目录
[1.string 类对象的部分修改操作](#1.string 类对象的部分修改操作)
前言
上篇博客我们对C++string的定义和一些函数接口做了讲解学习,接下来我们将继续对C++的函数进行学习。
1.string 类对象的部分修改操作
函数名称功能说明
push_back 在字符串后尾插字符c append 在字符串后追加一个字符串
operator+= (重点)在字符串后追加字符串
cpp
void string5() {
string s("Hello World");
string s1("I love you");
s.push_back('c');
cout << s << endl;
s.append(" and you");//字符串添加
cout << s << endl;
s1.append(s,6);//添加某字符串第n字符之后到另一字符串
cout << s1 << endl;
s1.append(5, 't');//添加n个字符
cout << s1 << endl;
s1.append("you", 2);//添加2个字符yo到s1
cout << s1 << endl;
string s2("Thanks");//加减字符串和字符
s2 += " you";
s2 += '!';
s2 += s;
cout << s2 << endl;
}
str c_str(重点)返回C格式字符串
string str;str.push_back(' '); // 在str后插入空格
str.append("hello"); // 在str后追加一个字符"hello"
str += 'w'; // 在str后追加一个字符'b'
str += "orld"; // 在str后追加一个字符串"it"
cout << str << endl;
cout << str.c_str() << endl; // 以C语言的方式打印字符串
find + npos(重 点) 从字符串pos位置开始往后找字符c, 返回该字符在字符串中的位置rfind从字符串pos位置开始往前找字符c,返回该字符在字符串中的位置
cpp
void string6() {
// 获取file的后缀
string file("string.cpp");
string s("test.cpp.zip");
size_t find1 = s.find('.');
size_t find2 = s.rfind('.');
cout << find1 << endl;
cout << find2 << endl;
size_t pos = file.rfind('.');
string suffix(file.substr(pos, file.size() - pos));
cout << suffix << endl;
// npos是string里面的一个静态成员变量
// static const size_t npos = -1;
}
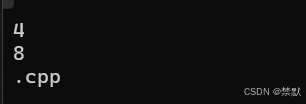
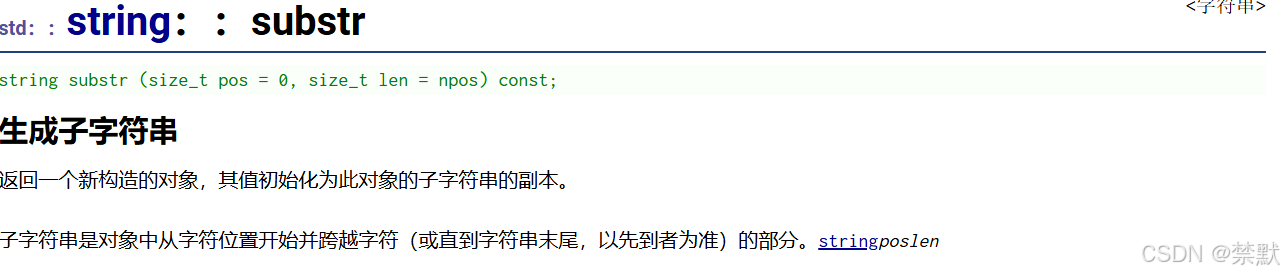
substr在str中从pos位置开始,截取n个字符,然后将其返回
