文章目录
- 1、创建demo工程
- 2、application.properties
- [3、Goods 实体类](#3、Goods 实体类)
- [4、EsDemoApplicationTests 测试类](#4、EsDemoApplicationTests 测试类)
- 5、pom.xml
- 6、查看索引库
- 7、查看单个索引(数据库)
- 8、从goods索引中检索出符合特定搜索条件的文档(或记录)
1、创建demo工程
2、application.properties
json
spring.application.name=es-demo
spring.elasticsearch.uris=http://192.168.74.148:9200
spring.elasticsearch.username=elastic
spring.elasticsearch.password=123456
3、Goods 实体类
java
package com.atguigu.es.demo.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Document(indexName = "goods")
public class Goods {
@Id
private Long id;
@Field(type = FieldType.Text,analyzer = "ik_max_word")
private String title;
@Field(type = FieldType.Keyword,index = false)
private String image;
@Field(type = FieldType.Integer)
private Integer price;
@Field(type = FieldType.Keyword)
private String brand;
@Field(type = FieldType.Keyword)
private String category;
}
4、EsDemoApplicationTests 测试类
java
package com.atguigu.es.demo;
import com.atguigu.es.demo.pojo.Goods;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.elasticsearch.client.elc.ElasticsearchTemplate;
import org.springframework.data.elasticsearch.core.IndexOperations;
@SpringBootTest
class EsDemoApplicationTests {
@Autowired
private ElasticsearchTemplate elasticsearchTemplate;
@Test
void contextLoads() {
// 获取Goods类对应的索引操作对象
IndexOperations indexOps = this.elasticsearchTemplate.indexOps(Goods.class);
// 创建索引
indexOps.create();
// 创建映射
indexOps.putMapping();
}
}
5、pom.xml
xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.0.5</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<!-- Generated by https://start.springboot.io -->
<!-- 优质的 spring/boot/data/security/cloud 框架中文文档尽在 => https://springdoc.cn -->
<groupId>com.atguigu</groupId>
<artifactId>es-demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>es-demo</name>
<description>es-demo</description>
<url/>
<licenses>
<license/>
</licenses>
<developers>
<developer/>
</developers>
<scm>
<connection/>
<developerConnection/>
<tag/>
<url/>
</scm>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
6、查看索引库

7、查看单个索引(数据库)
json
GET /goods
json
{
"goods": {
"aliases": {},
"mappings": {
"properties": {
"_class": {
"type": "keyword",
"index": false,
"doc_values": false
},
"brand": {
"type": "keyword"
},
"category": {
"type": "keyword"
},
"image": {
"type": "keyword",
"index": false
},
"price": {
"type": "integer"
},
"title": {
"type": "text",
"analyzer": "ik_max_word"
}
}
},
"settings": {
"index": {
"routing": {
"allocation": {
"include": {
"_tier_preference": "data_content"
}
}
},
"refresh_interval": "1s",
"number_of_shards": "1",
"provided_name": "goods",
"creation_date": "1724723254472",
"number_of_replicas": "1",
"uuid": "vBsgfYlfRjKs6uSISXbxtw",
"version": {
"created": "8080299"
}
}
}
}
}
8、从goods索引中检索出符合特定搜索条件的文档(或记录)
json
GET /goods/_search
json
{
"took": 2,
"timed_out": false,
"_shards": {
"total": 1,
"successful": 1,
"skipped": 0,
"failed": 0
},
"hits": {
"total": {
"value": 0,
"relation": "eq"
},
"max_score": null,
"hits": []
}
}
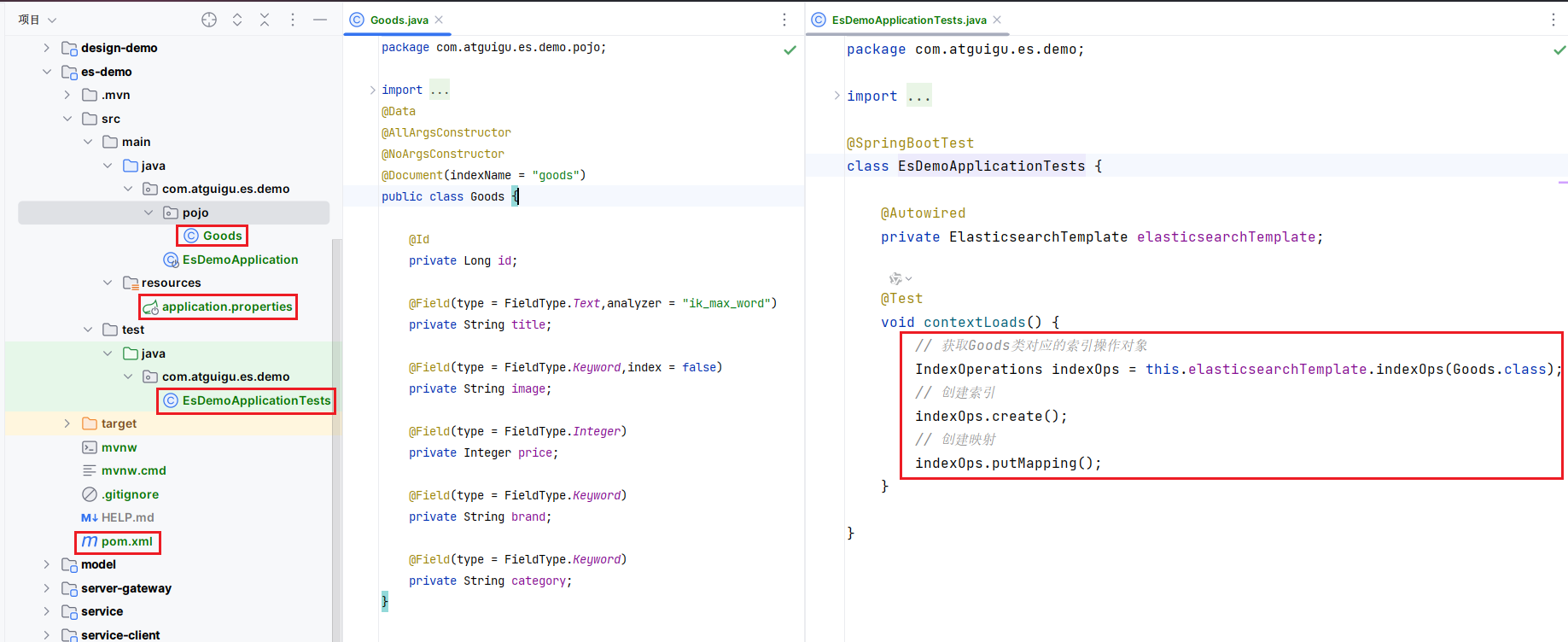