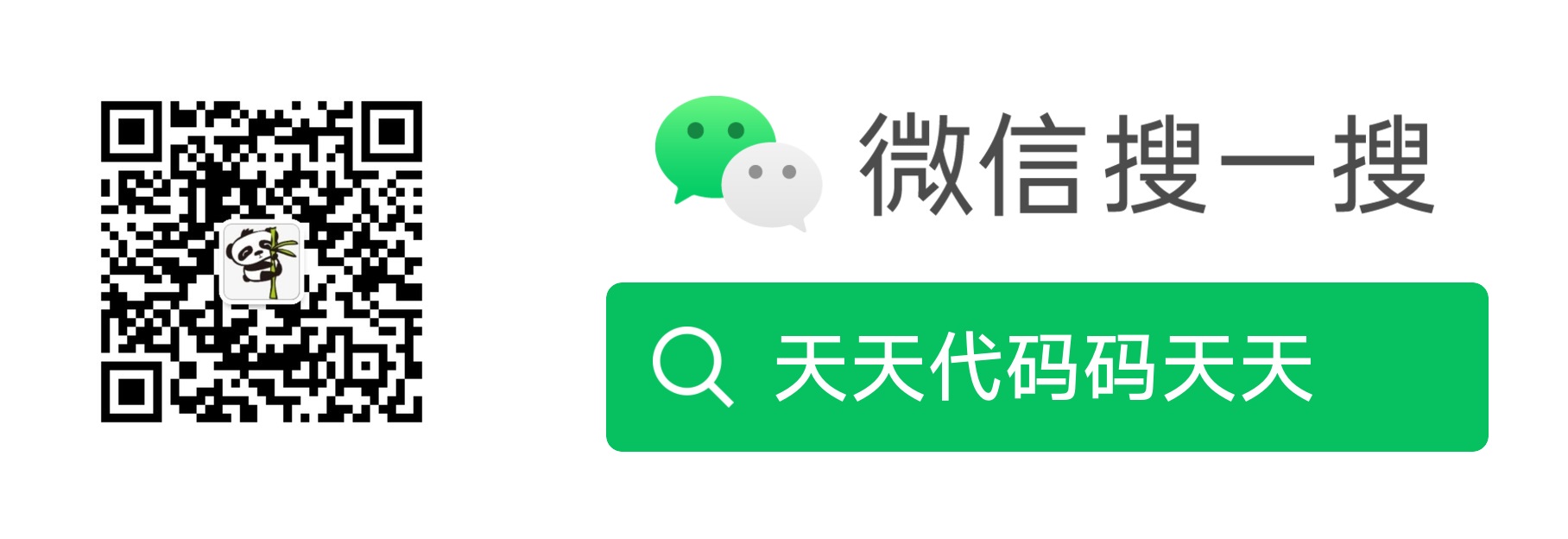
目录
效果
SM2
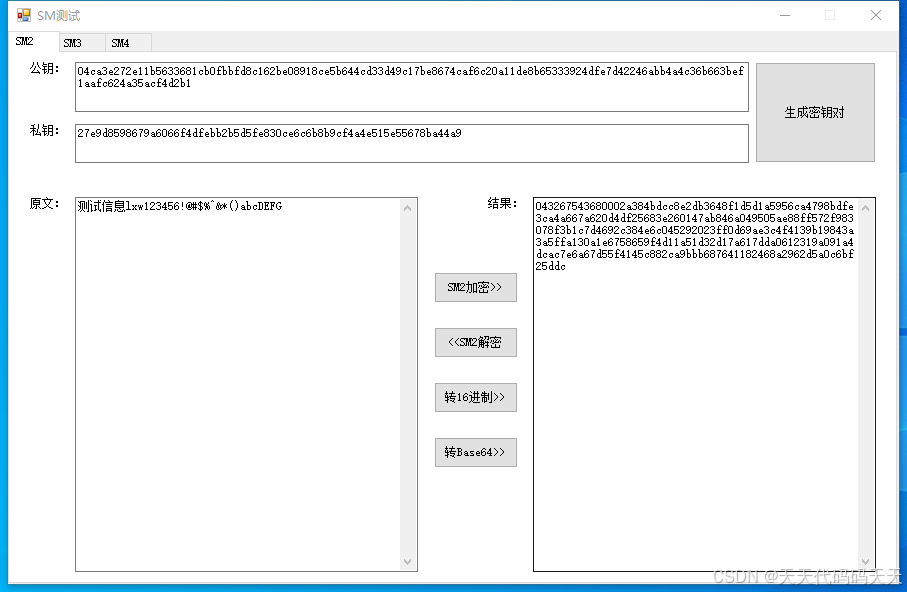
公钥:04ca3e272e11b5633681cb0fbbfd8c162be08918ce5b644cd33d49c17be8674caf6c20a11de8b65333924dfe7d42246abb4a4c36b663bef1aafc624a35acf4d2b1
私钥:27e9d8598679a6066f4dfebb2b5d5fe830ce6c6b8b9cf4a4e515e55678ba44a9
原文:测试信息lxw123456!@#$%^&*()abcDEFG
结果:043267543680002a384bdcc8e2db3648f1d5d1a5956ca4798bdfe3ca4a667a620d4df25683e260147ab846a049505ae88ff572f983078f3b1c7d4692c384e6c045292023ff0d69ae3c4f4139b19843a3a5ffa130a1e6758659f4d11a51d32d17a617dda0612319a091a4dcac7e6a67d55f4145c882ca9bbb687641182468a2962d5a0c6bf25ddc
在线校验
地址:https://the-x.cn/cryptography/Sm2.aspx
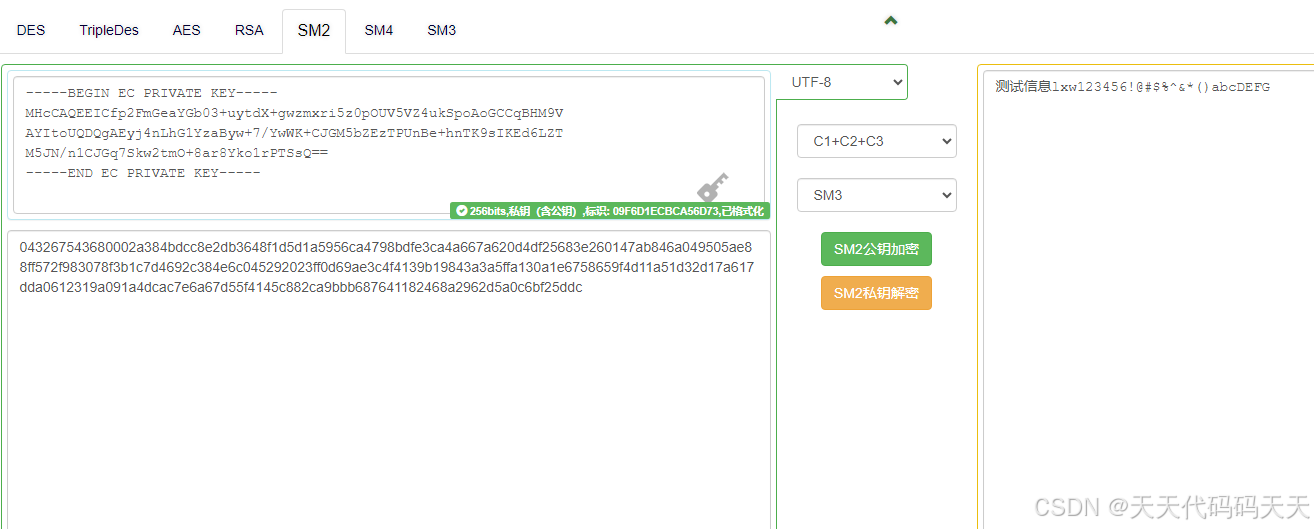
SM3
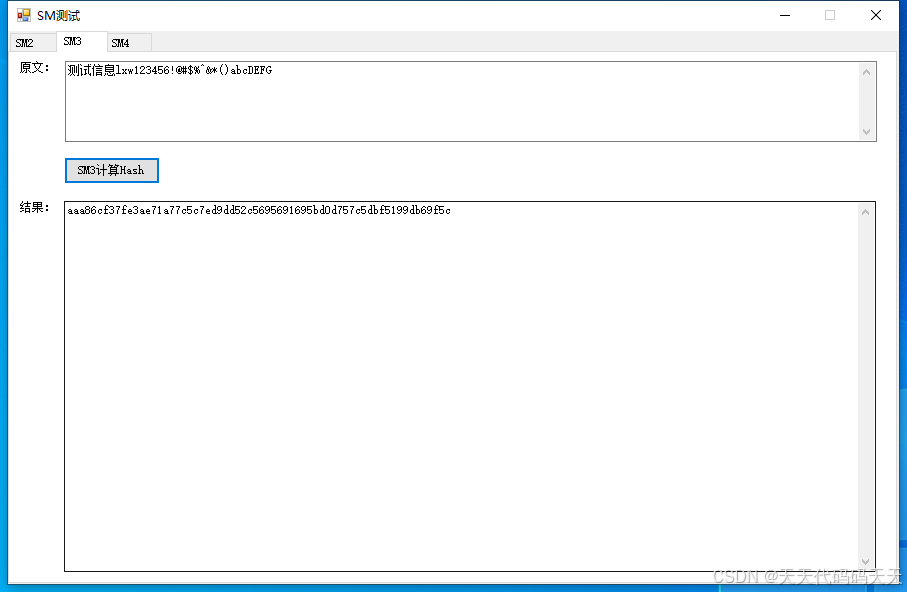
SM4
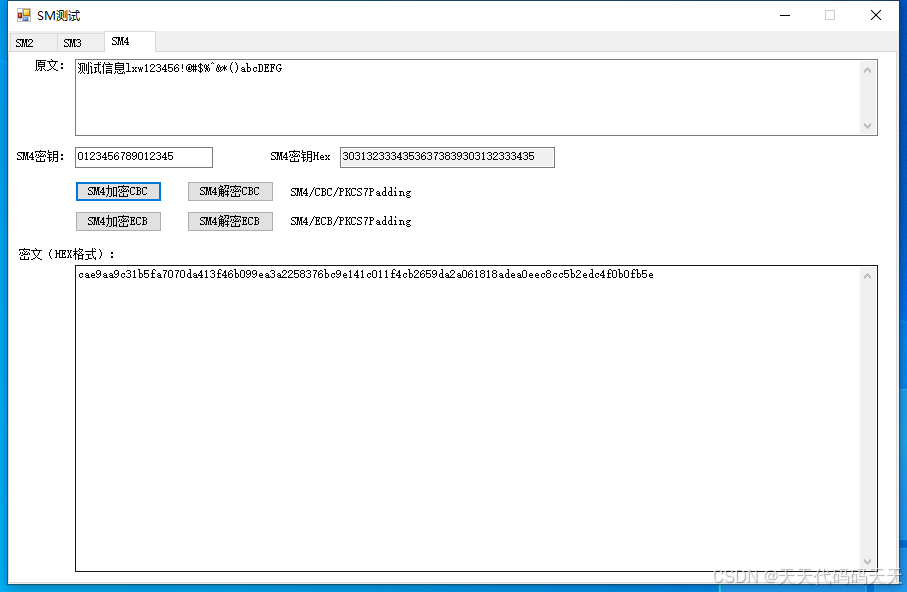
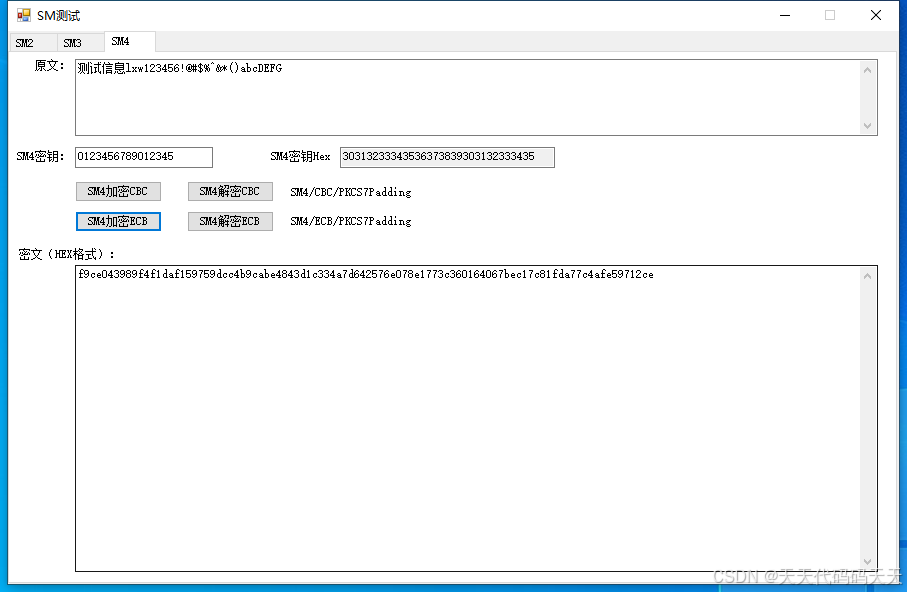
项目
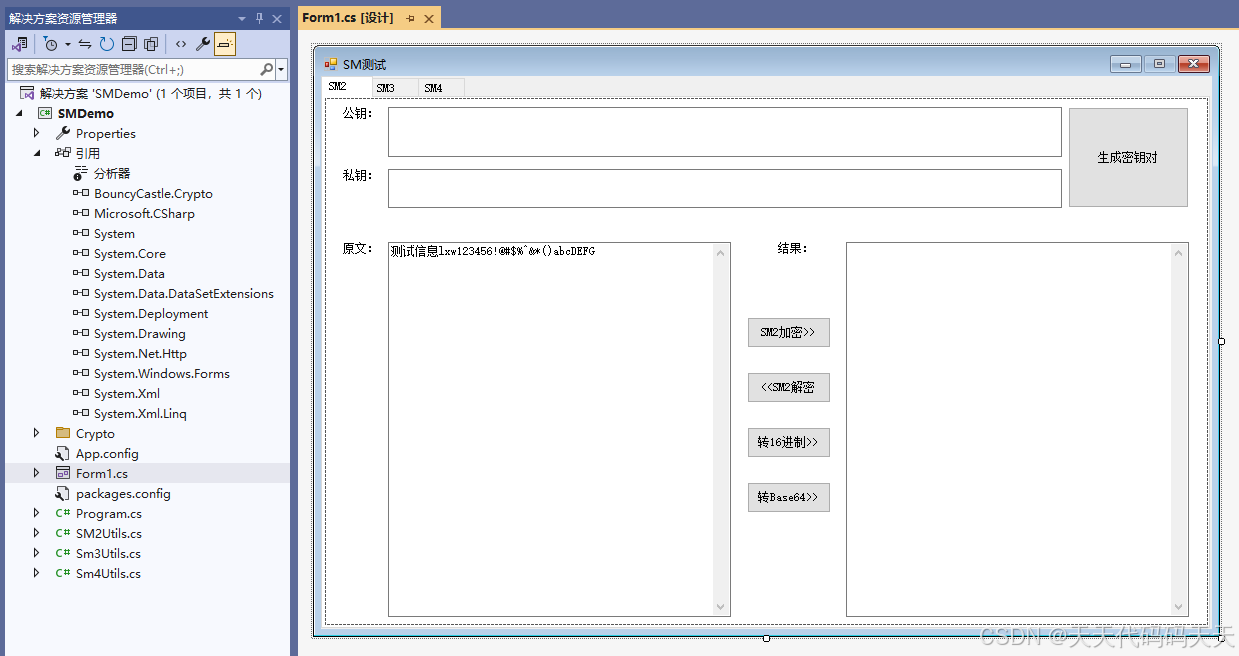
代码
SM2Utils.cs
using Org.BouncyCastle.Crypto;
using Org.BouncyCastle.Crypto.Engines;
using Org.BouncyCastle.Crypto.Generators;
using Org.BouncyCastle.Crypto.Parameters;
using Org.BouncyCastle.Math;
using Org.BouncyCastle.Math.EC;
using Org.BouncyCastle.Security;
using Org.BouncyCastle.Utilities.Encoders;
using System;
using System.Text;
namespace SMDemo
{
public class SM2KeyPair
{
public string PrivateKey { get; set; } //私钥
public string PublicKey { get; set; } //公钥
}
public class SM2Utils
{
//国密标准256位曲线参数
BigInteger SM2_ECC_P = new BigInteger("FFFFFFFEFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFF00000000FFFFFFFFFFFFFFFF", 16);
