《王者荣耀》也能用python面向对象编写
在Python中,面向对象编程(OOP)是一种编程范式,它将现实世界的事物抽象为类和对象。
英雄类(Hero)的定义
首先,我们可以定义一个英雄类,包含英雄的基本属性和方法
bash
class Hero:
def __init__(self, name, health, attack):
self.name = name
self.health = health
self.attackV = attack
def attack(self, target):
target.health -= self.attackV
print(f"{self.name } 攻击了 {target.name }, {target.name } 的生命值减少了 {self.attackV }。")
def __str__(self):
return f"英雄名称: {self.name }, 生命值: {self.health }, 攻击力: {self.attackV }"
创建英雄对象
接下来,我们可以创建几个具体的英雄对象,如李白、貂蝉等。
bash
wukong = Hero("孙悟空", 100, 20)
li_bai = Hero("李白", 120, 25)
英雄之间的互动
现在,我们可以让这些英雄对象进行互动,比如攻击对方。
bash
print(wukong) # 输出英雄的字符串表示形式print(li_bai)
wukong.attack (li_bai) # 孙悟空攻击李白
扩展英雄类
如果我们想要为英雄添加更多的功能,比如装备、技能等,我们可以通过继承来扩展英雄类。
bash
class MageHero(Hero):
def __init__(self, name, health, attack, skill_name): super().__init__(name, health, attack)
self.skill_name = skill_name
def cast_spell(self, enemy): # 施放法术
print(f"{self.name}对{enemy.name}施放了{self.skill_name}")
enemy.health -= self.attackV * 2 # 假设法术攻击力是普通攻击的两倍
zhou_yu = MageHero("周瑜", 2800, 450, "东风破")
zhou_yu.cast_spell(li_bai)
运行结果
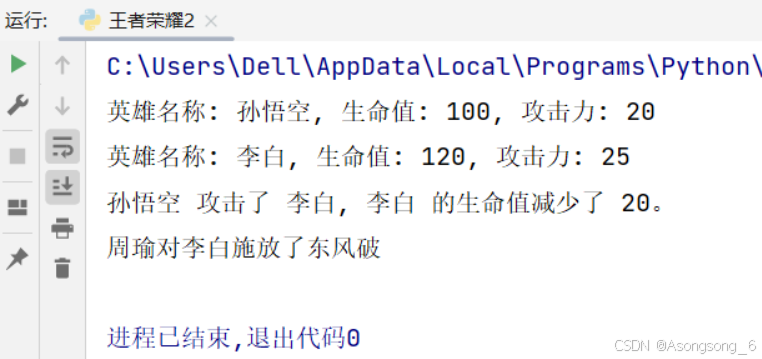