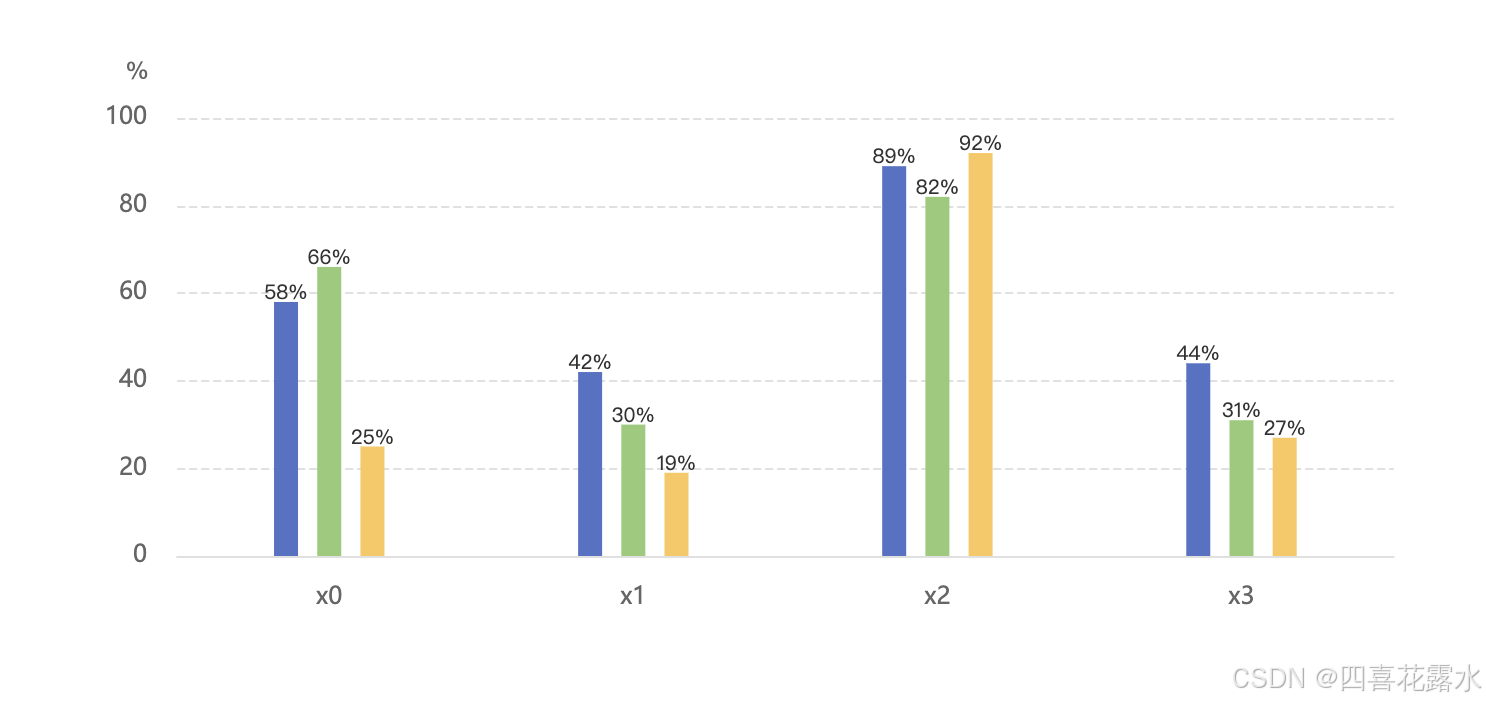
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="http://libs.baidu.com/jquery/2.0.0/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/echarts.min.js"></script>
<style>
.chart-item {
width: 50%;
height: 300px;
margin: 48px auto;
}
</style>
</head>
<body>
<div class="chart-item" id="chart"></div>
<script>
// 提取
// 把会重复用到的部分提取出来,可以封装到另一个js文件中
var commonTool = {
opt: {
axis: {
splitLine: {
show: true,
lineStyle: {
type: 'dashed',
color: "#e0e0e0",
width: 1
}
},
axisLine: {
show: true,
lineStyle: {
color: "#e0e0e0",
width: 1
}
},
axisLabel: function (opt) {
var defaulte = {
textStyle: {
color: "#666",
fontSize: 12
},
fontFamily: "Microsoft YaHei",
margin: 15
};
var opt = $.extend("", defaulte, opt);
return opt;
},
}
}
}
// 提取 END
var myChartArr = [];
function drawChart(id, data) {
var myEchart = echarts.init(document.getElementById(id));
var option = {
tooltip: {
trigger: "axis",
backgroundColor: "rgba(0,0,0,0.66)",
borderColor: 'rgba(0,0,0,0)',
axisPointer: {
type: "shadow",
label: {
show: false,
},
},
textStyle: {
color: '#fff',
fontSize: 12,
},
},
grid: {
left: "6%",
right: "3%",
bottom: "3%",
top: "15%",
containLabel: true,
z: 22,
},
xAxis: [{
type: "category",
data: data[0],
axisLabel: commonTool.opt.axis.axisLabel(),
axisLine: commonTool.opt.axis.axisLine,
axisTick: commonTool.opt.axis.axisTick,
z: 1
}],
yAxis: [{
type: "value",
name: "%",
nameTextStyle: {
color: '#666',
fontSize: 12,
padding: [0, 40, 3, 0]
},
axisLabel: commonTool.opt.axis.axisLabel(),
splitLine: commonTool.opt.axis.splitLine,
splitArea: {
show: false
},
axisTick: {
show: false
},
axisLine: {
show: false,
},
z: 1
}],
series: [{
name: "item1",
type: "bar",
barWidth: "12",
barGap: "80%",
data: data[1],
label: {
normal: {
show: true,
position: 'top',
color: "#333",
distance: 0,
formatter: '{c}%',
fontSize: 10
}
},
z: 1
}, {
name: "item2",
type: "bar",
barWidth: "12",
barGap: "80%",
data: data[2],
label: {
normal: {
show: true,
position: 'top',
color: "#333",
distance: 0,
formatter: '{c}%',
fontSize: 10
}
},
z: 1
}, {
name: "item3",
type: "bar",
barWidth: "12",
barGap: "80%",
data: data[3],
label: {
normal: {
show: true,
position: 'top',
color: "#333",
distance: 0,
formatter: '{c}%',
fontSize: 10
}
},
z: 1
}]
};
myEchart.setOption(option)
myChartArr.push(myEchart)
}
var dataX = [],
dataX2 = [],
dataY1 = [],
dataY2 = [],
dataY3 = [];
for (var i = 0; i < 4; i++) {
dataX.push("x" + i);
dataY1.push(Math.ceil(Math.random() * 1000 % 100));
dataY2.push(Math.ceil(Math.random() * 1000 % 100));
dataY3.push(Math.ceil(Math.random() * 1000 % 100));
}
drawChart('chart', [dataX, dataY1, dataY2, dataY3])
$(window).resize(function () {
for (var i = 0; i < myChartArr.length; i++) {
myChartArr[i].resize();
}
})
</script>
</body>
</html>