BigInteger SM2_ECC_A = new BigInteger("FFFFFFFEFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFF00000000FFFFFFFFFFFFFFFC", 16);
BigInteger SM2_ECC_B = new BigInteger("28E9FA9E9D9F5E344D5A9E4BCF6509A7F39789F515AB8F92DDBCBD414D940E93", 16);
BigInteger SM2_ECC_N = new BigInteger("FFFFFFFEFFFFFFFFFFFFFFFFFFFFFFFF7203DF6B21C6052B53BBF40939D54123", 16);
BigInteger SM2_ECC_H = BigInteger.One;
BigInteger SM2_ECC_GX = new BigInteger("32C4AE2C1F1981195F9904466A39C9948FE30BBFF2660BE1715A4589334C74C7", 16);
BigInteger SM2_ECC_GY = new BigInteger("BC3736A2F4F6779C59BDCEE36B692153D0A9877CC62A474002DF32E52139F0A0", 16);
public SM2KeyPair GenerateKey()
{
ECCurve curve = new FpCurve(SM2_ECC_P, SM2_ECC_A, SM2_ECC_B, SM2_ECC_N, SM2_ECC_H);
ECPoint g = curve.CreatePoint(SM2_ECC_GX, SM2_ECC_GY);
ECDomainParameters domainParams = new ECDomainParameters(curve, g, SM2_ECC_N);
ECKeyPairGenerator keyPairGenerator = new ECKeyPairGenerator();
ECKeyGenerationParameters aKeyGenParams = new ECKeyGenerationParameters(domainParams, new SecureRandom());
keyPairGenerator.Init(aKeyGenParams);
AsymmetricCipherKeyPair aKp = keyPairGenerator.GenerateKeyPair();
ECPublicKeyParameters aPub = (ECPublicKeyParameters)aKp.Public;
ECPrivateKeyParameters aPriv = (ECPrivateKeyParameters)aKp.Private;
BigInteger privateKey = aPriv.D;
ECPoint publicKey = aPub.Q;
byte[] pubkey = Hex.Encode(publicKey.GetEncoded());
string temp_pubkey = Encoding.UTF8.GetString(pubkey);
string temp_prikey = Encoding.UTF8.GetString(Hex.Encode(privateKey.ToByteArray()));
return new SM2KeyPair() { PrivateKey = temp_prikey, PublicKey = temp_pubkey };
}
/// <summary>
/// 加密函数
