1.体验
1.需求
验证QQ号是否合法,首位不能是0,长度6-20,必须纯数字
2.没有正则表达式
java
package cn.hdc.opp6.zhengzebiaodashi;
public class Main1 {
public static void main(String[] args) {
System.out.println(checkQQ("2652110187"));
}
public static boolean checkQQ(String QQ) {
if (QQ == null || QQ.charAt(0) == '0' || QQ.length() < 6 || QQ.length() > 20) {
return false;
}
for (int i = 0; i < QQ.length(); i++) {
if (QQ.charAt(i) < 48 || QQ.charAt(i) > 57) {
return false;
}
}
return true;
}
}
3.使用正则表达式
java
package cn.hdc.opp6.zhengzebiaodashi;
public class Main1 {
public static void main(String[] args) {
System.out.println(checkQQ1("2652110187"));
}
public static boolean checkQQ1(String QQ) {
return QQ != null && QQ.matches("[1-9]\\d{5,19}");
}
}
2.正则表达式
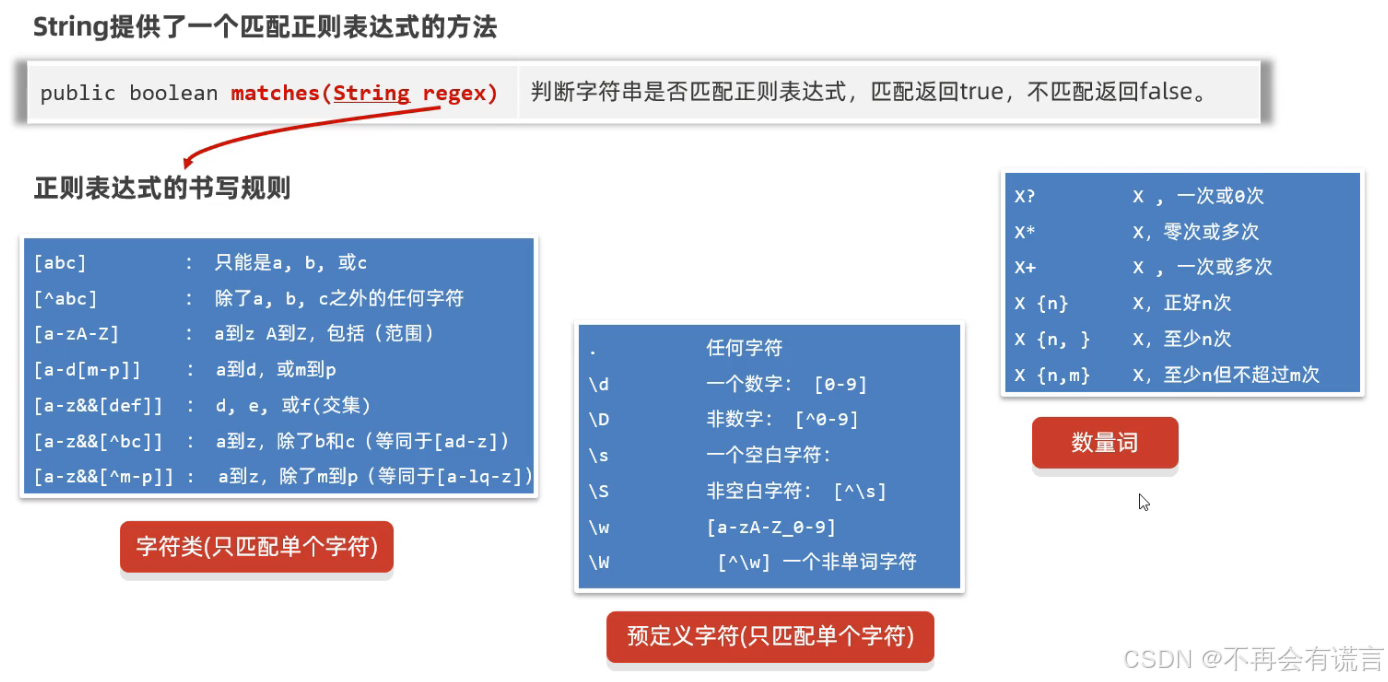
案例

java
package cn.hdc.opp6.ZhengZedemo;
import java.util.Scanner;
public class d1 {
public static void main(String[] args) {
checkPhone();
}
private static void checkPhone() {
while (true) {
System.out.println("请输入你的时间:");
Scanner sc = new Scanner(System.in);