cpp
#include <iostream>
#include <string>
using namespace std;
int main ()
{
string str="We think in generalities, but we live in details.";
string str2 = str.substr (3,5); // "think",第三个位置后的五个字符
size_t pos = str.find("live"); // position of "live" in str
string str3 = str.substr (pos); // get from "live" to the end
cout << str2 << ' ' << str3 << '\n';
return 0;
}

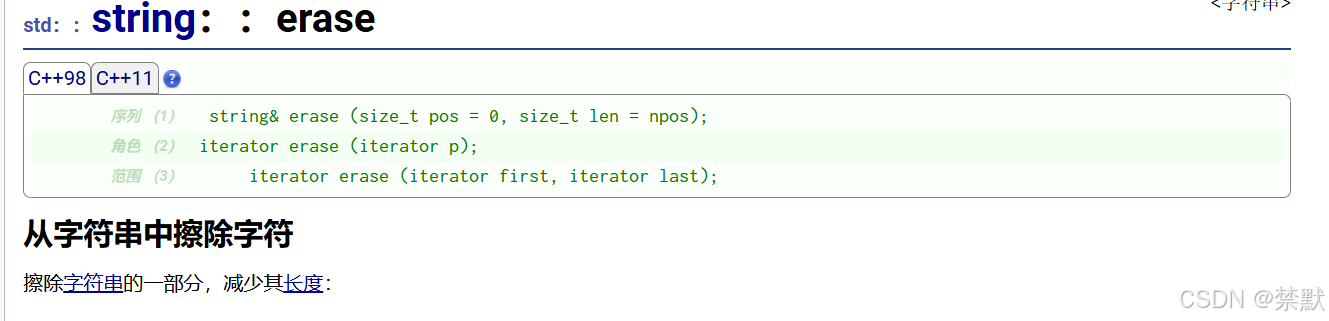
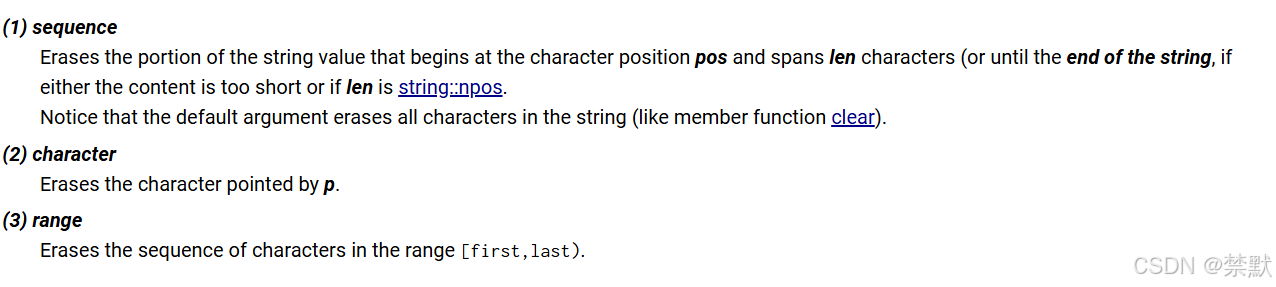
cpp
#include <iostream>
#include <string>
using namespace std;
int main ()
{
string str ("This is an example sentence.");
cout << str << '\n';
//删除第10个字符后面8个
str.erase (10,8);
cout << str << '\n';
// "This is an sentence."
str.erase (str.begin()+9); // 删除指向的字符 ^
cout << str << '\n';
// "This is a sentence."
str.erase (str.begin()+5, str.end()-9); //
cout << str << '\n';
// "This sentence."
string s("hello world"); // 头删
s.erase(0, 1);
cout << s << endl;
s.erase(s.begin());
cout << s << endl;
// 尾删
s.erase(--s.end());
cout << s << endl;
s.erase(s.size()-1, 1);
cout << s << endl;
return 0;
}
swap() 函数
-
功能:交换两个字符串的内容。
-
参数:接收两个std::string类型的参数。
-
返回值:无返回值。
`swap()`函数允许交换两个字符串的内容,而不会影响原始字符串。这是一个无返回值的函数,因此不能直接获取交换后的字符串。
replace() 函数
-
功能:用新的字符串替换字符串中的一个子串。
-
参数:
-
第一个参数:起始迭代器,指定要替换的子串的起始位置。
-
第二个参数:结束迭代器,指定要替换的子串的结束位置(不包括结束位置)。
-
第三个参数:新的字符串,用于替换子串。
-返回值:返回一个指向替换后的字符串的迭代器。