/// </summary>
/// <param name="publicKeyStr"></param>
/// <param name="plainText"></param>
/// <returns></returns>
public string Encrypt(string publicKeyStr, string plainText)
{
byte[] publicKey = Hex.Decode(publicKeyStr);
byte[] data = Encoding.UTF8.GetBytes(plainText);
if (null == publicKey || publicKey.Length == 0)
{
return null;
}
if (data == null || data.Length == 0)
{
return null;
}
byte[] source = new byte[data.Length];
Array.Copy(data, 0, source, 0, data.Length);
ECCurve curve = new FpCurve(SM2_ECC_P, SM2_ECC_A, SM2_ECC_B, SM2_ECC_N, SM2_ECC_H);
ECPoint g = curve.CreatePoint(SM2_ECC_GX, SM2_ECC_GY);
ECDomainParameters domainParams = new ECDomainParameters(curve, g, SM2_ECC_N);
ECPoint userkey = curve.DecodePoint(publicKey);
ECPublicKeyParameters aPub = new ECPublicKeyParameters(userkey, domainParams);
SM2Engine sm2Engine = new SM2Engine();
sm2Engine.Init(true, new ParametersWithRandom(aPub));
byte[] enc = sm2Engine.ProcessBlock(source, 0, source.Length);
return Encoding.UTF8.GetString(Hex.Encode(enc));
}
/// <summary>
/// 解密函数
/// </summary>
/// <param name="privateKey"></param>
/// <param name="encryptedData"></param>
/// <returns></returns>
public string Decrypt(string privateKeyStr, string chiperText)
{
byte[] privateKey = Hex.Decode(privateKeyStr);
byte[] encryptedData = Hex.Decode(chiperText);
if (null == privateKey || privateKey.Length == 0)
{
return null;
}
if (encryptedData == null || encryptedData.Length == 0)
{
return null;
}
byte[] enc = new byte[encryptedData.Length];
Array.Copy(encryptedData, 0, enc, 0, encryptedData.Length);