String phone = sc.nextLine();
// if (phone.matches("[0-2]?[0-3]点[0-5]?[0-9]分[0-5]?[0-9]秒")) {
if (phone.matches("([01]?[0-9]|2[0-3])点([0-5]?[0-9])分([0-5]?[0-9])秒")) {
System.out.println(true);
// break;
} else {
System.out.println(false);
}
}
/*
while (true) {
System.out.println("请输入你的邮箱:");
Scanner sc = new Scanner(System.in);
String phone = sc.nextLine();
if (phone.matches("\\w{2,}@[a-zA-Z0-9]{2,20}(\\.\\w{2,10}){1,2}")) {
System.out.println(true);
break;
} else {
System.out.println(false);
}
}
*/
/*
while (true) {
System.out.println("请输入你的手机号:");
Scanner sc = new Scanner(System.in);
String phone = sc.nextLine();
if (phone.matches("(1[3-9]\\d{9})|(0\\d{2,7}-?[1-9]\\d{4,19})")) {
System.out.println(true);
break;
} else {
System.out.println(false);
}
}
*/
}
}
爬取信息
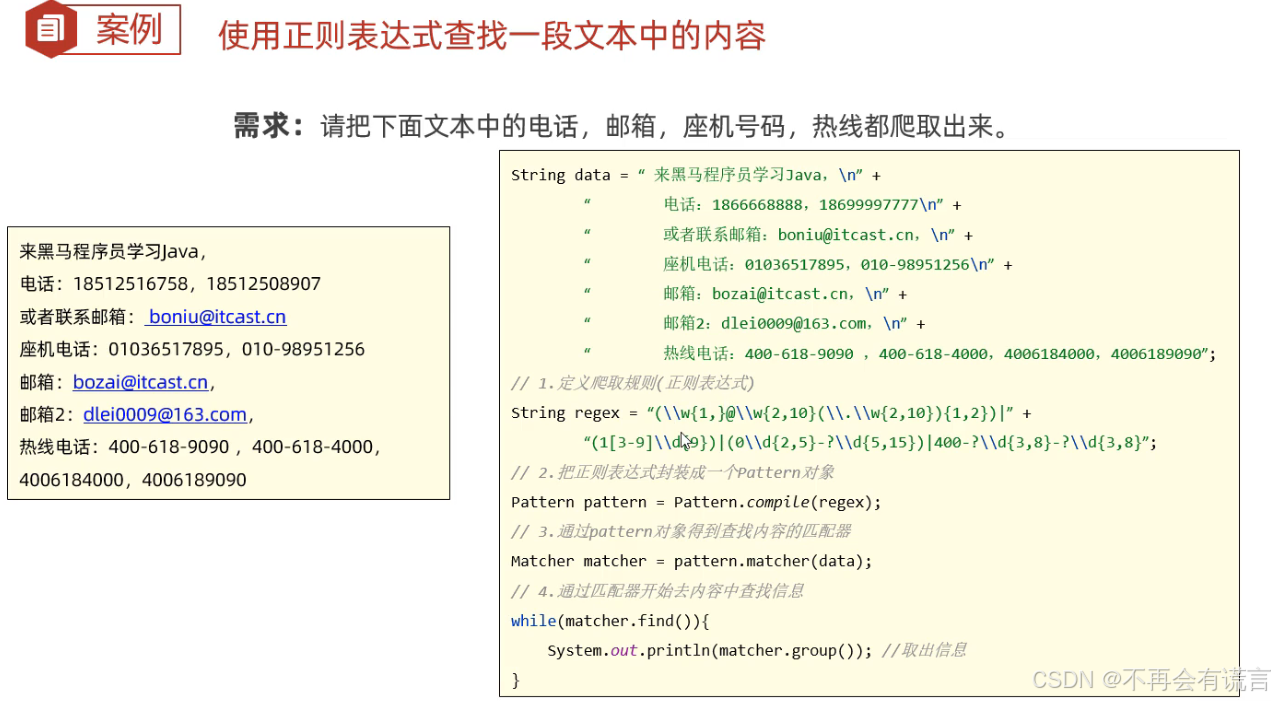
java
package cn.hdc.opp6.ZhengZeSelect;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class Main {
public static void main(String[] args) {
queryInfo();
}
private static void queryInfo() {
String str =
"来黑马程序员学习Java\n" +
