对象:具体,类的具体
类 -----> 对象
狗 ---> Dog ---> 抽象的
(两只耳朵,一只尾巴,四条腿,一张嘴,毛发)----特征
(陪伴(动作,行为))----职责
Class ---> Type
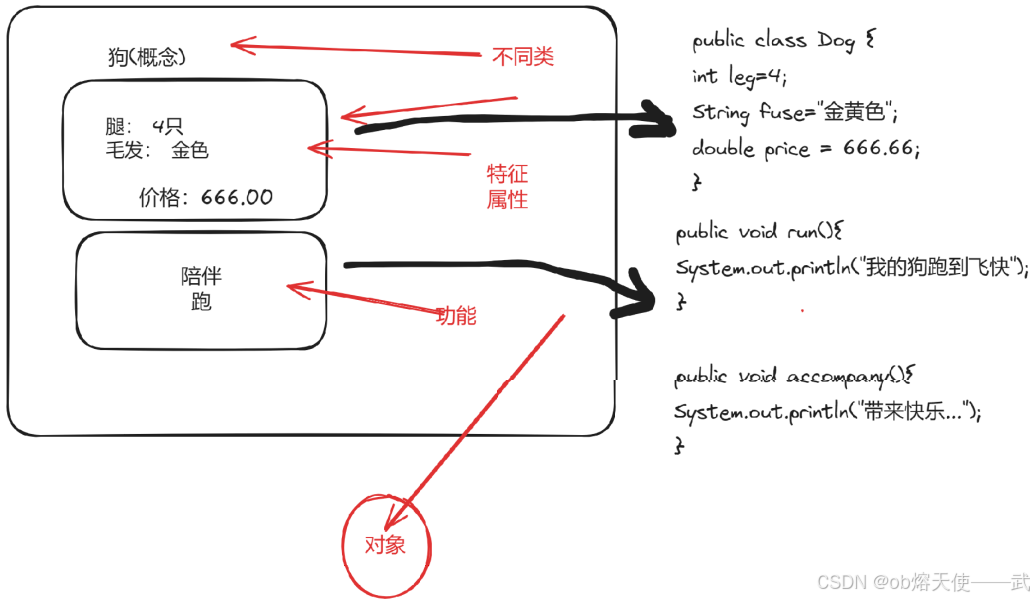
java
public class Dog {
//狗的信息
int leg = 4;
String hair = "金黄色";
double price = 666.66;
public void run(){
System.out.println("我的狗跑的飞快!");
}
public void accompany(){
System.out.println("带来快乐...");
}
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.run();
myDog.accompany();
}
}
3.以冰箱(Fridge)为例
1.名字(Fridge)
2.属性、特征、域(field)、xxx变量
3.方法、函数、动作
3.1Java的注释
*类、方法
java
/**
*
*
/
*行注释
java
//
*块注释
java
/*
*
*/
3.2冰箱的类
属性、方法
3.3执行冰箱操作
升高温度、降低温度工作
3.4异常情况
限制温度
java
import javax.swing.*;
//冰箱类
public class Fridge {
private String brand;
private String color;
private double size;
private double weight;
private double price;
private int year;//保修年限
private int temperature = 5;//温度
private String company;//公司
public String getBrand() {
return brand;
}
//给汽车的brand设置品牌
public void setBrand(String brand) {
this.brand = brand;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public double getSize() {
return size;
}
public void setSize(double size) {
this.size = size;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getTemperature() {
return temperature;
}
public void setTemperature(int temperature) {
this.temperature = temperature;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
//冰箱升高的温度方法
// n 要升高的温度
public void tempUp(int n){
if (temperature+n > 20){
JOptionPane.showMessageDialog(null,"温度超出冰箱范围,升温失败");
}else {
temperature += n;
}
}
//冰箱降低的温度方法
// n 要降低的温度
public void tempDown(int n){
if (temperature-n < -20){
JOptionPane.showMessageDialog(null,"温度超出冰箱范围,降温失败");
}else {
temperature -= n;
}
}
//显示当前冰箱的温度
public void print(){
System.out.println("当前温度:" + temperature);
}
public static void main(String[] args) {
Fridge myFridge = new Fridge();