BigInteger userD = new BigInteger(1, privateKey);
ECCurve curve = new FpCurve(SM2_ECC_P, SM2_ECC_A, SM2_ECC_B, SM2_ECC_N, SM2_ECC_H);
ECPoint g = curve.CreatePoint(SM2_ECC_GX, SM2_ECC_GY);
ECDomainParameters domainParams = new ECDomainParameters(curve, g, SM2_ECC_N);
ECPrivateKeyParameters aPriv = new ECPrivateKeyParameters(userD, domainParams);
SM2Engine sm2Engine = new SM2Engine();
sm2Engine.Init(false, aPriv);
byte[] dec = sm2Engine.ProcessBlock(enc, 0, enc.Length);
return Encoding.UTF8.GetString(dec);
}
}
}
using Org.BouncyCastle.Crypto;
using Org.BouncyCastle.Crypto.Engines;
using Org.BouncyCastle.Crypto.Generators;
using Org.BouncyCastle.Crypto.Parameters;
using Org.BouncyCastle.Math;
using Org.BouncyCastle.Math.EC;
using Org.BouncyCastle.Security;
using Org.BouncyCastle.Utilities.Encoders;
using System;
using System.Text;
namespace SMDemo
{
public class SM2KeyPair
{
public string PrivateKey { get; set; } //私钥
public string PublicKey { get; set; } //公钥
}
public class SM2Utils
{
//国密标准256位曲线参数
BigInteger SM2_ECC_P = new BigInteger("FFFFFFFEFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFF00000000FFFFFFFFFFFFFFFF", 16);
BigInteger SM2_ECC_A = new BigInteger("FFFFFFFEFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFFF00000000FFFFFFFFFFFFFFFC", 16);
BigInteger SM2_ECC_B = new BigInteger("28E9FA9E9D9F5E344D5A9E4BCF6509A7F39789F515AB8F92DDBCBD414D940E93", 16);
BigInteger SM2_ECC_N = new BigInteger("FFFFFFFEFFFFFFFFFFFFFFFFFFFFFFFF7203DF6B21C6052B53BBF40939D54123", 16);
BigInteger SM2_ECC_H = BigInteger.One;