"电话:18512516758,18512508907\n" +
"或者联系邮箱: boniu@itcast.cn\n" +
"座机电话:01036517895,010-98951256\n" +
"邮箱:bozai@itcast.cn\n" +
"邮箱2:dlei0009@163.com\n" +
"热线电话:400-618-9090,400-618-4000\n" +
"4006184000,4006189090";
//爬取电话
String regex =
"(1[3-9]\\d{9})|" +
//爬取邮箱
"(\\w{1,}@\\w{2,10}\\.(\\w{2,10}){1,2})|" +
//爬取座机号码
"010[- ]?\\d{3,8}|" +
//爬取热线
"400[- ]?\\d{3,8}[- ]?\\d{3,8}";
Pattern p = Pattern.compile(regex);
Matcher m = p.matcher(str);
while (m.find()) {
System.out.println(m.group());
}
}
}
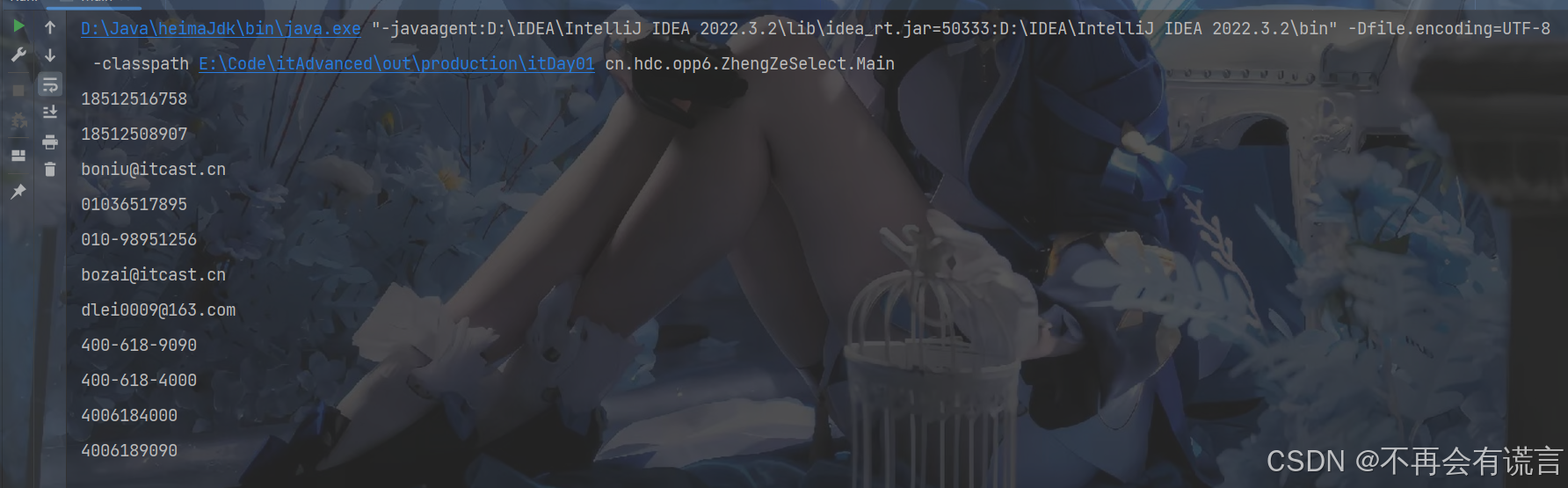
搜索、替换内容
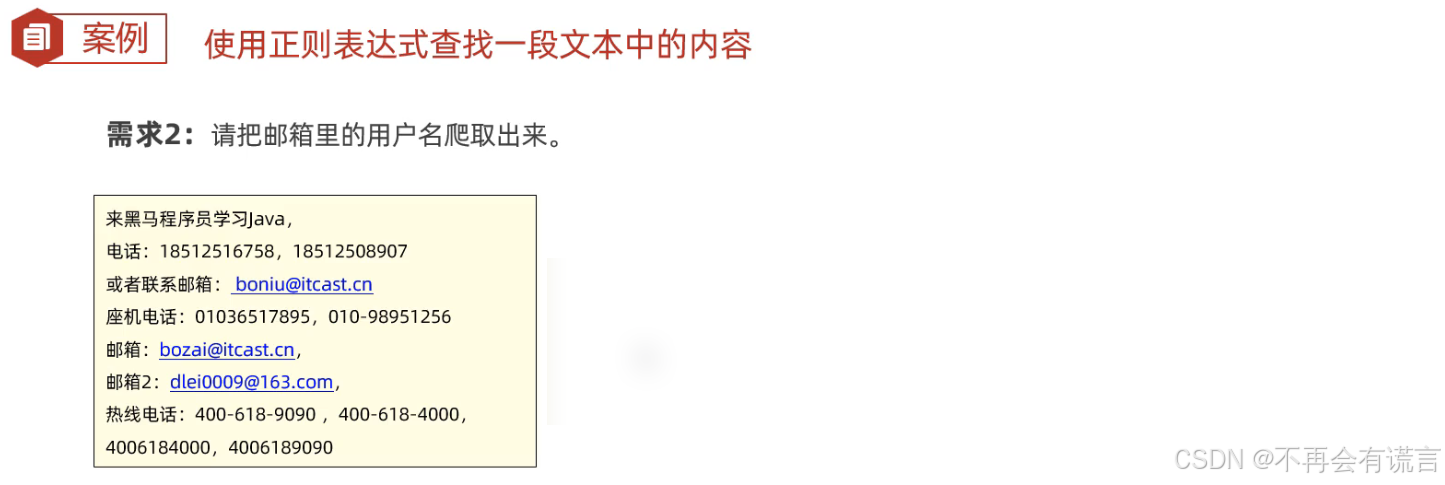
java
private static void queryUserName() {
String str = "来黑马程序员学习Java\n" +
"电话:18512516758,18512508907\n" +
"或者联系邮箱: boniu@itcast.cn\n" +
"座机电话:01036517895,010-98951256\n" +
"邮箱:bozai@itcast.cn\n" +
"邮箱2:dlei0009@163.com\n" +
"热线电话:400-618-9090,400-618-4000\n" +
"4006184000,4006189090";
String regex = "\\w{1,}@\\w{2,10}(\\.\\w{2,10}){1,2}";
Pattern p = Pattern.compile(regex);
Matcher m = p.matcher(str);
while (m.find()) {
