1. ==运算符
- ==是一个运算符,其用于比较两个变量的内存地址是否相等;
- 对于基本数据类型(int、char、Boolean等),==比较的是它们的值;
- 而对于引用数据类型的话(String、Object、ArrayList等),==比较的是引用,也就是对象在内存中的地址,即检查两个引用是否指向堆内存中的同一个对象实例。
代码举例:
java
public class Main {
public static void main(String[] args) {
int a = 100;
int b = 100;
System.out.println(a == b);
String str1 = new String("hello");
String str2 = new String("hello");
System.out.println(str1 == str2);
}
}
第一次输出结果为true,因为a和b都是基本数据类型,==比较的是他们两个的值是否相等;第二次的输出结果为false,因为str1和str2都是引用数据类型,==比较的是他们两个在内存中的地址。
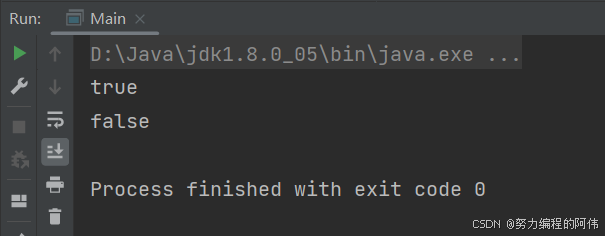
2. equals()方法
equals()是Object类中的方法,默认实现的是比较两个对象的内存地址是否相等,即与==相同。
许多类(如String、Integer、List等)重写了equals(方法),用于比较对象的内容是否相等,而不仅仅是内存地址。
在Java中,如果两个对象的内存地址相等,即它们是同一个对象的引用,那么它们的内容也一定是相等的。这是因为内存地址相等意味着它们指向堆内存中的同一个对象实例。相反,如果两个对象的内容相等,并不意味着它们的内存地址一定相等,除非它们是同一个对象的引用。
代码举例:
java
public class Main {
public static void main(String[] args) {
String str1 = new String("hello");
String str2 = new String("hello");
System.out.println(str1.equals(str2));
Integer num1 = new Integer(666);
Integer num2 = new Integer(666);
System.out.println(num1.equals(num2));
}
}
两次运行结果均为true;
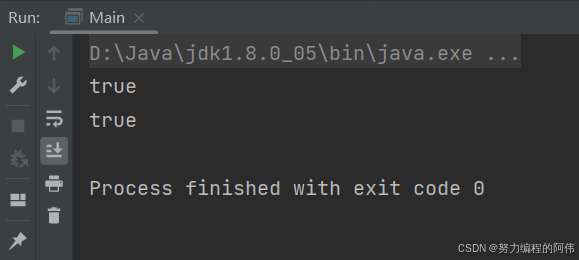
3. 适用场景
- ==更适合于比较基本数据类型,或者是判断两个对象是否是同一个引用;
- equals()更适合用于比较两个对象的内容是否相等。
4.常见错误
初学者很容易误用==来比较字符串或对象的内容,从而导致了比较结果不符合预期。