BigInteger SM2_ECC_GX = new BigInteger("32C4AE2C1F1981195F9904466A39C9948FE30BBFF2660BE1715A4589334C74C7", 16);
BigInteger SM2_ECC_GY = new BigInteger("BC3736A2F4F6779C59BDCEE36B692153D0A9877CC62A474002DF32E52139F0A0", 16);
public SM2KeyPair GenerateKey()
{
ECCurve curve = new FpCurve(SM2_ECC_P, SM2_ECC_A, SM2_ECC_B, SM2_ECC_N, SM2_ECC_H);
ECPoint g = curve.CreatePoint(SM2_ECC_GX, SM2_ECC_GY);
ECDomainParameters domainParams = new ECDomainParameters(curve, g, SM2_ECC_N);
ECKeyPairGenerator keyPairGenerator = new ECKeyPairGenerator();
ECKeyGenerationParameters aKeyGenParams = new ECKeyGenerationParameters(domainParams, new SecureRandom());
keyPairGenerator.Init(aKeyGenParams);
AsymmetricCipherKeyPair aKp = keyPairGenerator.GenerateKeyPair();
ECPublicKeyParameters aPub = (ECPublicKeyParameters)aKp.Public;
ECPrivateKeyParameters aPriv = (ECPrivateKeyParameters)aKp.Private;
BigInteger privateKey = aPriv.D;
ECPoint publicKey = aPub.Q;
byte[] pubkey = Hex.Encode(publicKey.GetEncoded());
string temp_pubkey = Encoding.UTF8.GetString(pubkey);
string temp_prikey = Encoding.UTF8.GetString(Hex.Encode(privateKey.ToByteArray()));
return new SM2KeyPair() { PrivateKey = temp_prikey, PublicKey = temp_pubkey };
}
/// <summary>
/// 加密函数
/// </summary>
/// <param name="publicKeyStr"></param>
/// <param name="plainText"></param>
/// <returns></returns>
public string Encrypt(string publicKeyStr, string plainText)
{
byte[] publicKey = Hex.Decode(publicKeyStr);
byte[] data = Encoding.UTF8.GetBytes(plainText);
if (null == publicKey || publicKey.Length == 0)
{
return null;
}