`replace()`函数允许用一个新的字符串替换字符串中的一个子串。如果新字符串的长度大于或等于旧子串的长度,它会替换整个子串;如果新字符串的长度小于旧子串的长度,它会在旧子串的末尾添加额外的空字符,以保持字符串的长度不变。
cpp
void string7() {
/*
string s1("hello world");
string s2("I love you");
string s3("thank you");
s1.swap(s2);
cout << s1 << endl;
s3.replace(0, s3.size(), "Programming");
cout << "After replace: " << s3 << std::endl;
*/
//替换空格为指定字符
string s("hello world and today!");
size_t pos = s.find(" ");
while (pos != string::npos) {
s.replace(pos, 1, "%%");
cout << s << endl;
pos = s.find(" ", pos + 2);
}
cout << s << endl;
//s.replace(5, 1, "%%");
}
void string8() {
string s("hello world and today!");
string temp;
temp.reserve(s.size());
for (auto ch :s) {
if (ch == ' ')
temp += "%%";
else
temp += ch;
}
//cout << temp << endl;
s. swap(temp);
cout << s << endl;
}
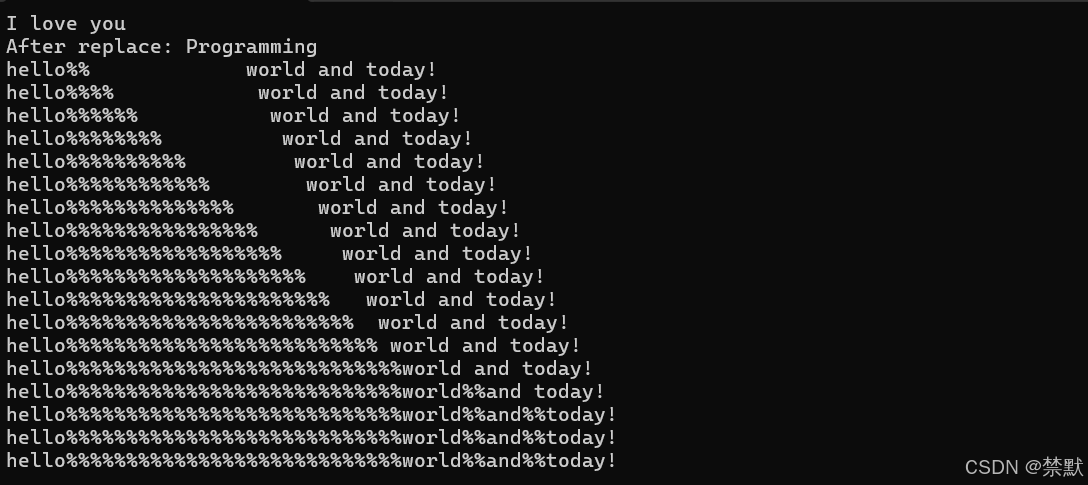
注意:replace参数的设置有很多种,对应了不同实现功能,可以通过上网查询资料进行学习。
补充了解

查询一段字符中的任意一个字符。
cpp
void string9() {
string str("Please, replace the vowels in this sentence by asterisks.");
/*
size_t found = str.find_first_not_of("abcdef");
while (found != string::npos) {
str[found] = '*';
found = str.find_first_not_of("abcdef", found + 1);
}
cout << str << endl;
*/
size_t found = str.find_first_of("abcdef");
while (found != string::npos) {
str[found] = '*';
found = str.find_first_of("abcdef", found + 1);
}
cout << str << endl;
}
abcdef都被替换成*
官网的样例:
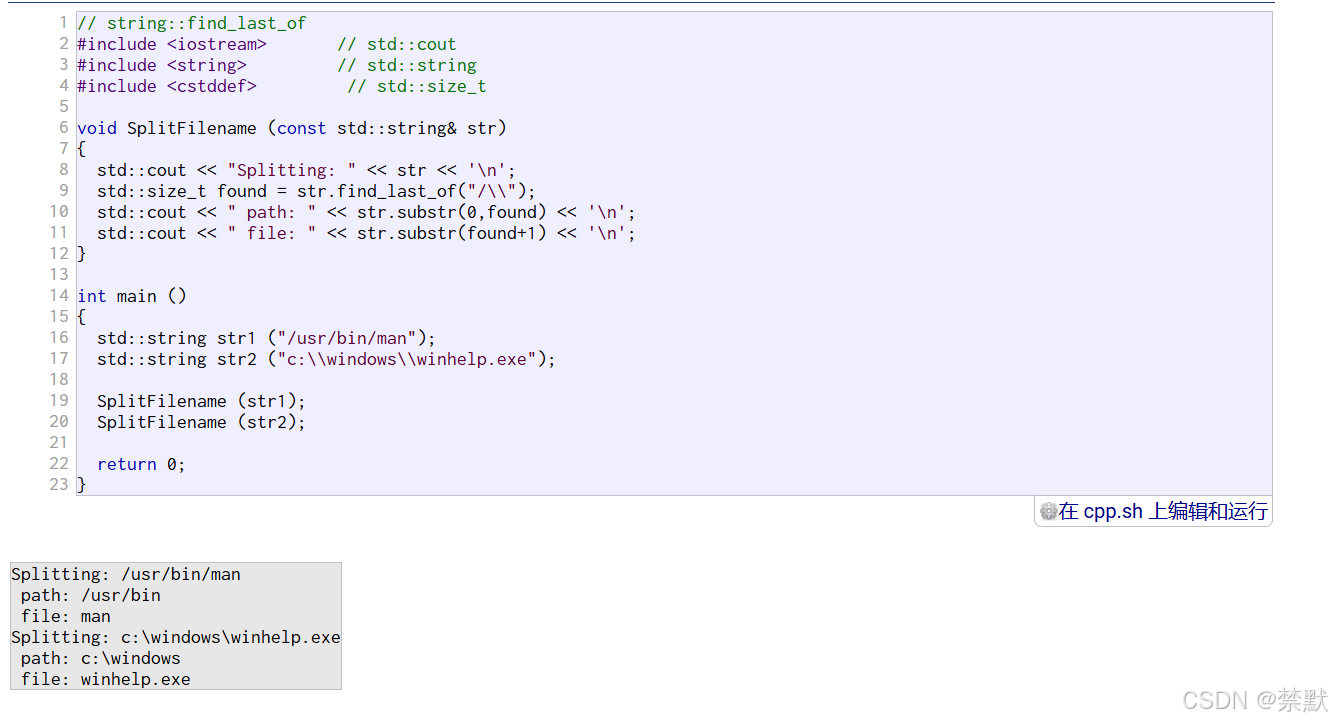
2.其他函数的部分讲解
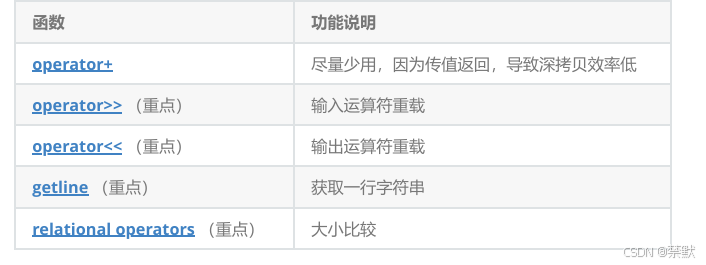
