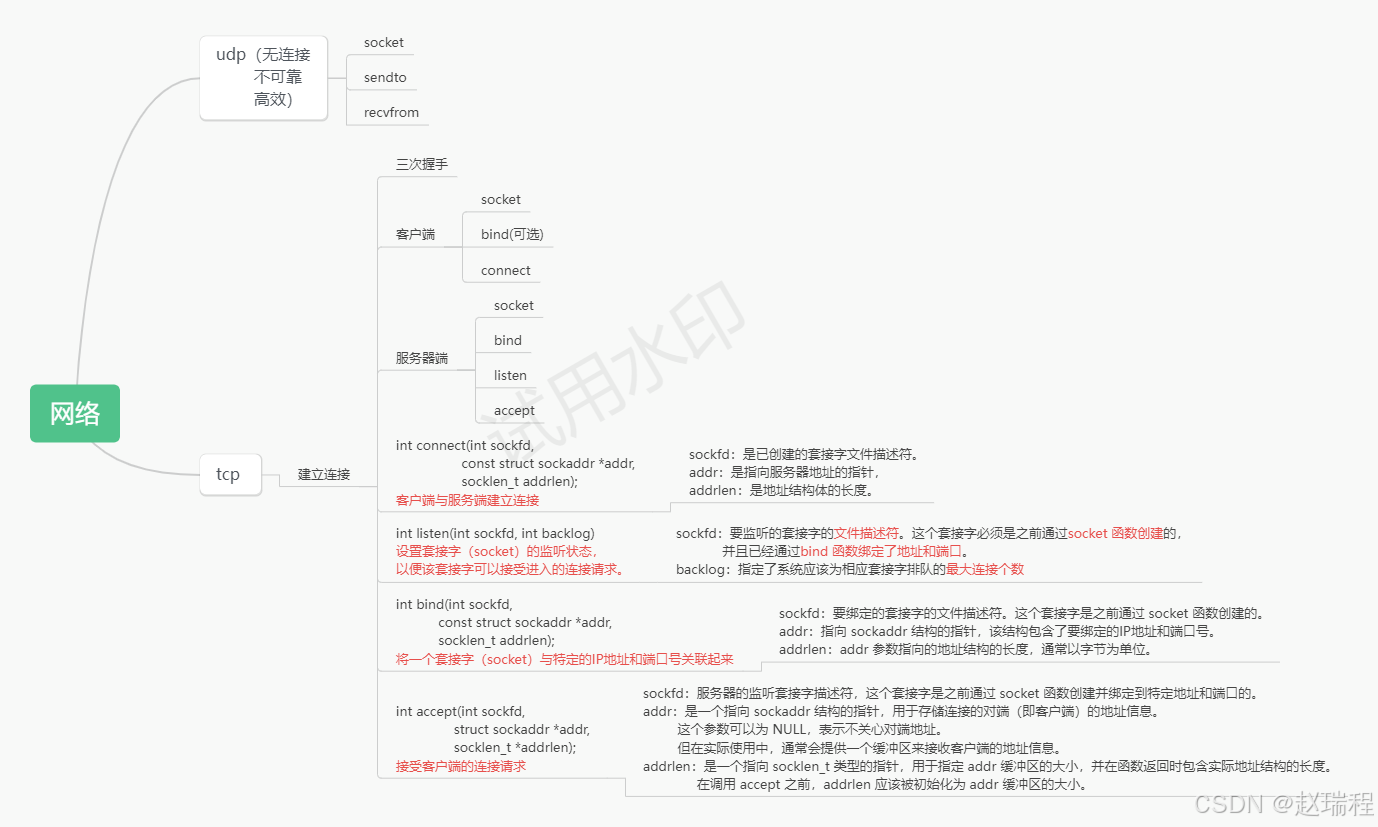
客户端
cs
/*************************************************************************
> File Name: client.c
> Author: yas
> Mail: [email protected]
> Created Time: Thu 22 Aug 2024 04:04:26 PM CST
************************************************************************/
#include<stdio.h>
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <arpa/inet.h>
#include <string.h>
#include <signal.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/wait.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <stdlib.h>
int c_fd;
int f_fd;
void handler(int signal)
{
close(f_fd);
kill(getpid(),'9');
wait(NULL);
}
int main(void)
{
int fd = socket(AF_INET, SOCK_STREAM,0);
if(fd == -1)
{
perror("socket fail");
return 1;
}
struct sockaddr_in seraddr;
seraddr.sin_family = AF_INET;
seraddr.sin_port = htons(50000);
seraddr.sin_addr.s_addr = inet_addr("192.168.149.128");
if((connect(fd,(const struct sockaddr*)&seraddr,sizeof(seraddr))) < 0)
{
perror("connect fail");
return 1;
}
char buf[1024];
pid_t pid = fork();
if(pid == -1)
{
perror("fork fail");
return 1;
}
if(pid > 0)
{
f_fd = fd;
while(1)
{
signal(SIGCHLD,handler);
fgets(buf,sizeof(buf),stdin);
buf[strlen(buf) - 1] = '\0';
write(fd,buf,strlen(buf) + 1);
if(strncmp(buf,"q",1) == 0)
{
kill(pid,'9');
break;
}
}
}
else if(pid == 0)
{
c_fd = fd;
while(1)
{
read(fd,buf,sizeof(buf));
printf("buf = %s\n", buf);
if(strncmp(buf,"q",1) == 0)
{
close(c_fd);
break;
}
}
}
return 0;
}
服务器端
cs
/*************************************************************************
> File Name: server.c
> Author: yas
> Mail: [email protected]
> Created Time: Thu 22 Aug 2024 05:22:36 PM CST
************************************************************************/
#include<stdio.h>
#include <stdio.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <arpa/inet.h>
#include <string.h>
#include <signal.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/wait.h>
#include <stdlib.h>
int f_fd;
int c_fd;
void handler(int signal)
{
wait(NULL);
close(f_fd);
kill(getpid(),'9');
}
int main(void)
{
int listenfd = socket(AF_INET, SOCK_STREAM,0);
if(listenfd == -1)
{
perror("socket fail");
return 1;
}
struct sockaddr_in seraddr;
seraddr.sin_family = AF_INET;
seraddr.sin_port = htons(50000);
seraddr.sin_addr.s_addr = inet_addr("192.168.149.128");
if((bind(listenfd,(const struct sockaddr*)&seraddr,sizeof(seraddr))) < 0)
{
perror("connect fail");
return 1;
}
if(listen(listenfd,5) < 0)
{
perror("listen fail");
return 1;
}
int connfd = accept(listenfd,NULL,NULL);
if(connfd == -1)
{
perror("connfd fail");
return 1;
}
printf("connfd = %d\n", connfd);
char buf[1024];
pid_t pid = fork();
if (pid == -1)
{
perror("fork fail");
return 1;
}
if(pid > 0)
{
f_fd = listenfd;
while(1)
{
signal(SIGCHLD,handler);
fgets(buf, sizeof(buf), stdin);
buf[strlen(buf) - 1] = '\0';
write(connfd,buf,strlen(buf) + 1);
if(strncmp(buf,"q",1) == 0)
{
kill(pid,'9');
break;
}
}
}
else if(pid == 0)
{
c_fd = listenfd;
while(1)
{
read(connfd,buf,sizeof(buf));
printf("buf = %s\n", buf);
if(strncmp(buf, "q",1) == 0)
{
close(c_fd);
break;
}
}
}
return 0;
}