if (data == null || data.Length == 0)
{
return null;
}
byte[] source = new byte[data.Length];
Array.Copy(data, 0, source, 0, data.Length);
ECCurve curve = new FpCurve(SM2_ECC_P, SM2_ECC_A, SM2_ECC_B, SM2_ECC_N, SM2_ECC_H);
ECPoint g = curve.CreatePoint(SM2_ECC_GX, SM2_ECC_GY);
ECDomainParameters domainParams = new ECDomainParameters(curve, g, SM2_ECC_N);
ECPoint userkey = curve.DecodePoint(publicKey);
ECPublicKeyParameters aPub = new ECPublicKeyParameters(userkey, domainParams);
SM2Engine sm2Engine = new SM2Engine();
sm2Engine.Init(true, new ParametersWithRandom(aPub));
byte[] enc = sm2Engine.ProcessBlock(source, 0, source.Length);
return Encoding.UTF8.GetString(Hex.Encode(enc));
}
/// <summary>
/// 解密函数
/// </summary>
/// <param name="privateKey"></param>
/// <param name="encryptedData"></param>
/// <returns></returns>
public string Decrypt(string privateKeyStr, string chiperText)
{
byte[] privateKey = Hex.Decode(privateKeyStr);
byte[] encryptedData = Hex.Decode(chiperText);
if (null == privateKey || privateKey.Length == 0)
{
return null;
}
if (encryptedData == null || encryptedData.Length == 0)
{
return null;
}
byte[] enc = new byte[encryptedData.Length];
Array.Copy(encryptedData, 0, enc, 0, encryptedData.Length);
BigInteger userD = new BigInteger(1, privateKey);
ECCurve curve = new FpCurve(SM2_ECC_P, SM2_ECC_A, SM2_ECC_B, SM2_ECC_N, SM2_ECC_H);
ECPoint g = curve.CreatePoint(SM2_ECC_GX, SM2_ECC_GY);
ECDomainParameters domainParams = new ECDomainParameters(curve, g, SM2_ECC_N);
ECPrivateKeyParameters aPriv = new ECPrivateKeyParameters(userD, domainParams);