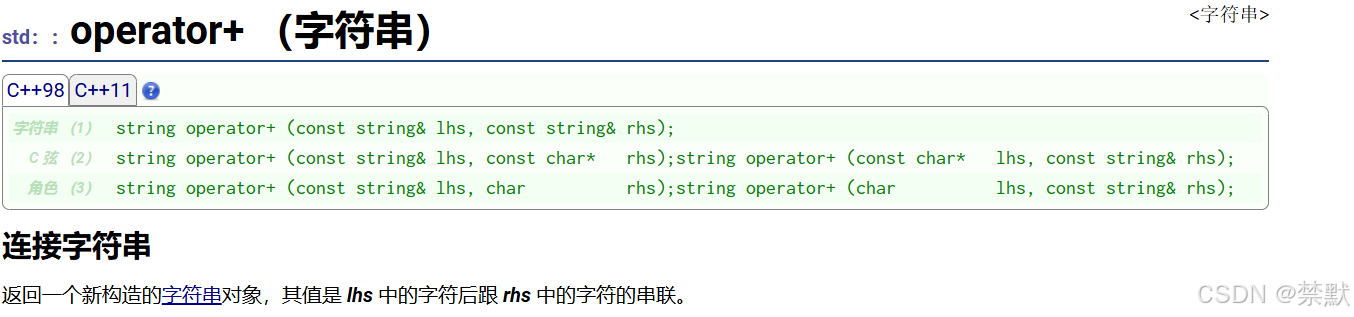
cpp
#include <iostream>
#include <string>
using namespace std;
int main(){
string s1("hello");
string s2("world");
s1=s1+s2;
cout<<s1<<endl;
s1=s1+"and bit";
cout<<s1<<endl;
return 0;
}
输入与输出运算符重载
cpp
#include <iostream>
#include <string>
using namespace std;
main ()
{
string name;
cout << "Please, enter your name: ";
cin >> name;
cout << "Hello, " << name << "!\n";
return 0;
}
请注意,istream 提取操作使用空格作为分隔符;因此,此操作只会从流中提取可被视为单词的内容
即当我们输入的数据有空格时,就相当于结束了,不会输出空格后面的了。

为了克服这种情况,C++引入了getline函数,可以读取空格。

cpp
void string11() {
string s;
cout << "please enter your name:" << endl;
getline(cin, s);
cout << "name:" << s << endl;
//自己设置终止符
string s1;
getline(cin, s1, '#');
cout << s1 << endl;
}
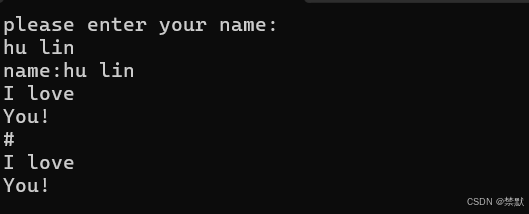
下面是官方的比较运算符的代码
cpp
// string comparisons
#include <iostream>
#include <vector>
int main ()
{
std::string foo = "alpha";
std::string bar = "beta";
if (foo==bar) std::cout << "foo and bar are equal\n";
if (foo!=bar) std::cout << "foo and bar are not equal\n";
if (foo< bar) std::cout << "foo is less than bar\n";
if (foo> bar) std::cout << "foo is greater than bar\n";
if (foo<=bar) std::cout << "foo is less than or equal to bar\n";
if (foo>=bar) std::cout << "foo is greater than or equal to bar\n";
return 0;
}
这个操作符大家可以在官网上进行查询了解,我就偷个懒哈哈!!!
结束语
本节内容就到此结束啦,下节我们将对string类的功能进行底层的模拟实现,最后感谢各位友友的支持!!!