Problem: 86. 分隔链表
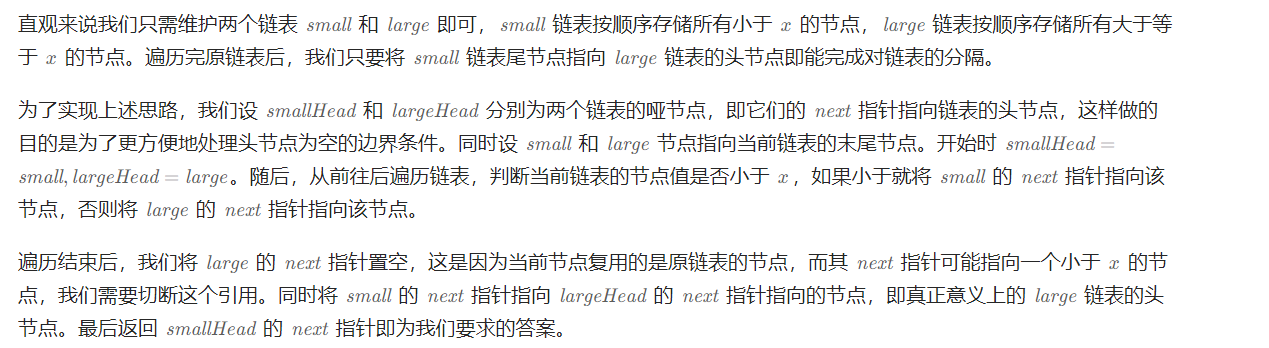
Code
Java
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode partition(ListNode head, int x) {
// 创建两个哑节点,用于存储小于x和大于等于x的节点
ListNode small = new ListNode(0);
ListNode smallHead = small; // small链表的头指针
ListNode large = new ListNode(0);
ListNode largeHead = large; // large链表的头指针
// 遍历原始链表
while (head != null) {
if (head.val < x) {
// 如果当前节点值小于x,将该节点连接到small链表
small.next = head;
small = small.next; // 更新small指针到当前节点
} else {
// 如果当前节点值大于等于x,将该节点连接到large链表
large.next = head;
large = large.next; // 更新large指针到当前节点
}
// 移动到下一个节点
head = head.next;
}
// large链表的最后一个节点需要指向null,以防止形成循环链表
large.next = null;
// 将small链表的最后一个节点指向large链表的头节点
small.next = largeHead.next;
// 返回small链表的头节点,即分隔后的新链表的头节点
return smallHead.next;
}
}