SM2Engine sm2Engine = new SM2Engine();
sm2Engine.Init(false, aPriv);
byte[] dec = sm2Engine.ProcessBlock(enc, 0, enc.Length);
return Encoding.UTF8.GetString(dec);
}
}
}
Sm3Utils.cs
using Org.BouncyCastle.Crypto.Digests;
using Org.BouncyCastle.Utilities.Encoders;
using System;
using System.Text;
namespace SMDemo
{
public class Sm3Utils
{
public static string GetHash(string plainText)
{
String resultHexString = "";
// 将字符串转换成byte数组
byte[] srcData = Encoding.UTF8.GetBytes(plainText);
SM3Digest digest = new SM3Digest();
digest.BlockUpdate(srcData, 0, srcData.Length);
byte[] hash = new byte[digest.GetDigestSize()];
digest.DoFinal(hash, 0);
// 将返回的hash值转换成16进制字符串
resultHexString = new UTF8Encoding().GetString(Hex.Encode(hash));
return resultHexString;
}
}
}
Sm4Utils.cs
using Org.BouncyCastle.Crypto;
using Org.BouncyCastle.Crypto.Parameters;
using Org.BouncyCastle.Security;
using Org.BouncyCastle.Utilities.Encoders;
using System.Text;
namespace SMDemo
{
public class Sm4Utils
{
public static string EncryptEBC(string plaintext, string key)
{
var dataBytes = Encoding.UTF8.GetBytes(plaintext);
var keyBytes = Encoding.UTF8.GetBytes(key);
KeyParameter keyParam = ParameterUtilities.CreateKeyParameter("SM4", keyBytes);
IBufferedCipher inCipher = CipherUtilities.GetCipher("SM4/ECB/PKCS7Padding");
inCipher.Init(true, keyParam);
byte[] cipher = inCipher.DoFinal(dataBytes);
return Hex.ToHexString(cipher, 0, cipher.Length);
}
public static string DecryptEBC(string chipherText, string key)
{
var dataBytes = Hex.Decode(chipherText);
var keyBytes = Encoding.UTF8.GetBytes(key);