String res = m.group().split("@")[0];
System.out.println(res);
}
}
java
package cn.hdc.opp6.ZhengZeSelect;
import java.util.Arrays;
public class ReplaceAndSplit {
public static void main(String[] args) {
//1、public String replaceAll(String regex,String newstr):按照正则表达式匹配的内容进行替换
// 需求1:请把 古力娜扎ai8888迪丽热巴999aa5566马尔扎哈fbbfsfs42425卡尔扎巴,中间的非中文字符替换成"_"
String str = "古力娜扎ai8888迪丽热巴999aa5566马尔扎哈fbbfsfs42425卡尔扎巴";
String regex = "\\w+";
System.out.println(str.replaceAll(regex, "-"));
// 需求2(拓展):某语音系统,收到一个口吃的人说的 "我我我喜欢编编编编编编编编编编编编程程程!",需要优化成 "我喜欢编程!"
String str1 = "我我我喜欢编编编编编编编编编编编编程程程!";
String reges1 = "(.)\\1+";
/**
* (.)代表任意一组,.代表匹配任意字符
* \\1 :为这个组声明一个组号:1号(一定用\\1,这是规定好的)
* + :声明必须是重复的字:一次以上
* $1 :取到第一组代表的那个重复的字
*/
System.out.println(str1.replaceAll(reges1, "$1"));
// 2、public string[] split(String regex):按照正则表达式匹配的内容进行分割字符串,反回一个字符串数组。
//需求1:请把古力娜扎ai8888迪丽热巴999aa5566马尔扎哈fbbfsfs42425卡尔扎巴,中的人名获取出来。
String str2 = "古力娜扎ai8888迪丽热巴999aa5566马尔扎哈fbbfsfs42425卡尔扎巴";
String regex2 = "\\w+";
System.out.println(Arrays.toString(str2.split(regex2)));
}
}