前言:
elasticsearch的整合流程可以参考:Elasticsearch7.15版本后新版本的接入-CSDN博客
索引
1.创建索引
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
boolean exists = elasticsearchClient.indices().exists(query -> query.index("new_ceshi")).value();
System.out.println(exists);
if (exists) {
System.out.println("已存在");
} else {
final CreateIndexResponse products = elasticsearchClient.indices().create(builder -> builder.index("new_ceshi"));
System.out.println(products.acknowledged());
}
}
2.查询索引
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
GetIndexResponse products = elasticsearchClient.indices().get(query -> query.index("new_ceshi"));
System.out.println(products.toString());
}
3.删除索引
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
boolean exists = elasticsearchClient.indices().exists(query -> query.index("new_ceshi")).value();
System.out.println(exists);
if (exists) {
DeleteIndexResponse response = elasticsearchClient.indices().delete(query -> query.index("new_ceshi"));
System.out.println(response.acknowledged());
} else {
System.out.println("索引不存在");
}
}
索引映射
4.查询索引的映射
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
GetIndexResponse response = elasticsearchClient.indices().get(builder -> builder.index("new_bank"));
System.out.println(response.result().get("new_bank").mappings().toString());
}
5.创建索引以及初始化索引映射
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
elasticsearchClient.indices()
.create(builder -> builder.index("new_product")
.mappings(map -> map.properties("name", p -> p.text(textProperty -> textProperty.analyzer("ik_max_word").searchAnalyzer("ik_max_word")))
.properties("intro", p -> p.text(textProperty -> textProperty.analyzer("ik_max_word").searchAnalyzer("ik_max_word")))
.properties("stock", p -> p.integer(integerProperty -> integerProperty)))
);
}
文档
6.创建文档-自定义类数据存储容器
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
Produce produce = new Produce("饼干", "上好的饼干", 2000);
IndexResponse response = elasticsearchClient.index(builder -> builder.index("new_product").id("1").document(produce));
System.err.println(response.version());
}
结果:
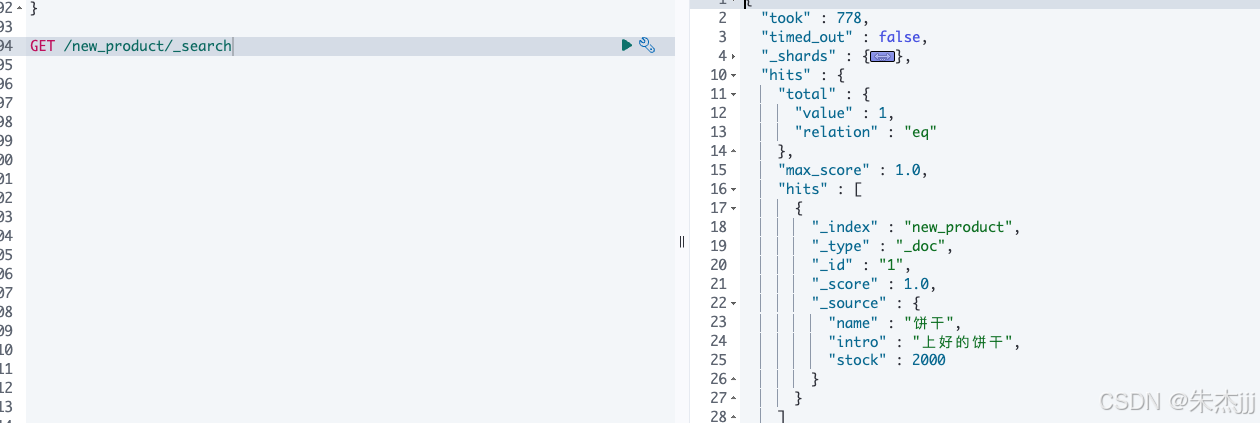
7.创建文档-HashMap存储容器
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
HashMap<String, Object> doc = new HashMap<>();
doc.put("name","油条");
doc.put("intro","纯油炸的油条");
doc.put("stock","999");
final IndexResponse response = elasticsearchClient.index(builder -> builder.index("new_product").id("2").document(doc));
}
8.查询所有文档
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
SearchResponse<Object> response = elasticsearchClient.search(builder -> builder.index("new_product"), Object.class);
List<Hit<Object>> hits = response.hits().hits();
hits.forEach(
x-> System.out.println(x.toString())
);
}
9.查询某个id的文档
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
GetRequest new_product = new GetRequest.Builder()
.index("new_product")
.id("1")
.build();
GetResponse<Object> objectGetResponse = elasticsearchClient.get(new_product, Object.class);
System.out.printf("objectGetResponse=========="+objectGetResponse.source());
}
10删除文档
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
//删除文档
DeleteRequest new_product = new DeleteRequest.Builder()
.index("new_product")
.id("1")
.build();
DeleteResponse delete = elasticsearchClient.delete(new_product);
System.out.printf("delete==========" + delete);
}
11.更新文档-自定义类
全更新
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
Produce produce = new Produce("铁锤", "全刚的大铁锤", 666);
UpdateResponse<Produce> new_product = elasticsearchClient.update(builder -> builder.index("new_product").id("2").doc(produce), Produce.class);
System.err.println(new_product.shards().successful());
}
指定字段修改.docAsUpsert(true)
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
Produce produce = new Produce();
produce.setName("小铁锤");
UpdateResponse<Produce> new_product = elasticsearchClient.update(builder -> builder.index("new_product").id("2").docAsUpsert(true).doc(produce), Produce.class);
System.err.println(new_product.shards().successful());
}
12更新文档-Map更新
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
Map<String, Object> updateJson = new HashMap<>();
updateJson.put("name","巨大铁锤");
UpdateRequest<Object, Object> updateRequest = new UpdateRequest.Builder<>()
.index("new_product")
.id("2")
.doc(updateJson)
.build();
UpdateResponse<Object> updateResponse = elasticsearchClient.update(updateRequest, Object.class);
System.out.println("Document updated: " + updateResponse.result());
}
批量操作
13批量添加
java
@Test
public void contextLoads() throws IOException {
ElasticsearchClient elasticsearchClient = elasticSearchConfig.esRestClient();
List<SkuEsModel> skuEsModels = new ArrayList<>();
BulkRequest.Builder br = new BulkRequest.Builder();
for (SkuEsModel skuEsModel : skuEsModels) {
br.operations(op->op.index(idx->idx.index("produces").id(String.valueOf(skuEsModel.getSkuId())).document(skuEsModel)));
}
BulkResponse response = elasticsearchClient.bulk(br.build());
}
复杂检索请查看:elasticsearch复杂检索,match、matchAll、matchPhrase、term、多条件查询、multiMatch多字段查询等