KeyParameter keyParam = ParameterUtilities.CreateKeyParameter("SM4", keyBytes);
IBufferedCipher inCipher = CipherUtilities.GetCipher("SM4/ECB/PKCS7Padding");
inCipher.Init(false, keyParam);
byte[] plain = inCipher.DoFinal(dataBytes);
return Encoding.UTF8.GetString(plain);
}
/// <summary>
/// CBC模式加密
/// </summary>
/// <param name="data"></param>
/// <param name="key"></param>
/// <returns></returns>
public static string EncryptCBC(string plaintext, string key)
{
var dataBytes = Encoding.UTF8.GetBytes(plaintext);
var keyBytes = Encoding.UTF8.GetBytes(key);
KeyParameter keyParam = ParameterUtilities.CreateKeyParameter("SM4", keyBytes);
ParametersWithIV keyParamWithIv = new ParametersWithIV(keyParam, keyBytes);
IBufferedCipher inCipher = CipherUtilities.GetCipher("SM4/CBC/PKCS7Padding");
inCipher.Init(true, keyParamWithIv);
byte[] cipher = inCipher.DoFinal(dataBytes);
return Hex.ToHexString(cipher, 0, cipher.Length);
}
/// <summary>
/// CBC模式解密
/// </summary>
/// <param name="data"></param>
/// <param name="key"></param>
/// <returns></returns>
public static string DecryptCBC(string chipherText, string key)
{
var dataBytes = Hex.Decode(chipherText);
var keyBytes = Encoding.UTF8.GetBytes(key);
KeyParameter keyParam = ParameterUtilities.CreateKeyParameter("SM4", keyBytes);
ParametersWithIV keyParamWithIv = new ParametersWithIV(keyParam, keyBytes);
IBufferedCipher inCipher = CipherUtilities.GetCipher("SM4/CBC/PKCS7Padding");
inCipher.Init(false, keyParamWithIv);
byte[] plain = inCipher.DoFinal(dataBytes);
return Encoding.UTF8.GetString(plain);
}
}
}