在 C++ 中,数组是一种基本的数据结构,用于存储固定大小的相同类型的元素。数组在声明时必须指定其大小,并且一旦创建,其大小就不可改变。数组的索引从 0 开始。
int myArray[5]; // 声明一个整型数组,包含5个元素int anotherArray[5] = {1, 2, 3, 4, 5}; // 声明并初始化一个整型数组
虽然传统的 C++ 数组在声明时大小固定,但 C++ 标准库提供了 std::vector 类,它是一个动态数组,可以在运行时调整大小。
#include <vector>std::vector<int> myVector; // 声明一个动态数组myVector.push_back(10); // 添加元素
在 C++ 中,没有内置的函数直接对应于 NumPy 的 linspace 或 arange,但可以通过结合使用标准库中的函数来实现类似的功能。以下是两种方法:
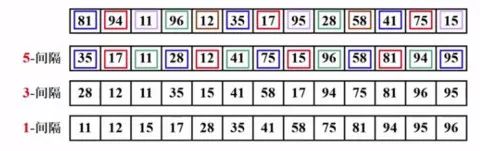
1. 使用 std::vector 和循环来创建等间隔的数组(类似 linspace)
#include <iostream>#include <vector>#include <algorithm> // for std::transformstd::vector<double> linspace(double start, double end, int num) { std::vector<double> linspaced; if (num == 0) { return linspaced; } if (num == 1) { linspaced.push_back(start); return linspaced; } double delta = (end - start) / (num - 1); linspaced.push_back(start); std::generate_n(std::back_inserter(linspaced), num - 1, [&]() { double next = start + delta; delta = (end - start) / (num - 1); start = next; return next; }); linspaced.push_back(end); // Ensure the end is included return linspaced;}int main() { auto vec = linspace(0, 1, 5); for (auto v : vec) { std::cout << v << " "; } std::cout << std::endl; return 0;}
2. 使用 std::vector 和 std::iota 来创建一个序列(类似 arange)
在 C++11 之前,std::iota 是定义在 <algorithm> 头文件中的,但 C++11 之后,它被移到了 <numeric>。
#include <iostream>#include <vector>#include <numeric> // for std::iotastd::vector<int> arange(int start, int end, int step) { std::vector<int> range; range.reserve((end - start) / step + 1); // 预分配足够的空间 int value = start; while (value < end) { range.push_back(value); value += step; } return range;}int main() { auto vec = arange(0, 10, 2); for (auto v : vec) { std::cout << v << " "; } std::cout << std::endl; return 0;}
补充:
-
**np.linspace(start, stop, num):**返回一个从 start 开始到 stop 结束的等间隔数字的数组,包括结束值,总共 num 个数字。
-
**np.arange(start, stop, step):**返回一个从 start 开始到 stop 结束(不包括 stop),以 step 为步长的等间隔数字的数组。