myFridge.setBrand("无敌");
System.out.println(myFridge.getBrand());
System.out.println("冰箱启动");
myFridge.print();
while (true){
String opt = JOptionPane.showInputDialog("请选择操作(1-升温,2-降温)");
//String s = null;
int n = 0;
if ("1".equals(opt)) {
//升温操作
//String s = JOptionPane.showInputDialog("请输入要升高的度数");
n = Integer.parseInt(JOptionPane.showInputDialog("请输入要升高的度数"));
myFridge.tempUp(n);
}else if ("2".equals(opt)){
//降温操作
//s = JOptionPane.showInputDialog("请输入要降低的度数");
n = Integer.parseInt(JOptionPane.showInputDialog("请输入要降低的度数"));
myFridge.tempDown(n);
}
myFridge.print();
//判断是否退出操作
int c = JOptionPane.showConfirmDialog(null,"是否继续进行");
if (0 != c){
JOptionPane.showMessageDialog(null,"操作完成,谢谢使用");
break;
}
}
}
}
4.面向对象描述类
4.1文字描述
4.2代码描述
4.3类图描述
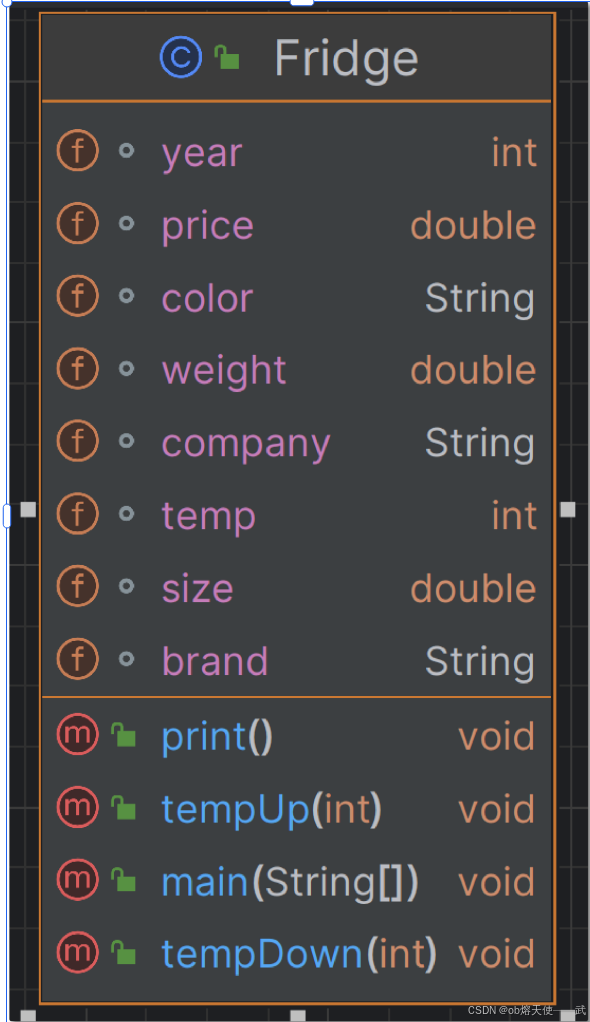
5.访问控制符
5.1 public---公开类型
任何代码都能访问
5.2 private---私有的
java
private String brand;
private String color;
private double size;
private double weight;
private double price;
private int year;//保修年限
private int temperature = 5;//温度
private String company;//公司
只有在本class内可以访问
6.属性操作
java
public String getBrand() {
return brand;
}
//给汽车的brand设置品牌
public void setBrand(String brand) {
this.brand = brand;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public double getSize() {
return size;
}
public void setSize(double size) {
this.size = size;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getTemperature() {
return temperature;
}
public void setTemperature(int temperature) {
this.temperature = temperature;
}
public String getCompany() {
return company;
}
public void setCompany(String company) {
this.company = company;
}
setter / getter方式
通过setXXX(参数)存储
通过getXXX()取出数据
7.this关键字
java
//给汽车的brand设置品牌
public void setBrand(String brand) {
this.brand = brand;
}
this ----- 当前对象
this.brand = brand; //当前对象的属性brand,值为传入的变量brand的值